"landmarks", local_image) print("\nLandmarks in the local image:") if len(local_image_landmarks.result["landmarks"]) == 0: print("No landmarks detected.") else: for landmark in local_image_landmarks.result["landmarks"]: print(landmark["name"]) # END Detect domain-specific content (celebrities/landmarks) in a local image # Detect domain-specific content (celebrities/landmarks) in a remote image by: # 1. Calling the Computer Vision service's analyze_image_by_domain with the: # - domain-specific content to search for # - image # 2. Displaying any domain-specific content (celebrities/landmarks). remote_image_celebs = computervision_client.analyze_image_by_domain( "celebrities", remote_image_url) print("\nCelebrities in the remote image:") if len(remote_image_celebs.result["celebrities"]) == 0: print("No celebrities detected.") else: for celeb in remote_image_celebs.result["celebrities"]: print(celeb["name"]) remote_image_landmarks = computervision_client.analyze_image_by_domain( "landmarks", remote_image_url) print("\nLandmarks in the remote image:") if len(remote_image_landmarks.result["landmarks"]) == 0: print("No landmarks detected.") else:
display momentarily. Location: {}""".format(url)) # Type of prediction. domain = "landmarks" mlpreview(url) # English language response. language = "en" try: analysis = client.analyze_image_by_domain(domain, url, language) except Exception as e: catch_exception(e, url) mlask() for landmark in analysis.result["landmarks"]: print('\nIdentified "{}" with confidence {}.'.format( landmark["name"], round(landmark["confidence"], 2))) mlask(begin="\n", end="\n") url1 = "https://cdn.britannica.com/" url2 = "95/94195-050-FCBF777E/" url3 = "Golden-Gate-Bridge-San-Francisco.jpg" url = url1 + url2 + url3
print(landmark["name"]) print() ''' END - Detect Domain-specific Content - local ''' # <snippet_celebs> ''' Detect Domain-specific Content - remote This example detects celebrites and landmarks in remote images. ''' print("===== Detect Domain-specific Content - remote =====") # URL of one or more celebrities remote_image_url_celebs = "https://raw.githubusercontent.com/Azure-Samples/cognitive-services-sample-data-files/master/ComputerVision/Images/faces.jpg" # Call API with content type (celebrities) and URL detect_domain_results_celebs_remote = computervision_client.analyze_image_by_domain("celebrities", remote_image_url_celebs) # Print detection results with name print("Celebrities in the remote image:") if len(detect_domain_results_celebs_remote.result["celebrities"]) == 0: print("No celebrities detected.") else: for celeb in detect_domain_results_celebs_remote.result["celebrities"]: print(celeb["name"]) # </snippet_celebs> # <snippet_landmarks> # Call API with content type (landmarks) and URL detect_domain_results_landmarks = computervision_client.analyze_image_by_domain("landmarks", remote_image_url) print()
client = ComputerVisionClient(uri, credencias) client.api_version """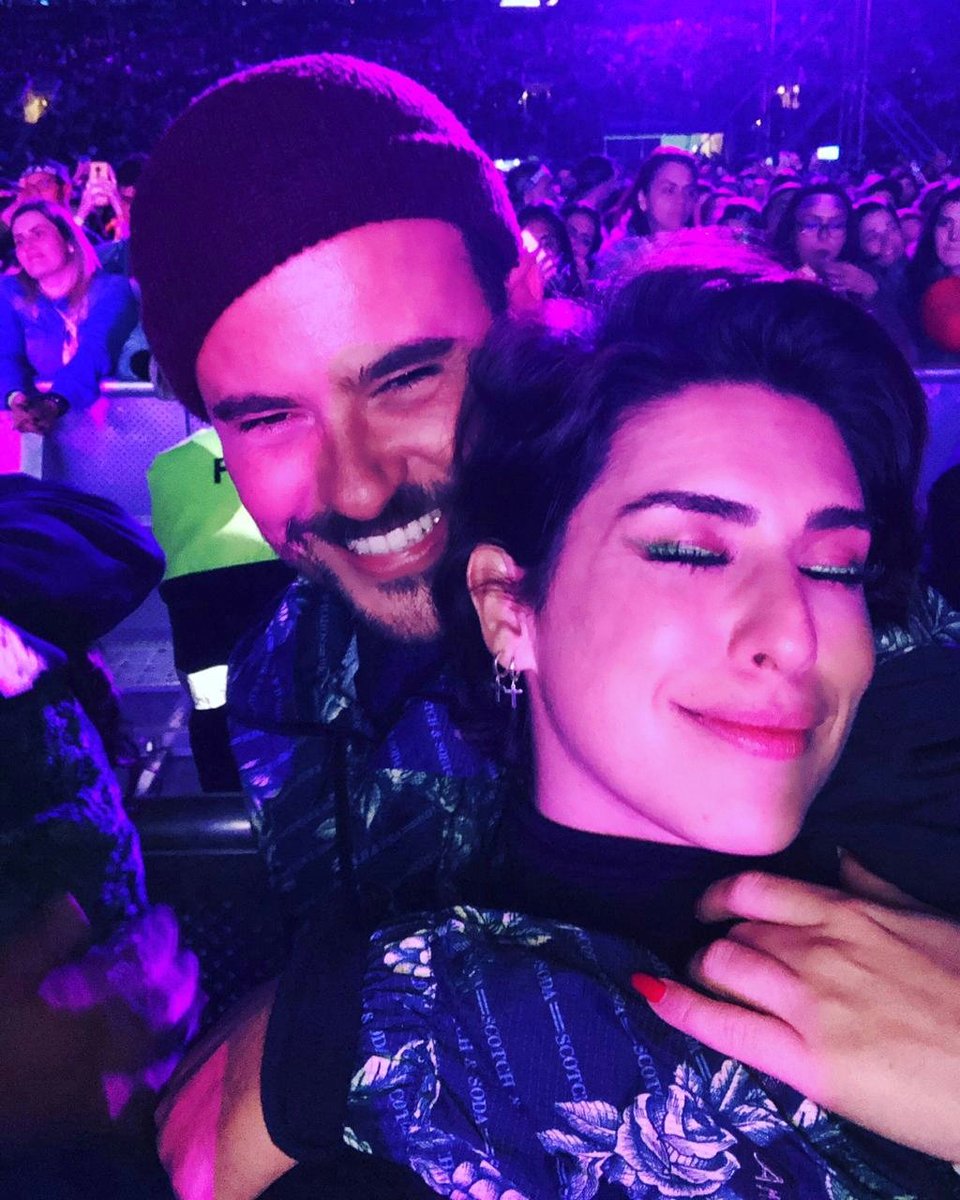""" from azure.cognitiveservices.vision.computervision.models import VisualFeatureTypes url = 'https://pbs.twimg.com/media/ECx6hK-WwAAPzeE.jpg' analise_de_imagem = client.analyze_image(url, visual_features = [VisualFeatureTypes.tags]) for tag in analise_de_imagem.tags: print(tag) analise_de_celebridades = client.analyze_image_by_domain("celebrities", url, "en") for celebridade in analise_de_celebridades.result["celebrities"]: print(celebridade['name']) print(celebridade['confidence']) descricacao = client.describe_image(url,3,"en") descricacao for caption in descricacao.captions: print(caption.text) print(caption.confidence) """# Streaming + Vision API""" import json
# Collect the Config # # # ################################################# with open('config.json') as config_file: data = json.load(config_file) KEY = data['key'] ENDPOINT = data['endpoint'] computervision_client = ComputerVisionClient(ENDPOINT, CognitiveServicesCredentials(KEY)) # Get an image to find its tags remote_image_url = "https://www.thetimes.co.uk/imageserver/image/methode%2Ftimes%2Fprod%2Fweb%2Fbin%2Fc994ba96-5632-11e9-a8f5-a9ee11ff7e6d.jpg?crop=4896%2C2754%2C0%2C255&resize=1200" # Alternate 1 remote_image_url = "https://mymodernmet.com/wp/wp-content/uploads/2018/11/egyptian-pyramids-3.jpg" # Alternate 2 remote_image_url = "https://cdn.galaxy.tf/unit-media/tc-default/uploads/images/poi_photo/001/549/654/golden-gate-bridge-1-standard.jpg" # Call API with content type (landmarks) and URL detect_domain_results_landmarks = computervision_client.analyze_image_by_domain( "landmarks", remote_image_url) print() print("Landmarks in the remote image:") if len(detect_domain_results_landmarks.result["landmarks"]) == 0: print("No landmarks detected.") else: for landmark in detect_domain_results_landmarks.result["landmarks"]: print(landmark["name"])
img.show() # Call API to describe image description_result = computer_vision_client.describe_image(query_image_url) # Get the captions (descriptions) from the response, with confidence level print() print("Description of image: ") if (len(description_result.captions) == 0): print("No description detected.") else: for caption in description_result.captions: print("'{}' with confidence {:.2f}%".format(caption.text, caption.confidence * 100)) print() # Detect domain-specific content, celebrities, in image # Call API with content type (celebrities) and URL detect_domain_celebrity_result = computer_vision_client.analyze_image_by_domain( "celebrities", query_image_url) # Print detection results with name celebrities = detect_domain_celebrity_result.result["celebrities"] celebrity_name = '' print("Celebrities in the image:") if len(detect_domain_celebrity_result.result["celebrities"]) == 0: print("No celebrities detected.") else: for celeb in celebrities: celebrity_name = celeb["name"] print(celeb["name"])