def sign_up_post(v): if v: abort(403) agent = request.headers.get("User-Agent", None) if not agent: abort(403) form_timestamp = request.form.get("now", 0) form_formkey = request.form.get("formkey", "none") submitted_token = session["signup_token"] correct_formkey_hashstr = form_timestamp + submitted_token + agent correct_formkey = hmac.new(key=bytes(environ.get("MASTER_KEY"), "utf-16"), msg=bytes(correct_formkey_hashstr, "utf-16")).hexdigest() now = int(time.time()) username = request.form.get("username") #define function that takes an error message and generates a new signup form def new_signup(error): args = {"error": error} if request.form.get("referred_by"): user = db.query(User).filter_by( id=request.form.get("referred_by")).first() if user: args["ref"] = user.username return redirect(f"/signup?{urlencode(args)}") #check for tokens ## if now-int(form_timestamp)>120: ## print(f"signup fail - {username } - form expired") return new_signup("There was a problem. Please try again.") if now - int(form_timestamp) < 5: print(f"signup fail - {username } - too fast") return new_signup("There was a problem. Please try again.") if not hmac.compare_digest(correct_formkey, form_formkey): print(f"signup fail - {username } - mismatched formkeys") return new_signup("There was a problem. Please try again.") #check for matched passwords if not request.form.get("password") == request.form.get( "password_confirm"): return new_signup("Passwords did not match. Please try again.") #check username/pass conditions if not re.match(valid_username_regex, request.form.get("username")): print(f"signup fail - {username } - mismatched passwords") return new_signup("Invalid username") if not re.match(valid_password_regex, request.form.get("password")): print(f"signup fail - {username } - invalid password") return new_signup("Password must be 8 characters or longer") #if not re.match(valid_email_regex, request.form.get("email")): # return new_signup("That's not a valid email.") #Check for existing acocunts email = request.form.get("email") if not email: email = None if (db.query(User).filter(User.username.ilike( request.form.get("username"))).first() or (email and db.query(User).filter(User.email.ilike(email)).first())): print(f"signup fail - {username } - email already exists") return new_signup( "An account with that username or email already exists.") #success #kill tokens session.pop("signup_token") #get referral ref_id = int(request.form.get("referred_by", 0)) ref_id = None if not ref_id else ref_id #make new user try: new_user = User(username=username, password=request.form.get("password"), email=email, created_utc=int(time.time()), creation_ip=request.remote_addr, referred_by=ref_id) except Exception as e: print(e) return new_signup("Please enter a valid email") db.add(new_user) db.commit() #give a beta badge beta_badge = Badge(user_id=new_user.id, badge_id=1) db.add(beta_badge) db.commit() check_for_alts(new_user.id) if email: send_verification_email(new_user) session["user_id"] = new_user.id session["session_id"] = token_hex(16) redir = request.form.get("redirect", None) if redir: return redirect(redir) else: return redirect(new_user.permalink)
def sign_up_post(v): if v: abort(403) agent=request.headers.get("User-Agent", None) if not agent: abort(403) form_timestamp = request.form.get("now", 0) form_formkey = request.form.get("formkey","none") submitted_token=session.get("signup_token", "") if not submitted_token: abort(400) correct_formkey_hashstr = form_timestamp+submitted_token+agent correct_formkey = hmac.new(key=bytes(environ.get("MASTER_KEY"), "utf-16"), msg=bytes(correct_formkey_hashstr, "utf-16") ).hexdigest() now=int(time.time()) username=request.form.get("username") #define function that takes an error message and generates a new signup form def new_signup(error): args={"error":error} if request.form.get("referred_by"): user=g.db.query(User).filter_by(id=request.form.get("referred_by")).first() if user: args["ref"]=user.username return redirect(f"/signup?