def test_configure(self): client = in3.Client('goerli') self.assertIsInstance(client, in3.Client) client = in3.Client('goErli') self.assertIsInstance(client, in3.Client) client = in3.Client('goerli', in3.model.ClientConfig()) self.assertIsInstance(client, in3.Client)
def test_configure(self): client = in3.Client('kovan') self.assertIsInstance(client, in3.Client) client = in3.Client('koVan') self.assertIsInstance(client, in3.Client) client = in3.Client('kovan', in3.model.ClientConfig()) self.assertIsInstance(client, in3.Client)
def test_configure(self): client = in3.Client() self.assertIsInstance(client, in3.Client) client = in3.Client('mainnet') self.assertIsInstance(client, in3.Client) client = in3.Client('mainNet') self.assertIsInstance(client, in3.Client) client = in3.Client(in3_config=in3.model.ClientConfig()) self.assertIsInstance(client, in3.Client)
def setUp(self): # self.client = in3.Client('goerli', in3_config=mainchain_mock_config) self.client = in3.Client('goerli', in3_config=mainchain_mock_config, cache_enabled=False, transport=mock_transport, test_instance=True)
def test_send_transact_without_key(self): main = ContractActivities(in3.Client("goerli")) main.setVisible(True) self.assertEqual(main.convict_modal.isVisible(), False) self.assertEqual(main.no_key_error_label.isHidden(), True) main.people_buttons[1].click() self.assertEqual(main.convict_modal.isHidden(), True) self.assertEqual(main.no_key_error_label.isHidden(), False)
def test_send_admin_transact_with_nonadmin_key(self): main = ContractActivities(in3.Client("goerli")) main.setVisible(True) main.p_key = "bae4f8f1b2778d75625157b108d4de1a469ad330f88c6d699703d2b309dd7744" self.assertEqual(main.remove_node_modal.isVisible(), False) self.assertEqual(main.no_key_error_label.isHidden(), True) main.admin_buttons[0].click() self.assertEqual(main.remove_node_modal.isHidden(), True) self.assertEqual(main.no_key_error_label.isHidden(), False)
def test_correct_account_addition(self): main = ContractActivities(in3.Client("goerli")) cont = ContractModal(main) self.assertEqual(cont.isVisible(), False) cont.setVisible(True) cont.new_addr_line.setText("0xFB55cC4bE5496629169219FeeEFDF98d423AFf8B") cont.modal_add.click() # self.assertEqual(acc.isHidden(), True) self.assertEqual(cont.modal_error_label.isVisible(), False) self.assertEqual(cont.new_addr_line.text(), "")
def test_wrong_contract_addition(self): main = ContractActivities(in3.Client("goerli")) cont = ContractModal(main) self.assertEqual(cont.isVisible(), False) cont.setVisible(True) cont.new_addr_line.setText("Hello darkness") cont.modal_add.click() # self.assertEqual(acc.isHidden(), True) self.assertEqual(cont.modal_error_label.isVisible(), True) self.assertEqual(cont.new_addr_line.text(), "")
def test_instantiate(self): with self.assertRaises(AssertionError): in3.Client(None) with self.assertRaises(AssertionError): in3.Client(1) with self.assertRaises(AssertionError): in3.Client(-1) with self.assertRaises(AssertionError): in3.Client('œ∑´´†√¨') with self.assertRaises(AssertionError): in3.Client('!@# asd') with self.assertRaises(AssertionError): in3.Client({1: 1}) with self.assertRaises(AssertionError): in3.Client((1)) with self.assertRaises(AssertionError): in3.Client([1])
