def loadTCGAData(self): slicer.util.openAddVolumeDialog() red_logic = slicer.app.layoutManager().sliceWidget("Red").sliceLogic() red_cn = red_logic.GetSliceCompositeNode() fgrdVolID = red_cn.GetBackgroundVolumeID() fgrdNode = slicer.util.getNode(fgrdVolID) fMat=vtk.vtkMatrix4x4() fgrdNode.GetIJKToRASDirectionMatrix(fMat) bgrdName = fgrdNode.GetName() + '_gray' self.tilename = fgrdNode.GetName() + '_gray' self.parameterNode.SetParameter("SlicerPathology,tilename", self.tilename) # Create dummy grayscale image magnitude = vtk.vtkImageMagnitude() magnitude.SetInputData(fgrdNode.GetImageData()) magnitude.Update() bgrdNode = slicer.vtkMRMLScalarVolumeNode() bgrdNode.SetImageDataConnection(magnitude.GetOutputPort()) bgrdNode.SetName(bgrdName) bgrdNode.SetIJKToRASDirectionMatrix(fMat) slicer.mrmlScene.AddNode(bgrdNode) bgrdVolID = bgrdNode.GetID() red_cn.SetForegroundVolumeID(fgrdVolID) red_cn.SetBackgroundVolumeID(bgrdVolID) red_cn.SetForegroundOpacity(1) self.checkAndSetLUT() print bgrdName cv = slicer.util.getNode(bgrdName) self.volumesLogic = slicer.modules.volumes.logic() labelName = bgrdName+'-label' refLabel = self.volumesLogic.CreateAndAddLabelVolume(slicer.mrmlScene,cv,labelName) refLabel.GetDisplayNode().SetAndObserveColorNodeID(self.SlicerPathologyColorNode.GetID()) self.editorWidget.helper.setMasterVolume(cv)
def Execute(self): if not self.KSpace: self.PrintError('Error: no KSpace.') ifft = vtk.vtkImageRFFT() ifft.SetInput(self.KSpace) ifft.SetDimensionality(self.KSpaceDimensionality) ifft.Update() ifftMagnitude = vtk.vtkImageMagnitude() ifftMagnitude.SetInput(ifft.GetOutput()) ifftMagnitude.Update() origin = self.KSpace.GetOrigin() kspacing = self.KSpace.GetSpacing() dimensions = self.KSpace.GetDimensions() spacing = [1.0/(dimensions[0]*kspacing[0]),1.0/(dimensions[1]*kspacing[1]),1.0/(dimensions[2]*kspacing[2])] imageInformation = vtk.vtkImageChangeInformation() imageInformation.SetInput(ifftMagnitude.GetOutput()) imageInformation.SetOutputSpacing(spacing) imageInformation.SetOutputOrigin(origin) imageInformation.Update() self.Image = imageInformation.GetOutput()
def Execute(self): if not self.KSpace: self.PrintError("Error: no KSpace.") ifft = vtk.vtkImageRFFT() ifft.SetInput(self.KSpace) ifft.SetDimensionality(self.KSpaceDimensionality) ifft.Update() ifftMagnitude = vtk.vtkImageMagnitude() ifftMagnitude.SetInput(ifft.GetOutput()) ifftMagnitude.Update() origin = self.KSpace.GetOrigin() kspacing = self.KSpace.GetSpacing() dimensions = self.KSpace.GetDimensions() spacing = [ 1.0 / (dimensions[0] * kspacing[0]), 1.0 / (dimensions[1] * kspacing[1]), 1.0 / (dimensions[2] * kspacing[2]), ] imageInformation = vtk.vtkImageChangeInformation() imageInformation.SetInput(ifftMagnitude.GetOutput()) imageInformation.SetOutputSpacing(spacing) imageInformation.SetOutputOrigin(origin) imageInformation.Update() self.Image = imageInformation.GetOutput()
def __init__(self, module_manager): SimpleVTKClassModuleBase.__init__( self, module_manager, vtk.vtkImageMagnitude(), 'Processing.', ('vtkImageData',), ('vtkImageData',), replaceDoc=True, inputFunctions=None, outputFunctions=None)
def rfft(self, mode='magnitude'): """Reverse Fast Fourier transform of a picture.""" ffti = vtk.vtkImageRFFT() ffti.SetInputData(self._data) ffti.Update() if 'mag' in mode: mag = vtk.vtkImageMagnitude() mag.SetInputData(ffti.GetOutput()) mag.Update() out = mag.GetOutput() elif 'real' in mode: extractRealFilter = vtk.vtkImageExtractComponents() extractRealFilter.SetInputData(ffti.GetOutput()) extractRealFilter.SetComponents(0) extractRealFilter.Update() out = extractRealFilter.GetOutput() elif 'imaginary' in mode: extractImgFilter = vtk.vtkImageExtractComponents() extractImgFilter.SetInputData(ffti.GetOutput()) extractImgFilter.SetComponents(1) extractImgFilter.Update() out = extractImgFilter.GetOutput() elif 'complex' in mode: out = ffti.GetOutput() else: colors.printc("Error in rfft(): unknown mode", mode) raise RuntimeError() return Picture(out)
def mutate(self): red_logic = slicer.app.layoutManager().sliceWidget("Red").sliceLogic() red_cn = red_logic.GetSliceCompositeNode() fgrdVolID = red_cn.GetBackgroundVolumeID() fgrdNode = slicer.util.getNode("WEB") fgrdVolID = fgrdNode.GetID() fMat=vtk.vtkMatrix4x4() fgrdNode.GetIJKToRASDirectionMatrix(fMat) bgrdName = fgrdNode.GetName() + '_gray' magnitude = vtk.vtkImageMagnitude() magnitude.SetInputData(fgrdNode.GetImageData()) magnitude.Update() bgrdNode = slicer.vtkMRMLScalarVolumeNode() bgrdNode.SetImageDataConnection(magnitude.GetOutputPort()) bgrdNode.SetName(bgrdName) bgrdNode.SetIJKToRASDirectionMatrix(fMat) slicer.mrmlScene.AddNode(bgrdNode) bgrdVolID = bgrdNode.GetID() red_cn.SetForegroundVolumeID(fgrdVolID) red_cn.SetBackgroundVolumeID(bgrdVolID) red_cn.SetForegroundOpacity(1) resourcesPath = os.path.join(slicer.modules.slicerpathology.path.replace("SlicerPathology.py",""), 'Resources') colorFile = os.path.join(resourcesPath, "Colors/SlicerPathology.csv") try: slicer.modules.EditorWidget.helper.structureListWidget.merge = None except AttributeError: pass allColorTableNodes = slicer.util.getNodes('vtkMRMLColorTableNode*').values() for ctn in allColorTableNodes: if ctn.GetName() == 'SlicerPathologyColor': slicer.mrmlScene.RemoveNode(ctn) break SlicerPathologyColorNode = slicer.vtkMRMLColorTableNode() colorNode = SlicerPathologyColorNode colorNode.SetName('SlicerPathologyColor') slicer.mrmlScene.AddNode(colorNode) colorNode.SetTypeToUser() with open(colorFile) as f: n = sum(1 for line in f) colorNode.SetNumberOfColors(n-1) colorNode.NamesInitialisedOn() import csv structureNames = [] with open(colorFile, 'rb') as csvfile: reader = csv.DictReader(csvfile, delimiter=',') for index,row in enumerate(reader): success = colorNode.SetColor(index ,row['Label'],float(row['R'])/255,float(row['G'])/255,float(row['B'])/255,float(row['A'])) if not success: print "color %s could not be set" % row['Label'] structureNames.append(row['Label']) volumesLogic = slicer.modules.volumes.logic() labelName = bgrdName+'-label' refLabel = volumesLogic.CreateAndAddLabelVolume(slicer.mrmlScene,bgrdNode,labelName) refLabel.GetDisplayNode().SetAndObserveColorNodeID(SlicerPathologyColorNode.GetID()) self.editorWidget.helper.setMasterVolume(bgrdNode)
def __init__(self, module_manager): SimpleVTKClassModuleBase.__init__(self, module_manager, vtk.vtkImageMagnitude(), 'Processing.', ('vtkImageData', ), ('vtkImageData', ), replaceDoc=True, inputFunctions=None, outputFunctions=None)
def render_image(png_reader): square = 8 color_map = vtk.vtkLookupTable() color_map.SetNumberOfColors(16) color_map.SetHueRange(0, 0.667) magnitude = vtk.vtkImageMagnitude() magnitude.SetInput(png_reader.GetOutput()) geometry = vtk.vtkImageDataGeometryFilter() geometry.SetInput(magnitude.GetOutput()) warp = vtk.vtkWarpScalar() warp.SetInput(geometry.GetOutput()) warp.SetScaleFactor(0.25) merge = vtk.vtkMergeFilter() merge.SetGeometry(warp.GetOutput()) merge.SetScalars(png_reader.GetOutput()) elevation_mtHood = vtk.vtkElevationFilter() elevation_mtHood.SetInput(merge.GetOutput()) elevation_mtHood.SetLowPoint(0, 0, 0) elevation_mtHood.SetHighPoint(0, 0, 50) mapper_3D_mtHood = vtk.vtkDataSetMapper() mapper_3D_mtHood.SetInput(elevation_mtHood.GetOutput()) mapper_3D_mtHood.SetLookupTable(color_map) mapper_2D_mtHood = vtk.vtkPolyDataMapper2D() mapper_2D_mtHood.SetInput(elevation_mtHood.GetOutput()) mapper_2D_mtHood.SetLookupTable(color_map) actor_2D_mtHood = vtk.vtkActor2D() actor_2D_mtHood.SetMapper(mapper_2D_mtHood) actor_2D_mtHood.GetPositionCoordinate().SetCoordinateSystemToNormalizedDisplay() actor_2D_mtHood.GetPositionCoordinate().SetValue(0.25,0.25) actor_3D_mtHood = vtk.vtkActor() actor_3D_mtHood.SetMapper(mapper_3D_mtHood) renderer = vtk.vtkRenderer() renderWindow = vtk.vtkRenderWindow() renderWindow.AddRenderer(renderer) renderWindowInteractor = vtk.vtkRenderWindowInteractor() renderWindowInteractor.SetRenderWindow(renderWindow) renderer.AddActor(actor_3D_mtHood) renderer.SetBackground(.5, .5, .5) renderWindow.SetSize(600, 600) renderWindow.Render() renderWindowInteractor.Start()
def fft(self, mode='magnitude', logscale=12, center=True): """Fast Fourier transform of a picture. :param float logscale: if non-zero, take the logarithm of the intensity and scale it by this factor. :param str mode: either [magnitude, real, imaginary, complex], compute the point array data accordingly. :param bool center: shift constant zero-frequency to the center of the image for display. (FFT converts spatial images into frequency space, but puts the zero frequency at the origin) """ ffti = vtk.vtkImageFFT() ffti.SetInputData(self._data) ffti.Update() if 'mag' in mode: mag = vtk.vtkImageMagnitude() mag.SetInputData(ffti.GetOutput()) mag.Update() out = mag.GetOutput() elif 'real' in mode: extractRealFilter = vtk.vtkImageExtractComponents() extractRealFilter.SetInputData(ffti.GetOutput()) extractRealFilter.SetComponents(0) extractRealFilter.Update() out = extractRealFilter.GetOutput() elif 'imaginary' in mode: extractImgFilter = vtk.vtkImageExtractComponents() extractImgFilter.SetInputData(ffti.GetOutput()) extractImgFilter.SetComponents(1) extractImgFilter.Update() out = extractImgFilter.GetOutput() elif 'complex' in mode: out = ffti.GetOutput() else: colors.printc("Error in fft(): unknown mode", mode) raise RuntimeError() if center: center = vtk.vtkImageFourierCenter() center.SetInputData(out) center.Update() out = center.GetOutput() if 'complex' not in mode: if logscale: ils = vtk.vtkImageLogarithmicScale() ils.SetInputData(out) ils.SetConstant(logscale) ils.Update() out = ils.GetOutput() return Picture(out)
def Execute(self): if not self.KSpace: self.PrintError('Error: No input KSpace.') imageMathematics = vtk.vtkImageMathematics() imageMathematics.SetInput(self.KSpace) imageMathematics.SetInput2(self.KSpace2) imageMathematics.SetOperationToSubtract() imageMathematics.Update() imageMagnitude = vtk.vtkImageMagnitude() imageMagnitude.SetInput(imageMathematics.GetOutput()) imageMagnitude.Update() self.ErrorImage = imageMagnitude.GetOutput()
def Execute(self): if not self.KSpace: self.PrintError('Error: No input KSpace.') imageMathematics = vtk.vtkImageMathematics() imageMathematics.SetInput(self.KSpace) imageMathematics.SetInput2(self.KSpace2) imageMathematics.SetOperationToSubtract() imageMathematics.Update() imageMagnitude = vtk.vtkImageMagnitude() imageMagnitude.SetInput(imageMathematics.GetOutput()) imageMagnitude.Update() self.ErrorImage = imageMagnitude.GetOutput()