{urlencode(args)}") #check for tokens ## if now-int(form_timestamp)>120: ## print(f"signup fail - {username } - form expired") return new_signup("There was a problem. Please try again.") if now-int(form_timestamp)<5: print(f"signup fail - {username } - too fast") return new_signup("There was a problem. Please try again.") if not hmac.compare_digest(correct_formkey, form_formkey): print(f"signup fail - {username } - mismatched formkeys") return new_signup("There was a problem. Please try again.") #check for matched passwords if not request.form.get("password") == request.form.get("password_confirm"): return new_signup("Passwords did not match. Please try again.") #check username/pass conditions if not re.match(valid_username_regex, request.form.get("username")): print(f"signup fail - {username } - mismatched passwords") return new_signup("Invalid username") if not re.match(valid_password_regex, request.form.get("password")): print(f"signup fail - {username } - invalid password") return new_signup("Password must be 8 characters or longer") #if not re.match(valid_email_regex, request.form.get("email")): # return new_signup("That's not a valid email.") #Check for existing acocunts email=request.form.get("email") if not email: email=None existing_account=g.db.query(User).filter(User.username.ilike(request.form.get("username"))).first() if existing_account and existing_account.reserved: return redirect(existing_account.permalink) if existing_account or (email and g.db.query(User).filter(User.email.ilike(email)).first()): print(f"signup fail - {username } - email already exists") return new_signup("An account with that username or email already exists.") #check bans if any([x.is_banned for x in [g.db.query(User).filter_by(id=y).first() for y in session.get("history",[])] if x]): abort(403) #success #kill tokens session.pop("signup_token") #get referral ref_id = int(request.form.get("referred_by") or 0) #upgrade user badge ref_user=g.db.query(User).filter_by(id=ref_id).first() if not ref_user: ref_id=None #make new user try: new_user=User(username=username, password=request.form.get("password"), email=email, created_utc=int(time.time()), creation_ip=request.remote_addr, referred_by=ref_id, tos_agreed_utc=int(time.time()) ) except Exception as e: print(e) return new_signup("Please enter a valid email") g.db.add(new_user) g.db.commit() #give a beta badge beta_badge=Badge(user_id=new_user.id, badge_id=6) g.db.add(beta_badge) #check alts check_for_alts(new_user.id) #send welcome/verify email if email: send_verification_email(new_user) #send welcome message text=f"""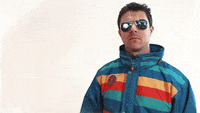 \n\nWelcome to Ruqqus, {new_user.username}. We're glad to have you here. \n\nWhile you get settled in, here a couple things we recommend for newcomers: - View the [quickstart guide](https://ruqqus.com/post/86i) - Customize your profile by [adding a custom avatar and banner](/settings/profile) - Personalize your front page by [joining some guilds](/browse) \n\nYou're welcome to say anything protected by the First Amendment here - even if you don't live in the United States. And since we're committed to [open-source](https://github.com/ruqqus/ruqqus) transparency, your front page (and your posted content) won't be artificially manipulated. \n\nReally, it's what social media should have been doing all along. \n\nNow, go enjoy your digital freedom. \n\n-The Ruqqus Team""" send_notification(new_user, text) session["user_id"]=new_user.id session["session_id"]=token_hex(16) redir=request.form.get("redirect", None) print(f"Signup event: @{new_user.username}") if redir: return redirect(redir) else: return redirect(new_user.permalink)
def sign_up_post(v): if v: abort(403) agent=request.headers.get("User-Agent", None) if not agent: abort(403) form_timestamp = request.form.get("now", 0) form_formkey = request.form.get("formkey","none") submitted_token=session["signup_token"] correct_formkey_hashstr = form_timestamp+submitted_token+agent correct_formkey = hmac.new(key=bytes(environ.get("MASTER_KEY"), "utf-16"), msg=bytes(correct_formkey_hashstr, "utf-16") ).hexdigest() now=int(time.time()) #define function that takes an error message and generates a new signup form def new_signup(error): #Reset tokens and return to signup form token = token_hex(16) session["signup_token"]=token