def test_configure(self): with self.assertRaises(AssertionError): in3.Client(in3_config=1) with self.assertRaises(AssertionError): in3.Client(in3_config=-1) with self.assertRaises(AssertionError): in3.Client(in3_config='œ∑´´†√¨') with self.assertRaises(AssertionError): in3.Client(in3_config={1: 1}) with self.assertRaises(AssertionError): in3.Client(in3_config=(1)) with self.assertRaises(AssertionError): in3.Client(in3_config=[1])
def __init__(self, network="goerli"): QWidget.__init__(self) # check if the app is connected to the network self.client = in3.Client(network) try: check = self.client.eth.block_number() except ClientException: print("Unable to connect to the" + network) return self.p_key = "" self.server_listing_box = ServerListing(self.client) self.buttons_listing_box = ContractActivities(self.client) self.server_listing_box.setMinimumWidth(800) # Private key setup button creation(assigned to the top right corner) self.top = QWidget() self.account_layout = QVBoxLayout() self.settings_button = QToolButton() self.settings_button.setIcon(QIcon("./resources/account.png")) self.account_layout.addWidget(self.settings_button) self.account_layout.setAlignment(Qt.AlignRight) self.top.setLayout(self.account_layout) # Main layout, with all of the functionality on it self.main_layout = QFormLayout() self.main_layout.addRow(QLabel("Network: " + network), self.top) self.main_layout.addRow(self.server_listing_box, self.buttons_listing_box) self.setLayout(self.main_layout) # Connecting the signal self.settings_button.clicked.connect(self.account_click) # Modal window to set up user's account self.add_account_modal = AccountModal(self)
import in3 def _print(): print('\nAddress for {} @ {}: {}'.format(domain, chain, address)) print('Owner for {} @ {}: {}'.format(domain, chain, owner)) # Find ENS for the desired chain or the address of your own ENS resolver. https://docs.ens.domains/ens-deployments domain = 'depraz.eth' print('\nEthereum Name Service') # Instantiate In3 Client for Goerli chain = 'goerli' client = in3.Client(chain) address = client.ens_address(domain) # Instantiate In3 Client for Mainnet chain = 'mainnet' client = in3.Client(chain) address = client.ens_address(domain) owner = client.ens_owner(domain) _print() # Instantiate In3 Client for Kovan chain = 'kovan' client = in3.Client(chain) try: address = client.ens_address(domain) owner = client.ens_owner(domain)
""" Resolves ENS domains to Ethereum addresses ENS is a smart-contract system that registers and resolves `.eth` domains. """ import in3 # Find ENS for the desired chain or the address of your own ENS resolver. https://docs.ens.domains/ens-deployments # Instantiate In3 Client for the Ethereum main net disabling cache to get the freshest address. client = in3.Client(cache_enabled=False) domain = 'depraz.eth' if __name__ == '__main__': try: print('\nEthereum Name Service') address = client.ens_address(domain) owner = client.ens_owner(domain) print('\nAddress for {} @ {}: {}'.format(domain, 'mainnet', address)) print('Owner for {} @ {}: {}'.format(domain, 'mainnet', owner)) except in3.ClientException as e: print('Network might be unstable, try again later.\n Reason: ', str(e)) # Produces """ Ethereum Name Service Address for depraz.eth @ mainnet: 0x0b56ae81586d2728ceaf7c00a6020c5d63f02308 Owner for depraz.eth @ mainnet: 0x6fa33809667a99a805b610c49ee2042863b1bb83 """
def setUp(self): # self.client = in3.Client('goerli', in3_config=mock_config) self.client = in3.Client('goerli', in3_config=mock_config, transport=mock_transport)
