def show(self): st_display = st.container() st_display.header(self.title) st_configs = st.container() menu = st.sidebar.expander(self.title) group_identical_res = GroupIdenticalResults(self.data, self.title) with menu: group_identical_res.show() show_config_list = st.checkbox( "Show configurations list", False, key=f"Show configurations list {self.title}", ) config_select = ConfigurationsSelection(group_identical_res.data, self.title) if show_config_list: with st_configs: config_select.show() res_list = list( map(lambda d: d.pop("results"), copy.deepcopy(config_select.data))) names = config_select.config_names colors = plt.get_cmap("gnuplot")(np.linspace(0.1, 0.80, len(names))) with menu: results = self.prepare(res_list) with st_display: self._display(results, names, colors)
def test_nested_with(self): with st.container(): with st.container(): st.markdown("Level 2 with") msg = self.get_message_from_queue() self.assertEqual( make_delta_path(RootContainer.MAIN, (0, 0), 0), msg.metadata.delta_path, ) st.markdown("Level 1 with") msg = self.get_message_from_queue() self.assertEqual( make_delta_path(RootContainer.MAIN, (0, ), 1), msg.metadata.delta_path, )
def test_container_paths(self): level3 = st.container().container().container() level3.markdown("hi") level3.markdown("bye") msg = self.get_message_from_queue() self.assertEqual(make_delta_path(RootContainer.MAIN, (0, 0, 0), 1), msg.metadata.delta_path)
def wrapper(*args, **kwargs): auth_status = get_auth_status() if auth_status ==1: return run_func(*args, **kwargs) else: st_asset = st.container() st_asset.warning(f'please rerun app (press r) after login') return interactive_login(st_asset = st_asset, b_show_logo = False)
def app(): header = st.container() body = st.container() activities = st.container() github = st.container() with header: st.title('Preprocessing Galaxy-data') # site title h1 st.text(' ') st.text(' ') st.markdown("Lets check data in galaxy file :sparkles:") st.text(' ') # Load 10,000 rows of data into the dataframe. data = load_data("galaxy") data_load_state = st.text('Loading data...') st.write(data.head(10)) # Notify the reader that the data was successfully loaded. data_load_state.text('Loading data...done!') st.text(' ') with body: st.title('Procedures') st.markdown( '* Create model with 3 clusters KMeans adn fit the model ') st.markdown('* Find Clusters') st.markdown('* Separete cluster from each other ') st.markdown('* Make a scatter plot to see ') st.text(' ') st.subheader(' All galaxy ') image = Image.open('./graphics/gaalxy_all.png') st.image(image, caption="") st.header('* What is next ') st.markdown( "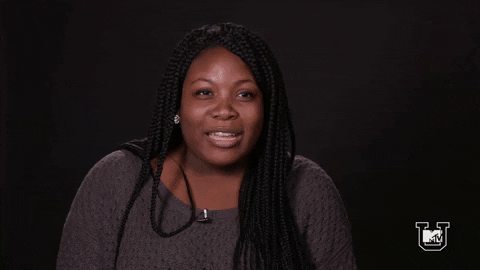" ) st.markdown('* Find the planet where the most righ side ') st.markdown('* We find the planet ') image = Image.open('./graphics/beby.png') st.image(image, caption="") st.markdown("* Let's go") st.markdown( "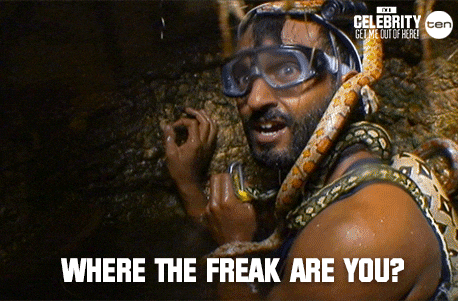" )
def test_deep_implicit_form_parent(self): """Within a `with form` statement, any `st.foo` element becomes part of that form, regardless of how deeply nested the element is.""" with st.form("form"): cols1 = st.columns(2) with cols1[0]: with st.container(): st.checkbox("widget") self.assertEqual("form", self._get_last_checkbox_form_id()) # The sidebar, and any other DG parent created outside # the form, does not create children inside the form. with st.form("form2"): cols1 = st.columns(2) with cols1[0]: with st.container(): st.sidebar.checkbox("widget2") self.assertEqual(NO_FORM_ID, self._get_last_checkbox_form_id())
def _create_layout(self) -> dict: pgbar = st.empty() summary = st.container() summcol, _, pathcol = summary.columns([3, 1, 10]) with summary: with summcol: summ_ph = st.empty() legend_ph = st.container() with pathcol: fw_ph = st.empty() table_expander = st.empty() failed_tables = st.empty() return ({ 'pgbar': pgbar, 'summary': summ_ph, 'legend': legend_ph, 'fw_path': fw_ph, 'table': table_expander, 'failed_tables': failed_tables, })
def mainpage(): st.image( 'https://images.unsplash.com/photo-1530099486328-e021101a494a?ixlib=rb-1.2.1&ixid=MnwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8&auto=format&fit=crop&w=1247&q=80' ) with st.container(): st.write( "This data set consists of Placement data of students in a XYZ campus. It includes secondary and higher secondary school percentage and specialization. It also includes degree specialization, type and Work experience and salary offers to the placed students" ) st.write( "https://www.kaggle.com/benroshan/factors-affecting-campus-placement" )
def main(): st.set_page_config(page_title="NGUYEN APPS", page_icon='logo.jpg', layout='centered', initial_sidebar_state='auto') st.markdown( f""" <style> .reportview-container .main .block-container{{ max-width: 90%; }} </style> """, unsafe_allow_html=True, ) st.sidebar.title("Navigation") st.sidebar.header("App Selection") goto = st.sidebar.radio('Go to:',['NGUYEN=MC2', 'I DRAW NGUYEN']) if goto == 'I DRAW NGUYEN': with st.container(): st.title("I DRAW NGUYEN: Interactive Depth Residual Analysis With NGUYEN") st.header("Visualization App for QC & editing depth misties(Delta-Z) between horizons and well tops") st.subheader('Parameter Selections:') with st.spinner(text='Data loading in progress... Please wait!'): time.sleep(1) idrawu() else: with st.container(): st.title('NGUYEN=MC2: Non-Gaussian UncertaintY EstimatioN by MCMC*') st.header("V0,K and Uncertainty Estimation using MCMC - Markov chain Monte Carlo method") #CSS to display content correctly st.subheader('Parameter Selections:') v0kmcmc() st.sidebar.info( "**Created by:** [KHANH NGUYEN ](mailto:[email protected])" "**©️**2️⃣0️⃣2️⃣0️⃣" ) return None