now=int(time.time()) agent=request.headers.get("User-Agent", None) new_formkey_hashstr=str(now)+submitted_token+agent new_formkey = hmac.new(key=bytes(environ.get("MASTER_KEY"), "utf-16"), msg=bytes(new_formkey_hashstr, "utf-16") ).hexdigest() return render_template("sign_up.html", formkey=new_formkey, now=now, error=error, i=random_image()) #check for tokens if now-int(form_timestamp)>120: print("form expired") return new_signup("There was a problem. Please refresh the page and try again.") elif now-int(form_timestamp)<5: print("slow down!") return new_signup("There was a problem. Please refresh the page and try again.") if not hmac.compare_digest(correct_formkey, form_formkey): print(f"{request.form.get('username')} - mismatched formkeys") return new_signup("There was a problem. Please refresh the page and try again.") #check for matched passwords if not request.form.get("password") == request.form.get("password_confirm"): return new_signup("Passwords did not match. Please try again.") #check username/pass conditions if not re.match(valid_username_regex, request.form.get("username")): return new_signup("Invalid username") if not re.match(valid_password_regex, request.form.get("password")): return new_signup("Password must be 8 characters or longer") #Check for existing acocunts if (db.query(User).filter(User.username.ilike(request.form.get("username"))).first() or db.query(User).filter(User.email.ilike(request.form.get("email"))).first()): return new_signup("An account with that username or email already exists.") #success #kill tokens session.pop("signup_token") #get referral ref_id = int(request.form.get("referred_by", 0)) ref_id=None if not ref_id else ref_id #make new user try: new_user=User(username=request.form.get("username"), password=request.form.get("password"), email=request.form.get("email"), created_utc=int(time.time()), creation_ip=request.remote_addr, referred_by=ref_id ) except Exception as e: print(e) return new_signup("Please enter a valid email") db.add(new_user) db.commit() prebeta_badge=Badge(user_id=new_user.id, badge_id=1) db.add(prebeta_badge) db.commit() send_verification_email(new_user) session["user_id"]=new_user.id session["session_id"]=token_hex(16) redir=request.form.get("redirect", None) if redir: return redirect(redir) else: return redirect(new_user.permalink)
def sign_up_post(v): if v: abort(403) agent = request.headers.get("User-Agent", None) if not agent: abort(403) # check tor # if request.headers.get("CF-IPCountry")=="T1": # return render_template("sign_up_tor.html", # i=random_image() # ) form_timestamp = request.form.get("now", 0) form_formkey = request.form.get("formkey", "none") submitted_token = session.get("signup_token", "") if not submitted_token: abort(400) correct_formkey_hashstr = form_timestamp + submitted_token + agent correct_formkey = hmac.new(key=bytes(environ.get("MASTER_KEY"), "utf-16"), msg=bytes(correct_formkey_hashstr, "utf-16") ).hexdigest() now = int(time.time()) username = request.form.get("username") # define function that takes an error message and generates a new signup # form def new_signup(error): args = {"error": error} if request.form.get("referred_by"): user = g.db.query(User).filter_by( id=request.form.get("referred_by")).first() if user: args["ref"] = user.username return redirect(f"/signup?{urlencode(args)}") if app.config["DISABLE_SIGNUPS"]: return new_signup("New account registration is currently closed. Please come back later.") if now - int(form_timestamp) < 5: #print(f"signup fail - {username } - too fast") return new_signup("There was a problem. Please try again.") if not hmac.compare_digest(correct_formkey, form_formkey): #print(f"signup fail - {username } - mismatched formkeys") return new_signup("There was a problem. Please try again.") # check for matched passwords if not request.form.get( "password") == request.form.get("password_confirm"): return new_signup("Passwords did not match. Please try again.") # check username/pass conditions if not re.match(valid_username_regex, request.form.get("username")): #print(f"signup fail - {username } - mismatched passwords") return new_signup("Invalid username") if not re.match(valid_password_regex, request.form.get("password")): #print(f"signup