def render_image(png_reader): colorLookup = vtk.vtkLookupTable() colorLookup.SetNumberOfColors(256) colorLookup.SetTableRange(0, 255) for ii in range(0, 256): colorLookup.SetTableValue(ii, 0, 0, 0, 1) magnitude = vtk.vtkImageMagnitude() magnitude.SetInput(png_reader.GetOutput()) geometry = vtk.vtkImageDataGeometryFilter() geometry.SetInput(magnitude.GetOutput()) warp = vtk.vtkWarpScalar() warp.SetInput(geometry.GetOutput()) warp.SetScaleFactor(0.25) merge = vtk.vtkMergeFilter() merge.SetGeometry(warp.GetOutput()) merge.SetGeometry(warp.GetOutput()) merge.SetScalars(png_reader.GetOutput()) mapper = vtk.vtkDataSetMapper() mapper.SetInput(merge.GetOutput()) mapper.ScalarVisibilityOn() mapper.SetLookupTable(colorLookup) # mapper.SetScalarRange(0,255) actor = vtk.vtkActor() actor.SetMapper(mapper) renderer = vtk.vtkRenderer() renderWindow = vtk.vtkRenderWindow() renderWindow.AddRenderer(renderer) renderWindowInteractor = vtk.vtkRenderWindowInteractor() renderWindowInteractor.SetRenderWindow(renderWindow) renderer.AddActor(actor) renderer.SetBackground(0.5, 0.5, 0.5) renderWindow.SetSize(600, 600) renderWindow.Render() renderWindowInteractor.Start()
def render_image(png_reader): colorLookup = vtk.vtkLookupTable() colorLookup.SetNumberOfColors(256) colorLookup.SetTableRange(0, 255) for ii in range(0, 256): colorLookup.SetTableValue(ii, 0, 0, 0, 1) magnitude = vtk.vtkImageMagnitude() magnitude.SetInput(png_reader.GetOutput()) geometry = vtk.vtkImageDataGeometryFilter() geometry.SetInput(magnitude.GetOutput()) warp = vtk.vtkWarpScalar() warp.SetInput(geometry.GetOutput()) warp.SetScaleFactor(0.25) merge = vtk.vtkMergeFilter() merge.SetGeometry(warp.GetOutput()) merge.SetGeometry(warp.GetOutput()) merge.SetScalars(png_reader.GetOutput()) mapper = vtk.vtkDataSetMapper() mapper.SetInput(merge.GetOutput()) mapper.ScalarVisibilityOn() mapper.SetLookupTable(colorLookup) #mapper.SetScalarRange(0,255) actor = vtk.vtkActor() actor.SetMapper(mapper) renderer = vtk.vtkRenderer() renderWindow = vtk.vtkRenderWindow() renderWindow.AddRenderer(renderer) renderWindowInteractor = vtk.vtkRenderWindowInteractor() renderWindowInteractor.SetRenderWindow(renderWindow) renderer.AddActor(actor) renderer.SetBackground(.5, .5, .5) renderWindow.SetSize(600, 600) renderWindow.Render() renderWindowInteractor.Start()
def threshold(self, value=None, flip=False): """ Create a polygonal Mesh from a Picture by filling regions with pixels luminosity above a specified value. Parameters ---------- value : float, optional The default is None, e.i. 1/3 of the scalar range. flip: bool, optional Flip polygon orientations Returns ------- Mesh A polygonal mesh. """ mgf = vtk.vtkImageMagnitude() mgf.SetInputData(self._data) mgf.Update() msq = vtk.vtkMarchingSquares() msq.SetInputData(mgf.GetOutput()) if value is None: r0, r1 = self._data.GetScalarRange() value = r0 + (r1 - r0) / 3 msq.SetValue(0, value) msq.Update() if flip: rs = vtk.vtkReverseSense() rs.SetInputData(msq.GetOutput()) rs.ReverseCellsOn() rs.ReverseNormalsOff() rs.Update() output = rs.GetOutput() else: output = msq.GetOutput() ctr = vtk.vtkContourTriangulator() ctr.SetInputData(output) ctr.Update() return vedo.Mesh(ctr.GetOutput(), c='k').bc('t').lighting('off')
def loademup(self): self.dirty=True import EditorLib editUtil = EditorLib.EditUtil.EditUtil() imsainode = editUtil.getBackgroundVolume() imsai = imsainode.GetImageData() if imsai.GetNumberOfScalarComponents() > 3: lala = self.Four2ThreeChannel(imsai) print lala.GetNumberOfScalarComponents() imsainode.SetAndObserveImageData(lala) imsainode.Modified() red_logic = slicer.app.layoutManager().sliceWidget("Red").sliceLogic() red_cn = red_logic.GetSliceCompositeNode() fgrdVolID = red_cn.GetBackgroundVolumeID() fgrdNode = slicer.util.getNode(fgrdVolID) fMat=vtk.vtkMatrix4x4() fgrdNode.GetIJKToRASDirectionMatrix(fMat) bgrdName = fgrdNode.GetName() + '_gray' self.tilename = fgrdNode.GetName() + '_gray' self.parameterNode.SetParameter("SlicerPathology,tilename", self.tilename) # Create dummy grayscale image magnitude = vtk.vtkImageMagnitude() magnitude.SetInputData(fgrdNode.GetImageData()) magnitude.Update() bgrdNode = slicer.vtkMRMLScalarVolumeNode() bgrdNode.SetImageDataConnection(magnitude.GetOutputPort()) bgrdNode.SetName(bgrdName) bgrdNode.SetIJKToRASDirectionMatrix(fMat) slicer.mrmlScene.AddNode(bgrdNode) bgrdVolID = bgrdNode.GetID() red_cn.SetForegroundVolumeID(fgrdVolID) red_cn.SetBackgroundVolumeID(bgrdVolID) red_cn.SetForegroundOpacity(1) self.checkAndSetLUT() cv = slicer.util.getNode(bgrdName) self.volumesLogic = slicer.modules.volumes.logic() labelName = bgrdName+'-label' refLabel = self.volumesLogic.CreateAndAddLabelVolume(slicer.mrmlScene,cv,labelName) refLabel.GetDisplayNode().SetAndObserveColorNodeID(self.SlicerPathologyColorNode.GetID()) self.editorWidget.helper.setMasterVolume(cv)
def loadTCGAData(self): slicer.util.openAddVolumeDialog() red_logic = slicer.app.layoutManager().sliceWidget("Red").sliceLogic() red_cn = red_logic.GetSliceCompositeNode() fgrdVolID = red_cn.GetBackgroundVolumeID() fgrdNode = slicer.util.getNode(fgrdVolID) fMat = vtk.vtkMatrix4x4() fgrdNode.GetIJKToRASDirectionMatrix(fMat) bgrdName = fgrdNode.GetName() + '_gray' self.tilename = fgrdNode.GetName() + '_gray' self.parameterNode.SetParameter("SlicerPathology,tilename", self.tilename) # Get dummy grayscale image magnitude = vtk.vtkImageMagnitude() magnitude.SetInputData(fgrdNode.GetImageData()) magnitude.Update() bgrdNode = slicer.vtkMRMLScalarVolumeNode() bgrdNode.SetImageDataConnection(magnitude.GetOutputPort()) bgrdNode.SetName(bgrdName) bgrdNode.SetIJKToRASDirectionMatrix(fMat) slicer.mrmlScene.AddNode(bgrdNode) bgrdVolID = bgrdNode.GetID() # Reset slice configuration red_cn.SetForegroundVolumeID(fgrdVolID) red_cn.SetBackgroundVolumeID(bgrdVolID) red_cn.SetForegroundOpacity(1) self.checkAndSetLUT() print bgrdName cv = slicer.util.getNode(bgrdName) self.volumesLogic = slicer.modules.volumes.logic() labelName = bgrdName + '-label' refLabel = self.volumesLogic.CreateAndAddLabelVolume( slicer.mrmlScene, cv, labelName) refLabel.GetDisplayNode().SetAndObserveColorNodeID( self.SlicerPathologyColorNode.GetID()) self.editorWidget.helper.setMasterVolume(cv)
def main(argv): try: opts, args = getopt.getopt(argv, "hi:o:x:a:y:b:z:c:d:u:", [ "ifile=", "ofile=", "sx=", "ex=", "sy=", "ez=", "dataset=", "comp=" ]) except getopt.GetoptError as err: print 'getmultithresh.py -i <inputfile.h5> -o <outputfile.vti> -sx -ex -sy -ey -sz -ez -dataset -comptype' print(str(err)) for opt, arg in opts: if opt == '-h': print 'getmultithresh.py -i <inputfile.h5> -o <outputfile.vti> -sx -ex -sy -ey -sz -ez -dataset' sys.exit() elif opt in ("-i", "--ifile"): inputfile = arg elif opt in ("-o", "--ofile"): outputfile = arg elif opt in ("-x", "--sx"): sx = int(arg) elif opt in ("-a", "--ex"): ex = int(arg) elif opt in ("-y", "--sy"): sy = int(arg) elif opt in ("-b", "--ey"): ey = int(arg) elif opt in ("-z", "--sz"): sz = int(arg) elif opt in ("-c", "--ez"): ez = int(arg) elif opt in ("-d", "--dataset"): dataset = str(arg) elif opt in ("-u", "--du"): comptype = str(arg) print("Loading file, %s" % inputfile) #Determine if file is h5 or numpy if (inputfile.split(".")[1] == "npy"): rs = timeit.default_timer() vel = np.load(inputfile) re = timeit.default_timer() else: #read in file rs = timeit.default_timer() data_file = h5py.File(inputfile, 'r') vel = np.array(data_file[dataset]) data_file.close() re = timeit.default_timer() cs = timeit.default_timer() #convert numpy array to vtk vtkdata = numpy_support.numpy_to_vtk(vel.flat, deep=True, array_type=vtk.VTK_FLOAT) vtkdata.SetNumberOfComponents(3) vtkdata.SetName("Velocity") image = vtk.vtkImageData() image.GetPointData().SetVectors(vtkdata) image.SetExtent(sx, ex, sy, ey, sz, ez) #NOTE: Hardcoding Spacing image.SetSpacing(.006135923, .006135923, .006135923) print("Doing computation") ce = timeit.default_timer() vs = timeit.default_timer() ve = timeit.default_timer() print("Generating contour") ms = timeit.default_timer() mag = vtk.vtkImageMagnitude() for x in range(0, 1): start = timeit.default_timer() threshold = (22.39 * (2.5)) if (comptype == "q"): threshold = (783.3) vort = vtk.vtkGradientFilter() vort.SetInputData(image) vort.SetInputScalars(image.FIELD_ASSOCIATION_POINTS, "Velocity") vort.ComputeQCriterionOn() vort.Update() image.GetPointData().SetScalars( vort.GetOutput().GetPointData().GetVectors("Q-criterion")) else: v = vtk.vtkCellDerivatives() v.SetVectorModeToComputeVorticity() v.SetTensorModeToPassTensors() v.SetInputData(image) v.Update() vort = vtk.vtkImageMagnitude() cp = vtk.vtkCellDataToPointData() cp.SetInputData(v.GetOutput()) cp.Update() image.GetPointData().SetScalars( cp.GetOutput().GetPointData().GetVectors()) vort.SetInputData(image) vort.Update() #ni = vtk.vtkImageData() #ni.SetSpacing(.006135923, .006135923, .006135923) #ni.SetExtent(sx,ex,sy,ey,sz,ez) #ni.GetPointData().SetScalars(q.GetOutput().GetPointData().GetVectors("Q-criterion")) mend = timeit.default_timer() me = mend comptime = mend - start print("Magnitude Computation time: " + str(comptime) + "s") c = vtk.vtkContourFilter() c.SetValue(0, threshold) if (comptype == "q"): c.SetInputData(image) else: c.SetInputData(vort.GetOutput()) print("Computing Contour with threshold", threshold) c.Update() w = vtk.vtkXMLPolyDataWriter() w.SetEncodeAppendedData(0) #turn of base 64 encoding for fast write w.SetFileName(outputfile + str(x) + ".vtp ") w.SetInputData(c.GetOutput()) ws = timeit.default_timer() w.Write() we = timeit.default_timer() print("Results:") print("Read time: %s" % str(re - rs)) print("Convert to vtk: %s" % str(ce - cs)) if (comptype == "q"): print("Q Computation: %s" % str(ve - vs)) print("Q Magnitude: %s" % str(me - ms)) else: print("Vorticity Computation: %s" % str(ve - vs)) print("Vorticity Magnitude: %s" % str(me - ms)) #print ("Threshold: %s" % str(te-ts)) print("Write %s" % str(we - ws)) print("Total time: %s" % str(we - rs))
