def post_whaleshare(whaleshares_key, whaleshares_username, tags, title, body, permlink=None): wls = Steem(node=[ "https://wls.kennybll.com", "https://rpc.whaleshares.io", "ws://188.166.99.136:8090" ], keys=[whaleshares_key]) wls_res = wls.post(title=title, body=body, author=whaleshares_username, tags=tags, permlink=permlink, json_metadata={ 'extensions': [[ 0, { 'beneficiaries': [ { 'account': 'fiasteemproject', 'weight': 2500 }, ] } ]] }) return wls_res
def post_smoke(smoke_key, smoke_username, tags, title, body, permlink=None): smk = Steem( node=['https://rpc.smoke.io/'], keys=[smoke_key], custom_chains={ "SMOKE": { "chain_id": "1ce08345e61cd3bf91673a47fc507e7ed01550dab841fd9cdb0ab66ef576aaf0", "min_version": "0.0.0", "prefix": "SMK", "chain_assets": [{ "asset": "STEEM", "symbol": "SMOKE", "precision": 3, "id": 1 }, { "asset": "VESTS", "symbol": "VESTS", "precision": 6, "id": 2 }] } }) smk_res = smk.post(title=title, body=body, author=smoke_username, tags=tags, permlink=permlink, json_metadata={ 'extensions': [[ 0, { 'beneficiaries': [ { 'account': 'fiasteem', 'weight': 2500 }, ] } ]] }) return smk_res
member_data[ops["author"]]["curation_rshares"] / rshares_denom * 100) reply_body += "* %.2f %% has come from new account bonus or extra value from pre-automation rewards\n" % ( member_data[ops["author"]]["other_rshares"] / rshares_denom * 100) if len(comment_footer) > 0: reply_body += "<br>\n" reply_body += comment_footer account_name = account_list[random.randint( 0, len(account_list) - 1)] try: stm.post("", reply_body, app="steembasicincome/%s" % sbiversion, author=account_name, reply_identifier=c.identifier) # c.reply(reply_body, author=account_name) time.sleep(4) except: continue already_voted = False #for v in c["active_votes"]: # if v["voter"] in accounts: # already_voted = True dt_created = c["created"] dt_created = dt_created.replace(tzinfo=None)
for u in users: user_delegations = Account(u).get_vesting_delegations() for d in user_delegations: if d['delegatee'] == 'hybridbot' and users[u].amount_delegated is 0: vests = float(d['vesting_shares'].split()[0]) users[u].update_amount_delegated(convert_VESTS_to_STEEM(vests)) # Create an HTML chart entry and fills in values for each user. for u in (sorted(users.values(), key=operator.attrgetter('amount_delegated'), reverse=True)): if u.username != 'hybridbot': chart = chart + '<tr><td><a href="/@{0}">@{0}</a></td><td>{1}SP</td><td>{2}</td><td>{3}</td><td>{4:.2f}%</td></tr>'.format( u.username, int(u.amount_delegated), u.steem_payout, u.sbd_payout, calculate_APR(u.steem_payout, u.sbd_payout, u.amount_delegated)) # Create post information. title = 'Hybridbot Testing - {0}'.format(datetime.today().strftime('%m/%d/%Y')) body = '<center>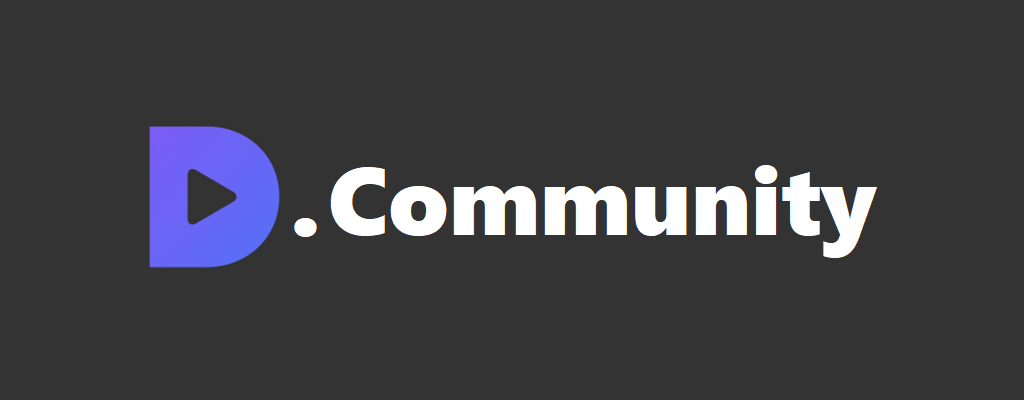</center' \ '>\n\nWe want to thank each of our delegators for their support of @hybridbot. Delegators receive 95% of ' \ 'all bids that are sent to @hybridbot in accordance with the amount of delegation they have contributed. ' \ 'The remaining 5% is sent to @prizeportal to help support content creators on @dlive.\n\nToday, we\'ve been' \ ' able to support @dlivecommunity with a payment of {0} STEEM while our delegators received ' \ 'the following payments:\n\n<table><thead><tr><th>Delegator</th><th>Amount ' \ 'Delegated</th><th>Steem Payout</th><th>SBD Payout</th><th>APR</th></tr></thead><tbody>{1}' \ '</table>\n\n'.format(str(calculate_hybridbot_payment()), chart) tags = ['promotion', 'test', 'bots', 'bidbot'] # Post the report so Patrick can get more delegators. :) s.post(title=title, body=body, author='hybridbot', tags=tags)
mdfile.write('<a href="https://discordapp.com/invite/fmE7Q9q">discord server.</a><hr>') for user in allusers: pstruct["users"].append(user) with open(join(mypath, "wtw-steem-meta-" + date + ".json"), "w") as jsonfile: jsonfile.write(json.dumps(pstruct)) transport = paramiko.Transport((fjson.conf["ssh-server"], 22)) transport.connect(username = fjson.conf["ssh-account"], password = fjson.conf["ssh-password"]) sftp = paramiko.SFTPClient.from_transport(transport) for grp in ["downvote","flag"]: for size in ["large", "small"]: formats = ["png"] if size == "large": formats = ["svg","pdf"] for fmt in formats: filename = "wtw-" + date + "-" + grp + "-" + size + "." + fmt sftp.put(join(mypath,filename),"WWW/wtw/" + filename) print "Uploaded", filename, "to",fjson.conf["ssh-server"] beneficiaries = [{'account':'freezepeach', 'weight':5000},{'account':'pibara', 'weight':5000}] metafile = join(mypath,"wtw-steem-meta-" + date + ".json") postfile = join(mypath,"wtw-" + date + ".MD") with open(metafile) as m: json_metadata = json.loads(m.read()) with open(postfile) as p: body = p.read() s = Steem(keys=fjson.conf["steem-posting-key"], nobroadcast=False) subject = "Flag-war stats for posts made on " + date tx = s.post(subject, body, author=fjson.conf["steem-account"], tags=["stats","steem","steemit","flags","flagwars"], json_metadata=json_metadata, beneficiaries=beneficiaries) print "DONE"
class RedFisher: def __init__(self): """Initialisation.""" self.load_settings() self.nodes = [ 'https://api.steemit.com', 'https://rpc.buildteam.io', 'https://api.steem.house', 'https://steemd.steemitdev.com', 'https://steemd.steemitstage.com', 'https://steemd.steemgigs.org' ] self.s = Steem(keys=self.posting_key) self.s.set_default_nodes(self.nodes) self.b = Blockchain(self.s) set_shared_steem_instance(self.s) self.p = Pool(4) def timer_start(self): """Set a timer to restart the program at 7pm the next day.""" # Calculate how many seconds until 7pm tomorrow now = datetime.today() print(now.day) a_time = now.replace(day=now.day + 1, hour=19, minute=0, microsecond=0) d_time = a_time - now secs = d_time.seconds + 1 # Reload settings from external file self.load_settings() # Start the timer t = threading.Timer(secs, self.redfisher) t.start() print('Timer set for ' + str(secs / 3600) + ' hours.') def load_settings(self): """Load the account requrements from an external file.""" # Load the external .json settings = json.loads(open('settings.json', 'r').read()) # Import the settings to variables self.days = settings['days'] self.posts_per_week = settings['posts_per_week'] self.min_sp = settings['min_sp'] self.max_sv = settings['max_sv'] self.posting_account = settings['posting_account'] self.posting_key = settings['posting_key'] print('settings loaded\ndays: %d\nposts per week: %d\nminimum steem ' 'power: %d' % (self.days, self.posts_per_week, self.min_sp)) def redfisher(self): """ Streams through all posts within given timeframe and sends accounts to be validated. """ t1 = time.process_time() # Calculate the start block based on how many days were specified # in the settings now = datetime.now() start_date = now - timedelta(days=self.days) start_block = self.b.get_estimated_block_num(start_date) # Get the end block end_block = self.b.get_current_block_num() # Create/reset approved user dictionary self.user_list = dict() # stream comments from start block to current block print('streaming comments now') # Create checked accounts list checked_accounts = list() # Infinite while allows for many node swaps while True: try: # Start/continue the stream from start/lastchecked block for post in self.b.stream(opNames=['comment'], start=start_block, stop=end_block): # Set start block to the one currently being checked start_block = post['block_num'] # Assure that the post is not a comment if post['parent_author'] == '': author = post['author'] # Don't check accounts that have already been checked if author not in checked_accounts: # Add account to checked accounts checked_accounts.append(author) # Add account to pool to be checked self.p.enqueue(func=self.check, user=author) self.p.run() # All checks completed, break loop break except Exception as e: # Switch nodes print(e) self.failover() time.sleep(1) t2 = time.process_time() print(t2 - t1) # Wait for task pool to empty while self.p.done() == False: time.sleep(2) # Attempt to post every 20 seconds until complete while True: try: self.post() break except: time.sleep(20) def get_account_age(self, acc): """Calculate how old an account is. Keyword arguments: acc -- the account to be checked -- type: Account """ # Declare account creation types types = [ 'create_claimed_account', 'account_create_with_delegation', 'account_create' ] # Look through history until account creation is found for i in acc.history(only_ops=types): # Get account creation timestamp creation_date_raw = i['timestamp'] break print(creation_date_raw) # Convert timestamp into datetime obj creation_date = datetime.strptime(creation_date_raw, '%Y-%m-%dT%H:%M:%S') # Calculate age in days now = datetime.now() acc_age = (now - creation_date).days return acc_age def post(self): """Make the post containing all the collected info for the round.""" # Get date in string format date = datetime.today().strftime('%Y-%m-%d') print(date) # Read the post body from external file post_body = open('post_body.txt', 'r').read() # Order the approved accounts by age sorted_ul = sorted(self.user_list.keys(), key=lambda y: (self.user_list[y]['age'])) # For each approved user, add them to the bottom of the post table for username in sorted_ul: data = self.user_list[username] sp = str(round(data['sp'], 3)) age = data['age'] svp = data['svp'] post_body += '\n@%s | %s | %s | %s' % (username, sp, age, svp) print(post_body) # Broadcast post transaction self.s.post(title='Small Accounts To Support - ' + date, body=post_body, author=self.posting_account, tags=[ 'onboarding', 'retention', 'steem', 'help', 'delegation', 'community', 'giveaway' ]) print('posted') # Reset the cycle self.refresh() def check(self, user): """ Check that the users meet the requirements. user -- the account to be checked -- type: Account/str """ # If an account wasn't passed, make str into account if type(user) != Account: acc = Account(user) # Check account steempower sp = self.sp_check(acc) print(user + " sp == " + str(sp)) if sp <= float(self.min_sp): print('min sp met') # Check account posts per week ppw_check = self.post_check(acc) print(user + " posts per week check == " + str(ppw_check)) if ppw_check: print('posts per week met') # Check self vote percent self_vote_pct = self.vote_check(acc) print(user + ' self votes == %' + str(self_vote_pct)) if self_vote_pct < self.max_sv: print('self vote check met') # Check for powerups and powerdowns powered_up, powered_down = self.vest_check(acc) print(user + " powered up == " + str(powered_up)) print(user + " powered down == " + str(powered_down)) if powered_up and not powered_down: print('vest check met') # All checks completed # Get account age age = self.get_account_age(acc) # Add account to list so their data is stored for the # daily post. self.user_list[user] = { 'sp': round(sp, 3), 'age': age, 'svp': round(self_vote_pct, 2) } print(self.user_list) def sp_check(self, acc): """ Return the users steem power (ignoring outgoing delegations). acc -- the account to be checked -- type: Account """ # Get absolute steempower sp = float(acc.get_steem_power()) # Get all outgoing delegations and add them to account sp for delegation in acc.get_vesting_delegations(): vests = float(delegation['vesting_shares']['amount']) precision = delegation['vesting_shares']['precision'] dele_sp_raw = self.s.vests_to_sp(vests) dele_sp = dele_sp_raw / 10**precision sp += dele_sp return sp def vest_check(self, acc): """Check for opwer ups/downs and return powered_up (bool), powered_down (bool). acc -- the account to be checked -- type: Account """ # get all time powerup and powerdown history powered_up = False powered_down = False vest_history = acc.history_reverse( only_ops=['withdraw_vesting', 'transfer_to_vesting']) # check for powerups and powerdowns for change in vest_history: if change['type'] == 'withdraw_vesting': powered_down = True if change['type'] == 'transfer_to_vesting': powered_up = True if powered_up and powered_down: break return powered_up, powered_down def vote_check(self, acc): """Return the self vote percentage based on weight. acc -- the account to be checked -- type: Account """ # Declare variables total = 0 self_vote = 0 # Date datetime for a week ago wa = self._get_date_past() # Go through all votes in the past week for i in acc.history_reverse(only_ops=['vote'], stop=wa): # Update total total += int(i['weight']) # Update self vote total if self vote if i['author'] == i['voter']: self_vote += int(i['weight']) # No votes made results in /0 error, return 0% self vote if total == 0: return 0 self_vote_pct = (self_vote / total) * 100 return self_vote_pct def post_check(self, acc): """Return true if posts per week requirement is met, false if not. acc -- the account to be checked -- type: Account """ # Get datetime for a week ago wa = self._get_date_past() # Get comment history for the last week blog_history = acc.history_reverse(only_ops=['comment'], stop=wa) # Count how many posts were made in the last week count = 0 for post in blog_history: # Check to make sure it isn't a reply if post['parent_author'] == '': count += 1 if self.posts_per_week >= count: return True return True def _get_date_past(self, days_ago=7): """Returns the datetime for the current time, x days ago. days_ago -- how many days ago you want the datetime for -- type: int """ now = datetime.now() return now - timedelta(days=days_ago) def refresh(self): """Called when the main module has finished executing and the post has been made. It resets all the settings and resets the cycle. """ self.user_list = dict() self.load_settings() self.timer_start() def failover(self): """Switch/cycle through nodes.""" self.nodes = self.nodes[1:] + [self.nodes[0]] print('Switching nodes to ' + self.nodes[0])
from beem import Steem steem = Steem(keys=["<priv_key>"], ) beneficiaries = [ { 'account': 'crokkon', 'weight': 1000 }, { 'account': 'holger80', 'weight': 1000 }, ] steem.post( "Benef test with beem", "Benef test", author="beemtutorials", permlink="beemtutorials-test-post-benefs-2", tags="beem test test-post", beneficiaries=beneficiaries, )
def post_to_hive(title, body, permlink, app, tags, beneficiaries): hivenode = nodelist.get_nodes(hive=True) hive = Steem(node=hivenode, is_hive=True) hive.wallet.unlock(walletpw) hive.post(title, body, author="travelfeed", permlink=permlink, json_metadata=None, app=app, tags=tags, beneficiaries=beneficiaries, parse_body=True)
def post_to_steem(title, body, permlink, app, tags, beneficiaries): node_list = nodelist.get_nodes() steem = Steem(node=node_list) steem.wallet.unlock(walletpw) steem.post(title, body, author="travelfeed", permlink=permlink, json_metadata=None, app=app, tags=tags, beneficiaries=beneficiaries, parse_body=True)
class Distribubot: def __init__(self, config, data_file, steemd_instance): self.config = config self.data_file = data_file self.stm = steemd_instance self.token_config = {} # add log stats self.log_data = { "start_time": 0, "last_block_num": None, "new_commands": 0, "stop_block_num": 0, "stop_block_num": 0, "time_for_blocks": 0 } config_cnt = 0 necessary_fields = [ "token_account", "symbol", "min_token_in_wallet", "comment_command", "token_memo", "reply", "sucess_reply_body", "fail_reply_body", "no_token_left_body", "user_can_specify_amount", "usage_upvote_percentage", "no_token_left_for_today", "token_in_wallet_for_each_outgoing_token", "maximum_amount_per_comment", "count_only_staked_token" ] self.token_config = check_config(self.config["config"], necessary_fields, self.stm) self.blockchain = Blockchain(mode='head', steem_instance=self.stm) def run(self, start_block, stop_block): self.stm.wallet.unlock(self.config["wallet_password"]) self.blockchain = Blockchain(mode='head', steem_instance=self.stm) current_block = self.blockchain.get_current_block_num() if stop_block is None or stop_block > current_block: stop_block = current_block if start_block is None: start_block = current_block last_block_num = current_block - 1 else: last_block_num = start_block - 1 self.log_data["start_block_num"] = start_block for op in self.blockchain.stream(start=start_block, stop=stop_block, opNames=["comment"], max_batch_size=50): self.log_data = print_block_log(self.log_data, op, self.config["print_log_at_block"]) last_block_num = op["block_num"] if op["type"] == "comment": token = None for key in self.token_config: if op["body"].find( self.token_config[key]["comment_command"]) >= 0: token = key if token is None: continue if op["author"] == self.token_config[token]["token_account"]: continue cnt = 0 c_comment = None while c_comment is None and cnt < 5: cnt += 1 try: c_comment = Comment(op, steem_instance=self.stm) c_comment.refresh() except: nodelist = NodeList() nodelist.update_nodes() self.stm = Steem(node=nodelist.get_nodes(), num_retries=5, call_num_retries=3, timeout=15) time.sleep(1) if cnt == 5: logger.warn("Could not read %s/%s" % (op["author"], op["permlink"])) continue if c_comment.is_main_post(): continue if abs((c_comment["created"] - op['timestamp']).total_seconds()) > 9.0: logger.warn("Skip %s, as edited" % c_comment["authorperm"]) continue already_voted = False for v in c_comment["active_votes"]: if self.token_config[token]["token_account"] == v["voter"]: already_voted = True if already_voted: continue already_replied = None cnt = 0 while already_replied is None and cnt < 5: cnt += 1 try: already_replied = False for r in c_comment.get_all_replies(): if r["author"] == self.token_config[token][ "token_account"]: already_replied = True except: already_replied = None self.stm.rpc.next() if already_replied is None: already_replied = False for r in c_comment.get_all_replies(): if r["author"] == self.token_config[token][ "token_account"]: already_replied = True if already_replied: continue muting_acc = Account(self.token_config[token]["token_account"], steem_instance=self.stm) blocked_accounts = muting_acc.get_mutings() if c_comment["author"] in blocked_accounts: logger.info("%s is blocked" % c_comment["author"]) continue # Load bot token balance bot_wallet = Wallet(self.token_config[token]["token_account"], steem_instance=self.stm) symbol = bot_wallet.get_token( self.token_config[token]["symbol"]) # parse amount when user_can_specify_amount is true amount = self.token_config[token]["default_amount"] if self.token_config[token]["user_can_specify_amount"]: start_index = c_comment["body"].find( self.token_config[token]["comment_command"]) stop_index = c_comment["body"][start_index:].find("\n") if stop_index >= 0: command = c_comment["body"][start_index + 1:start_index + stop_index] else: command = c_comment["body"][start_index + 1:] command_args = command.replace(' ', ' ').split(" ")[1:] if len(command_args) > 0: try: amount = float(command_args[0]) except Exception as e: exc_type, exc_obj, exc_tb = sys.exc_info() fname = os.path.split( exc_tb.tb_frame.f_code.co_filename)[1] logger.warn("%s - %s - %s" % (str(exc_type), str(fname), str(exc_tb.tb_lineno))) logger.info("Could not parse amount") if self.token_config[token][ "maximum_amount_per_comment"] and amount > self.token_config[ token]["maximum_amount_per_comment"]: amount = self.token_config[token][ "maximum_amount_per_comment"] if not self.config["no_broadcast"] and self.stm.wallet.locked( ): self.stm.wallet.unlock(self.config["wallet_password"]) self.log_data["new_commands"] += 1 wallet = Wallet(c_comment["author"], steem_instance=self.stm) token_in_wallet = wallet.get_token( self.token_config[token]["symbol"]) balance = 0 if token_in_wallet is not None: logger.info(token_in_wallet) if self.token_config[token]["count_only_staked_token"]: balance = 0 else: balance = float(token_in_wallet["balance"]) if "stake" in token_in_wallet: balance += float(token_in_wallet['stake']) if 'delegationsIn' in token_in_wallet and float( token_in_wallet['delegationsIn']) > 0: balance += float(token_in_wallet['delegationsIn']) if 'pendingUnstake' in token_in_wallet and float( token_in_wallet['pendingUnstake']) > 0: balance += float(token_in_wallet['pendingUnstake']) if balance > self.token_config[token][ "min_token_in_wallet"]: if self.token_config[token][ "token_in_wallet_for_each_outgoing_token"] > 0: max_token_to_give = int( balance / self.token_config[token] ["token_in_wallet_for_each_outgoing_token"]) else: max_token_to_give = self.token_config[token][ "maximum_amount_per_comment"] else: max_token_to_give = 0 else: max_token_to_give = 0 logger.info("token to give for %s: %f" % (c_comment["author"], max_token_to_give)) db_data = read_data(self.data_file) if "accounts" in db_data and c_comment["author"] in db_data[ "accounts"] and token in db_data["accounts"][ c_comment["author"]]: if db_data["accounts"][c_comment["author"]][token][ "last_date"] == date.today( ) and self.token_config[token][ "token_in_wallet_for_each_outgoing_token"] > 0: max_token_to_give = max_token_to_give - db_data[ "accounts"][c_comment["author"]][token]["amount"] if amount > max_token_to_give: amount = max_token_to_give if amount > self.token_config[token][ "maximum_amount_per_comment"]: amount = self.token_config[token][ "maximum_amount_per_comment"] if token_in_wallet is None or balance < self.token_config[ token]["min_token_in_wallet"]: reply_body = self.token_config[token]["fail_reply_body"] elif max_token_to_give < 1: reply_body = self.token_config[token][ "no_token_left_for_today"] elif c_comment["parent_author"] == c_comment["author"]: reply_body = "You cannot sent token to yourself." elif float(symbol["balance"]) < amount: reply_body = self.token_config[token]["no_token_left_body"] else: if "{}" in self.token_config[token]["sucess_reply_body"]: reply_body = (self.token_config[token] ["sucess_reply_body"]).format( c_comment["parent_author"]) else: reply_body = self.token_config[token][ "sucess_reply_body"] if "{}" in self.token_config[token]["token_memo"]: token_memo = ( self.token_config[token]["token_memo"]).format( c_comment["author"]) else: token_memo = self.token_config[token]["token_memo"] sendwallet = Wallet( self.token_config[token]["token_account"], steem_instance=self.stm) try: logger.info( "Sending %.2f %s to %s" % (amount, self.token_config[token]["symbol"], c_comment["parent_author"])) sendwallet.transfer(c_comment["parent_author"], amount, self.token_config[token]["symbol"], token_memo) if "accounts" in db_data: accounts = db_data["accounts"] else: accounts = {} if c_comment["author"] not in accounts: accounts[c_comment["author"]] = {} accounts[c_comment["author"]][token] = { "last_date": date.today(), "n_comments": 1, "amount": amount } elif token not in accounts[c_comment["author"]]: accounts[c_comment["author"]][token] = { "last_date": date.today(), "n_comments": 1, "amount": amount } else: if accounts[c_comment["author"]][token][ "last_date"] < date.today(): accounts[c_comment["author"]][token] = { "last_date": date.today(), "n_comments": 1, "amount": amount } else: accounts[c_comment["author"]][token][ "n_comments"] += 1 accounts[c_comment["author"]][token][ "amount"] += amount store_data(self.data_file, "accounts", accounts) logger.info( "%s - %s" % (c_comment["author"], str(accounts[c_comment["author"]][token]))) except Exception as e: exc_type, exc_obj, exc_tb = sys.exc_info() fname = os.path.split( exc_tb.tb_frame.f_code.co_filename)[1] logger.warn( "%s - %s - %s" % (str(exc_type), str(fname), str(exc_tb.tb_lineno))) logger.warn("Could not send %s token" % self.token_config[token]["symbol"]) continue reply_identifier = construct_authorperm( c_comment["parent_author"], c_comment["parent_permlink"]) if self.config["no_broadcast"]: logger.info("%s" % reply_body) else: try: self.stm.post( "", reply_body, author=self.token_config[token]["token_account"], reply_identifier=reply_identifier) if self.token_config[token][ "usage_upvote_percentage"] <= 0: time.sleep(5) self.stm.post( "", "Command accepted!", author=self.token_config[token] ["token_account"], reply_identifier=c_comment["authorperm"]) except Exception as e: exc_type, exc_obj, exc_tb = sys.exc_info() fname = os.path.split( exc_tb.tb_frame.f_code.co_filename)[1] logger.warn( "%s - %s - %s" % (str(exc_type), str(fname), str(exc_tb.tb_lineno))) logger.warn("Could not reply to post") continue if self.token_config[token]["usage_upvote_percentage"] > 0: time.sleep(5) try: c_comment.upvote(self.token_config[token] ["usage_upvote_percentage"], voter=self.token_config[token] ["token_account"]) except Exception as e: exc_type, exc_obj, exc_tb = sys.exc_info() fname = os.path.split( exc_tb.tb_frame.f_code.co_filename)[1] logger.warn("%s - %s - %s" % (str(exc_type), str(fname), str(exc_tb.tb_lineno))) logger.warn("Could not upvote comment") time.sleep(4) return last_block_num
from beem import Steem steem = Steem(keys=["<private_posting_wif>"]) steem.post("My title for the post", "Beem is awesome! (post body)", author="beemtutorials", permlink="beemtutorials-test-post", app="myapp/0.0.1", tags="beem test test-post")
if c_comment.is_main_post(): c = c_comment else: c = Comment(construct_authorperm(c_comment["parent_author"], c_comment["parent_permlink"]), steem_instance=stm) if not (c.is_pending() and valid_age(c)): body = "The reward of this comment goes 100 %% to the author %s. This is done by setting the beneficiaries of this comment to 100 %%.\n\n" % ( c["author"]) comment_beneficiaries = [{"account": c["author"], "weight": 10000}] permlink = derive_permlink("rewarding %s" % c["author"], c_comment["permlink"]) stm.post("rewarding %s" % c["author"], body, author=rewarding_account, permlink=permlink, reply_identifier=c_comment["authorperm"], beneficiaries=comment_beneficiaries) time.sleep(3) authorperm = construct_authorperm(rewarding_account, permlink) comment_timestamp = datetime.utcnow() main_post = False else: authorperm = c["authorperm"] main_post = c.is_main_post() comment_timestamp = c["created"].replace(tzinfo=None) if command["valid"] and posting_auth: pending_vote = {