fail - {username } - invalid password") return new_signup("Password must be between 8 and 100 characters.") # if not re.match(valid_email_regex, request.form.get("email")): # return new_signup("That's not a valid email.") # Check for existing acocunts email = request.form.get("email") email = email.lstrip().rstrip() if not email: email = None #counteract gmail username+2 and extra period tricks - convert submitted email to actual inbox if email and email.endswith("@gmail.com"): gmail_username=email.split('@')[0] gmail_username=gmail_username.split('+')[0] gmail_username=gmail_username.replace('.','') email=f"{gmail_username}@gmail.com" existing_account = get_user(request.form.get("username"), graceful=True) if existing_account and existing_account.reserved: return redirect(existing_account.permalink) if existing_account or (email and g.db.query( User).filter(User.email.ilike(email)).first()): # #print(f"signup fail - {username } - email already exists") return new_signup( "An account with that username or email already exists.") # ip ratelimit previous = g.db.query(User).filter_by( creation_ip=request.remote_addr).filter( User.created_utc > int( time.time()) - 60 * 60).first() if previous: abort(429) # check bot if app.config.get("HCAPTCHA_SITEKEY"): token = request.form.get("h-captcha-response") if not token: return new_signup("Unable to verify captcha [1].") data = {"secret": app.config["HCAPTCHA_SECRET"], "response": token, "sitekey": app.config["HCAPTCHA_SITEKEY"]} url = "https://hcaptcha.com/siteverify" x = requests.post(url, data=data) if not x.json()["success"]: #print(x.json()) return new_signup("Unable to verify captcha [2].") # kill tokens session.pop("signup_token") # get referral ref_id = int(request.form.get("referred_by", 0)) # upgrade user badge if ref_id: ref_user = g.db.query(User).options( lazyload('*')).filter_by(id=ref_id).first() if ref_user: ref_user.refresh_selfset_badges() g.db.add(ref_user) # make new user try: new_user = User(username=username, password=request.form.get("password"), email=email, created_utc=int(time.time()), creation_ip=request.remote_addr, referred_by=ref_id or None, tos_agreed_utc=int(time.time()), creation_region=request.headers.get("cf-ipcountry"), ban_evade = int(any([x.is_banned for x in g.db.query(User).filter(User.id.in_(tuple(session.get("history", [])))).all() if x])) ) except Exception as e: #print(e) return new_signup("Please enter a valid email") g.db.add(new_user) g.db.commit() # give a beta badge beta_badge = Badge(user_id=new_user.id, badge_id=6) g.db.add(beta_badge) # check alts check_for_alts(new_user.id) # send welcome/verify email if email: send_verification_email(new_user) # send welcome message text = f"""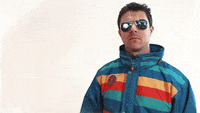 \n\nWelcome to Ruqqus, {new_user.username}. We're glad to have you here. \n\nWhile you get settled in, here a couple things we recommend for newcomers: - View the [quickstart guide](https://ruqqus.com/post/86i) - Personalize your front page by [joining some guilds](/browse) \n\nYou're welcome to say anything protected by the First Amendment here - even if you don't live in the United States. And since we're committed to [open-source](https://github.com/ruqqus/ruqqus) transparency, your front page (and your posted content) won't be artificially manipulated. \n\nReally, it's what social media should have been doing all along. \n\nNow, go enjoy your digital freedom. \n\n-The Ruqqus Team""" send_notification(new_user, text) session["user_id"] = new_user.id session["session_id"] = token_hex(16) redir = request.form.get("redirect", None) # #print(f"Signup event: @{new_user.username}") return redirect("/")