def getthresh(args): inputfile = args[0] outputfile = args[1] sx = args[2] ex = args[3] sy = args[4] ey = args[5] sz = args[6] ez = args[7] dataset = args[8] comptype = args[9] print("Loading file, %s" % inputfile) #Determine if file is h5 or numpy if (inputfile.split(".")[1] == "npy"): rs = timeit.default_timer() vel = np.load(inputfile) re = timeit.default_timer() else: #read in file rs = timeit.default_timer() data_file = h5py.File(inputfile, 'r') vel = np.array(data_file[dataset]) data_file.close() re = timeit.default_timer() cs = timeit.default_timer() #convert numpy array to vtk vtkdata = numpy_support.numpy_to_vtk(vel.flat, deep=True, array_type=vtk.VTK_FLOAT) vtkdata.SetNumberOfComponents(3) vtkdata.SetName("Velocity") image = vtk.vtkImageData() image.GetPointData().SetVectors(vtkdata) image.SetExtent(sx, ex, sy, ey, sz, ez) #NOTE: Hardcoding Spacing image.SetSpacing(.006135923, .006135923, .006135923) print("Doing computation") ce = timeit.default_timer() vs = timeit.default_timer() if (comptype == "v"): vorticity = vtk.vtkCellDerivatives() vorticity.SetVectorModeToComputeVorticity() vorticity.SetTensorModeToPassTensors() vorticity.SetInputData(image) vorticity.Update() elif (comptype == "q"): vorticity = vtk.vtkGradientFilter() vorticity.SetInputData(image) vorticity.SetInputScalars(image.FIELD_ASSOCIATION_POINTS, "Velocity") vorticity.ComputeQCriterionOn() vorticity.SetComputeGradient(0) vorticity.Update() ve = timeit.default_timer() #Generate contour for comparison c = vtk.vtkContourFilter() c.SetValue(0, 1128) if (comptype == "q"): image.GetPointData().SetScalars( vorticity.GetOutput().GetPointData().GetVectors("Q-criterion")) else: image.GetPointData().SetScalars( vorticity.GetOutput().GetPointData().GetVectors()) c.SetInputData(image) c.Update() w = vtk.vtkXMLPolyDataWriter() w.SetEncodeAppendedData(0) #turn of base 64 encoding for fast write w.SetFileName("contour.vtp") w.SetInputData(c.GetOutput()) ws = timeit.default_timer() w.Write() ms = timeit.default_timer() if (comptype == "v"): mag = vtk.vtkImageMagnitude() cp = vtk.vtkCellDataToPointData() cp.SetInputData(vorticity.GetOutput()) cp.Update() image.GetPointData().SetScalars( cp.GetOutput().GetPointData().GetVectors()) mag.SetInputData(image) mag.Update() m = mag.GetOutput() m.GetPointData().RemoveArray("Velocity") else: image.GetPointData().SetScalars( vorticity.GetOutput().GetPointData().GetVectors("Q-criterion")) me = timeit.default_timer() print("Thresholding.") ts = timeit.default_timer() t = vtk.vtkImageThreshold() #t = vtk.vtkThreshold() #sparse representation if (comptype == "q"): t.SetInputData(image) t.ThresholdByUpper(783.3) #.25*67.17^2 = 1127 #t.SetInputArrayToProcess(0,0,0, vorticity.GetOutput().FIELD_ASSOCIATION_POINTS, "Q-criterion") print("q criterion") else: t.SetInputData(m) t.SetInputArrayToProcess(0, 0, 0, mag.GetOutput().FIELD_ASSOCIATION_POINTS, "Magnitude") t.ThresholdByUpper(44.79) #44.79) #Set values in range to 1 and values out of range to 0 t.SetInValue(1) t.SetOutValue(0) #t.ReplaceInOn() #t.ReplaceOutOn() print("Update thresh") t.Update() #wt = vtk.vtkXMLImageDataWriter() #wt.SetInputData(t.GetOutput()) #wt.SetFileName("thresh.vti") #wt.Write() d = vtk.vtkImageDilateErode3D() d.SetInputData(t.GetOutput()) d.SetKernelSize(3, 3, 3) d.SetDilateValue(1) d.SetErodeValue(0) print("Update dilate") d.Update() iis = vtk.vtkImageToImageStencil() iis.SetInputData(d.GetOutput()) iis.ThresholdByUpper(1) stencil = vtk.vtkImageStencil() stencil.SetInputConnection(2, iis.GetOutputPort()) stencil.SetBackgroundValue(0) #image.GetPointData().RemoveArray("Vorticity") #Set scalars to velocity so it can be cut by the stencil image.GetPointData().SetScalars(image.GetPointData().GetVectors()) #if (comptype == "q"): #Use this to get just q-criterion data instead of velocity data. Do we need both? # image.GetPointData().SetScalars(vorticity.GetOutput().GetPointData().GetScalars("Q-criterion")) stencil.SetInputData(image) print("Update stencil") stencil.Update() te = timeit.default_timer() print("Setting up write") ws = timeit.default_timer() #Make velocity a vector again velarray = stencil.GetOutput().GetPointData().GetScalars() image.GetPointData().RemoveArray("Velocity") image.GetPointData().SetVectors(velarray) w = vtk.vtkXMLImageDataWriter() w.SetCompressorTypeToZLib() #w.SetCompressorTypeToNone() Need to figure out why this fails. w.SetEncodeAppendedData(0) #turn of base 64 encoding for fast write w.SetFileName(outputfile) w.SetInputData(image) if (0): w.SetCompressorTypeToZfp() w.GetCompressor().SetNx(ex - sx + 1) w.GetCompressor().SetNy(ey - sy + 1) w.GetCompressor().SetNz(ez - sz + 1) w.GetCompressor().SetTolerance(1e-1) w.GetCompressor().SetNumComponents(3) w.Write() we = timeit.default_timer() print("Results:") print("Read time: %s" % str(re - rs)) print("Convert to vtk: %s" % str(ce - cs)) if (comptype == "q"): print("Q Computation: %s" % str(ve - vs)) print("Q Magnitude: %s" % str(me - ms)) else: print("Vorticity Computation: %s" % str(ve - vs)) print("Vorticity Magnitude: %s" % str(me - ms)) print("Threshold: %s" % str(te - ts)) print("Write %s" % str(we - ws)) print("Total time: %s" % str(we - rs))
FileNameTemplate[:FileNameTemplate.rfind("/")],timeID) vtkMaskReader = vtk.vtkDataSetReader() vtkMaskReader.SetFileName( maskFileName ) vtkMaskReader.Update() # set threshold vtkImageMask.ThresholdByLower( 100.0) vtkImageMask.SetInput(vtkMaskReader.GetOutput()) except: # if nothing available threshold the phase image # take gradient vtkPhaseData = ConvertNumpyVTKImage( phase_curr ) vtkGradientImage = vtk.vtkImageGradient() vtkGradientImage.SetInput(vtkPhaseData) vtkGradientImage.Update() # take magnitude of gradient vtkImageNorm = vtk.vtkImageMagnitude() vtkImageNorm.SetInput(vtkGradientImage.GetOutput()) vtkImageNorm.Update() # set threshold vtkImageMask.ThresholdByLower( 100.0* tmap_factor * 2.0*numpy.pi/4095.) vtkImageMask.SetInput(vtkImageNorm.GetOutput()) vtkImageMask.Update( ) # check output vtkWriter = vtk.vtkXMLImageDataWriter() vtkWriter.SetFileName("threshold.%04d.vti" % timeID ) vtkWriter.SetInput( vtkImageMask.GetOutput() ) vtkWriter.Update() # pass pointer to c++ image_cells = vtkImageMask.GetOutput().GetPointData() data_array = vtkNumPy.vtk_to_numpy( image_cells.GetArray(0) )
def getvtkdata(self, ci, timestep): PI= 3.141592654 contour=False firstval = ci.datafields.split(',')[0] #print ("First: ", firstval) if ((firstval == 'vo') or (firstval == 'qc') or (firstval == 'cvo') or (firstval == 'pcvo') or (firstval == 'qcc')): datafields = 'u' computation = firstval #We are doing a computation, so we need to know which one. if ((firstval == 'cvo') or (firstval == 'qcc') or (firstval == 'pcvo')): overlap = 3 #This was 2, but due to rounding because of the spacing, 3 is required. #Save a copy of the original request oci = jhtdblib.CutoutInfo() oci.xstart = ci.xstart oci.ystart = ci.ystart oci.zstart = ci.zstart oci.xlen = ci.xlen oci.ylen = ci.ylen oci.zlen = ci.zlen ci = self.expandcutout(ci, overlap) #Expand the cutout by the overlap contour = True else: datafields = ci.datafields.split(',') #There could be multiple components, so we will have to loop computation = '' #Split component into list and add them to the image #Check to see if we have a value for vorticity or q contour fieldlist = list(datafields) image = vtk.vtkImageData() rg = vtk.vtkRectilinearGrid() for field in fieldlist: if (ci.xlen > 61 and ci.ylen > +61 and ci.zlen > 61 and ci.xstep ==1 and ci.ystep ==1 and ci.zstep ==1 and not contour): #Do this if cutout is too large #Note: we don't want to get cubed data if we are doing cubes for contouring. data=GetData().getcubedrawdata(ci, timestep, field) else: data=GetData().getrawdata(ci, timestep, field) vtkdata = numpy_support.numpy_to_vtk(data.flat, deep=True, array_type=vtk.VTK_FLOAT) components = Datafield.objects.get(shortname=field).components vtkdata.SetNumberOfComponents(components) vtkdata.SetName(Datafield.objects.get(shortname=field).longname) #We need to see if we need to subtract one on end of extent edges. image.SetExtent(ci.xstart, ci.xstart+((ci.xlen+ci.xstep-1)/ci.xstep)-1, ci.ystart, ci.ystart+((ci.ylen+ci.ystep-1)/ci.ystep)-1, ci.zstart, ci.zstart+((ci.zlen+ci.zstep-1)/ci.zstep)-1) #image.SetExtent(ci.xstart, ci.xstart+int(ci.xlen)-1, ci.ystart, ci.ystart+int(ci.ylen)-1, ci.zstart, ci.zstart+int(ci.zlen)-1) image.GetPointData().AddArray(vtkdata) if (Datafield.objects.get(shortname=field).longname == "Velocity"): #Set the Velocity Array as vectors in the image. image.GetPointData().SetVectors(image.GetPointData().GetArray("Velocity")) #Get spacing from database and multiply it by the step. Don't do this on the contour--it is performed later on. #if (contour): #We need to scale the threshold to the spacing of the dataset. This is because we build the cubes #on a 1 spacing cube in order to get proper overlap on the contours. #ci.threshold = ci.threshold*Dataset.objects.get(dbname_text=ci.dataset).xspacing #else: xspacing = Dataset.objects.get(dbname_text=ci.dataset).xspacing yspacing = Dataset.objects.get(dbname_text=ci.dataset).yspacing zspacing = Dataset.objects.get(dbname_text=ci.dataset).zspacing #Check if we need a rectilinear grid, and set it up if so. if (ci.dataset == 'channel'): ygrid = jhtdblib.JHTDBLib().getygrid() #print("Ygrid: ") #print (ygrid) #Not sure about contouring channel yet, so we are going back to original variables at this point. rg.SetExtent(ci.xstart, ci.xstart+((ci.xlen+ci.xstep-1)/ci.xstep)-1, ci.ystart, ci.ystart+((ci.ylen+ci.ystep-1)/ci.ystep)-1, ci.zstart, ci.zstart+((ci.zlen+ci.zstep-1)/ci.zstep)-1) #components = Datafield.objects.get(shortname=field).components #vtkdata.SetNumberOfComponents(components) #vtkdata.SetName(Datafield.objects.get(shortname=field).longname) rg.GetPointData().AddArray(vtkdata) #import pdb;pdb.set_trace() #This isn't possible--we will have to do something about this in the future. #rg.SetSpacing(ci.xstep,ci.ystep,ci.zstep) xg = np.arange(0,2047.0) zg = np.arange(0,1535.0) for x in xg: xg[x] = 8*PI/2048*x for z in zg: zg[z] = 3*PI/2048*z vtkxgrid=numpy_support.numpy_to_vtk(xg, deep=True, array_type=vtk.VTK_FLOAT) vtkzgrid=numpy_support.numpy_to_vtk(zg, deep=True, array_type=vtk.VTK_FLOAT) vtkygrid=numpy_support.numpy_to_vtk(ygrid, deep=True, array_type=vtk.VTK_FLOAT) rg.SetXCoordinates(vtkxgrid) rg.SetZCoordinates(vtkzgrid) rg.SetYCoordinates(vtkygrid) image = rg #we rewrite the image since we may be doing a #computation below else: image.SetSpacing(xspacing*ci.xstep,yspacing*ci.ystep,zspacing*ci.zstep) #See if we are doing a computation if (computation == 'vo'): start = time.time() vorticity = vtk.vtkCellDerivatives() vorticity.SetVectorModeToComputeVorticity() vorticity.SetTensorModeToPassTensors() vorticity.SetInputData(image) #print("Computing Vorticity") vorticity.Update() end = time.time() comptime = end-start print("Vorticity Computation time: " + str(comptime) + "s") return image elif (computation == 'cvo' or computation == 'pcvo'): start = time.time() vorticity = vtk.vtkCellDerivatives() vorticity.SetVectorModeToComputeVorticity() vorticity.SetTensorModeToPassTensors() vorticity.SetInputData(image) #print("Computing Voricity") vorticity.Update() vend = time.time() comptime = vend-start print("Vorticity Computation time: " + str(comptime) + "s") mag = vtk.vtkImageMagnitude() cp = vtk.vtkCellDataToPointData() cp.SetInputData(vorticity.GetOutput()) #print("Computing magnitude") cp.Update() mend = time.time() image.GetPointData().SetScalars(cp.GetOutput().GetPointData().GetVectors()) mag.SetInputData(image) mag.Update() comptime = mend-vend print("Magnitude Computation time: " + str(comptime) + "s") c = vtk.vtkContourFilter() c.SetValue(0,ci.threshold) c.SetInputData(mag.GetOutput()) print("Computing Contour with threshold", ci.threshold) c.Update() cend = time.time() comptime = cend-mend print("Contour Computation time: " + str(comptime) + "s") #Now we need to clip out the overlap box = vtk.vtkBox() #set box to requested size #The OCI deepcopy didn't seem to work. Manually taking the overlap again. box.SetBounds(oci.xstart*xspacing, (oci.xstart+oci.xlen)*xspacing, oci.ystart*yspacing, (oci.ystart+oci.ylen)*yspacing, oci.zstart*yspacing,(oci.zstart+oci.zlen)*yspacing) clip = vtk.vtkClipPolyData() clip.SetClipFunction(box) clip.GenerateClippedOutputOn() clip.SetInputData(c.GetOutput()) clip.InsideOutOn() clip.Update() #import pdb;pdb.set_trace() cropdata = clip.GetOutput() #Cleanup image.ReleaseData() #mag.ReleaseData() #box.ReleaseData() #clip.ReleaseData() #image.Delete() #box.Delete() #vorticity.Delete() end = time.time() comptime = end-start print("Total Computation time: " + str(comptime) + "s") #return cropdata #We need the output port for appending, so return the clip instead return clip elif (computation == 'qcc'): start = time.time() q = vtk.vtkGradientFilter() q.SetInputData(image) q.SetInputScalars(image.FIELD_ASSOCIATION_POINTS,"Velocity") q.ComputeQCriterionOn() q.Update() image.GetPointData().SetScalars(q.GetOutput().GetPointData().GetVectors("Q-criterion")) #mag = vtk.vtkImageMagnitude() #mag.SetInputData(image) #mag.Update() mend = time.time() comptime = mend-start #print("Magnitude Computation time: " + str(comptime) + "s") c = vtk.vtkContourFilter() c.SetValue(0,ci.threshold) c.SetInputData(image) print("Computing Contour with threshold", ci.threshold) c.Update() cend = time.time() comptime = cend-mend print("Q Contour Computation time: " + str(comptime) + "s") #clip out the overlap here box = vtk.vtkBox() #set box to requested size box.SetBounds(oci.xstart, oci.xstart+oci.xlen-1, oci.ystart, oci.ystart+oci.ylen-1, oci.zstart,oci.zstart+oci.zlen-1) clip = vtk.vtkClipPolyData() clip.SetClipFunction(box) clip.GenerateClippedOutputOn() clip.SetInputData(c.GetOutput()) clip.InsideOutOn() clip.Update() cropdata = clip.GetOutput() end = time.time() comptime = end-start print("Computation time: " + str(comptime) + "s") #return cropdata return clip else: return image