def setUp(self): # self.client = in3.Client(in3_config=mainchain_mock_config) self.client = in3.Client(in3_config=mainchain_mock_config, cache_enabled=False, transport=mock_transport)
""" Connects to Ethereum and fetches attested information from each chain. """ import in3 if __name__ == '__main__': client = in3.Client() try: print('\nEthereum Main Network') latest_block = client.eth.block_number() gas_price = client.eth.gas_price() print('Latest BN: {}\nGas Price: {} Wei'.format( latest_block, gas_price)) except in3.ClientException as e: print('Network might be unstable, try again later.\n Reason: ', str(e)) goerli_client = in3.Client('goerli') try: print('\nEthereum Goerli Test Network') latest_block = goerli_client.eth.block_number() gas_price = goerli_client.eth.gas_price() print('Latest BN: {}\nGas Price: {} Wei'.format( latest_block, gas_price)) except in3.ClientException as e: print('Network might be unstable, try again later.\n Reason: ', str(e)) # Produces """ Ethereum Main Network Latest BN: 9801135
sg.theme('DarkAmber') # Add a touch of color # All the stuff inside your window. layout = [[sg.Button("Mainnet")], [sg.Button("Kovan")], [sg.Button("Goerli")], [sg.Button("EWC")], [sg.Button("Exit")]] # Create the Window window = sg.Window('Fetch Ethereum Data', layout) # Event Loop to process "events" and get the "values" of the inputs while True: event, values = window.read() if event == sg.WIN_CLOSED or event == 'Cancel': # if user closes window or clicks cancel break elif event == "Mainnet": # on every event, the client is instantiated differently and the list of client nodes are refreshedgt client = in3.Client("Mainnet") node_list = client.refresh_node_list() sg.Print('\nIncubed Registry:') sg.Print('\ttotal servers:{}'.format(node_list.totalServers)) sg.Print('\tlast updated in block:{}'.format( node_list.lastBlockNumber)) sg.Print('\tregistry ID:{}'.format(node_list.registryId)) sg.Print('\tcontract address:{}'.format(node_list.contract)) sg.Print('\nNodes Registered:\n') for node in node_list.nodes: sg.Print('\turl:{}'.format(node.url)) sg.Print('\tdeposit:', node.deposit) sg.Print('\tweight:', node.weight) sg.Print('\tregistered in block:', node.registerTime) sg.Print('\n')
import in3 import time conf = in3.Config() conf.chainId = str(in3.Chain.KOVAN) eth = in3.Client(in3_config=conf).eth filter = eth.new_block_filter() count = 0 while (count < 10): changes = eth.get_filter_changes(filter) time.sleep(10) print(changes) count += 1 print(">>>")
def setUp(self): self.client = in3.Client(in3_config=mainchain_mock_config, transport=mock_transport, test_instance=True)
def setUp(self): self.client = in3.Client()
""" import json import in3 import time # On Metamask, be sure to be connected to the correct chain, click on the `...` icon on the right corner of # your Account name, select `Account Details`. There, click `Export Private Key`, copy the value to use as secret. # By reading the terminal input, this value will stay in memory only. Don't forget to cls or clear terminal after ;) sender_secret = input("Sender secret: ") receiver = input("Receiver address: ") # 1000000000000000000 == 1 ETH # 1000000000 == 1 Gwei Check https://etherscan.io/gasTracker. value_in_wei = 1463926659 # None for Eth mainnet chain = 'goerli' client = in3.Client(chain if chain else 'mainnet') # A transaction is only final if a certain number of blocks are mined on top of it. # This