def show_statistics(): st.subheader('Statistics') st.plotly_chart(genderchart(df)) st.subheader('SSC Percentage') col1, col2 = st.columns(2) with st.container(): col1.plotly_chart(ssc_pchart(df)) with st.container(): col2.plotly_chart(ssc_p_piechart(df)) st.subheader('Degree Type') st.plotly_chart(degree_chart(df)) st.subheader('HSC Percentage') st.plotly_chart(hsc_pchart(df)) st.subheader('Status') st.plotly_chart(status_chart(df)) st.subheader('Salary Distribution') st.plotly_chart(salary_histogram(df)) st.plotly_chart(salary_scatter(df)) st.subheader('Education Percentage') st.plotly_chart(sscb_bar(df)) st.plotly_chart(hsc_p_bar(df)) st.plotly_chart(degree_p_bar(df)) st.plotly_chart(etest_p_bar(df))
def test_with(self): # Same as test_container_paths, but using `with` syntax level3 = st.container().container().container() with level3: st.markdown("hi") st.markdown("bye") msg = self.get_message_from_queue() self.assertEqual(make_delta_path(RootContainer.MAIN, (0, 0, 0), 1), msg.metadata.delta_path) # Now we're out of the `with` block, commands should use the main dg st.markdown("outside") msg = self.get_message_from_queue() self.assertEqual(make_delta_path(RootContainer.MAIN, (), 1), msg.metadata.delta_path)
def show(self): db_selection = DatabaseSelection(self.db) with st.sidebar.expander("Select benchmark criterias"): db_selection.show() views = { "Profile": ProfileView, "Search": SearchView, "PercUtil": PercUtilView, "Table": TableView, "Compare": ComparatorView, } view_selection = ViewSelection(db_selection.data, views) with st.sidebar.expander("Select views to display"): view_selection.show() for view in view_selection.selected_views: with st.container(): view.show()
def test_parent_created_inside_form(self): """If a parent DG is created inside a form, any children of that parent belong to the form.""" with st.form("form"): with st.container(): # Create a (deeply nested) column inside the form form_col = st.columns(2)[0] # Attach children to the column in various ways. # They'll all belong to the form. with form_col: st.checkbox("widget1") self.assertEqual("form", self._get_last_checkbox_form_id()) form_col.checkbox("widget2") self.assertEqual("form", self._get_last_checkbox_form_id()) form_col.checkbox("widget3") self.assertEqual("form", self._get_last_checkbox_form_id())
def show(self): # Get the selectable caracteristics from the database headers = list(map(lambda x: dict(x), self.db.all())) criterias = {} not_criterias = [ ["results"], ] default_ignore = [ ["summary", "description"], ["summary", "date"], ["parameters", "random_state"], ] list(map(partial(_merge_dict_in, criterias, not_criterias), headers)) # Show the possible criterias with st.container(): choices, to_ignore = self._select_choices(criterias, default_ignore) # Select the corresponding runs query = self._generate_query(choices) self.data = list(map(lambda x: dict(x), self.db.search(query))) for path in to_ignore: for entry in self.data: try: item = reduce(dict.get, path[:-1], entry) except: item = None if item: del item[path[-1]] select_size = len(self.data) message = "{nb} benchmark{s} found.".format( nb=select_size, s="s" if select_size > 1 else "") if select_size != 0: st.info(message) else: st.warning(message)
def _create_layout(self) -> None: grid1 = st.container() with grid1: headercol, uniq_col = st.columns(2) header_ph = headercol.empty() uniq_ph = uniq_col.container() scol1, scol2 = st.columns(2) scol1_ph = scol1.empty() scol2_ph = scol2.empty() if self._state.table in ['interfaces', 'ospf', 'bgp', 'evpnVni']: validate_ph = st.empty() else: validate_ph = None table_ph = st.empty() return { 'header': header_ph, # summary header 'uniq_col': uniq_ph, # unique column selector 'summary': scol1_ph, # summary table 'uniq': scol2_ph, # unique table 'assert': validate_ph, # assert expander 'table': table_ph, # table dataframe expander }
def view(model): st.set_page_config(layout='wide') ## Header st.header(model.header) commentaryCol, spaceCol, chartCol = st.columns((2, 1, 6)) # Description with commentaryCol: with st.container(): st.write('') st.write('') st.write(model.description) ## Year Slider st.write('') st.write('') year = st.slider(model.sliderCaption, model.yearStart, model.yearEnd, model.yearStart, model.yearStep) #Chart with chartCol: st.plotly_chart(model.chart(year), use_container_width=True)
def chi_file_upload(): """ Python code adapted from Brownlee (June 15, 2018) """ st.title('chi2 Test of Homogeneity') st.write( 'This chi2 calculator assumes that your data is in the form of a contingency table:' ) st.markdown(""" |values|sample 1|sample 2| |-------|------|------| |val1|30|30| |val2|20|30| |val3|40|15| |val4|24|20| """) st.write('To use the chi2 calculator:') st.write(""" 1. Input the significance value. 2. Upload your frequency table as an .csv or .xlsx file. Make sure that the column names for your two samples are "sample 1" and "sample 2." """) significance = float( st.text_input('Input significance value (default/max value is .05)', value='.05')) uploaded = st.file_uploader('Upload your .csv or .xlsx file.') st.caption("📝 This app does not retain user data.") if uploaded != None: if uploaded.name.endswith('csv'): df = pd.read_csv(uploaded) s1 = [int(c) for c in df['sample 1']] s2 = [int(c) for c in df['sample 2']] chi, p_val, dof, ex = chi2_contingency([s1, s2], correction=False) p = 1 - significance crit_val = chi2.ppf(p, dof) st.subheader('Results') st.write('Uploaded Contingency Table:') st.write(df) c1 = st.container() c2, c3, c4, c5 = st.columns(4) c1.metric('p-value', str(p_val)) c2.metric('# of Samples', str(len(s1))) c3.metric('Degrees of Freedom', "{:.2f}".format(dof)) c4.metric('\n chi2 test statistic', "{:.5f}".format(chi)) c5.metric('critical value', "{:.5f}".format(crit_val)) st.write( "For an extended discussion of using chi2 tests for homogeneity for qualitative coding, see [Geisler and Swarts (2019)](https://wac.colostate.edu/docs/books/codingstreams/chapter9.pdf)" ) elif uploaded.name.endswith('xlsx'): df = pd.read_excel(uploaded) s1 = [int(c) for c in df['sample 1']] s2 = [int(c) for c in df['sample 2']] chi, p_val, dof, ex = chi2_contingency([s1, s2], correction=False) p = 1 - significance crit_val = chi2.ppf(p, dof) st.subheader('Results') st.write('Uploaded Contingency Table:') st.write(df) c1 = st.container() c2, c3, c4, c5 = st.columns(4) c1.metric('p-value', str(p_val)) c2.metric('# of Samples', str(len(s1))) c3.metric('Degrees of Freedom', "{:.2f}".format(dof)) c4.metric('\n chi2 test statistic', "{:.5f}".format(chi)) c5.metric('critical value', "{:.5f}".format(crit_val)) st.write( "For an extended discussion of using chi2 tests for homogeneity for qualitative coding, see [Geisler and Swarts (2019)](https://wac.colostate.edu/docs/books/codingstreams/chapter9.pdf)" )
def chi(): """ Python code adapted from Brownlee (June 15, 2018) """ st.title('chi2 Test of Homogeneity') st.write( 'This chi2 calculator assumes that your data takes the form of a frequency table:' ) st.markdown(""" |sample|value1|value2|value3| |------|------|------|------| |sample1|10|20|30| |sample2|10|15|25| """) st.caption("In this case, you copy and paste row-wise values") st.markdown('Or') st.markdown(""" |value|sample 1|sample 2| |-----|--------|--------| |value1|10|10| |value2|20|15| |value3|30|25| """) st.caption("In this case, you copy and paste column-wise values") st.write( 'The chi2 calculator accepts the first row of your data in the Sample 1 field and the second row of your data in the Sample 2 field.' ) st.write('To use the chi2 calculator:') st.write(""" 1. Input the significant value (default/max value is .05) 2. Copy the values of your first sample and paste into the Sample 1 text entry field and hit "Enter." 3. Copy the values for your second sample and paste into the Sample 2 text entry field and hit "Enter." ❗Samples 1 and Sample 2 must be numerical values. """) significance = float( st.text_input('Input significance value (default/max value is .05)', value='.05')) col1 = st.text_input('Sample 1', value='10 20 30') col2 = st.text_input('Sample 2', value='10 15 25') st.caption("📝 This app does not retain user data.") s1 = [int(c) for c in col1.split()] s2 = [int(c) for c in col2.split()] chi, p_val, dof, ex = chi2_contingency([s1, s2], correction=False) p = 1 - significance crit_val = chi2.ppf(p, dof) st.subheader('Results') c1 = st.container() c2, c3, c4, c5 = st.columns(4) c1.metric('p-value', str(p_val)) c2.metric('Dataset Length', str(len(s1))) c3.metric('degree of freedom', "{:.2f}".format(dof)) c4.metric('\n chi2 test statistic', "{:.5f}".format(chi)) c5.metric('critical value', "{:.5f}".format(crit_val)) st.write( "For an extended discussion of using chi2 tests for homogeneity for qualitative coding, see [Geisler and Swarts (2019)](https://wac.colostate.edu/docs/books/codingstreams/chapter9.pdf)" )
model = load(filename=filename) return model def encode_Yes(inpt): if inpt == 'Yes': return 1 else: return 0 main_df = get_data() model = get_model('HRR.joblib') header = st.container() dataset = st.container() visualization = st.container() prediction = st.container() with st.sidebar: st.header('Select Input data to predict') no_proj = st.slider('Number of project by employee?', min_value=main_df['number_project'].min() - 1, max_value=main_df['number_project'].max() + 3, value=2, step=1) avr_mothly_hrs = st.slider( 'Average monthly working hours?',
# Copyright 2018-2022 Streamlit Inc. # # Licensed under the Apache License, Version 2.0 (the "License"); # you may not use this file except in compliance with the License. # You may obtain a copy of the License at # # http://www.apache.org/licenses/LICENSE-2.0 # # Unless required by applicable law or agreed to in writing, software # distributed under the License is distributed on an "AS IS" BASIS, # WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. # See the License for the specific language governing permissions and # limitations under the License. import streamlit as st container = st.container() st.write("Line 1") container.write("Line 2") with container: "Line 3" st.write("Line 4") # Ensure widget states persist when React nodes shift if st.button("Step 2: Press me"): st.header("Pressed!") c = st.container() if c.checkbox("Step 1: Check me"): c.title("Checked!")
"../results/twitter15/debug/0/for_demo_final/gen_result", post_id + ".txt"), sep="\t") st.sidebar.subheader("Post Label") label_text = num_to_text(gen_data["ground-truth"][0]) label_color = num_to_color(gen_data["ground-truth"][0]) text = f"<div> <span class='highlight {label_color} bold'> {label_text} </span></div>" st.sidebar.markdown(text, unsafe_allow_html=True) st.sidebar.write("") st.sidebar.write("") st.sidebar.subheader("Post Content") st.sidebar.json(json_data) with st.container(): col1, _, col2 = st.columns([1, 0.1, 1]) with col1: st.subheader("Data Visualization") # Graph visualization HtmlFile = open("data.html", 'r', encoding='utf-8') source_code = HtmlFile.read() components.html(source_code, height=500, width=570) with col2: st.subheader("Prediction") with st.container(): text = '<p style="font-size:20px;"> Without Attack </p>' st.markdown(text, unsafe_allow_html=True)
def app(): # Preprocessing average curve aft_slow_list = list(aft_median[aft_median['0'] >= 41.537768]['SubjectID']) aft_intermediate_list = list( aft_median[(aft_median['0'] >= 18.924361) & (aft_median['0'] < 41.537768)]['SubjectID']) aft_rapid_list = list(aft_median[aft_median['0'] < 18.924361]['SubjectID']) X_aft_slow = df_train[df_train['SubjectID'].isin( aft_slow_list)][aft_final_feature_list] X_aft_intermediate = df_train[df_train['SubjectID'].isin( aft_intermediate_list)][aft_final_feature_list] X_aft_rapid = df_train[df_train['SubjectID'].isin( aft_rapid_list)][aft_final_feature_list] X_aft_full = df_train[aft_final_feature_list] result_aft_slow = pd.DataFrame( aft.predict_survival_function(X_aft_slow.iloc[:, :]).mean(axis=1)) result_aft_intermediate = pd.DataFrame( aft.predict_survival_function( X_aft_intermediate.iloc[:, :]).mean(axis=1)) result_aft_rapid = pd.DataFrame( aft.predict_survival_function(X_aft_rapid.iloc[:, :]).mean(axis=1)) result_aft_full = pd.DataFrame( aft.predict_survival_function(X_aft_full.iloc[:, :]).mean(axis=1)) cph_slow_list = list( cph_median[cph_median['0.5'] >= 41.690000]['SubjectID']) cph_intermediate_list = list( cph_median[(cph_median['0.5'] >= 17.230000) & (cph_median['0.5'] < 41.690000)]['SubjectID']) cph_rapid_list = list( cph_median[cph_median['0.5'] < 17.230000]['SubjectID']) X_cph_slow = df_train[df_train['SubjectID'].isin( cph_slow_list)][cph_final_feature_list] X_cph_intermediate = df_train[df_train['SubjectID'].isin( cph_intermediate_list)][cph_final_feature_list] X_cph_rapid = df_train[df_train['SubjectID'].isin( cph_rapid_list)][cph_final_feature_list] X_cph_full = df_train[cph_final_feature_list] result_cph_slow = pd.DataFrame( cph.predict_survival_function(X_cph_slow.iloc[:, :]).mean(axis=1)) result_cph_intermediate = pd.DataFrame( cph.predict_survival_function( X_cph_intermediate.iloc[:, :]).mean(axis=1)) result_cph_rapid = pd.DataFrame( cph.predict_survival_function(X_cph_rapid.iloc[:, :]).mean(axis=1)) result_cph_full = pd.DataFrame( cph.predict_survival_function(X_cph_full.iloc[:, :]).mean(axis=1)) rsf_slow_list = list(rsf_median[rsf_median['0'] >= 74.610988]['SubjectID']) rsf_intermediate_list = list( rsf_median[rsf_median['0'] >= 16.73]['SubjectID']) rsf_rapid_list = list(rsf_median[rsf_median['0'] < 16.73]['SubjectID']) X_rsf_slow = df_train[df_train['SubjectID'].isin(rsf_slow_list)] X_rsf_intermediate = df_train[df_train['SubjectID'].isin( rsf_intermediate_list)] X_rsf_rapid = df_train[df_train['SubjectID'].isin(rsf_rapid_list)] X_rsf_full = df_train[rsf_final_feature_list] rsf_pred_slow = np.squeeze( rsf.predict_survival_function( X_rsf_slow[rsf_final_feature_list].iloc[:, :].to_numpy(), return_array=True)) rsf_pred_intermediate = np.squeeze( rsf.predict_survival_function( X_rsf_intermediate[rsf_final_feature_list].iloc[:, :].to_numpy(), return_array=True)) rsf_pred_rapid = np.squeeze( rsf.predict_survival_function( X_rsf_rapid[rsf_final_feature_list].iloc[:, :].to_numpy(), return_array=True)) rsf_pred_full = np.squeeze( rsf.predict_survival_function( X_rsf_full[rsf_final_feature_list].iloc[:, :].to_numpy(), return_array=True)) result_rsf_slow = np.transpose(pd.DataFrame(rsf_pred_slow)).set_index( rsf.event_times_) result_rsf_intermediate = np.transpose( pd.DataFrame(rsf_pred_intermediate)).set_index(rsf.event_times_) result_rsf_rapid = np.transpose(pd.DataFrame(rsf_pred_rapid)).set_index( rsf.event_times_) result_rsf_full = np.transpose(pd.DataFrame(rsf_pred_full)).set_index( rsf.event_times_) result_rsf_slow = pd.DataFrame(result_rsf_slow.mean(axis=1)) result_rsf_intermediate = pd.DataFrame( result_rsf_intermediate.mean(axis=1)) result_rsf_rapid = pd.DataFrame(result_rsf_rapid.mean(axis=1)) result_rsf_full = pd.DataFrame(result_rsf_full.mean(axis=1)) # loading navigation bar style st.markdown( '<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css" integrity="sha384-Gn5384xqQ1aoWXA+058RXPxPg6fy4IWvTNh0E263XmFcJlSAwiGgFAW/dAiS6JXm" crossorigin="anonymous">', unsafe_allow_html=True) # create blue navigation bar st.markdown(""" <nav class="navbar fixed-top navbar-expand-lg navbar-dark" style="background-color: #153888;"> <a class="navbar-brand" href="#" target="_blank">LASF prediction model</a> """, unsafe_allow_html=True) header = st.container() model_training = st.container() container = st.container() with model_training: # Create space for feature input in sidebar st.sidebar.title('Features') st.sidebar.write( 'If any values are unknown in user data, please input the existing default value.' ) st.markdown( """ <style> .sidebar .sidebar-content { background-image: linear-gradient(#DAE3F3,#DAE3F3); color: blue; } </style> """, unsafe_allow_html=True, ) Age = st.sidebar.text_input('Age (years)', value=56) mean_ALSFRS_R_Total = st.sidebar.slider( 'mean ALSFRS-R total score (points)', min_value=20, max_value=50, value=40, step=5) mean_bulbar = st.sidebar.slider('mean ALSFRS-R bulbar score (points)', min_value=5, max_value=10, value=8, step=1) mean_Q5_Cutting = st.sidebar.slider( 'mean ALSFRS-R Q5_Cutting (points)', min_value=1, max_value=4, value=2, step=1) mean_fvc = st.sidebar.slider('mean FVC (%)', min_value=10, max_value=100, value=70, step=10) mean_Creatinine = st.sidebar.slider('mean Creatinine (mmol/L)', min_value=10, max_value=100, value=50, step=10) onset_delta = st.sidebar.text_input('Time from onset (months)', value=-20) slope_ALSFRS_R_Total = st.sidebar.text_input( 'ALSFRS-R total slope (points per month)', value=-1) slope_bulbar = st.sidebar.text_input( 'ALSFRS-R bulbar slope (points per month)', value=-1) mean_weight = st.sidebar.text_input('mean weight (kg)', value=60) slope_weight = st.sidebar.text_input( 'weight change rate (kg per month)', value=-1) Age_calculated = (float(Age) - float(15)) // 5 # patient info filled with input value patient_aft = pd.DataFrame( { 'mean_bulbar': mean_bulbar, 'slope_bulbar': slope_bulbar, 'Age': Age_calculated, 'mean_fvc': mean_fvc, 'onset_delta': onset_delta, 'slope_weight': slope_weight, 'slope_ALSFRS_R_Total': slope_ALSFRS_R_Total, 'mean_Q5_Cutting': mean_Q5_Cutting, 'mean_Creatinine': mean_Creatinine }, index=['Current patient']) patient_cph = pd.DataFrame( { 'mean_bulbar': mean_bulbar, 'slope_bulbar': slope_bulbar, 'slope_ALSFRS_R_Total': slope_ALSFRS_R_Total, 'Age': Age_calculated, 'slope_weight': slope_weight, 'onset_delta': onset_delta, 'mean_fvc': mean_fvc, 'mean_ALSFRS_R_Total': mean_ALSFRS_R_Total }, index=['Current patient']) patient_rsf = pd.DataFrame( { 'mean_bulbar': mean_bulbar, 'slope_ALSFRS_R_Total': slope_ALSFRS_R_Total, 'mean_ALSFRS_R_Total': mean_ALSFRS_R_Total, 'onset_delta': onset_delta, 'mean_fvc': mean_fvc, 'slope_weight': slope_weight, 'Age': Age_calculated, 'mean_weight': mean_weight }, index=['Current patient']) show_average = [] show_progressor = [] with container: option = st.selectbox( 'Select model type', ('Accelerated failure time', 'Cox proportional hazard', 