def __init__( self, inputobj=None, c='RdBu_r', alpha=(0.0, 0.0, 0.2, 0.4, 0.8, 1.0), alphaGradient=None, alphaUnit=1, mode=0, shade=False, spacing=None, dims=None, origin=None, mapper='smart', ): vtk.vtkVolume.__init__(self) BaseGrid.__init__(self) ################### if isinstance(inputobj, str): if "https://" in inputobj: from vedo.io import download inputobj = download(inputobj, verbose=False) # fpath elif os.path.isfile(inputobj): pass else: inputobj = sorted(glob.glob(inputobj)) ################### if 'gpu' in mapper: self._mapper = vtk.vtkGPUVolumeRayCastMapper() elif 'opengl_gpu' in mapper: self._mapper = vtk.vtkOpenGLGPUVolumeRayCastMapper() elif 'smart' in mapper: self._mapper = vtk.vtkSmartVolumeMapper() elif 'fixed' in mapper: self._mapper = vtk.vtkFixedPointVolumeRayCastMapper() elif isinstance(mapper, vtk.vtkMapper): self._mapper = mapper else: print("Error unknown mapper type", [mapper]) raise RuntimeError() self.SetMapper(self._mapper) ################### inputtype = str(type(inputobj)) #colors.printc('Volume inputtype', inputtype) if inputobj is None: img = vtk.vtkImageData() elif utils.isSequence(inputobj): if isinstance(inputobj[0], str): # scan sequence of BMP files ima = vtk.vtkImageAppend() ima.SetAppendAxis(2) pb = utils.ProgressBar(0, len(inputobj)) for i in pb.range(): f = inputobj[i] picr = vtk.vtkBMPReader() picr.SetFileName(f) picr.Update() mgf = vtk.vtkImageMagnitude() mgf.SetInputData(picr.GetOutput()) mgf.Update() ima.AddInputData(mgf.GetOutput()) pb.print('loading...') ima.Update() img = ima.GetOutput() else: if "ndarray" not in inputtype: inputobj = np.array(inputobj) if len(inputobj.shape) == 1: varr = numpy_to_vtk(inputobj, deep=True, array_type=vtk.VTK_FLOAT) else: if len(inputobj.shape) > 2: inputobj = np.transpose(inputobj, axes=[2, 1, 0]) varr = numpy_to_vtk(inputobj.ravel(order='F'), deep=True, array_type=vtk.VTK_FLOAT) varr.SetName('input_scalars') img = vtk.vtkImageData() if dims is not None: img.SetDimensions(dims) else: if len(inputobj.shape) == 1: colors.printc( "Error: must set dimensions (dims keyword) in Volume.", c='r') raise RuntimeError() img.SetDimensions(inputobj.shape) img.GetPointData().SetScalars(varr) #to convert rgb to numpy # img_scalar = data.GetPointData().GetScalars() # dims = data.GetDimensions() # n_comp = img_scalar.GetNumberOfComponents() # temp = numpy_support.vtk_to_numpy(img_scalar) # numpy_data = temp.reshape(dims[1],dims[0],n_comp) # numpy_data = numpy_data.transpose(0,1,2) # numpy_data = np.flipud(numpy_data) elif "ImageData" in inputtype: img = inputobj elif isinstance(inputobj, Volume): img = inputobj.inputdata() elif "UniformGrid" in inputtype: img = inputobj elif hasattr( inputobj, "GetOutput"): # passing vtk object, try extract imagdedata if hasattr(inputobj, "Update"): inputobj.Update() img = inputobj.GetOutput() elif isinstance(inputobj, str): from vedo.io import loadImageData, download if "https://" in inputobj: inputobj = download(inputobj, verbose=False) img = loadImageData(inputobj) else: colors.printc("Volume(): cannot understand input type:\n", inputtype, c='r') return if dims is not None: img.SetDimensions(dims) if origin is not None: img.SetOrigin(origin) ### DIFFERENT from volume.origin()! if spacing is not None: img.SetSpacing(spacing) self._data = img self._mapper.SetInputData(img) self.mode(mode).color(c).alpha(alpha).alphaGradient(alphaGradient) self.GetProperty().SetShade(True) self.GetProperty().SetInterpolationType(1) self.GetProperty().SetScalarOpacityUnitDistance(alphaUnit) # remember stuff: self._mode = mode self._color = c self._alpha = alpha self._alphaGrad = alphaGradient self._alphaUnit = alphaUnit
scalardata = img1_data[x][y][z] if scalardata < intRange[0]: scalardata = intRange[0] if scalardata > intRange[1]: scalardata = intRange[1] scalardata = max_u_short * np.float( scalardata - intRange[0]) / np.float(intRange[1] - intRange[0]) image.SetScalarComponentFromFloat(x, y, z, 0, scalardata) gradientFilter = vtk.vtkImageGradient() #生成梯度图 gradientFilter.SetInputData(image) gradientFilter.SetDimensionality(3) gradientFilter.Update() ################################################# magnitude = vtk.vtkImageMagnitude() #生成梯度模值图 magnitude.SetInputConnection(gradientFilter.GetOutputPort()) magnitude.Update() imageCast = vtk.vtkImageCast() imageCast.SetInputConnection(magnitude.GetOutputPort()) imageCast.SetOutputScalarTypeToUnsignedShort() imageCast.Update() magimage = imageCast.GetOutput() # Create transfer mapping scalar value to opacity opacityTransferFunction = vtk.vtkPiecewiseFunction() opacityTransferFunction.AddPoint(100, 0.0) opacityTransferFunction.AddSegment(101, 0.3, 400, 0.5) opacityTransferFunction.AddPoint(500, 0.0) opacityTransferFunction.ClampingOff()
def __init__( self, inputobj=None, c=('b', 'lb', 'lg', 'y', 'r'), alpha=(0.0, 0.0, 0.2, 0.4, 0.8, 1), alphaGradient=None, mode=0, origin=None, spacing=None, shape=None, mapperType='smart', ): vtk.vtkVolume.__init__(self) ActorBase.__init__(self) inputtype = str(type(inputobj)) #colors.printc('Volume inputtype', inputtype) if isinstance(inputobj, str): import glob inputobj = sorted(glob.glob(inputobj)) if inputobj is None: img = vtk.vtkImageData() elif utils.isSequence(inputobj): if isinstance(inputobj[0], str): # scan sequence of BMP files ima = vtk.vtkImageAppend() ima.SetAppendAxis(2) pb = utils.ProgressBar(0, len(inputobj)) for i in pb.range(): f = inputobj[i] picr = vtk.vtkBMPReader() picr.SetFileName(f) picr.Update() mgf = vtk.vtkImageMagnitude() mgf.SetInputData(picr.GetOutput()) mgf.Update() ima.AddInputData(mgf.GetOutput()) pb.print('loading..') ima.Update() img = ima.GetOutput() else: if "ndarray" not in inputtype: inputobj = np.array(inputobj) varr = numpy_to_vtk(inputobj.ravel(order='F'), deep=True, array_type=vtk.VTK_FLOAT) varr.SetName('input_scalars') img = vtk.vtkImageData() if shape is not None: img.SetDimensions(shape) else: img.SetDimensions(inputobj.shape) img.GetPointData().SetScalars(varr) #to convert rgb to numpy # img_scalar = data.GetPointData().GetScalars() # dims = data.GetDimensions() # n_comp = img_scalar.GetNumberOfComponents() # temp = numpy_support.vtk_to_numpy(img_scalar) # numpy_data = temp.reshape(dims[1],dims[0],n_comp) # numpy_data = numpy_data.transpose(0,1,2) # numpy_data = np.flipud(numpy_data) elif "ImageData" in inputtype: img = inputobj elif "UniformGrid" in inputtype: img = inputobj elif "UnstructuredGrid" in inputtype: img = inputobj mapperType = 'tetra' elif hasattr( inputobj, "GetOutput"): # passing vtk object, try extract imagdedata if hasattr(inputobj, "Update"): inputobj.Update() img = inputobj.GetOutput() else: colors.printc("Volume(): cannot understand input type:\n", inputtype, c=1) return if 'gpu' in mapperType: self._mapper = vtk.vtkGPUVolumeRayCastMapper() elif 'opengl_gpu' in mapperType: self._mapper = vtk.vtkOpenGLGPUVolumeRayCastMapper() elif 'smart' in mapperType: self._mapper = vtk.vtkSmartVolumeMapper() elif 'fixed' in mapperType: self._mapper = vtk.vtkFixedPointVolumeRayCastMapper() elif 'tetra' in mapperType: self._mapper = vtk.vtkProjectedTetrahedraMapper() elif 'unstr' in mapperType: self._mapper = vtk.vtkUnstructuredGridVolumeRayCastMapper() else: print("Error unknown mapperType", mapperType) raise RuntimeError() if origin is not None: img.SetOrigin(origin) if spacing is not None: img.SetSpacing(spacing) if shape is not None: img.SetDimensions(shape) self._imagedata = img self._mapper.SetInputData(img) self.SetMapper(self._mapper) self.mode(mode).color(c).alpha(alpha).alphaGradient(alphaGradient) self.GetProperty().SetInterpolationType(1) # remember stuff: self._mode = mode self._color = c self._alpha = alpha self._alphaGrad = alphaGradient
imageIn.SetFileName("" + str(VTK_DATA_ROOT) + "/Data/earth.ppm") il = vtk.vtkImageLuminance() il.SetInputConnection(imageIn.GetOutputPort()) ic = vtk.vtkImageCast() ic.SetOutputScalarTypeToFloat() ic.SetInputConnection(il.GetOutputPort()) # smooth the image gs = vtk.vtkImageGaussianSmooth() gs.SetInputConnection(ic.GetOutputPort()) gs.SetDimensionality(2) gs.SetRadiusFactors(1,1,0) # gradient the image imgGradient = vtk.vtkImageGradient() imgGradient.SetInputConnection(gs.GetOutputPort()) imgGradient.SetDimensionality(2) imgMagnitude = vtk.vtkImageMagnitude() imgMagnitude.SetInputConnection(imgGradient.GetOutputPort()) imgMagnitude.Update() # non maximum suppression nonMax = vtk.vtkImageNonMaximumSuppression() nonMax.SetMagnitudeInputData(imgMagnitude.GetOutput()) nonMax.SetVectorInputData(imgGradient.GetOutput()) nonMax.SetDimensionality(2) pad = vtk.vtkImageConstantPad() pad.SetInputConnection(imgGradient.GetOutputPort()) pad.SetOutputNumberOfScalarComponents(3) pad.SetConstant(0) pad.Update() i2sp1 = vtk.vtkImageToStructuredPoints() i2sp1.SetInputConnection(nonMax.GetOutputPort()) i2sp1.SetVectorInputData(pad.GetOutput())