number varies with the chain's consensus algorithm. Time can be calculated over using: # wait_time = blocks_for_consensus * avg_block_time_in_secs # For mainnet and paying low gas, it might take 10 minutes. confirmation_wait_time_in_seconds = 30 etherscan_link_mask = 'https://{}{}etherscan.io/tx/{}' print('-= Ethereum Transaction using Incubed =- \n') try: sender = client.eth.account.recover(sender_secret) tx = in3.eth.NewTransaction(to=receiver, value=value_in_wei) print('[.] Sending {} Wei from {} to {}. Please wait.\n'.format( tx.value, sender.address, tx.to)) tx_hash = client.eth.account.send_transaction(sender, tx) print('[.] Transaction accepted with hash {}.'.format(tx_hash))
""" Manually calling the ENS smart-contract 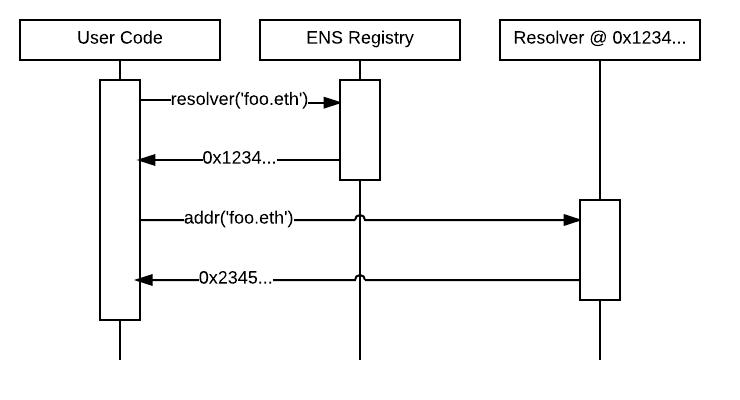 """ import in3 client = in3.Client('goerli') if __name__ == '__main__': try: print('-= Smart-Contract Call on Ethereum using Incubed =- \n') domain_name = client.ens_namehash('depraz.eth') ens_registry_addr = '0x00000000000c2e074ec69a0dfb2997ba6c7d2e1e' ens_resolver_abi = 'resolver(bytes32):address' # Find resolver contract for ens name resolver_tx = { "to": ens_registry_addr, "data": client.eth.contract.encode(ens_resolver_abi, domain_name) } tx = in3.eth.NewTransaction(**resolver_tx) except in3.ClientException as e: print('Something went wrong converting data.\n Reason: ', str(e)) try: # Make the smart contract call print('Calling the ENS registry contract.') encoded_resolver_addr = client.eth.contract.call(tx) resolver_address = client.eth.contract.decode(ens_resolver_abi, encoded_resolver_addr) except in3.ClientException as e:
""" Connects to Ethereum and fetches attested information from each chain. """ import in3 print('\nEthereum Main Network') client = in3.Client() latest_block = client.eth.block_number() gas_price = client.eth.gas_price() print('Latest BN: {}\nGas Price: {} Wei'.format(latest_block, gas_price)) print('\nEthereum Kovan Test Network') client = in3.Client('kovan') latest_block = client.eth.block_number() gas_price = client.eth.gas_price() print('Latest BN: {}\nGas Price: {} Wei'.format(latest_block, gas_price)) print('\nEthereum Goerli Test Network') client = in3.Client('goerli') latest_block = client.eth.block_number() gas_price = client.eth.gas_price() print('Latest BN: {}\nGas Price: {} Wei'.format(latest_block, gas_price)) # Produces """ Ethereum Main Network Latest BN: 9801135 Gas Price: 2000000000 Wei Ethereum Kovan Test Network Latest BN: 17713464
def setUp(self): conf = in3.Config() conf.chainId = str(in3.Chain.KOVAN) self.in3_client = in3.Client(in3_config=conf)
sg.InputText()], [sg.Submit(), sg.Cancel()]] window = sg.Window('Resolve ENS to an Ethereum Address', layout) while True: # The Event Loop event, values = window.read() def _print(): sg.Print('\nAddress for {} @ {}: {}'.format(domain, chain, address)) sg.Print('Owner for {} @ {}: {}'.format(domain, chain, owner)) domain = str(values[0]) # Instantiate In3 Client for Goerli chain = 'goerli' client = in3.Client(chain, cache_enabled=False) address = client.ens_address(domain) owner = client.ens_owner(domain) _print() # Instantiate In3 Client for Mainnet chain = 'mainnet' client = in3.Client(chain, cache_enabled=False) address = client.ens_address(domain) owner = client.ens_owner(domain) _print() # Instantiate In3 Client for Kovan chain = 'kovan' client = in3.Client(chain, cache_enabled=True) try: