def __init__(self, module_manager): SimpleVTKClassModuleBase.__init__( self, module_manager, vtk.vtkImageMedian3D(), 'Processing.', ('vtkImageData',), ('vtkImageData',), replaceDoc=True, inputFunctions=None, outputFunctions=None)
def applyMedianFilterVTK(self, imgData, kern=[3, 3, 3]): med = vtk.vtkImageMedian3D() med.SetInputData(imgData.GetOutput()) med.SetKernelSize(kern[0], kern[1], kern[2]) med.Update() return med
def __init__(self): """ Initialization """ MorphologicalFilter.__init__(self) self.vtkfilter = vtk.vtkImageMedian3D() self.vtkfilter.AddObserver('ProgressEvent', lib.messenger.send) lib.messenger.connect(self.vtkfilter, "ProgressEvent", self.updateProgress) self.filterDesc = "Replaces each pixel/voxel with median value inside kernel\nInput: Grayscale/Binary image\nOutput: Grayscale/Binary image"
def __init__(self, module_manager): SimpleVTKClassModuleBase.__init__(self, module_manager, vtk.vtkImageMedian3D(), 'Processing.', ('vtkImageData', ), ('vtkImageData', ), replaceDoc=True, inputFunctions=None, outputFunctions=None)
def __init__(self): """ Initialization """ MorphologicalFilter.__init__(self) self.vtkfilter = vtk.vtkImageMedian3D() self.vtkfilter.AddObserver('ProgressEvent', lib.messenger.send) lib.messenger.connect(self.vtkfilter, "ProgressEvent", self.updateProgress) self.filterDesc = "Replaces each pixel/voxel with median value inside kernel\nInput: Grayscale/Binary image\nOutput: Grayscale/Binary image"
def medianSmooth(self, neighbours=(2, 2, 2)): """Median filter that replaces each pixel with the median value from a rectangular neighborhood around that pixel. """ imgm = vtk.vtkImageMedian3D() imgm.SetInputData(self.imagedata()) if utils.isSequence(neighbours): imgm.SetKernelSize(neighbours[0], neighbours[1], neighbours[2]) else: imgm.SetKernelSize(neighbours, neighbours, neighbours) imgm.Update() return self._update(imgm.GetOutput())
def ipl_median_filter(image_in, support): ipl_median_filter_settings(support) extent = image_in.GetExtent() voi = vtk.vtkExtractVOI() voi.SetInput(image_in) voi.SetVOI(extent[0] + support, extent[1] - support, extent[2] + support, extent[3] - support, extent[4] + support, extent[5] - support) voi.Update() median = vtk.vtkImageMedian3D() median.SetKernelSize(support, support, support) median.SetInputConnection(voi.GetOutputPort()) median.Update() image_out = median.GetOutput() return image_out
def __init__(self, module_manager): # initialise our base class ModuleBase.__init__(self, module_manager) self._config.kernelSize = (3, 3, 3) configList = [ ('Kernel size:', 'kernelSize', 'tuple:int,3', 'text', 'Size of structuring element in pixels.')] self._imageMedian3D = vtk.vtkImageMedian3D() ScriptedConfigModuleMixin.__init__( self, configList, {'Module (self)' : self, 'vtkImageMedian3D' : self._imageMedian3D}) module_utils.setup_vtk_object_progress(self, self._imageMedian3D, 'Filtering with median') self.sync_module_logic_with_config()
def MedianFilter(self, image, kernel_size=3, image3d=True): """Returns a median-filtered version of image""" image.SetReleaseDataFlag(1) ks = int(kernel_size) ksx, ksy, ksz = ks, ks, ks dims = image.GetDimensions() if dims[2] == 1 or image3d == False: ksz = 1 _filter = vtk.vtkImageMedian3D() _filter.SetKernelSize(ksx, ksy, ksz) _filter.SetInput(image.GetRealImage()) _filter.SetProgressText("Median filtering image...") _filter.AddObserver('ProgressEvent', self.HandleVTKProgressEvent) logging.info( "Median filter applied with radius={0}".format(kernel_size)) _filter.Update() return MVImage.MVImage(_filter.GetOutputPort(), input=image)
def MedianFilter(self, image, kernel_size=3, image3d=True): """Returns a median-filtered version of image""" image.SetReleaseDataFlag(1) ks = int(kernel_size) ksx, ksy, ksz = ks, ks, ks dims = image.GetDimensions() if dims[2] == 1 or image3d == False: ksz = 1 _filter = vtk.vtkImageMedian3D() _filter.SetKernelSize(ksx, ksy, ksz) _filter.SetInput(image.GetRealImage()) _filter.SetProgressText("Median filtering image...") _filter.AddObserver('ProgressEvent', self.HandleVTKProgressEvent) logging.info( "Median filter applied with radius={0}".format(kernel_size)) _filter.Update() return MVImage.MVImage(_filter.GetOutputPort(), input=image)
def operation(self, operation, volume2=None): """ Perform operations with ``Volume`` objects. `volume2` can be a constant value. Possible operations are: ``+``, ``-``, ``/``, ``1/x``, ``sin``, ``cos``, ``exp``, ``log``, ``abs``, ``**2``, ``sqrt``, ``min``, ``max``, ``atan``, ``atan2``, ``median``, ``mag``, ``dot``, ``gradient``, ``divergence``, ``laplacian``. |volumeOperations| |volumeOperations.py|_ """ op = operation.lower() image1 = self._data if op in ["median"]: mf = vtk.vtkImageMedian3D() mf.SetInputData(image1) mf.Update() return Volume(mf.GetOutput()) elif op in ["mag"]: mf = vtk.vtkImageMagnitude() mf.SetInputData(image1) mf.Update() return Volume(mf.GetOutput()) elif op in ["dot", "dotproduct"]: mf = vtk.vtkImageDotProduct() mf.SetInput1Data(image1) mf.SetInput2Data(volume2._data) mf.Update() return Volume(mf.GetOutput()) elif op in ["grad", "gradient"]: mf = vtk.vtkImageGradient() mf.SetDimensionality(3) mf.SetInputData(image1) mf.Update() return Volume(mf.GetOutput()) elif op in ["div", "divergence"]: mf = vtk.vtkImageDivergence() mf.SetInputData(image1) mf.Update() return Volume(mf.GetOutput()) elif op in ["laplacian"]: mf = vtk.vtkImageLaplacian() mf.SetDimensionality(3) mf.SetInputData(image1) mf.Update() return Volume(mf.GetOutput()) mat = vtk.vtkImageMathematics() mat.SetInput1Data(image1) K = None if isinstance(volume2, (int, float)): K = volume2 mat.SetConstantK(K) mat.SetConstantC(K) elif volume2 is not None: # assume image2 is a constant value mat.SetInput2Data(volume2._data) if op in ["+", "add", "plus"]: if K: mat.SetOperationToAddConstant() else: mat.SetOperationToAdd() elif op in ["-", "subtract", "minus"]: if K: mat.SetConstantC(-K) mat.SetOperationToAddConstant() else: mat.SetOperationToSubtract() elif op in ["*", "multiply", "times"]: if K: mat.SetOperationToMultiplyByK() else: mat.SetOperationToMultiply() elif op in ["/", "divide"]: if K: mat.SetConstantK(1.0 / K) mat.SetOperationToMultiplyByK() else: mat.SetOperationToDivide() elif op in ["1/x", "invert"]: mat.SetOperationToInvert() elif op in ["sin"]: mat.SetOperationToSin() elif op in ["cos"]: mat.SetOperationToCos() elif op in ["exp"]: mat.SetOperationToExp() elif op in ["log"]: mat.SetOperationToLog() elif op in ["abs"]: mat.SetOperationToAbsoluteValue() elif op in ["**2", "square"]: mat.SetOperationToSquare() elif op in ["sqrt", "sqr"]: mat.SetOperationToSquareRoot() elif op in ["min"]: mat.SetOperationToMin() elif op in ["max"]: mat.SetOperationToMax() elif op in ["atan"]: mat.SetOperationToATAN() elif op in ["atan2"]: mat.SetOperationToATAN2() else: colors.printc("\times Error in volumeOperation: unknown operation", operation, c='r') raise RuntimeError() mat.Update() return self._update(mat.GetOutput())
def smoothSelectedSegment(self): try: # Get master volume image data import vtkSegmentationCorePython # Get modifier labelmap modifierLabelmap = self.scriptedEffect.defaultModifierLabelmap() selectedSegmentLabelmap = self.scriptedEffect.selectedSegmentLabelmap() smoothingMethod = self.scriptedEffect.parameter("SmoothingMethod") if smoothingMethod == GAUSSIAN: maxValue = 255 thresh = vtk.vtkImageThreshold() thresh.SetInputData(selectedSegmentLabelmap) thresh.ThresholdByLower(0) thresh.SetInValue(0) thresh.SetOutValue(maxValue) thresh.SetOutputScalarType(vtk.VTK_UNSIGNED_CHAR) standardDeviationMm = self.scriptedEffect.doubleParameter("GaussianStandardDeviationMm") gaussianFilter = vtk.vtkImageGaussianSmooth() gaussianFilter.SetInputConnection(thresh.GetOutputPort()) gaussianFilter.SetStandardDeviation(standardDeviationMm) gaussianFilter.SetRadiusFactor(4) thresh2 = vtk.vtkImageThreshold() thresh2.SetInputConnection(gaussianFilter.GetOutputPort()) thresh2.ThresholdByUpper(int(maxValue / 2)) thresh2.SetInValue(1) thresh2.SetOutValue(0) thresh2.SetOutputScalarType(selectedSegmentLabelmap.GetScalarType()) thresh2.Update() modifierLabelmap.DeepCopy(thresh2.GetOutput()) else: # size rounded to nearest odd number. If kernel size is even then image gets shifted. kernelSizePixel = self.getKernelSizePixel() if smoothingMethod == MEDIAN: # Median filter does not require a particular label value smoothingFilter = vtk.vtkImageMedian3D() smoothingFilter.SetInputData(selectedSegmentLabelmap) else: # We need to know exactly the value of the segment voxels, apply threshold to make force the selected label value labelValue = 1 backgroundValue = 0 thresh = vtk.vtkImageThreshold() thresh.SetInputData(selectedSegmentLabelmap) thresh.ThresholdByLower(0) thresh.SetInValue(backgroundValue) thresh.SetOutValue(labelValue) thresh.SetOutputScalarType(selectedSegmentLabelmap.GetScalarType()) smoothingFilter = vtk.vtkImageOpenClose3D() smoothingFilter.SetInputConnection(thresh.GetOutputPort()) if smoothingMethod == MORPHOLOGICAL_OPENING: smoothingFilter.SetOpenValue(labelValue) smoothingFilter.SetCloseValue(backgroundValue) else: # must be smoothingMethod == MORPHOLOGICAL_CLOSING: smoothingFilter.SetOpenValue(backgroundValue) smoothingFilter.SetCloseValue(labelValue) smoothingFilter.SetKernelSize(kernelSizePixel[0],kernelSizePixel[1],kernelSizePixel[2]) smoothingFilter.Update() modifierLabelmap.DeepCopy(smoothingFilter.GetOutput()) except IndexError: logging.error('apply: Failed to apply smoothing') # Apply changes self.scriptedEffect.modifySelectedSegmentByLabelmap(modifierLabelmap, slicer.qSlicerSegmentEditorAbstractEffect.ModificationModeSet)
def autocontour_buie(img, args): # these hard-coded constants just make sure that the connectivity filter # actually works, they don't need to be configuable in my opinion CONN_SCALAR_RANGE = [1, args.in_value + 1] CONN_SIZE_RANGE = [0, int(1E10)] # Step 1 was just loading the AIM # Step 2: Threshold s2_threshold = vtk.vtkImageThreshold() s2_threshold.ThresholdByUpper(args.threshold_1) s2_threshold.SetInValue(args.in_value) s2_threshold.SetOutValue(args.out_value) s2_threshold.SetInputData(img) # Step 3: Median s3_median = vtk.vtkImageMedian3D() s3_median.SetKernelSize(*args.step_3_median_kernel) s3_median.SetInputConnection(s2_threshold.GetOutputPort()) # Step 4: Dilate s4_dilate = vtk.vtkImageDilateErode3D() s4_dilate.SetDilateValue(args.in_value) s4_dilate.SetErodeValue(args.out_value) s4_dilate.SetKernelSize(*args.step_4_and_6_dilate_erode_kernel) s4_dilate.SetInputConnection(s3_median.GetOutputPort()) # Step 5: Connectivity (applied to non-bone) # first flip 0 <-> 127 s5a_invert = vtk.vtkImageThreshold() s5a_invert.ThresholdByLower(args.in_value / 2) s5a_invert.SetInValue(args.in_value) s5a_invert.SetOutValue(args.out_value) s5a_invert.SetInputConnection(s4_dilate.GetOutputPort()) # then connectivity s5b_connectivity = vtk.vtkImageConnectivityFilter() s5b_connectivity.SetExtractionModeToLargestRegion() s5b_connectivity.SetScalarRange(*CONN_SCALAR_RANGE) s5b_connectivity.SetSizeRange(*CONN_SIZE_RANGE) s5b_connectivity.SetInputConnection(s5a_invert.GetOutputPort()) # then flip 0 <-> 127 again s5c_invert = vtk.vtkImageThreshold() s5c_invert.ThresholdByLower(0.5) s5c_invert.SetInValue(args.in_value) s5c_invert.SetOutValue(args.out_value) s5c_invert.SetInputConnection(s5b_connectivity.GetOutputPort()) # Step 6: Erode s6_erode = vtk.vtkImageDilateErode3D() s6_erode.SetDilateValue(args.out_value) s6_erode.SetErodeValue(args.in_value) s6_erode.SetKernelSize(*args.step_4_and_6_dilate_erode_kernel) s6_erode.SetInputConnection(s5c_invert.GetOutputPort()) # Step 7: Threshold s7_threshold = vtk.vtkImageThreshold() s7_threshold.ThresholdByLower(args.threshold_2) s7_threshold.SetInValue(args.in_value) s7_threshold.SetOutValue(args.out_value) s7_threshold.SetInputData(img) # Step 8: Mask s8a_mask = vtk.vtkImageMask() s8a_mask.SetInputConnection(0, s7_threshold.GetOutputPort()) s8a_mask.SetInputConnection(1, s6_erode.GetOutputPort()) s8b_invert = vtk.vtkImageThreshold() s8b_invert.ThresholdByLower(args.in_value / 2) s8b_invert.SetInValue(args.in_value) s8b_invert.SetOutValue(args.out_value) s8b_invert.SetInputConnection(s8a_mask.GetOutputPort()) # Step 9: Dilate s9_dilate = vtk.vtkImageDilateErode3D() s9_dilate.SetDilateValue(args.out_value) s9_dilate.SetErodeValue(args.in_value) s9_dilate.SetKernelSize(*args.step_9_and_11_dilate_erode_kernel) s9_dilate.SetInputConnection(s8b_invert.GetOutputPort()) # Step 10: Connectivity # connectivity s10a_connectivity = vtk.vtkImageConnectivityFilter() s10a_connectivity.SetExtractionModeToLargestRegion() s10a_connectivity.SetScalarRange(*CONN_SCALAR_RANGE) s10a_connectivity.SetSizeRange(*CONN_SIZE_RANGE) s10a_connectivity.SetInputConnection(s9_dilate.GetOutputPort()) # then convert to 127 and 0 again s10b_convert = vtk.vtkImageThreshold() s10b_convert.ThresholdByLower(0.5) s10b_convert.SetInValue(args.out_value) s10b_convert.SetOutValue(args.in_value) s10b_convert.SetInputConnection(s10a_connectivity.GetOutputPort()) # Step 11: Erode s11_erode = vtk.vtkImageDilateErode3D() s11_erode.SetDilateValue(args.in_value) s11_erode.SetErodeValue(args.out_value) s11_erode.SetKernelSize(*args.step_9_and_11_dilate_erode_kernel) s11_erode.SetInputConnection(s10b_convert.GetOutputPort()) # Step 12: Gaussian Smooth s12_gauss = vtk.vtkImageGaussianSmooth() s12_gauss.SetStandardDeviation(args.step_12_gaussian_std) s12_gauss.SetRadiusFactors(*args.step_12_gaussian_kernel) s12_gauss.SetInputConnection(s11_erode.GetOutputPort()) # Step 13: Threshold s13_threshold = vtk.vtkImageThreshold() s13_threshold.ThresholdByLower(S13_THRESH) s13_threshold.SetInValue(args.out_value) s13_threshold.SetOutValue(args.in_value) s13_threshold.SetInputConnection(s12_gauss.GetOutputPort()) # Step 14: Mask s14_mask = vtk.vtkImageMask() s14_mask.SetInputConnection(0, s6_erode.GetOutputPort()) s14_mask.SetInputConnection(1, s13_threshold.GetOutputPort()) # Step 15: Invert the Trabecular Mask s15_invert = vtk.vtkImageThreshold() s15_invert.ThresholdByLower(args.in_value / 2) s15_invert.SetInValue(args.in_value) s15_invert.SetOutValue(args.out_value) s15_invert.SetInputConnection(s13_threshold.GetOutputPort()) # update the pipeline s14_mask.Update() s15_invert.Update() # get masks cort_mask = s14_mask.GetOutput() trab_mask = s15_invert.GetOutput() return cort_mask, trab_mask
def main(): # colors = vtk.vtkNamedColors() fileName = get_program_parameters() # Read the image. readerFactory = vtk.vtkImageReader2Factory() reader = readerFactory.CreateImageReader2(fileName) reader.SetFileName(fileName) reader.Update() scalarRange = [0] * 2 scalarRange[0] = reader.GetOutput().GetPointData().GetScalars().GetRange( )[0] scalarRange[1] = reader.GetOutput().GetPointData().GetScalars().GetRange( )[1] print("Range:", scalarRange) middleSlice = (reader.GetOutput().GetExtent()[5] - reader.GetOutput().GetExtent()[4]) // 2 # Work with double images. cast = vtk.vtkImageCast() cast.SetInputConnection(reader.GetOutputPort()) cast.SetOutputScalarTypeToDouble() cast.Update() originalData = vtk.vtkImageData() originalData.DeepCopy(cast.GetOutput()) noisyData = vtk.vtkImageData() AddShotNoise(originalData, noisyData, 2000.0, 0.1, reader.GetOutput().GetExtent()) median = vtk.vtkImageMedian3D() median.SetInputData(noisyData) median.SetKernelSize(5, 5, 1) hybridMedian1 = vtk.vtkImageHybridMedian2D() hybridMedian1.SetInputData(noisyData) hybridMedian = vtk.vtkImageHybridMedian2D() hybridMedian.SetInputConnection(hybridMedian1.GetOutputPort()) colorWindow = (scalarRange[1] - scalarRange[0]) * 0.8 colorLevel = colorWindow / 2 originalActor = vtk.vtkImageActor() originalActor.GetMapper().SetInputData(originalData) originalActor.GetProperty().SetColorWindow(colorWindow) originalActor.GetProperty().SetColorLevel(colorLevel) originalActor.GetProperty().SetInterpolationTypeToNearest() originalActor.SetDisplayExtent(reader.GetDataExtent()[0], reader.GetDataExtent()[1], reader.GetDataExtent()[2], reader.GetDataExtent()[3], middleSlice, middleSlice) noisyActor = vtk.vtkImageActor() noisyActor.GetMapper().SetInputData(noisyData) noisyActor.GetProperty().SetColorWindow(colorWindow) noisyActor.GetProperty().SetColorLevel(colorLevel) noisyActor.GetProperty().SetInterpolationTypeToNearest() noisyActor.SetDisplayExtent(originalActor.GetDisplayExtent()) hybridMedianActor = vtk.vtkImageActor() hybridMedianActor.GetMapper().SetInputConnection( hybridMedian.GetOutputPort()) hybridMedianActor.GetProperty().SetColorWindow(colorWindow) hybridMedianActor.GetProperty().SetColorLevel(colorLevel) hybridMedianActor.GetProperty().SetInterpolationTypeToNearest() hybridMedianActor.SetDisplayExtent(originalActor.GetDisplayExtent()) medianActor = vtk.vtkImageActor() medianActor.GetMapper().SetInputConnection(median.GetOutputPort()) medianActor.GetProperty().SetColorWindow(colorWindow) medianActor.GetProperty().SetColorLevel(colorLevel) medianActor.GetProperty().SetInterpolationTypeToNearest() # Setup the renderers. originalRenderer = vtk.vtkRenderer() originalRenderer.AddActor(originalActor) noisyRenderer = vtk.vtkRenderer() noisyRenderer.AddActor(noisyActor) hybridRenderer = vtk.vtkRenderer() hybridRenderer.AddActor(hybridMedianActor) medianRenderer = vtk.vtkRenderer() medianRenderer.AddActor(medianActor) renderers = list() renderers.append(originalRenderer) renderers.append(noisyRenderer) renderers.append(hybridRenderer) renderers.append(medianRenderer) # Setup viewports for the renderers. rendererSize = 400 xGridDimensions = 2 yGridDimensions = 2 renderWindow = vtk.vtkRenderWindow() renderWindow.SetSize(rendererSize * xGridDimensions, rendererSize * yGridDimensions) for row in range(0, yGridDimensions): for col in range(xGridDimensions): index = row * xGridDimensions + col # (xmin, ymin, xmax, ymax) viewport = [ float(col) / xGridDimensions, float(yGridDimensions - (row + 1)) / yGridDimensions, float(col + 1) / xGridDimensions, float(yGridDimensions - row) / yGridDimensions ] renderers[index].SetViewport(viewport) renderWindow.AddRenderer(renderers[index]) renderWindowInteractor = vtk.vtkRenderWindowInteractor() style = vtk.vtkInteractorStyleImage() renderWindowInteractor.SetInteractorStyle(style) renderWindowInteractor.SetRenderWindow(renderWindow) # The renderers share one camera. renderWindow.Render() for r in range(1, len(renderers)): renderers[r].SetActiveCamera(renderers[0].GetActiveCamera()) renderWindowInteractor.Initialize() renderWindowInteractor.Start()