def getvtkimage(self, webargs, timestep): #Setup query DBSTRING = os.environ['db_connection_string'] conn = pyodbc.connect(DBSTRING, autocommit=True) cursor = conn.cursor() #url = "http://localhost:8000/cutout/getcutout/"+ token + "/" + dataset + "/" + datafield + "/" + ts + "," +te + "/" + xs + "," + xe +"/" + ys + "," + ye +"/" + zs + "," + ze w = webargs.split("/") ts = int(w[3].split(',')[0]) te = int(w[3].split(',')[1]) xs = int(w[4].split(',')[0]) xe = int(w[4].split(',')[1]) ys = int(w[5].split(',')[0]) ye = int(w[5].split(',')[1]) zs = int(w[6].split(',')[0]) ze = int(w[6].split(',')[1]) extent = (xs, ys, zs, xe, ye, ze) overlap = 2 #Used only on contours--vorticity and Q-criterion #Look for step parameters if (len(w) > 9): step = True; s = w[8].split(",") tstep = s[0] xstep = float(s[1]) ystep = float(s[2]) zstep = float(s[3]) filterwidth = w[9] else: step = False; xstep = 1 ystep = 1 zstep = 1 filterwidth = 1 cfieldlist = w[2].split(",") firstval = cfieldlist[0] maxrange = self.getmaxrange(w[1]) if ((firstval == 'vo') or (firstval == 'qc') or (firstval == 'cvo') or (firstval == 'qcc')): component = 'u' computation = firstval #We are doing a computation, so we need to know which one. #check to see if we have a threshold (only for contours) if (len(cfieldlist) > 1): threshold = float(cfieldlist[1]) else: threshold = .6 #New: We need an expanded cutout if contouring. Push the cutout out by 2 in all directions (unless at boundary). if ((firstval == 'cvo') or (firstval == 'qcc')): newextent = self.expandcutout(extent, maxrange[0], maxrange[1], maxrange[2], overlap) contour = True else: component = w[2] #There could be multiple components, so we will have to loop computation = '' #Split component into list and add them to the image #Check to see if we have a value for vorticity or q contour fieldlist = list(component) for field in fieldlist: print("Field = %s" % field) cursor.execute("{CALL turbdev.dbo.GetAnyCutout(?,?,?,?,?,?,?,?,?,?,?,?,?,?,?,?)}",w[1], field, timestep, extent[0], extent[1], extent[2], xstep, ystep, zstep, 1,1,extent[3], extent[4], extent[5],filterwidth,1) #If data spans across multiple servers, we get multiple sets, so concatenate them. row = cursor.fetchone() raw = row[0] part = 0 print ("First part size is %d" % len(row[0])) while(cursor.nextset()): row = cursor.fetchone() raw = raw + row[0] part = part +1 print ("added part %d" % part) print ("Part size is %d" % len(row[0])) print ("Raw size is %d" % len(raw)) data = np.frombuffer(raw, dtype=np.float32) conn.close() vtkdata = numpy_support.numpy_to_vtk(data, deep=True, array_type=vtk.VTK_FLOAT) components = self.numcomponents(field) vtkdata.SetNumberOfComponents(components) vtkdata.SetName(self.componentname(field)) image = vtk.vtkImageData() if (step): xes = int(extent[3])/int(xstep)-1 yes = int(extent[4])/int(ystep)-1 zes = int(extent[5])/int(zstep)-1 image.SetExtent(extent[0], extent[0]+extent[3], extent[1], extent[1]+extent[4], extent[2], extenet[2]+extenet[5]) print("Step extent=" +str(xes)) print("xs=" + str(xstep) + " ys = "+ str(ystep) +" zs = " + str(zstep)) else: image.SetExtent(extent[0], extent[0]+extent[3]-1, extent[1], extent[1]+extent[4]-1, extent[2], extent[2]+extent[5]-1) image.GetPointData().SetVectors(vtkdata) if (step): #Magnify to original size image.SetSpacing(xstep,ystep,zstep) #Check if we need a rectilinear grid, and set it up if so. if (w[1] == 'channel'): ygrid = self.getygrid() #print("Ygrid: ") #print (ygrid) rg = vtk.vtkRectilinearGrid() #Not sure about contouring channel yet, so we are going back to original variables at this point. rg.SetExtent(xs, xs+xe-1, ys, ys+ye-1, zs, zs+ze-1) rg.GetPointData().SetVectors(vtkdata) xg = np.arange(float(xs),float(xe)) zg = np.arange(float(zs),float(ze)) for x in xg: xg[x] = 8*3.141592654/2048*x for z in zg: zg[z] = 3*3.141592654/2048*z vtkxgrid=numpy_support.numpy_to_vtk(xg, deep=True, array_type=vtk.VTK_FLOAT) vtkzgrid=numpy_support.numpy_to_vtk(zg, deep=True, array_type=vtk.VTK_FLOAT) vtkygrid=numpy_support.numpy_to_vtk(ygrid, deep=True, array_type=vtk.VTK_FLOAT) rg.SetXCoordinates(vtkxgrid) rg.SetZCoordinates(vtkzgrid) rg.SetYCoordinates(vtkygrid) image = rg #we rewrite the image since we may be doing a #computation below #See if we are doing a computation if (computation == 'vo'): vorticity = vtk.vtkCellDerivatives() vorticity.SetVectorModeToComputeVorticity() vorticity.SetTensorModeToPassTensors() vorticity.SetInputData(image) print("Computing Vorticity") vorticity.Update() elif (computation == 'cvo'): vorticity = vtk.vtkCellDerivatives() vorticity.SetVectorModeToComputeVorticity() vorticity.SetTensorModeToPassTensors() vorticity.SetInputData(image) print("Computing Voricity") vorticity.Update() mag = vtk.vtkImageMagnitude() cp = vtk.vtkCellDataToPointData() cp.SetInputData(vorticity.GetOutput()) print("Computing magnitude") cp.Update() image.GetPointData().SetScalars(cp.GetOutput().GetPointData().GetVectors()) mag.SetInputData(image) mag.Update() c = vtk.vtkContourFilter() c.SetValue(0,threshold) c.SetInputData(mag.GetOutput()) print("Computing Contour") c.Update() #Now we need to clip out the overlap box = vtk.vtkBox() #set box to requested size box.SetBounds(xs, xs+xe-1, ys, ys+ye-1, zs,zs+ze-1) clip = vtk.vtkClipPolyData() clip.SetClipFunction(box) clip.GenerateClippedOutputOn() clip.SetInputData(c.GetOutput()) clip.InsideOutOn() clip.Update() cropdata = clip.GetOutput() return cropdata elif (computation == 'qcc'): q = vtk.vtkGradientFilter() q.SetInputData(image) q.SetInputScalars(image.FIELD_ASSOCIATION_POINTS,"Velocity") q.ComputeQCriterionOn() q.Update() #newimage = vtk.vtkImageData() image.GetPointData().SetScalars(q.GetOutput().GetPointData().GetVectors("Q-criterion")) mag = vtk.vtkImageMagnitude() mag.SetInputData(image) mag.Update() c = vtk.vtkContourFilter() c.SetValue(0,threshold) c.SetInputData(mag.GetOutput()) c.Update() #clip out the overlap here box = vtk.vtkBox() #set box to requested size box.SetBounds(xs, xs+xe-1, ys, ys+ye-1, zs,zs+ze-1) clip = vtk.vtkClipPolyData() clip.SetClipFunction(box) clip.GenerateClippedOutputOn() clip.SetInputData(c.GetOutput()) clip.InsideOutOn() clip.Update() cropdata = clip.GetOutput() return cropdata else: return image
FileNameTemplate[:FileNameTemplate.rfind("/")], timeID) vtkMaskReader = vtk.vtkDataSetReader() vtkMaskReader.SetFileName(maskFileName) vtkMaskReader.Update() # set threshold vtkImageMask.ThresholdByLower(100.0) vtkImageMask.SetInput(vtkMaskReader.GetOutput()) except: # if nothing available threshold the phase image # take gradient vtkPhaseData = ConvertNumpyVTKImage(phase_curr) vtkGradientImage = vtk.vtkImageGradient() vtkGradientImage.SetInput(vtkPhaseData) vtkGradientImage.Update() # take magnitude of gradient vtkImageNorm = vtk.vtkImageMagnitude() vtkImageNorm.SetInput(vtkGradientImage.GetOutput()) vtkImageNorm.Update() # set threshold vtkImageMask.ThresholdByLower(100.0 * tmap_factor * 2.0 * numpy.pi / 4095.) vtkImageMask.SetInput(vtkImageNorm.GetOutput()) vtkImageMask.Update() # check output vtkWriter = vtk.vtkXMLImageDataWriter() vtkWriter.SetFileName("threshold.%04d.vti" % timeID) vtkWriter.SetInput(vtkImageMask.GetOutput()) vtkWriter.Update() # pass pointer to c++ image_cells = vtkImageMask.GetOutput().GetPointData()
def vortvelvolume(args): #inputfile, outputfile, sx, ex, sy, ey, sz, ez, dataset): p = args[0] cubenum = args[1] print("Cube", cubenum) #Check for additonal parameters if (p["param1"] != ''): comptype = p["param1"] else: comptype = "q" #Default to q criterion if (p["param2"] != ''): thresh = float(p["param2"]) else: thresh = 783.3 #Default for q threshold on isotropic data #We use 0 for structured grid (vti) and 1 for unstructured grid (vtu) if (p["param3"] != ''): grid = 0 else: grid = 1 if (p["param4"] != ''): kernelsize = int(p["param4"]) else: kernelsize = 3 inputfile = p["inputfile"] +str(cubenum) + ".npy" outputfile = p["outputfile"] + str(cubenum) + ".vti" #always VTK Image Data for this. sx = p["sx"] sy = p["sy"] sz = p["sz"] ex = p["ex"] ey = p["ey"] ez = p["ez"] print ("Loading file, %s" % inputfile) #Determine if file is h5 or numpy rs = timeit.default_timer() vel = np.load(inputfile) re = timeit.default_timer() #convert numpy array to vtk cs = timeit.default_timer() #convert numpy array to vtk vtkdata = numpy_support.numpy_to_vtk(vel.flat, deep=True, array_type=vtk.VTK_FLOAT) vtkdata.SetNumberOfComponents(3) vtkdata.SetName("Velocity") image = vtk.vtkImageData() image.GetPointData().SetVectors(vtkdata) image.SetExtent(sx,ex,sy,ey,sz,ez) #NOTE: Hardcoding Spacing image.SetSpacing(.006135923, .006135923, .006135923) ce = timeit.default_timer() vs = timeit.default_timer() if (comptype == "v"): vorticity = vtk.vtkCellDerivatives() vorticity.SetVectorModeToComputeVorticity() vorticity.SetTensorModeToPassTensors() vorticity.SetInputData(image) vorticity.Update() elif (comptype == "q"): vorticity = vtk.vtkGradientFilter() vorticity.SetInputData(image) vorticity.SetInputScalars(image.FIELD_ASSOCIATION_POINTS,"Velocity") vorticity.ComputeQCriterionOn() vorticity.SetComputeGradient(0) vorticity.Update() ve = timeit.default_timer() ms = timeit.default_timer() if (comptype == "v"): mag = vtk.vtkImageMagnitude() cp = vtk.vtkCellDataToPointData() cp.SetInputData(vorticity.GetOutput()) cp.Update() image.GetPointData().SetScalars(cp.GetOutput().GetPointData().GetVectors()) mag.SetInputData(image) mag.Update() m = mag.GetOutput() m.GetPointData().RemoveArray("Velocity") else: image.GetPointData().SetScalars(vorticity.GetOutput().GetPointData().GetVectors("Q-criterion")) me = timeit.default_timer() print ("Thresholding.") ts = timeit.default_timer() t = vtk.vtkImageThreshold() #t = vtk.vtkThreshold() #sparse representation if (comptype == "q"): t.SetInputData(image) t.ThresholdByUpper(thresh) #.25*67.17^2 = 1127 #t.SetInputArrayToProcess(0,0,0, vorticity.GetOutput().FIELD_ASSOCIATION_POINTS, "Q-criterion") print("q criterion") else: t.SetInputData(m) t.SetInputArrayToProcess(0,0,0, mag.GetOutput().FIELD_ASSOCIATION_POINTS, "Magnitude") t.ThresholdByUpper(thresh) #44.79) #Set values in range to 1 and values out of range to 0 t.SetInValue(1) t.SetOutValue(0) #t.ReplaceInOn() #t.ReplaceOutOn() print("Update thresh") t.Update() #wt = vtk.vtkXMLImageDataWriter() #wt.SetInputData(t.GetOutput()) #wt.SetFileName("thresh.vti") #wt.Write() d = vtk.vtkImageDilateErode3D() d.SetInputData(t.GetOutput()) d.SetKernelSize(kernelsize,kernelsize,kernelsize) d.SetDilateValue(1) d.SetErodeValue(0) print ("Update dilate") d.Update() iis = vtk.vtkImageToImageStencil() iis.SetInputData(d.GetOutput()) iis.ThresholdByUpper(1) stencil = vtk.vtkImageStencil() stencil.SetInputConnection(2, iis.GetOutputPort()) stencil.SetBackgroundValue(0) #image.GetPointData().RemoveArray("Vorticity") #Set scalars to velocity so it can be cut by the stencil image.GetPointData().SetScalars(image.GetPointData().GetVectors()) #if (comptype == "q"): #Use this to get just q-criterion data instead of velocity data. Do we need both? # image.GetPointData().SetScalars(vorticity.GetOutput().GetPointData().GetScalars("Q-criterion")) stencil.SetInputData(image) print ("Update stencil") stencil.Update() te = timeit.default_timer() print("Setting up write") ws = timeit.default_timer() #Make velocity a vector again velarray = stencil.GetOutput().GetPointData().GetScalars() image.GetPointData().RemoveArray("Velocity") image.GetPointData().SetVectors(velarray) if (grid == 0): w = vtk.vtkXMLImageDataWriter() else: w = vtk.vtkXMLUnstructuredGridWriter() w.SetCompressorTypeToZLib() #w.SetCompressorTypeToNone() Need to figure out why this fails. w.SetEncodeAppendedData(0) #turn of base 64 encoding for fast write w.SetFileName(outputfile) w.SetInputData(image) if (0): w.SetCompressorTypeToZfp() w.GetCompressor().SetNx(ex-sx+1) w.GetCompressor().SetNy(ey-sy+1) w.GetCompressor().SetNz(ez-sz+1) w.GetCompressor().SetTolerance(1e-2) w.GetCompressor().SetNumComponents(3) result = w.Write() result = 1 #don't write for benchmarking we = timeit.default_timer() print("Results:") print("Read time: %s" % str(re-rs)) print ("Convert to vtk: %s" % str(ce-cs)) if (comptype == "q"): print ("Q Computation: %s" % str(ve-vs)) print ("Q Magnitude: %s" % str(me-ms)) else: print ("Vorticity Computation: %s" % str(ve-vs)) print ("Vorticity Magnitude: %s" % str(me-ms)) print ("Threshold: %s" % str(te-ts)) print ("Write %s" % str(we-ws)) print ("Total time: %s" % str(we-rs)) if (result): p["message"] = "Success" p["computetime"] = str(we-rs) return p #return the packet