'Random survival forests')) model_name_dic = { "Accelerated failure time": [aft, patient_aft, result_aft_full], "Cox proportional hazard": [cph, patient_cph, result_cph_full], "Random survival forests": [rsf, patient_rsf, result_rsf_full] } progressor_dic = { "Accelerated failure time": [result_aft_slow, result_aft_intermediate, result_aft_rapid], "Cox proportional hazard": [result_cph_slow, result_cph_intermediate, result_cph_rapid], "Random survival forests": [result_rsf_slow, result_rsf_intermediate, result_rsf_rapid] } selected_model = model_name_dic[option][0] selected_patient = model_name_dic[option][1] selected_average = model_name_dic[option][2] result_slow = progressor_dic[option][0] result_intermediate = progressor_dic[option][1] result_rapid = progressor_dic[option][2] check_col1, check_col2, empty_col = st.columns(3) with check_col1: check_average = st.checkbox('Show average curve') if check_average: show_average.append(1) with check_col2: check_group = st.checkbox('Show progressor group') if check_group: show_progressor.append(1) fig, ax = plt.subplots(figsize=(25, 14)) if show_average == [1] and show_progressor == [1]: ax = plt.plot(result_slow.index, result_slow[0], marker='None', color='#C0C0C0', linestyle="--", linewidth=1.8, label='Slow') ax = plt.plot(result_intermediate.index, result_intermediate[0], marker='None', color='#696969', linestyle="--", linewidth=1.8, label='Intermediate') ax = plt.plot(result_rapid.index, result_rapid[0], marker='None', color='k', linestyle="--", linewidth=1.8, label='Rapid') ax = plt.plot(selected_average.index, selected_average[0], marker='None', color='blue', linestyle="--", linewidth=1.8, label='Average') if selected_model == rsf: result_rsf = rsf.predict_survival_function(selected_patient, return_array=True) for i, s in enumerate(result_rsf): plt.step(rsf.event_times_, s, where="post") plt.legend(labels=list(patient_rsf.index)) plt.xlabel("Time in months") plt.ylim([0, 1.08]) st.pyplot(fig) else: result = selected_model.predict_survival_function(selected_patient) ax = sns.lineplot(data=result) ax.set(xlabel='Time in months', ylabel='S(t)') st.pyplot(fig) elif show_average == [1] and show_progressor == []: ax = plt.plot(selected_average.index, selected_average[0], marker='None', color='blue', linestyle="--", linewidth=1.8, label='Average') if selected_model == rsf: result_rsf = rsf.predict_survival_function(selected_patient, return_array=True) for i, s in enumerate(result_rsf): plt.step(rsf.event_times_, s, where="post") plt.legend(labels=list(patient_rsf.index)) plt.xlabel("Time in months") plt.ylim([0, 1.08]) st.pyplot(fig) else: result = selected_model.predict_survival_function(selected_patient) ax = sns.lineplot(data=result) ax.set(xlabel='Time in months', ylabel='S(t)') st.pyplot(fig) elif show_average == [] and show_progressor == [1]: ax = plt.plot(result_slow.index, result_slow[0], marker='None', color='#C0C0C0', linestyle="--", linewidth=1.8, label='Slow') ax = plt.plot(result_intermediate.index, result_intermediate[0], marker='None', color='#696969', linestyle="--", linewidth=1.8, label='Intermediate') ax = plt.plot(result_rapid.index, result_rapid[0], marker='None', color='k', linestyle="--", linewidth=1.8, label='Rapid') if selected_model == rsf: result_rsf = rsf.predict_survival_function(selected_patient, return_array=True) for i, s in enumerate(result_rsf): plt.step(rsf.event_times_, s, where="post") plt.legend(labels=list(patient_rsf.index)) plt.xlabel("Time in months") plt.ylim([0, 1.08]) st.pyplot(fig) else: result = selected_model.predict_survival_function(selected_patient) ax = sns.lineplot(data=result) ax.set(xlabel='Time in months', ylabel='S(t)') st.pyplot(fig) else: if selected_model == rsf: result_rsf = rsf.predict_survival_function(selected_patient, return_array=True) for i, s in enumerate(result_rsf): plt.step(rsf.event_times_, s, where="post") plt.legend(labels=list(patient_rsf.index)) plt.xlabel("Time in months") plt.ylim([0, 1.08]) st.pyplot(fig) else: result = selected_model.predict_survival_function(selected_patient) ax = sns.lineplot(data=result) ax.set(xlabel='Time in months', ylabel='S(t)') st.pyplot(fig) probability = st.slider('Predict probability point (%)', min_value=30, max_value=100, value=60, step=1) if selected_model == rsf: time2 = predict_rsf_percentile(patient_rsf, 0.01 * probability) if time2 == np.inf: function = pd.DataFrame( rsf.predict_survival_function( patient_rsf[rsf_final_feature_list].iloc[:, :].to_numpy(), return_array=True)) function.columns = rsf.event_times_ last_point = pd.DataFrame(function.iloc[:, -1]) linear = (0.5 * last_point.columns[0]) / (1 - last_point.iloc[:, 0]) time = linear.values[0] else: time = time2 st.write( str(round(float(time), 2)) + ' months from past 3 months (lineary extended)') else: time = selected_model.predict_percentile(selected_patient, p=0.01 * probability) st.write(str(round(float(time), 2)) + ' months from past 3 months')
def _add_application_description(self) -> None: with st.container(): self._add_application_description_header() self._add_application_description_content() columns = self._get_application_description_columns() self._add_application_description_metrics(columns)
address = st.sidebar.text_input(label="address") #TODO: get lat-long from address lat = st.sidebar.number_input(label="latitude") lng = st.sidebar.number_input(label="longitude") #TODO: parse latlong for format / correctness # st.sidebar.write("Distance from epicenter in miles") # radius = st.sidebar.number_input(label="radius") # MAIN st.title("SENSOR GRID") st.write("<hr />", unsafe_allow_html=True) #TODO: Fill sensors, sort by distance from epicenter. # SENSOR GRID SENSORS = [(145, "nearest"), (98, "second"), (125, "third"), (98, "fourth")] for sensor in SENSORS: c = st.container()