def imageOperation(image1, operation='+', image2=None): ''' Perform operations with vtkImageData objects. Image2 can contain a constant value. Possible operations are: +, -, /, 1/x, sin, cos, exp, log, abs, **2, sqrt, min, max, atan, atan2, median, mag, dot, gradient, divergence, laplacian. [**Example**](https://github.com/marcomusy/vtkplotter/blob/master/examples/volumetric/imageOperations.py) 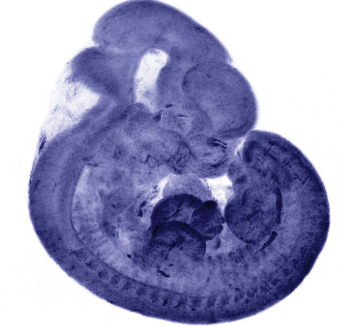 ''' op = operation.lower() if op in ['median']: mf = vtk.vtkImageMedian3D() mf.SetInputData(image1) mf.Update() return mf.GetOutput() elif op in ['mag']: mf = vtk.vtkImageMagnitude() mf.SetInputData(image1) mf.Update() return mf.GetOutput() elif op in ['dot', 'dotproduct']: mf = vtk.vtkImageDotProduct() mf.SetInput1Data(image1) mf.SetInput2Data(image2) mf.Update() return mf.GetOutput() elif op in ['grad', 'gradient']: mf = vtk.vtkImageGradient() mf.SetDimensionality(3) mf.SetInputData(image1) mf.Update() return mf.GetOutput() elif op in ['div', 'divergence']: mf = vtk.vtkImageDivergence() mf.SetInputData(image1) mf.Update() return mf.GetOutput() elif op in ['laplacian']: mf = vtk.vtkImageLaplacian() mf.SetDimensionality(3) mf.SetInputData(image1) mf.Update() return mf.GetOutput() mat = vtk.vtkImageMathematics() mat.SetInput1Data(image1) K = None if image2: if isinstance(image2, vtk.vtkImageData): mat.SetInput2Data(image2) else: # assume image2 is a constant value K = image2 mat.SetConstantK(K) mat.SetConstantC(K) if op in ['+', 'add', 'plus']: if K: mat.SetOperationToAddConstant() else: mat.SetOperationToAdd() elif op in ['-', 'subtract', 'minus']: if K: mat.SetConstantC(-K) mat.SetOperationToAddConstant() else: mat.SetOperationToSubtract() elif op in ['*', 'multiply', 'times']: if K: mat.SetOperationToMultiplyByK() else: mat.SetOperationToMultiply() elif op in ['/', 'divide']: if K: mat.SetConstantK(1.0/K) mat.SetOperationToMultiplyByK() else: mat.SetOperationToDivide() elif op in ['1/x', 'invert']: mat.SetOperationToInvert() elif op in ['sin']: mat.SetOperationToSin() elif op in ['cos']: mat.SetOperationToCos() elif op in ['exp']: mat.SetOperationToExp() elif op in ['log']: mat.SetOperationToLog() elif op in ['abs']: mat.SetOperationToAbsoluteValue() elif op in ['**2', 'square']: mat.SetOperationToSquare() elif op in ['sqrt', 'sqr']: mat.SetOperationToSquareRoot() elif op in ['min']: mat.SetOperationToMin() elif op in ['max']: mat.SetOperationToMax() elif op in ['atan']: mat.SetOperationToATAN() elif op in ['atan2']: mat.SetOperationToATAN2() else: vc.printc('Error in imageOperation: unknown operation', operation, c=1) exit() mat.Update() return mat.GetOutput()
HipoRight = vtk.vtkNIFTIImageReader() HipoRight.SetFileName("head_bet_Right_Hipocampus_Resample.nii") HipoRight.Update() #Load original image Head = vtk.vtkNIFTIImageReader() Head.SetFileName("head.nii") Head.Update() #Load skull stripped mask Brain = vtk.vtkNIFTIImageReader() Brain.SetFileName("head_bet.nii") Brain.Update() BrainMask = vtk.vtkImageData() BrainMask.DeepCopy(Brain.GetOutput()) #Smooth the original image smooth = vtk.vtkImageMedian3D() smooth.SetInputConnection(Head.GetOutputPort()) smooth.SetKernelSize(3, 3, 3) smooth.Update() Salt = smooth.GetOutput() HeadMask = vtk.vtkImageData() HeadMask.DeepCopy(Salt) #................................................................................ '''Segment the whole head in head.nii image and call it HeadMask then emty the headmask by using skullstripped brain and then empty skullstripped brain regions that belong to both hippocampi''' for z in range(0, Salt.GetDimensions()[2]): for y in range(0, Salt.GetDimensions()[1]): for x in range(0, Salt.GetDimensions()[0]): voxelValueSalt = Salt.GetScalarComponentAsFloat(x, y, z, 0) if 70 < voxelValueSalt < 1000:
def smoothSelectedSegment(self): try: # Get master volume image data import vtkSegmentationCorePython # Get modifier labelmap modifierLabelmap = self.scriptedEffect.defaultModifierLabelmap() selectedSegmentLabelmap = self.scriptedEffect.selectedSegmentLabelmap( ) smoothingMethod = self.scriptedEffect.parameter("SmoothingMethod") if smoothingMethod == GAUSSIAN: maxValue = 255 thresh = vtk.vtkImageThreshold() thresh.SetInputData(selectedSegmentLabelmap) thresh.ThresholdByLower(0) thresh.SetInValue(0) thresh.SetOutValue(maxValue) thresh.SetOutputScalarType(vtk.VTK_UNSIGNED_CHAR) standardDeviationMm = self.scriptedEffect.doubleParameter( "GaussianStandardDeviationMm") gaussianFilter = vtk.vtkImageGaussianSmooth() gaussianFilter.SetInputConnection(thresh.GetOutputPort()) gaussianFilter.SetStandardDeviation(standardDeviationMm) gaussianFilter.SetRadiusFactor(4) thresh2 = vtk.vtkImageThreshold() thresh2.SetInputConnection(gaussianFilter.GetOutputPort()) thresh2.ThresholdByUpper(int(maxValue / 2)) thresh2.SetInValue(1) thresh2.SetOutValue(0) thresh2.SetOutputScalarType( selectedSegmentLabelmap.GetScalarType()) thresh2.Update() modifierLabelmap.DeepCopy(thresh2.GetOutput()) else: # size rounded to nearest odd number. If kernel size is even then image gets shifted. kernelSizePixel = self.getKernelSizePixel() if smoothingMethod == MEDIAN: # Median filter does not require a particular label value smoothingFilter = vtk.vtkImageMedian3D() smoothingFilter.SetInputData(selectedSegmentLabelmap) else: # We need to know exactly the value of the segment voxels, apply threshold to make force the selected label value labelValue = 1 backgroundValue = 0 thresh = vtk.vtkImageThreshold() thresh.SetInputData(selectedSegmentLabelmap) thresh.ThresholdByLower(0) thresh.SetInValue(backgroundValue) thresh.SetOutValue(labelValue) thresh.SetOutputScalarType( selectedSegmentLabelmap.GetScalarType()) smoothingFilter = vtk.vtkImageOpenClose3D() smoothingFilter.SetInputConnection(thresh.GetOutputPort()) if smoothingMethod == MORPHOLOGICAL_OPENING: smoothingFilter.SetOpenValue(labelValue) smoothingFilter.SetCloseValue(backgroundValue) else: # must be smoothingMethod == MORPHOLOGICAL_CLOSING: smoothingFilter.SetOpenValue(backgroundValue) smoothingFilter.SetCloseValue(labelValue) smoothingFilter.SetKernelSize(kernelSizePixel[0], kernelSizePixel[1], kernelSizePixel[2]) smoothingFilter.Update() modifierLabelmap.DeepCopy(smoothingFilter.GetOutput()) except IndexError: logging.error('apply: Failed to apply smoothing') # Apply changes self.scriptedEffect.modifySelectedSegmentByLabelmap( modifierLabelmap, slicer.qSlicerSegmentEditorAbstractEffect.ModificationModeSet)
shotNoiseThresh2.SetInValue(-shotNoiseAmplitude) shotNoiseThresh2.SetOutValue(0.0) shotNoiseThresh2.Update() shotNoise = vtk.vtkImageMathematics() shotNoise.SetInput1Data(shotNoiseThresh1.GetOutput()) shotNoise.SetInput2Data(shotNoiseThresh2.GetOutput()) shotNoise.SetOperationToAdd() shotNoise.Update() add = vtk.vtkImageMathematics() add.SetInput1Data(shotNoise.GetOutput()) add.SetInput2Data(imageCanvas.GetOutput()) add.SetOperationToAdd() median = vtk.vtkImageMedian3D() median.SetInputConnection(add.GetOutputPort()) median.SetKernelSize(3, 3, 1) hybrid1 = vtk.vtkImageHybridMedian2D() hybrid1.SetInputConnection(add.GetOutputPort()) hybrid2 = vtk.vtkImageHybridMedian2D() hybrid2.SetInputConnection(hybrid1.GetOutputPort()) viewer = vtk.vtkImageViewer() viewer.SetInputConnection(hybrid1.GetOutputPort()) viewer.SetColorWindow(256) viewer.SetColorLevel(127.5) viewer.GetRenderWindow().SetSize(256, 256) viewer.Render()