def getthresh(args): inputfile = args[0] outputfile = args[1] sx = args[2] ex = args[3] sy = args[4] ey = args[5] sz = args[6] ez = args[7] dataset = args[8] comptype = args[9] print ("Loading file, %s" % inputfile) #Determine if file is h5 or numpy if (inputfile.split(".")[1] == "npy"): rs = timeit.default_timer() vel = np.load(inputfile) re = timeit.default_timer() else: #read in file rs = timeit.default_timer() data_file = h5py.File(inputfile, 'r') vel = np.array(data_file[dataset]) data_file.close() re = timeit.default_timer() cs = timeit.default_timer() #convert numpy array to vtk vtkdata = numpy_support.numpy_to_vtk(vel.flat, deep=True, array_type=vtk.VTK_FLOAT) vtkdata.SetNumberOfComponents(3) vtkdata.SetName("Velocity") image = vtk.vtkImageData() image.GetPointData().SetVectors(vtkdata) image.SetExtent(sx,ex,sy,ey,sz,ez) #NOTE: Hardcoding Spacing image.SetSpacing(.006135923, .006135923, .006135923) print ("Doing computation") ce = timeit.default_timer() vs = timeit.default_timer() if (comptype == "v"): vorticity = vtk.vtkCellDerivatives() vorticity.SetVectorModeToComputeVorticity() vorticity.SetTensorModeToPassTensors() vorticity.SetInputData(image) vorticity.Update() elif (comptype == "q"): vorticity = vtk.vtkGradientFilter() vorticity.SetInputData(image) vorticity.SetInputScalars(image.FIELD_ASSOCIATION_POINTS,"Velocity") vorticity.ComputeQCriterionOn() vorticity.SetComputeGradient(0) vorticity.Update() ve = timeit.default_timer() #Generate contour for comparison c = vtk.vtkContourFilter() c.SetValue(0,1128) if (comptype == "q"): image.GetPointData().SetScalars(vorticity.GetOutput().GetPointData().GetVectors("Q-criterion")) else: image.GetPointData().SetScalars(vorticity.GetOutput().GetPointData().GetVectors()) c.SetInputData(image) c.Update() w = vtk.vtkXMLPolyDataWriter() w.SetEncodeAppendedData(0) #turn of base 64 encoding for fast write w.SetFileName("contour.vtp") w.SetInputData(c.GetOutput()) ws = timeit.default_timer() w.Write() ms = timeit.default_timer() if (comptype == "v"): mag = vtk.vtkImageMagnitude() cp = vtk.vtkCellDataToPointData() cp.SetInputData(vorticity.GetOutput()) cp.Update() image.GetPointData().SetScalars(cp.GetOutput().GetPointData().GetVectors()) mag.SetInputData(image) mag.Update() m = mag.GetOutput() m.GetPointData().RemoveArray("Velocity") else: image.GetPointData().SetScalars(vorticity.GetOutput().GetPointData().GetVectors("Q-criterion")) me = timeit.default_timer() print ("Thresholding.") ts = timeit.default_timer() t = vtk.vtkImageThreshold() #t = vtk.vtkThreshold() #sparse representation if (comptype == "q"): t.SetInputData(image) t.ThresholdByUpper(783.3) #.25*67.17^2 = 1127 #t.SetInputArrayToProcess(0,0,0, vorticity.GetOutput().FIELD_ASSOCIATION_POINTS, "Q-criterion") print("q criterion") else: t.SetInputData(m) t.SetInputArrayToProcess(0,0,0, mag.GetOutput().FIELD_ASSOCIATION_POINTS, "Magnitude") t.ThresholdByUpper(44.79) #44.79) #Set values in range to 1 and values out of range to 0 t.SetInValue(1) t.SetOutValue(0) #t.ReplaceInOn() #t.ReplaceOutOn() print("Update thresh") t.Update() #wt = vtk.vtkXMLImageDataWriter() #wt.SetInputData(t.GetOutput()) #wt.SetFileName("thresh.vti") #wt.Write() d = vtk.vtkImageDilateErode3D() d.SetInputData(t.GetOutput()) d.SetKernelSize(3,3,3) d.SetDilateValue(1) d.SetErodeValue(0) print ("Update dilate") d.Update() iis = vtk.vtkImageToImageStencil() iis.SetInputData(d.GetOutput()) iis.ThresholdByUpper(1) stencil = vtk.vtkImageStencil() stencil.SetInputConnection(2, iis.GetOutputPort()) stencil.SetBackgroundValue(0) #image.GetPointData().RemoveArray("Vorticity") #Set scalars to velocity so it can be cut by the stencil image.GetPointData().SetScalars(image.GetPointData().GetVectors()) #if (comptype == "q"): #Use this to get just q-criterion data instead of velocity data. Do we need both? # image.GetPointData().SetScalars(vorticity.GetOutput().GetPointData().GetScalars("Q-criterion")) stencil.SetInputData(image) print ("Update stencil") stencil.Update() te = timeit.default_timer() print("Setting up write") ws = timeit.default_timer() #Make velocity a vector again velarray = stencil.GetOutput().GetPointData().GetScalars() image.GetPointData().RemoveArray("Velocity") image.GetPointData().SetVectors(velarray) w = vtk.vtkXMLImageDataWriter() w.SetCompressorTypeToZLib() #w.SetCompressorTypeToNone() Need to figure out why this fails. w.SetEncodeAppendedData(0) #turn of base 64 encoding for fast write w.SetFileName(outputfile) w.SetInputData(image) if (0): w.SetCompressorTypeToZfp() w.GetCompressor().SetNx(ex-sx+1) w.GetCompressor().SetNy(ey-sy+1) w.GetCompressor().SetNz(ez-sz+1) w.GetCompressor().SetTolerance(1e-1) w.GetCompressor().SetNumComponents(3) w.Write() we = timeit.default_timer() print("Results:") print("Read time: %s" % str(re-rs)) print ("Convert to vtk: %s" % str(ce-cs)) if (comptype == "q"): print ("Q Computation: %s" % str(ve-vs)) print ("Q Magnitude: %s" % str(me-ms)) else: print ("Vorticity Computation: %s" % str(ve-vs)) print ("Vorticity Magnitude: %s" % str(me-ms)) print ("Threshold: %s" % str(te-ts)) print ("Write %s" % str(we-ws)) print ("Total time: %s" % str(we-rs))
def __init__(self, inputobj=None): vtk.vtkImageSlice.__init__(self) Base3DProp.__init__(self) BaseVolume.__init__(self) self._mapper = vtk.vtkImageResliceMapper() self._mapper.SliceFacesCameraOn() self._mapper.SliceAtFocalPointOn() self._mapper.SetAutoAdjustImageQuality(False) self._mapper.BorderOff() self.lut = None self.property = vtk.vtkImageProperty() self.property.SetInterpolationTypeToLinear() self.SetProperty(self.property) ################### if isinstance(inputobj, str): if "https://" in inputobj: from vedo.io import download inputobj = download(inputobj, verbose=False) # fpath elif os.path.isfile(inputobj): pass else: inputobj = sorted(glob.glob(inputobj)) ################### inputtype = str(type(inputobj)) if inputobj is None: img = vtk.vtkImageData() if isinstance(inputobj, Volume): img = inputobj.imagedata() self.lut = utils.ctf2lut(inputobj) elif utils.isSequence(inputobj): if isinstance(inputobj[0], str): # scan sequence of BMP files ima = vtk.vtkImageAppend() ima.SetAppendAxis(2) pb = utils.ProgressBar(0, len(inputobj)) for i in pb.range(): f = inputobj[i] picr = vtk.vtkBMPReader() picr.SetFileName(f) picr.Update() mgf = vtk.vtkImageMagnitude() mgf.SetInputData(picr.GetOutput()) mgf.Update() ima.AddInputData(mgf.GetOutput()) pb.print('loading...') ima.Update() img = ima.GetOutput() else: if "ndarray" not in inputtype: inputobj = np.array(inputobj) if len(inputobj.shape) == 1: varr = utils.numpy2vtk(inputobj, dtype=float) else: if len(inputobj.shape) > 2: inputobj = np.transpose(inputobj, axes=[2, 1, 0]) varr = utils.numpy2vtk(inputobj.ravel(order='F'), dtype=float) varr.SetName('input_scalars') img = vtk.vtkImageData() img.SetDimensions(inputobj.shape) img.GetPointData().AddArray(varr) img.GetPointData().SetActiveScalars(varr.GetName()) elif "ImageData" in inputtype: img = inputobj elif isinstance(inputobj, Volume): img = inputobj.inputdata() elif "UniformGrid" in inputtype: img = inputobj elif hasattr( inputobj, "GetOutput"): # passing vtk object, try extract imagdedata if hasattr(inputobj, "Update"): inputobj.Update() img = inputobj.GetOutput() elif isinstance(inputobj, str): from vedo.io import loadImageData, download if "https://" in inputobj: inputobj = download(inputobj, verbose=False) img = loadImageData(inputobj) else: colors.printc("VolumeSlice: cannot understand input type:\n", inputtype, c='r') return self._data = img self._mapper.SetInputData(img) self.SetMapper(self._mapper)
def vortvelvolumei(args): #inputfile, outputfile, sx, ex, sy, ey, sz, ez, dataset): p = args[0] cubenum = args[1] print("Cube", cubenum) #Check for additonal parameters if (p["param1"] != ''): comptype = p["param1"] else: comptype = "q" #Default to q criterion if (p["param2"] != ''): thresh = float(p["param2"]) else: thresh = 783.3 #Default for q threshold on isotropic data inputfile = p["inputfile"] +str(cubenum) + ".npy" outputfile = p["outputfile"] + str(cubenum) + ".vti" #always VTK Image Data for this. sx = p["sx"] sy = p["sy"] sz = p["sz"] ex = p["ex"] ey = p["ey"] ez = p["ez"] print ("Loading file, %s" % inputfile) #Determine if file is h5 or numpy rs = timeit.default_timer() vel = np.load(inputfile) print ("File Loaded") re = timeit.default_timer() #convert numpy array to vtk cs = timeit.default_timer() #convert numpy array to vtk vtkdata = numpy_support.numpy_to_vtk(vel.flat, deep=True, array_type=vtk.VTK_FLOAT) vtkdata.SetNumberOfComponents(3) vtkdata.SetName("Velocity") image = vtk.vtkImageData() image.GetPointData().SetVectors(vtkdata) image.SetExtent(sx,ex,sy,ey,sz,ez) #NOTE: Hardcoding Spacing image.SetSpacing(.006135923, .006135923, .006135923) ce = timeit.default_timer() vs = timeit.default_timer() print ("Beginning computation: " + comptype) if (comptype == "v"): vorticity = vtk.vtkCellDerivatives() vorticity.SetVectorModeToComputeVorticity() vorticity.SetTensorModeToPassTensors() vorticity.SetInputData(image) vorticity.Update() elif (comptype == "q"): vorticity = vtk.vtkGradientFilter() vorticity.SetInputData(image) vorticity.SetInputScalars(image.FIELD_ASSOCIATION_POINTS,"Velocity") vorticity.ComputeQCriterionOn() vorticity.SetComputeGradient(0) vorticity.Update() ve = timeit.default_timer() print("Initial calculation done") ms = timeit.default_timer() if (comptype == "v"): mag = vtk.vtkImageMagnitude() cp = vtk.vtkCellDataToPointData() cp.SetInputData(vorticity.GetOutput()) cp.Update() image.GetPointData().SetScalars(cp.GetOutput().GetPointData().GetVectors()) mag.SetInputData(image) mag.Update() m = mag.GetOutput() m.GetPointData().RemoveArray("Velocity") else: image.GetPointData().SetScalars(vorticity.GetOutput().GetPointData().GetVectors("Q-criterion")) me = timeit.default_timer() print("Generating screenshot") c = vtk.vtkContourFilter() c.SetValue(0,thresh) c.SetInputData(image) c.Update() contour = c.GetOutput() mapper = vtk.vtkPolyDataMapper() mapper.SetInputData(contour) mapper.ScalarVisibilityOn() mapper.SetScalarRange(-1,1) mapper.SetScalarModeToUsePointFieldData() mapper.ColorByArrayComponent("Velocity", 0) actor = vtk.vtkActor() actor.SetMapper(mapper) ren = vtk.vtkRenderer() ren.AddActor(actor) ren.SetBackground(1,1,1) camera = vtk.vtkCamera() ren.SetActiveCamera(camera) ren.ResetCamera() camera.Zoom(1.5) #This reduces the whitespace around the image renWin = vtk.vtkRenderWindow() renWin.SetSize(1024,1024) renWin.AddRenderer(ren) renWin.SetOffScreenRendering(1) windowToImageFilter = vtk.vtkWindowToImageFilter() windowToImageFilter.SetInput(renWin) windowToImageFilter.Update() w = vtk.vtkPNGWriter() pngfilename = p["outputfile"] + str(cubenum) + ".png" w.SetFileName(pngfilename) w.SetInputConnection(windowToImageFilter.GetOutputPort()) w.Write() #Shift camera angle and take snapshots around the cube. for aznum in range(4): camera.Azimuth(90) windowToImageFilter = vtk.vtkWindowToImageFilter() windowToImageFilter.SetInput(renWin) windowToImageFilter.Update() pngfilename = p["outputfile"] + str(cubenum) + "-r" + str(aznum)+ ".png" w.SetFileName(pngfilename) w.SetInputConnection(windowToImageFilter.GetOutputPort()) w.Write() camera.Elevation(90) #Rotate camera to top windowToImageFilter = vtk.vtkWindowToImageFilter() windowToImageFilter.SetInput(renWin) windowToImageFilter.Update() pngfilename = p["outputfile"] + str(cubenum) + "-t1.png" w.SetFileName(pngfilename) w.SetInputConnection(windowToImageFilter.GetOutputPort()) w.Write() camera.Elevation(180) #Rotate camera to bottom windowToImageFilter = vtk.vtkWindowToImageFilter() windowToImageFilter.SetInput(renWin) windowToImageFilter.Update() pngfilename = p["outputfile"] + str(cubenum) + "-b1.png" w.SetFileName(pngfilename) w.SetInputConnection(windowToImageFilter.GetOutputPort()) w.Write() print ("Thresholding.") ts = timeit.default_timer() t = vtk.vtkImageThreshold() #Dense represenation (0's included, structured grid) #t = vtk.vtkThreshold() #sparse representation if (comptype == "q"): t.SetInputData(image) t.ThresholdByUpper(thresh) #.25*67.17^2 = 1127 #t.SetInputArrayToProcess(0,0,0, vorticity.GetOutput().FIELD_ASSOCIATION_POINTS, "Q-criterion") print("q criterion") else: t.SetInputData(m) t.SetInputArrayToProcess(0,0,0, mag.GetOutput().FIELD_ASSOCIATION_POINTS, "Magnitude") t.ThresholdByUpper(thresh) #44.79) #Set values in range to 1 and values out of range to 0 t.SetInValue(1) t.SetOutValue(0) #t.ReplaceInOn() #t.ReplaceOutOn() print("Update thresh") t.Update() #wt = vtk.vtkXMLImageDataWriter() #wt.SetInputData(t.GetOutput()) #wt.SetFileName("thresh.vti") #wt.Write() d = vtk.vtkImageDilateErode3D() d.SetInputData(t.GetOutput()) d.SetKernelSize(4,4,4) d.SetDilateValue(1) d.SetErodeValue(0) print ("Update dilate") d.Update() iis = vtk.vtkImageToImageStencil() iis.SetInputData(d.GetOutput()) iis.ThresholdByUpper(1) stencil = vtk.vtkImageStencil() stencil.SetInputConnection(2, iis.GetOutputPort()) stencil.SetBackgroundValue(0) #image.GetPointData().RemoveArray("Vorticity") #Set scalars to velocity so it can be cut by the stencil image.GetPointData().SetScalars(image.GetPointData().GetVectors()) #if (comptype == "q"): #Use this to get just q-criterion data instead of velocity data. Do we need both? # image.GetPointData().SetScalars(vorticity.GetOutput().GetPointData().GetScalars("Q-criterion")) stencil.SetInputData(image) print ("Update stencil") stencil.Update() te = timeit.default_timer() print("Setting up write") ws = timeit.default_timer() #Make velocity a vector again velarray = stencil.GetOutput().GetPointData().GetScalars() image.GetPointData().RemoveArray("Velocity") image.GetPointData().SetVectors(velarray) w = vtk.vtkXMLImageDataWriter() w.SetCompressorTypeToZLib() #w.SetCompressorTypeToNone() Need to figure out why this fails. w.SetEncodeAppendedData(0) #turn of base 64 encoding for fast write w.SetFileName(outputfile) w.SetInputData(image) if (0): w.SetCompressorTypeToZfp() w.GetCompressor().SetNx(ex-sx+1) w.GetCompressor().SetNy(ey-sy+1) w.GetCompressor().SetNz(ez-sz+1) w.GetCompressor().SetTolerance(1e-2) w.GetCompressor().SetNumComponents(3) #result = w.Write() result = 1 #don't write for benchmarking we = timeit.default_timer() print("Results:") print("Read time: %s" % str(re-rs)) print ("Convert to vtk: %s" % str(ce-cs)) if (comptype == "q"): print ("Q Computation: %s" % str(ve-vs)) print ("Q Magnitude: %s" % str(me-ms)) else: print ("Vorticity Computation: %s" % str(ve-vs)) print ("Vorticity Magnitude: %s" % str(me-ms)) print ("Threshold: %s" % str(te-ts)) print ("Write %s" % str(we-ws)) print ("Total time: %s" % str(we-rs)) if (result): p["message"] = "Success" p["computetime"] = str(we-rs) return p #return the packet
def main(): colors = vtk.vtkNamedColors() fileName = get_program_parameters() # Read the image. readerFactory = vtk.vtkImageReader2Factory() reader = readerFactory.CreateImageReader2(fileName) reader.SetFileName(fileName) reader.Update() fft = vtk.vtkImageFFT() fft.SetInputConnection(reader.GetOutputPort()) mag = vtk.vtkImageMagnitude() mag.SetInputConnection(fft.GetOutputPort()) center = vtk.vtkImageFourierCenter() center.SetInputConnection(mag.GetOutputPort()) compress = vtk.vtkImageLogarithmicScale() compress.SetInputConnection(center.GetOutputPort()) compress.SetConstant(15) compress.Update() # Create the actors. originalActor = vtk.vtkImageActor() originalActor.GetMapper().SetInputConnection(reader.GetOutputPort()) originalActor.GetProperty().SetInterpolationTypeToNearest() compressedActor = vtk.vtkImageActor() compressedActor.GetMapper().SetInputConnection(compress.GetOutputPort()) compressedActor.GetProperty().SetInterpolationTypeToNearest() CreateImageActor(compressedActor, 160, 120) # Define the viewport ranges. # (xmin, ymin, xmax, ymax) originalViewport = [0.0, 0.0, 0.5, 1.0] compressedViewport = [0.5, 0.0, 1.0, 1.0] # Setup the renderers. originalRenderer = vtk.vtkRenderer() originalRenderer.SetViewport(originalViewport) originalRenderer.AddActor(originalActor) originalRenderer.ResetCamera() originalRenderer.SetBackground(colors.GetColor3d("SlateGray")) compressedRenderer = vtk.vtkRenderer() compressedRenderer.SetViewport(compressedViewport) compressedRenderer.AddActor(compressedActor) compressedRenderer.ResetCamera() compressedRenderer.SetBackground(colors.GetColor3d("LightSlateGray")) renderWindow = vtk.vtkRenderWindow() renderWindow.SetSize(600, 300) renderWindow.SetWindowName('VTKSpectrum') renderWindow.AddRenderer(originalRenderer) renderWindow.AddRenderer(compressedRenderer) renderWindowInteractor = vtk.vtkRenderWindowInteractor() style = vtk.vtkInteractorStyleImage() renderWindowInteractor.SetInteractorStyle(style) renderWindowInteractor.SetRenderWindow(renderWindow) renderWindowInteractor.Initialize() renderWindowInteractor.Start()
def magnitude(self): """Colapses components with magnitude function.""" imgm = vtk.vtkImageMagnitude() imgm.SetInputData(self.imagedata()) imgm.Update() return self._update(imgm.GetOutput())
def main(argv): try: opts, args = getopt.getopt(argv,"hi:o:x:a:y:b:z:c:d:u:", ["ifile=","ofile=","sx=","ex=","sy=","ez=","dataset=","comp="]) except getopt.GetoptError as err: print 'getmultithresh.py -i <inputfile.h5> -o <outputfile.vti> -sx -ex -sy -ey -sz -ez -dataset -comptype' print (str(err)) for opt, arg in opts: if opt == '-h': print 'getmultithresh.py -i <inputfile.h5> -o <outputfile.vti> -sx -ex -sy -ey -sz -ez -dataset' sys.exit() elif opt in ("-i", "--ifile"): inputfile = arg elif opt in ("-o", "--ofile"): outputfile = arg elif opt in ("-x", "--sx"): sx = int(arg) elif opt in ("-a", "--ex"): ex = int(arg) elif opt in ("-y", "--sy"): sy = int(arg) elif opt in ("-b", "--ey"): ey = int(arg) elif opt in ("-z", "--sz"): sz = int(arg) elif opt in ("-c", "--ez"): ez = int(arg) elif opt in ("-d", "--dataset"): dataset = str(arg) elif opt in ("-u", "--du"): comptype = str(arg) print ("Loading file, %s" % inputfile) #Determine if file is h5 or numpy if (inputfile.split(".")[1] == "npy"): rs = timeit.default_timer() vel = np.load(inputfile) re = timeit.default_timer() else: #read in file rs = timeit.default_timer() data_file = h5py.File(inputfile, 'r') vel = np.array(data_file[dataset]) data_file.close() re = timeit.default_timer() cs = timeit.default_timer() #convert numpy array to vtk vtkdata = numpy_support.numpy_to_vtk(vel.flat, deep=True, array_type=vtk.VTK_FLOAT) vtkdata.SetNumberOfComponents(3) vtkdata.SetName("Velocity") image = vtk.vtkImageData() image.GetPointData().SetVectors(vtkdata) image.SetExtent(sx,ex,sy,ey,sz,ez) #NOTE: Hardcoding Spacing image.SetSpacing(.006135923, .006135923, .006135923) print ("Doing computation") ce = timeit.default_timer() vs = timeit.default_timer() ve = timeit.default_timer() print ("Generating contour") ms = timeit.default_timer() mag = vtk.vtkImageMagnitude() for x in range (0,1): start = timeit.default_timer() threshold = (22.39 * (2.5)) if (comptype == "q"): threshold= (783.3) vort = vtk.vtkGradientFilter() vort.SetInputData(image) vort.SetInputScalars(image.FIELD_ASSOCIATION_POINTS,"Velocity") vort.ComputeQCriterionOn() vort.Update() image.GetPointData().SetScalars(vort.GetOutput().GetPointData().GetVectors("Q-criterion")) else: v = vtk.vtkCellDerivatives() v.SetVectorModeToComputeVorticity() v.SetTensorModeToPassTensors() v.SetInputData(image) v.Update() vort = vtk.vtkImageMagnitude() cp = vtk.vtkCellDataToPointData() cp.SetInputData(v.GetOutput()) cp.Update() image.GetPointData().SetScalars(cp.GetOutput().GetPointData().GetVectors()) vort.SetInputData(image) vort.Update() #ni = vtk.vtkImageData() #ni.SetSpacing(.006135923, .006135923, .006135923) #ni.SetExtent(sx,ex,sy,ey,sz,ez) #ni.GetPointData().SetScalars(q.GetOutput().GetPointData().GetVectors("Q-criterion")) mend = timeit.default_timer() me = mend comptime = mend-start print("Magnitude Computation time: " + str(comptime) + "s") c = vtk.vtkContourFilter() c.SetValue(0,threshold) if (comptype == "q"): c.SetInputData(image) else: c.SetInputData(vort.GetOutput()) print("Computing Contour with threshold", threshold) c.Update() w = vtk.vtkXMLPolyDataWriter() w.SetEncodeAppendedData(0) #turn of base 64 encoding for fast write w.SetFileName(outputfile + str(x) + ".vtp ") w.SetInputData(c.GetOutput()) ws = timeit.default_timer() w.Write() we = timeit.default_timer() print("Results:") print("Read time: %s" % str(re-rs)) print ("Convert to vtk: %s" % str(ce-cs)) if (comptype == "q"): print ("Q Computation: %s" % str(ve-vs)) print ("Q Magnitude: %s" % str(me-ms)) else: print ("Vorticity Computation: %s" % str(ve-vs)) print ("Vorticity Magnitude: %s" % str(me-ms)) #print ("Threshold: %s" % str(te-ts)) print ("Write %s" % str(we-ws)) print ("Total time: %s" % str(we-rs))