import streamlit as st import requests import imageio #from multiselect_options import genres backend = 'http://backend:8000' ep_predict = '/predict-front' request_json = {} st.title("Get book rating") st.header("Book's rating pre" "clerdictor built on goodread's dataset") #book_image book_image_container = st.container() book_image_container.subheader('Upload cover') cover = book_image_container.file_uploader( label='Choose image', help='Book cover importance is 1.7%') if cover: book_image_container.image(cover.getvalue()) height, width = imageio.imread(cover.getvalue()).shape[:2] request_json['book_image_url'] = height * width else: request_json['book_image_url'] = 0 # book_genre book_genre_container = st.container() book_genre_container.subheader('Select genres') genres_select = book_genre_container.multiselect( label="Genres", options=genres, help=
import streamlit as st from datetime import datetime from PIL import Image from train_model import train_model st.write("# Scripted Artistry") main = st.container() col1, col2 = main.columns(2) col1.write(f"""## Your Image Upload the image you want to apply a filter onto below! """) user_image = col1.file_uploader("Upload image", type=["png", "jpeg", "jpg"]) col2.write(f"""## Style Image Upload the image you want us to extract the stylings from and apply onto your Image! """) style_image = col2.file_uploader("Upload image") if user_image is not None and style_image is not None: with st.spinner(text="Style Transfer in progress..."): user_image = Image.open(user_image) style_image = Image.open(style_image) styled_image = train_model(user_image=user_image, style_image=style_image) st.image(styled_image)
#Ujian Akhir Semester Pemrograman Komputer #Nama : Naza Zaida Mustofa #NIM : 12220036 #Kelas : 02 from numpy import e import pandas as pd import streamlit as st # Container declarations header_container = st.container() minyak_graph_container = st.container() n_besar_negara = st.container() n_terbesar = st.container() informasi_negara = st.container() negara_filtered = st.container() # Data load df = pd.read_csv('produksi_minyak_mentah.csv', index_col="kode_negara") dfC = pd.read_json("kode_negara_lengkap.json") # Data cleanup for nCode in df.index.unique().tolist(): if nCode not in dfC['alpha-3'].tolist(): df.drop([nCode], inplace=True) df.reset_index(inplace=True) with header_container: st.title("Data produksi minyak negara") st.markdown("***")
def chi_goodness(): """ """ st.title('chi2 Goodness of Fit Test') st.write( 'This chi2 calculator assumes that your data consists of a single frequency distribution:' ) st.markdown(""" |sample|value1|value2|value3| |------|------|------|------| |sample|37|75|98| """) st.caption("In this case, you copy and paste row-wise values") st.markdown('Or') st.markdown(""" |value|sample| |-----|--------| |value1|37| |value2|75| |value3|98| """) st.caption("In this case, you copy and paste column-wise values") st.write('To use the chi2 calculator:') st.write(""" 1. Input the significant value (default is .05) 2. Copy the values of your sample and paste into the Sample text entry field and hit "Enter." ❗By default, expected frequencies are equally likely. """) significance = float( st.text_input('Input significance value (default is .05)', value='.05')) st.caption("Significance values are often set to 0.005, 0.05, and 0.1") col1 = st.text_input('Sample', value='37 75 98') st.caption("📝 This app does not retain user data.") s1 = [int(c) for c in col1.split()] E = sum(s1) / len(s1) chis = [(s - E)**2 / E for s in s1] chi, p_val = chisquare(s1) p = 1 - significance crit_val = chi2.ppf(p, len(s1) - 1) st.subheader('Results') c1 = st.container() c2, c3, c4, c5 = st.columns(4) c1.metric('p-value', str(p_val)) c2.metric('Dataset Length', str(len(s1))) c3.metric('degree of freedom', "{:.2f}".format(len(s1) - 1)) chart_df = pd.DataFrame(chis, columns=['(O-E)**2/E']) st.bar_chart(chart_df) c4.metric('\n chi2 test statistic', "{:.5f}".format(chi)) c5.metric('critical value', "{:.5f}".format(crit_val)) st.write( "For an extended discussion of using chi2 goodness of fit tests for qualitative coding, see [Geisler and Swarts (2019)](https://wac.colostate.edu/docs/books/codingstreams/chapter9.pdf)" )
def chi_goodness_file_upload(): """ """ st.title('chi2 Goodness of Fit Test') st.write( 'This chi2 calculator assumes that your data consists of a single frequency distribution in a .csv or .xlsx file:' ) st.markdown(""" |value|sample| |-----|--------| |value1|37| |value2|75| |value3|98| """) st.caption("Required table format.") st.write('To use the chi2 calculator:') st.write(""" 1. Input the significant value (default is .05) 2. Upload your .csv or .xlsx file. Insure that you name your column of values "sample" like the example above. ❗By default, expected frequencies are equally likely across classes. """) significance = float( st.text_input('Input significance value (default is .05)', value='.05')) st.caption("Significance values are often set to 0.005, 0.05, and 0.1") uploaded = st.file_uploader('Upload your .csv or .xlsx file.') st.caption("📝 This app does not retain user data.") if uploaded != None: if uploaded.name.endswith('csv'): df = pd.read_csv(uploaded) s1 = df['sample'] chi, p_val = chisquare(s1) p = 1 - significance crit_val = chi2.ppf(p, len(s1) - 1) st.subheader('Results') c1 = st.container() c2, c3, c4, c5 = st.columns(4) c1.metric('p-value', str(p_val)) c2.metric('Dataset Length', str(len(s1))) c3.metric('degree of freedom', "{:.2f}".format(len(s1) - 1)) c4.metric('\n chi2 test statistic', "{:.5f}".format(chi)) c5.metric('critical value', "{:.5f}".format(crit_val)) st.write( "For an extended discussion of using chi2 goodness of fit tests for qualitative coding, see [Geisler and Swarts (2019)](https://wac.colostate.edu/docs/books/codingstreams/chapter9.pdf)" ) elif uploaded.name.endswith('xlsx'): df = pd.read_excel(uploaded) s1 = df['sample'] chi, p_val = chisquare(s1) p = 1 - significance crit_val = chi2.ppf(p, len(s1) - 1) st.subheader('Results') c1 = st.container() c2, c3, c4, c5 = st.columns(4) c1.metric('p-value', str(p_val)) c2.metric('Dataset Length', str(len(s1))) c3.metric('degree of freedom', "{:.2f}".format(len(s1) - 1)) c4.metric('\n chi2 test statistic', "{:.5f}".format(chi)) c5.metric('critical value', "{:.5f}".format(crit_val)) st.write( "For an extended discussion of using chi2 goodness of fit tests for qualitative coding, see [Geisler and Swarts (2019)](https://wac.colostate.edu/docs/books/codingstreams/chapter9.pdf)" ) else: st.write('Please upload a .csv or .xslx file')