def smoothSelectedSegment(self, maskImage=None, maskExtent=None): try: # Get modifier labelmap modifierLabelmap = self.scriptedEffect.defaultModifierLabelmap() selectedSegmentLabelmap = self.scriptedEffect.selectedSegmentLabelmap( ) smoothingMethod = self.scriptedEffect.parameter("SmoothingMethod") if smoothingMethod == GAUSSIAN: maxValue = 255 radiusFactor = 4.0 standardDeviationMM = self.scriptedEffect.doubleParameter( "GaussianStandardDeviationMm") spacing = modifierLabelmap.GetSpacing() standardDeviationPixel = [1.0, 1.0, 1.0] radiusPixel = [3, 3, 3] for idx in range(3): standardDeviationPixel[ idx] = standardDeviationMM / spacing[idx] radiusPixel[idx] = int( standardDeviationPixel[idx] * radiusFactor) + 1 if maskExtent: clippedSelectedSegmentLabelmap = self.clipImage( selectedSegmentLabelmap, maskExtent, radiusPixel) else: clippedSelectedSegmentLabelmap = selectedSegmentLabelmap thresh = vtk.vtkImageThreshold() thresh.SetInputData(clippedSelectedSegmentLabelmap) thresh.ThresholdByLower(0) thresh.SetInValue(0) thresh.SetOutValue(maxValue) thresh.SetOutputScalarType(vtk.VTK_UNSIGNED_CHAR) gaussianFilter = vtk.vtkImageGaussianSmooth() gaussianFilter.SetInputConnection(thresh.GetOutputPort()) gaussianFilter.SetStandardDeviation(*standardDeviationPixel) gaussianFilter.SetRadiusFactor(radiusFactor) thresh2 = vtk.vtkImageThreshold() thresh2.SetInputConnection(gaussianFilter.GetOutputPort()) thresh2.ThresholdByUpper(int(maxValue / 2)) thresh2.SetInValue(1) thresh2.SetOutValue(0) thresh2.SetOutputScalarType( selectedSegmentLabelmap.GetScalarType()) thresh2.Update() self.modifySelectedSegmentByLabelmap(thresh2.GetOutput(), selectedSegmentLabelmap, modifierLabelmap, maskImage, maskExtent) else: # size rounded to nearest odd number. If kernel size is even then image gets shifted. kernelSizePixel = self.getKernelSizePixel() if maskExtent: clippedSelectedSegmentLabelmap = self.clipImage( selectedSegmentLabelmap, maskExtent, kernelSizePixel) else: clippedSelectedSegmentLabelmap = selectedSegmentLabelmap if smoothingMethod == MEDIAN: # Median filter does not require a particular label value smoothingFilter = vtk.vtkImageMedian3D() smoothingFilter.SetInputData( clippedSelectedSegmentLabelmap) else: # We need to know exactly the value of the segment voxels, apply threshold to make force the selected label value labelValue = 1 backgroundValue = 0 thresh = vtk.vtkImageThreshold() thresh.SetInputData(clippedSelectedSegmentLabelmap) thresh.ThresholdByLower(0) thresh.SetInValue(backgroundValue) thresh.SetOutValue(labelValue) thresh.SetOutputScalarType( clippedSelectedSegmentLabelmap.GetScalarType()) smoothingFilter = vtk.vtkImageOpenClose3D() smoothingFilter.SetInputConnection(thresh.GetOutputPort()) if smoothingMethod == MORPHOLOGICAL_OPENING: smoothingFilter.SetOpenValue(labelValue) smoothingFilter.SetCloseValue(backgroundValue) else: # must be smoothingMethod == MORPHOLOGICAL_CLOSING: smoothingFilter.SetOpenValue(backgroundValue) smoothingFilter.SetCloseValue(labelValue) smoothingFilter.SetKernelSize(kernelSizePixel[0], kernelSizePixel[1], kernelSizePixel[2]) smoothingFilter.Update() self.modifySelectedSegmentByLabelmap( smoothingFilter.GetOutput(), selectedSegmentLabelmap, modifierLabelmap, maskImage, maskExtent) except IndexError: logging.error('apply: Failed to apply smoothing')
shotNoiseThresh2.SetInValue(-shotNoiseAmplitude) shotNoiseThresh2.SetOutValue(0.0) shotNoiseThresh2.Update() shotNoise = vtk.vtkImageMathematics() shotNoise.SetInput1Data(shotNoiseThresh1.GetOutput()) shotNoise.SetInput2Data(shotNoiseThresh2.GetOutput()) shotNoise.SetOperationToAdd() shotNoise.Update() add = vtk.vtkImageMathematics() add.SetInput1Data(shotNoise.GetOutput()) add.SetInput2Data(imageCanvas.GetOutput()) add.SetOperationToAdd() median = vtk.vtkImageMedian3D() median.SetInputConnection(add.GetOutputPort()) median.SetKernelSize(3, 3, 1) hybrid1 = vtk.vtkImageHybridMedian2D() hybrid1.SetInputConnection(add.GetOutputPort()) hybrid2 = vtk.vtkImageHybridMedian2D() hybrid2.SetInputConnection(hybrid1.GetOutputPort()) viewer = vtk.vtkImageViewer() viewer.SetInputConnection(hybrid1.GetOutputPort()) viewer.SetColorWindow(256) viewer.SetColorLevel(127.5) viewer.GetRenderWindow().SetSize(256, 256) viewer.Render()