def operation(self, operation, volume2=None): """ Perform operations with ``Volume`` objects. `volume2` can be a constant value. Possible operations are: ``+``, ``-``, ``/``, ``1/x``, ``sin``, ``cos``, ``exp``, ``log``, ``abs``, ``**2``, ``sqrt``, ``min``, ``max``, ``atan``, ``atan2``, ``median``, ``mag``, ``dot``, ``gradient``, ``divergence``, ``laplacian``. |volumeOperations| |volumeOperations.py|_ """ op = operation.lower() image1 = self._data if op in ["median"]: mf = vtk.vtkImageMedian3D() mf.SetInputData(image1) mf.Update() return Volume(mf.GetOutput()) elif op in ["mag"]: mf = vtk.vtkImageMagnitude() mf.SetInputData(image1) mf.Update() return Volume(mf.GetOutput()) elif op in ["dot", "dotproduct"]: mf = vtk.vtkImageDotProduct() mf.SetInput1Data(image1) mf.SetInput2Data(volume2._data) mf.Update() return Volume(mf.GetOutput()) elif op in ["grad", "gradient"]: mf = vtk.vtkImageGradient() mf.SetDimensionality(3) mf.SetInputData(image1) mf.Update() return Volume(mf.GetOutput()) elif op in ["div", "divergence"]: mf = vtk.vtkImageDivergence() mf.SetInputData(image1) mf.Update() return Volume(mf.GetOutput()) elif op in ["laplacian"]: mf = vtk.vtkImageLaplacian() mf.SetDimensionality(3) mf.SetInputData(image1) mf.Update() return Volume(mf.GetOutput()) mat = vtk.vtkImageMathematics() mat.SetInput1Data(image1) K = None if isinstance(volume2, (int, float)): K = volume2 mat.SetConstantK(K) mat.SetConstantC(K) elif volume2 is not None: # assume image2 is a constant value mat.SetInput2Data(volume2._data) if op in ["+", "add", "plus"]: if K: mat.SetOperationToAddConstant() else: mat.SetOperationToAdd() elif op in ["-", "subtract", "minus"]: if K: mat.SetConstantC(-K) mat.SetOperationToAddConstant() else: mat.SetOperationToSubtract() elif op in ["*", "multiply", "times"]: if K: mat.SetOperationToMultiplyByK() else: mat.SetOperationToMultiply() elif op in ["/", "divide"]: if K: mat.SetConstantK(1.0 / K) mat.SetOperationToMultiplyByK() else: mat.SetOperationToDivide() elif op in ["1/x", "invert"]: mat.SetOperationToInvert() elif op in ["sin"]: mat.SetOperationToSin() elif op in ["cos"]: mat.SetOperationToCos() elif op in ["exp"]: mat.SetOperationToExp() elif op in ["log"]: mat.SetOperationToLog() elif op in ["abs"]: mat.SetOperationToAbsoluteValue() elif op in ["**2", "square"]: mat.SetOperationToSquare() elif op in ["sqrt", "sqr"]: mat.SetOperationToSquareRoot() elif op in ["min"]: mat.SetOperationToMin() elif op in ["max"]: mat.SetOperationToMax() elif op in ["atan"]: mat.SetOperationToATAN() elif op in ["atan2"]: mat.SetOperationToATAN2() else: colors.printc("\times Error in volumeOperation: unknown operation", operation, c='r') raise RuntimeError() mat.Update() return self._update(mat.GetOutput())
def imageOperation(image1, operation='+', image2=None): ''' Perform operations with vtkImageData objects. Image2 can contain a constant value. Possible operations are: +, -, /, 1/x, sin, cos, exp, log, abs, **2, sqrt, min, max, atan, atan2, median, mag, dot, gradient, divergence, laplacian. [**Example**](https://github.com/marcomusy/vtkplotter/blob/master/examples/volumetric/imageOperations.py) 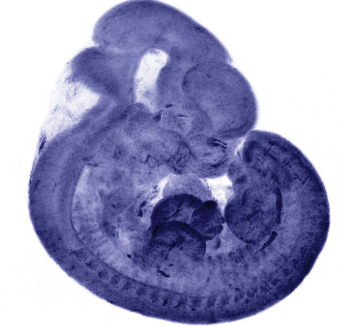 ''' op = operation.lower() if op in ['median']: mf = vtk.vtkImageMedian3D() mf.SetInputData(image1) mf.Update() return mf.GetOutput() elif op in ['mag']: mf = vtk.vtkImageMagnitude() mf.SetInputData(image1) mf.Update() return mf.GetOutput() elif op in ['dot', 'dotproduct']: mf = vtk.vtkImageDotProduct() mf.SetInput1Data(image1) mf.SetInput2Data(image2) mf.Update() return mf.GetOutput() elif op in ['grad', 'gradient']: mf = vtk.vtkImageGradient() mf.SetDimensionality(3) mf.SetInputData(image1) mf.Update() return mf.GetOutput() elif op in ['div', 'divergence']: mf = vtk.vtkImageDivergence() mf.SetInputData(image1) mf.Update() return mf.GetOutput() elif op in ['laplacian']: mf = vtk.vtkImageLaplacian() mf.SetDimensionality(3) mf.SetInputData(image1) mf.Update() return mf.GetOutput() mat = vtk.vtkImageMathematics() mat.SetInput1Data(image1) K = None if image2: if isinstance(image2, vtk.vtkImageData): mat.SetInput2Data(image2) else: # assume image2 is a constant value K = image2 mat.SetConstantK(K) mat.SetConstantC(K) if op in ['+', 'add', 'plus']: if K: mat.SetOperationToAddConstant() else: mat.SetOperationToAdd() elif op in ['-', 'subtract', 'minus']: if K: mat.SetConstantC(-K) mat.SetOperationToAddConstant() else: mat.SetOperationToSubtract() elif op in ['*', 'multiply', 'times']: if K: mat.SetOperationToMultiplyByK() else: mat.SetOperationToMultiply() elif op in ['/', 'divide']: if K: mat.SetConstantK(1.0/K) mat.SetOperationToMultiplyByK() else: mat.SetOperationToDivide() elif op in ['1/x', 'invert']: mat.SetOperationToInvert() elif op in ['sin']: mat.SetOperationToSin() elif op in ['cos']: mat.SetOperationToCos() elif op in ['exp']: mat.SetOperationToExp() elif op in ['log']: mat.SetOperationToLog() elif op in ['abs']: mat.SetOperationToAbsoluteValue() elif op in ['**2', 'square']: mat.SetOperationToSquare() elif op in ['sqrt', 'sqr']: mat.SetOperationToSquareRoot() elif op in ['min']: mat.SetOperationToMin() elif op in ['max']: mat.SetOperationToMax() elif op in ['atan']: mat.SetOperationToATAN() elif op in ['atan2']: mat.SetOperationToATAN2() else: vc.printc('Error in imageOperation: unknown operation', operation, c=1) exit() mat.Update() return mat.GetOutput()
import vtk print vtk.VTK_VERSION r = vtk.vtkXMLImageDataReader() r.SetFileName("iso0_67.vti") r.Update() image = r.GetOutput() vorticity = vtk.vtkCellDerivatives() vorticity.SetVectorModeToComputeVorticity() vorticity.SetTensorModeToPassTensors() vorticity.SetInputData(image) vorticity.Update() mag = vtk.vtkImageMagnitude() cp = vtk.vtkCellDataToPointData() cp.SetInputData(vorticity.GetOutput()) cp.Update() image.GetPointData().SetScalars(cp.GetOutput().GetPointData().GetVectors()) mag.SetInputData(image) mag.Update() #Remove velocity field #m = mag.GetOutput() #m.GetPointData().RemoveArray("Velocity") t = vtk.vtkImageThreshold() t.SetInputData(mag.GetOutput()) t.SetInputArrayToProcess(0,0,0, mag.GetOutput().FIELD_ASSOCIATION_POINTS, "Magnitude") #t.ThresholdByUpper(44.79)
import vtk print vtk.VTK_VERSION r = vtk.vtkXMLImageDataReader() r.SetFileName("iso0_67.vti") r.Update() image = r.GetOutput() vorticity = vtk.vtkCellDerivatives() vorticity.SetVectorModeToComputeVorticity() vorticity.SetTensorModeToPassTensors() vorticity.SetInputData(image) vorticity.Update() mag = vtk.vtkImageMagnitude() cp = vtk.vtkCellDataToPointData() cp.SetInputData(vorticity.GetOutput()) cp.Update() image.GetPointData().SetScalars(cp.GetOutput().GetPointData().GetVectors()) mag.SetInputData(image) mag.Update() #Remove velocity field #m = mag.GetOutput() #m.GetPointData().RemoveArray("Velocity") t = vtk.vtkImageThreshold() t.SetInputData(mag.GetOutput()) t.SetInputArrayToProcess(0, 0, 0, mag.GetOutput().FIELD_ASSOCIATION_POINTS, "Magnitude")
imageIn.SetFileName("" + str(VTK_DATA_ROOT) + "/Data/earth.ppm") il = vtk.vtkImageLuminance() il.SetInputConnection(imageIn.GetOutputPort()) ic = vtk.vtkImageCast() ic.SetOutputScalarTypeToFloat() ic.SetInputConnection(il.GetOutputPort()) # smooth the image gs = vtk.vtkImageGaussianSmooth() gs.SetInputConnection(ic.GetOutputPort()) gs.SetDimensionality(2) gs.SetRadiusFactors(1, 1, 0) # gradient the image imgGradient = vtk.vtkImageGradient() imgGradient.SetInputConnection(gs.GetOutputPort()) imgGradient.SetDimensionality(2) imgMagnitude = vtk.vtkImageMagnitude() imgMagnitude.SetInputConnection(imgGradient.GetOutputPort()) imgMagnitude.Update() # non maximum suppression nonMax = vtk.vtkImageNonMaximumSuppression() nonMax.SetMagnitudeInputData(imgMagnitude.GetOutput()) nonMax.SetVectorInputData(imgGradient.GetOutput()) nonMax.SetDimensionality(2) pad = vtk.vtkImageConstantPad() pad.SetInputConnection(imgGradient.GetOutputPort()) pad.SetOutputNumberOfScalarComponents(3) pad.SetConstant(0) pad.Update() i2sp1 = vtk.vtkImageToStructuredPoints() i2sp1.SetInputConnection(nonMax.GetOutputPort()) i2sp1.SetVectorInputData(pad.GetOutput())
def setupPipeline(): #read file global reader reader = vtk.vtkOBJReader() reader.SetFileName(filename) #map 3d model global mapper mapper = vtk.vtkPolyDataMapper() mapper.SetInputConnection(reader.GetOutputPort()) #set actor global actor actor = vtk.vtkActor() actor.SetMapper(mapper) # Create a rendering window and renderer global ren ren = vtk.vtkRenderer() ren.SetBackground(0, 0, 0) ren.AddActor(actor) global intermediateWindow intermediateWindow = vtk.vtkRenderWindow() intermediateWindow.AddRenderer(ren) #render image global renImage renImage = vtk.vtkRenderLargeImage() renImage.SetInput(ren) renImage.SetMagnification(magnificationFactor) #Canny edge detector inspired by #https://vtk.org/Wiki/VTK/Examples/Cxx/Images/CannyEdgeDetector #to grayscale global lumImage lumImage = vtk.vtkImageLuminance() lumImage.SetInputConnection(renImage.GetOutputPort()) #to float global floatImage floatImage = vtk.vtkImageCast() floatImage.SetOutputScalarTypeToFloat() floatImage.SetInputConnection(lumImage.GetOutputPort()) #gaussian convolution global smoothImage smoothImage = vtk.vtkImageGaussianSmooth() smoothImage.SetInputConnection(floatImage.GetOutputPort()) smoothImage.SetDimensionality(2) smoothImage.SetRadiusFactors(1, 1, 0) #gradient global gradientImage gradientImage = vtk.vtkImageGradient() gradientImage.SetInputConnection(smoothImage.GetOutputPort()) gradientImage.SetDimensionality(2) #gradient magnitude global magnitudeImage magnitudeImage = vtk.vtkImageMagnitude() magnitudeImage.SetInputConnection(gradientImage.GetOutputPort()) #non max suppression global nonmaxSuppr nonmaxSuppr = vtk.vtkImageNonMaximumSuppression() nonmaxSuppr.SetDimensionality(2) #padding global padImage padImage = vtk.vtkImageConstantPad() padImage.SetInputConnection(gradientImage.GetOutputPort()) padImage.SetOutputNumberOfScalarComponents(3) padImage.SetConstant(0) #to structured points global i2sp1 i2sp1 = vtk.vtkImageToStructuredPoints() i2sp1.SetInputConnection(nonmaxSuppr.GetOutputPort()) #link edges global linkImage linkImage = vtk.vtkLinkEdgels() linkImage.SetInputConnection(i2sp1.GetOutputPort()) linkImage.SetGradientThreshold(2) #thresholds links global thresholdEdgels thresholdEdgels = vtk.vtkThreshold() thresholdEdgels.SetInputConnection(linkImage.GetOutputPort()) thresholdEdgels.ThresholdByUpper(10) thresholdEdgels.AllScalarsOff() #filter global gf gf = vtk.vtkGeometryFilter() gf.SetInputConnection(thresholdEdgels.GetOutputPort()) #to structured points global i2sp i2sp = vtk.vtkImageToStructuredPoints() i2sp.SetInputConnection(magnitudeImage.GetOutputPort()) #subpixel global spe spe = vtk.vtkSubPixelPositionEdgels() spe.SetInputConnection(gf.GetOutputPort()) #stripper global strip strip = vtk.vtkStripper() strip.SetInputConnection(spe.GetOutputPort()) global dsm dsm = vtk.vtkPolyDataMapper() dsm.SetInputConnection(strip.GetOutputPort()) dsm.ScalarVisibilityOff() global planeActor planeActor = vtk.vtkActor() planeActor.SetMapper(dsm) planeActor.GetProperty().SetAmbient(1.0) planeActor.GetProperty().SetDiffuse(0.0) global edgeRender edgeRender = vtk.vtkRenderer() edgeRender.AddActor(planeActor) global renderWindow renderWindow = vtk.vtkRenderWindow() renderWindow.AddRenderer(edgeRender) global finalImage finalImage = vtk.vtkRenderLargeImage() finalImage.SetMagnification(magnificationFactor) finalImage.SetInput(edgeRender) global revImage revImage = vtk.vtkImageThreshold() revImage.SetInputConnection(finalImage.GetOutputPort()) revImage.ThresholdByUpper(127) revImage.ReplaceInOn() revImage.ReplaceOutOn() revImage.SetOutValue(255) revImage.SetInValue(0) #write image global imgWriter imgWriter = vtk.vtkPNGWriter() imgWriter.SetInputConnection(revImage.GetOutputPort()) imgWriter.SetFileName("test.png")