def sign_up_post(v): if v: abort(403) agent = request.headers.get("User-Agent", None) if not agent: abort(403) # check tor # if request.headers.get("CF-IPCountry")=="T1": # return render_template("sign_up_tor.html", # i=random_image() # ) form_timestamp = request.form.get("now", '0') form_formkey = request.form.get("formkey", "none") submitted_token = session.get("signup_token", "") if not submitted_token: abort(400) correct_formkey_hashstr = form_timestamp + submitted_token + agent correct_formkey = hmac.new(key=bytes(environ.get("MASTER_KEY"), "utf-16"), msg=bytes(correct_formkey_hashstr, "utf-16")).hexdigest() now = int(time.time()) username = request.form.get("username") # define function that takes an error message and generates a new signup # form def new_signup(error): args = {"error": error} if request.form.get("referred_by"): user = g.db.query(User).filter_by( id=request.form.get("referred_by")).first() if user: args["ref"] = user.username return redirect(f"/signup?{urlencode(args)}") if app.config["DISABLE_SIGNUPS"]: return new_signup( "New account registration is currently closed. Please come back later." ) if now - int(form_timestamp) < 5: #print(f"signup fail - {username } - too fast") return new_signup("There was a problem. Please try again.") if not hmac.compare_digest(correct_formkey, form_formkey): #print(f"signup fail - {username } - mismatched formkeys") return new_signup("There was a problem. Please try again.") # check for matched passwords if not request.form.get("password") == request.form.get( "password_confirm"): return new_signup("Passwords did not match. Please try again.") # check username/pass conditions if not re.match(valid_username_regex, request.form.get("username")): #print(f"signup fail - {username } - mismatched passwords") return new_signup("Invalid username") if not re.match(valid_password_regex, request.form.get("password")): #print(f"signup fail - {username } - invalid password") return new_signup("Password must be between 8 and 100 characters.") # if not re.match(valid_email_regex, request.form.get("email")): # return new_signup("That's not a valid email.") # Check for existing acocunts email = request.form.get("email") email = email.lstrip().rstrip() if not email: email = None #counteract gmail username+2 and extra period tricks - convert submitted email to actual inbox if email and email.endswith("@gmail.com"): gmail_username = email.split('@')[0] gmail_username = gmail_username.split('+')[0] gmail_username = gmail_username.replace('.', '') email = f"{gmail_username}@gmail.com" existing_account = get_user(request.form.get("username"), graceful=True) if existing_account and existing_account.reserved: return redirect(existing_account.permalink) if existing_account or (email and g.db.query(User).filter( User.email.ilike(email)).first()): # #print(f"signup fail - {username } - email already exists") return new_signup( "An account with that username or email already exists.") # check bot if app.config.get("HCAPTCHA_SITEKEY"): token = request.form.get("h-captcha-response") if not token: return new_signup("Unable to verify captcha [1].") data = { "secret": app.config["HCAPTCHA_SECRET"], "response": token, "sitekey": app.config["HCAPTCHA_SITEKEY"] } url = "https://hcaptcha.com/siteverify" x = requests.post(url, data=data) if not x.json()["success"]: #print(x.json()) return new_signup("Unable to verify captcha [2].") # kill tokens session.pop("signup_token") # get referral ref_id = int(request.form.get("referred_by", 0)) # upgrade user badge if ref_id: ref_user = g.db.query(User).options( lazyload('*')).filter_by(id=ref_id).first() if ref_user: ref_user.refresh_selfset_badges() g.db.add(ref_user) # make new user try: new_user = User( username=username, original_username=username, password=request.form.get("password"), email=email, created_utc=int(time.time()), creation_ip=request.remote_addr, referred_by=ref_id or None, tos_agreed_utc=int(time.time()), creation_region=request.headers.get("cf-ipcountry"), ban_evade=int( any([ x.is_banned for x in g.db.query(User).filter( User.id.in_(tuple(session.get("history", [])))).all() if x ]))) except Exception as e: #print(e) return new_signup("Please enter a valid email") g.db.add(new_user) g.db.commit() # give a beta badge beta_badge = Badge(user_id=new_user.id, badge_id=6) g.db.add(beta_badge) # check alts check_for_alts(new_user.id) # send welcome/verify email if email: send_verification_email(new_user) # send welcome message send_notification(new_user, "dude bussy lmao") session["user_id"] = new_user.id session["session_id"] = token_hex(16) redir = request.form.get("redirect", None) # #print(f"Signup event: @{new_user.username}") return redirect("/")