def main(): side_img = Image.open("images/emotion3.jpg") with st.sidebar: st.image(side_img, width=300) st.sidebar.subheader("Menu") website_menu = st.sidebar.selectbox( "Menu", ("Emotion Recognition", "Project description", "Our team", "Leave feedback", "Relax")) st.set_option('deprecation.showfileUploaderEncoding', False) if website_menu == "Emotion Recognition": st.sidebar.subheader("Model") model_type = st.sidebar.selectbox("How would you like to predict?", ("mfccs", "mel-specs")) em3 = em6 = em7 = gender = False st.sidebar.subheader("Settings") st.markdown("## Upload the file") with st.container(): col1, col2 = st.columns(2) # audio_file = None # path = None with col1: audio_file = st.file_uploader("Upload audio file", type=['wav', 'mp3', 'ogg']) if audio_file is not None: if not os.path.exists("audio"): os.makedirs("audio") path = os.path.join("audio", audio_file.name) if_save_audio = save_audio(audio_file) if if_save_audio == 1: st.warning("File size is too large. Try another file.") elif if_save_audio == 0: # extract features # display audio st.audio(audio_file, format='audio/wav', start_time=0) try: wav, sr = librosa.load(path, sr=44100) Xdb = get_melspec(path)[1] mfccs = librosa.feature.mfcc(wav, sr=sr) # # display audio # st.audio(audio_file, format='audio/wav', start_time=0) except Exception as e: audio_file = None st.error( f"Error {e} - wrong format of the file. Try another .wav file." ) else: st.error("Unknown error") else: if st.button("Try test file"): wav, sr = librosa.load("test.wav", sr=44100) Xdb = get_melspec("test.wav")[1] mfccs = librosa.feature.mfcc(wav, sr=sr) # display audio st.audio("test.wav", format='audio/wav', start_time=0) path = "test.wav" audio_file = "test" with col2: if audio_file is not None: fig = plt.figure(figsize=(10, 2)) fig.set_facecolor('#d1d1e0') plt.title("Wave-form") librosa.display.waveplot(wav, sr=44100) plt.gca().axes.get_yaxis().set_visible(False) plt.gca().axes.get_xaxis().set_visible(False) plt.gca().axes.spines["right"].set_visible(False) plt.gca().axes.spines["left"].set_visible(False) plt.gca().axes.spines["top"].set_visible(False) plt.gca().axes.spines["bottom"].set_visible(False) plt.gca().axes.set_facecolor('#d1d1e0') st.write(fig) else: pass # st.write("Record audio file") # if st.button('Record'): # with st.spinner(f'Recording for 5 seconds ....'): # st.write("Recording...") # time.sleep(3) # st.success("Recording completed") # st.write("Error while loading the file") if model_type == "mfccs": em3 = st.sidebar.checkbox("3 emotions", True) em6 = st.sidebar.checkbox("6 emotions", True) em7 = st.sidebar.checkbox("7 emotions") gender = st.sidebar.checkbox("gender") elif model_type == "mel-specs": st.sidebar.warning("This model is temporarily disabled") else: st.sidebar.warning("This model is temporarily disabled") # with st.sidebar.expander("Change colors"): # st.sidebar.write("Use this options after you got the plots") # col1, col2, col3, col4, col5, col6, col7 = st.columns(7) # # with col1: # a = st.color_picker("Angry", value="#FF0000") # with col2: # f = st.color_picker("Fear", value="#800080") # with col3: # d = st.color_picker("Disgust", value="#A52A2A") # with col4: # sd = st.color_picker("Sad", value="#ADD8E6") # with col5: # n = st.color_picker("Neutral", value="#808080") # with col6: # sp = st.color_picker("Surprise", value="#FFA500") # with col7: # h = st.color_picker("Happy", value="#008000") # if st.button("Update colors"): # global COLOR_DICT # COLOR_DICT = {"neutral": n, # "positive": h, # "happy": h, # "surprise": sp, # "fear": f, # "negative": a, # "angry": a, # "sad": sd, # "disgust": d} # st.success(COLOR_DICT) if audio_file is not None: st.markdown("## Analyzing...") if not audio_file == "test": st.sidebar.subheader("Audio file") file_details = { "Filename": audio_file.name, "FileSize": audio_file.size } st.sidebar.write(file_details) with st.container(): col1, col2 = st.columns(2) with col1: fig = plt.figure(figsize=(10, 2)) fig.set_facecolor('#d1d1e0') plt.title("MFCCs") librosa.display.specshow(mfccs, sr=sr, x_axis='time') plt.gca().axes.get_yaxis().set_visible(False) plt.gca().axes.spines["right"].set_visible(False) plt.gca().axes.spines["left"].set_visible(False) plt.gca().axes.spines["top"].set_visible(False) st.write(fig) with col2: fig2 = plt.figure(figsize=(10, 2)) fig2.set_facecolor('#d1d1e0') plt.title("Mel-log-spectrogram") librosa.display.specshow(Xdb, sr=sr, x_axis='time', y_axis='hz') plt.gca().axes.get_yaxis().set_visible(False) plt.gca().axes.spines["right"].set_visible(False) plt.gca().axes.spines["left"].set_visible(False) plt.gca().axes.spines["top"].set_visible(False) st.write(fig2) if model_type == "mfccs": st.markdown("## Predictions") with st.container(): col1, col2, col3, col4 = st.columns(4) mfccs = get_mfccs(path, model.input_shape[-1]) mfccs = mfccs.reshape(1, *mfccs.shape) pred = model.predict(mfccs)[0] with col1: if em3: pos = pred[3] + pred[5] * .5 neu = pred[2] + pred[5] * .5 + pred[4] * .5 neg = pred[0] + pred[1] + pred[4] * .5 data3 = np.array([pos, neu, neg]) txt = "MFCCs\n" + get_title(data3, CAT3) fig = plt.figure(figsize=(5, 5)) COLORS = color_dict(COLOR_DICT) plot_colored_polar(fig, predictions=data3, categories=CAT3, title=txt, colors=COLORS) # plot_polar(fig, predictions=data3, categories=CAT3, # title=txt, colors=COLORS) st.write(fig) with col2: if em6: txt = "MFCCs\n" + get_title(pred, CAT6) fig2 = plt.figure(figsize=(5, 5)) COLORS = color_dict(COLOR_DICT) plot_colored_polar(fig2, predictions=pred, categories=CAT6, title=txt, colors=COLORS) # plot_polar(fig2, predictions=pred, categories=CAT6, # title=txt, colors=COLORS) st.write(fig2) with col3: if em7: model_ = load_model("model4.h5") mfccs_ = get_mfccs(path, model_.input_shape[-2]) mfccs_ = mfccs_.T.reshape(1, *mfccs_.T.shape) pred_ = model_.predict(mfccs_)[0] txt = "MFCCs\n" + get_title(pred_, CAT7) fig3 = plt.figure(figsize=(5, 5)) COLORS = color_dict(COLOR_DICT) plot_colored_polar(fig3, predictions=pred_, categories=CAT7, title=txt, colors=COLORS) # plot_polar(fig3, predictions=pred_, categories=CAT7, # title=txt, colors=COLORS) st.write(fig3) with col4: if gender: with st.spinner('Wait for it...'): gmodel = load_model("model_mw.h5") gmfccs = get_mfccs(path, gmodel.input_shape[-1]) gmfccs = gmfccs.reshape(1, *gmfccs.shape) gpred = gmodel.predict(gmfccs)[0] gdict = [["female", "woman.png"], ["male", "man.png"]] ind = gpred.argmax() txt = "Predicted gender: " + gdict[ind][0] img = Image.open("images/" + gdict[ind][1]) fig4 = plt.figure(figsize=(3, 3)) fig4.set_facecolor('#d1d1e0') plt.title(txt) plt.imshow(img) plt.axis("off") st.write(fig4) # if model_type == "mel-specs": # st.markdown("## Predictions") # st.warning("The model in test mode. It may not be working properly.") # if st.checkbox("I'm OK with it"): # try: # with st.spinner("Wait... It can take some time"): # global tmodel # tmodel = load_model_cache("tmodel_all.h5") # fig, tpred = plot_melspec(path, tmodel) # col1, col2, col3 = st.columns(3) # with col1: # st.markdown("### Emotional spectrum") # dimg = Image.open("images/spectrum.png") # st.image(dimg, use_column_width=True) # with col2: # fig_, tpred_ = plot_melspec(path=path, # tmodel=tmodel, # three=True) # st.write(fig_, use_column_width=True) # with col3: # st.write(fig, use_column_width=True) # except Exception as e: # st.error(f"Error {e}, model is not loaded") elif website_menu == "Project description": import pandas as pd import plotly.express as px st.title("Project description") st.subheader("GitHub") link = '[GitHub repository of the web-application]' \ '(https://github.com/CyberMaryVer/speech-emotion-webapp)' st.markdown(link, unsafe_allow_html=True) st.subheader("Theory") link = '[Theory behind - Medium article]' \ '(https://talbaram3192.medium.com/classifying-emotions-using-audio-recordings-and-python-434e748a95eb)' st.markdown(link + ":clap::clap::clap: Tal!", unsafe_allow_html=True) with st.expander("See Wikipedia definition"): components.iframe( "https://en.wikipedia.org/wiki/Emotion_recognition", height=320, scrolling=True) st.subheader("Dataset") txt = """ This web-application is a part of the final **Data Mining** project for **ITC Fellow Program 2020**. Datasets used in this project * Crowd-sourced Emotional Mutimodal Actors Dataset (**Crema-D**) * Ryerson Audio-Visual Database of Emotional Speech and Song (**Ravdess**) * Surrey Audio-Visual Expressed Emotion (**Savee**) * Toronto emotional speech set (**Tess**) """ st.markdown(txt, unsafe_allow_html=True) df = pd.read_csv("df_audio.csv") fig = px.violin(df, y="source", x="emotion4", color="actors", box=True, points="all", hover_data=df.columns) st.plotly_chart(fig, use_container_width=True) st.subheader("FYI") st.write( "Since we are currently using a free tier instance of AWS, " "we disabled mel-spec and ensemble models.\n\n" "If you want to try them we recommend to clone our GitHub repo") st.code( "git clone https://github.com/CyberMaryVer/speech-emotion-webapp.git", language='bash') st.write( "After that, just uncomment the relevant sections in the app.py file " "to use these models:") elif website_menu == "Our team": st.subheader("Our team") st.balloons() col1, col2 = st.columns([3, 2]) with col1: st.info("*****@*****.**") st.info("*****@*****.**") st.info("*****@*****.**") with col2: liimg = Image.open("images/LI-Logo.png") st.image(liimg) st.markdown( f""":speech_balloon: [Maria Startseva](https://www.linkedin.com/in/maria-startseva)""", unsafe_allow_html=True) st.markdown( f""":speech_balloon: [Tal Baram](https://www.linkedin.com/in/tal-baram-b00b66180)""", unsafe_allow_html=True) st.markdown( f""":speech_balloon: [Asher Holder](https://www.linkedin.com/in/asher-holder-526a05173)""", unsafe_allow_html=True) elif website_menu == "Leave feedback": st.subheader("Leave feedback") user_input = st.text_area("Your feedback is greatly appreciated") user_name = st.selectbox( "Choose your personality", ["checker1", "checker2", "checker3", "checker4"]) if st.button("Submit"): st.success(f"Message\n\"\"\"{user_input}\"\"\"\nwas sent") if user_input == "log123456" and user_name == "checker4": with open("log0.txt", "r", encoding="utf8") as f: st.text(f.read()) elif user_input == "feedback123456" and user_name == "checker4": with open("log.txt", "r", encoding="utf8") as f: st.text(f.read()) else: log_file(user_name + " " + user_input) thankimg = Image.open("images/sticky.png") st.image(thankimg) else: import requests import json url = 'http://api.quotable.io/random' if st.button("get random mood"): with st.container(): col1, col2 = st.columns(2) n = np.random.randint(1, 1000, 1)[0] with col1: quotes = { "Good job and almost done": "checker1", "Great start!!": "checker2", "Please make corrections base on the following observation": "checker3", "DO NOT train with test data": "folk wisdom", "good work, but no docstrings": "checker4", "Well done!": "checker3", "For the sake of reproducibility, I recommend setting the random seed": "checker1" } if n % 5 == 0: a = np.random.choice(list(quotes.keys()), 1)[0] quote, author = a, quotes[a] else: try: r = requests.get(url=url) text = json.loads(r.text) quote, author = text['content'], text['author'] except Exception as e: a = np.random.choice(list(quotes.keys()), 1)[0] quote, author = a, quotes[a] st.markdown(f"## *{quote}*") st.markdown(f"### ***{author}***") with col2: st.image(image=f"https://picsum.photos/800/600?random={n}")