def continuous_ttest_from_stats_ui(): '''The Two-sample Student's t-test - Continuous variables (from statistics) section. ''' # Render the header. with st.beta_container(): st.title('Two-sample Student\'s t-test') st.header('Continuous variables') # Render input boxes and plots with st.beta_container(): col1, col2 = st.beta_columns([1, 1]) with col1: st.subheader('Group A') visitors_1 = st.number_input('Sample size A: ', value=80000) mu_1 = st.number_input('Sample mean A: ', value=64.5) sigma_1 = st.number_input('Sample standard deviation A: ', min_value=0.0, value=30.8) conf_level = st.select_slider('Confidence level: ', ('0.90', '0.95', '0.99')) with col2: st.subheader('Group B') visitors_2 = st.number_input('Sample size B: ', value=80000) mu_2 = st.number_input('Sample mean B: ', value=68.7) sigma_2 = st.number_input('Sample standard deviation B: ', min_value=0.0, value=38.1) hypo_type = st.radio('Hypothesis type: ', ('One-sided', 'Two-sided')) # Calculate statistics tstat, p_value, tstat_denom, pooled_sd, effect_size = scipy_ttest_ind_from_stats( mu_1, mu_2, sigma_1, sigma_2, visitors_1, visitors_2) observed_power = sm_tt_ind_solve_power(effect_size=effect_size, n1=visitors_1, n2=visitors_2, alpha=1-float(conf_level), power=None, hypo_type=hypo_type, if_plot=False) # Render the results ttest_plot(mu_1, mu_2, sigma_1, sigma_2, conf_level, tstat, p_value, tstat_denom, hypo_type, observed_power)
def app(): media = st.beta_container() image = st.beta_container() df = pd.read_csv('Books_total.csv') with media: st.subheader('Book Release:') pup = pd.DataFrame(df['1st Pub'].value_counts()).head(50) st.bar_chart(pup) st.markdown(''' The release in 2003 is the highst. ''') st.subheader('Authors and how many Books they wrote:') author = pd.DataFrame(df['Author'].value_counts()).head(50) st.area_chart(author) st.markdown( '''Here we can see clearly that **Stephen King** and **William Shakespeare** published the most books ''') st.subheader('Rating Count:') rating_count = pd.DataFrame(df['Rating Count'].value_counts()).head(50) st.area_chart(rating_count) with image: st.subheader('Avg-Ratings / Pages') image = Image.open('plot1.jpeg') st.image(image, caption='') st.subheader('Avg-Ratings / Author') image = Image.open('plot2.jpeg') st.image(image, caption='')
def login_widget(): st.markdown("<div class='spacediv'></div>", unsafe_allow_html=True) st.markdown("<div class='spacediv'></div>", unsafe_allow_html=True) st.markdown("<div class='spacediv'></div>", unsafe_allow_html=True) with st.beta_container(): lph, title, rph = st.beta_columns((1, 2, 1)) with title: st.header('Login') with st.beta_container(): lph, title, rph = st.beta_columns((1, 2, 1)) with title: session_id = load_session_id() cookie = st.text_input('Cookie', session_id) with st.beta_container(): lph, but, rph = st.beta_columns((10, 1, 10)) with but: if st.button('Entrar'): state.cookie = cookie state.authorized = connect_database() if state.authorized: load_data() st.experimental_rerun() st.markdown("<div class='spacediv'></div>", unsafe_allow_html=True) st.markdown("<div class='spacediv'></div>", unsafe_allow_html=True) st.markdown("<div class='spacediv'></div>", unsafe_allow_html=True)
def main(): header=st.beta_container() features=st.beta_container() result=st.beta_container() with header: st.title("Stroke Prediction WebApp") st.text("Enter the data asked below and know wheather you are at a risk of having a stroke or not!") with features: st.header("Enter the data of the features listed below.") gen=st.selectbox('Gender:', options=['Male','Female']) age=st.slider("Age:", 0, 120) marr=st.selectbox("Ever married:", options=['Yes','No']) res=st.selectbox("Residence Type:", options=["Urban", "Rural"]) glucose=st.number_input("Enter your Glucose Level (Lastest):") tension=st.selectbox("Hypertension:", options=['Yes','No']) hdisease=st.selectbox("Heart Disease:", options=['Yes','No']) bmi=st.number_input("Enter your BMI (in kilogram per metre square):") st.info("BMI=Weight(kg)/(Height*Height (m))") with result: st.text("") st.text("") if st.button("Predict for Stroke"): r=prediction2(gen,age,marr,res,glucose,tension,hdisease,bmi) if r==0: st.success('You are safe') else: st.error('You are at risk')
def main(): st.beta_container() st.beta_columns(2) #st.set_page_config(layout="centered") st.title("Association Rule Mining") html_temp = """ <div style="background-color:#025246 ;padding:10px"> <h2 style="color:white;text-align:center;">Association Rule Generator </h2> </div> </br> """ st.markdown(html_temp, unsafe_allow_html=True) file = st.file_uploader('Select a dataset file.') if file is None: return None, None, None, None, None, None df = process_file(file) support = st.number_input("Support", 0.01) confidence = st.number_input("Confidence", 0.01) lift = st.number_input("Lift", 2) if st.button("Generate Rule"): output = modelling(df, support, confidence, lift) sizeResult = getLength(output) printOutput = print_result2(output) st.success('Number of rules generated : {} rules'.format(sizeResult)) st.table(printOutput)
def app(): header = st.beta_container() image = st.beta_container() with header: st.title('Books That Everyone Should Read At Least Once\n') st.text('') st.text('') st.text('') st.text('') st.title('⚜️ About ⚜️:') st.markdown(''' Books are disponible for everyone, rich and poor, young and old. From love stroies to Sci Fi, it's gonna change the world for everyone. How will your life be affected ?📖 ⬇️⬇️ This Project was created by 4 ***[Strive School](https://strive.school/)*** Students.⬇️⬇️ ''') st.markdown('## Get to know us 👋🏻:') if st.button('Click here'): st.markdown("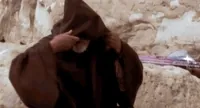") st.write('***The Developer Team***:') st.write('• Sven Skyth Henriksen: [GitHub ](https://github.com/Sven-Skyth-Henriksen)&[ LinkedIn](https://www.linkedin.com/in/sven-skyth-henriksen-4857bb1a2/)') st.write('• Madina Zhenisbek: [GitHub ](https://github.com/madinach)&[ LinkedIn](https://www.linkedin.com/in/madina-zhenisbek/)') st.write('• Olatunde Salami: [GitHub ](https://github.com/salamituns)&[ LinkedIn](https://www.linkedin.com/in/olatunde-salami/)') st.write('• Paramveer Singh: [GitHub ](https://github.com/paramveer)&[ LinkedIn](https://www.linkedin.com/in/paramveer-singh07/)') with image: image = Image.open('book.jpeg') st.image(image, caption='Quote by ERNEST HEMINGWAY')
def app(): with st.beta_container(): st.title('Options Sniper') st.write('Coming Soon - the fattest spread premiums for a list of stocks') st.subheader('Options Chain') st.write('gets the option chain for a stock from the datasets folder') with st.beta_container(): col1, col2, col3 = st.beta_columns([1,1,2]) symbol_input = col1.text_input('Enter Stock Symbol', max_chars=5) max_dte = col2.number_input('Enter Max DTE', value=60, format='%d') col2.write('When functional, this will limit the chain to max DTE {}'.format(max_dte)) with st.beta_container(): s = symbol_input.upper() if not symbol_input: st.warning('Please enter a symbol.') st.text_input('Please enter') try: df = pd.read_csv('datasets/o_{}.csv'.format(s)) st.write('Options Chain for', s, df) except: msg = '{} not found. This only works for AMD'.format(s) st.write(msg)
def ttest_power_ui(): '''The Power Analysis for Two-sample Student's t-test section. ''' # Render the header. with st.beta_container(): st.title('Two-sample Student\'s t-test') st.header('Power analysis') # Render input boxes and plots with st.beta_container(): param_list = ['Standardized effect size', 'alpha (1 - Significance level)', 'Power (1 - beta)', 'Group A sample size'] param_name = {'Standardized effect size': 'effect_size', 'alpha (1 - Significance level)': 'alpha', 'Power (1 - beta)': 'power', 'Group A sample size': 'n1'} param_default = { 'Standardized effect size': {'min_value': 0.0, 'value': 0.2}, 'alpha (1 - Significance level)': {'min_value': 0.01, 'max_value': 0.5, 'value': 0.05}, 'Power (1 - beta)': {'min_value': 0.01, 'max_value': 0.99, 'value': 0.8}, 'Group A sample size': {'min_value': 10, 'value': 1000}} kwargs = {} unknown_param = st.selectbox('Solve for: ', param_list) param_list.remove(unknown_param) for param in param_list: kwargs[param] = st.number_input(param, **param_default[param]) kwargs = {param_name[param]: kwargs[param] for param in param_list} kwargs['ratio'] = st.number_input('Sample size Ratio (Group B : Group A)', min_value=0.01, value=1.0) try: value, fig = sm_tt_ind_solve_power(**kwargs) except: st.exception('The solution is out of bound. Please adjust the input parameters. ') st.stop() st.success('{}: `{:.4f}`'.format(unknown_param, value)) st.write(fig, width=200)
def run_app(): a: float b: float dataset_select: str with st.sidebar.beta_container(): st.write('## Parameters') dataset_select = st.selectbox( 'Select dataset', [ 'turkish.csv' # 'nasa93.csv', ]) a = st.number_input('A', value=2.94) b = st.number_input('B', value=0.91) c = create_cocomo(dataset_select, a, b) st.write( f'## Load dataset \n Currently using `{dataset_select}` dataset (change on sidebar)' ) st.dataframe(c.data) '---' st.write('## Compute EM, EE, MRE') with st.beta_container(): left, right = st.beta_columns(2) left.write('### Effort multiplier (EM)') left.latex(r'EM = \prod_{i=1}^{17} SF_i') left.write('> SF = scale factor') left.write('### Estimated effort (EE)') left.latex(r'F(A,B) = A \times Size^B \times EM') left.write('> EE = objective function -> F(A,B)') left.write('### Magnitude relative error (MRE)') left.latex(r'MRE = \frac{|AE-EE|}{EE} \times 100\%') right.write('### Results') right.dataframe({ 'EM': c.effort_multipliers, 'F(A,B)': c.estimated_efforts, 'MRE': c.mre * 100, }) st.write('---') st.write('## Compute MMRE') with st.beta_container(): left, right = st.beta_columns([2, 1]) left.write('### Mean magnitude relative error (MMRE)') left.latex(r'MMRE = \frac{1}{N} \sum_{x=1}^{N} MRE') right.write('### Results') right.write(pd.DataFrame({'MMRE': [c.mmre * 100]}))
def test_nested_with(self): with st.beta_container(): with st.beta_container(): st.markdown("Level 2 with") msg = self.get_message_from_queue() self.assertEqual(msg.metadata.parent_block.path, [0, 0]) st.markdown("Level 1 with") msg = self.get_message_from_queue() self.assertEqual(msg.metadata.parent_block.path, [0])
def write(): tweet_input = st.beta_container() model_results = st.beta_container() contact = st.beta_container() with tweet_input: st.header('Is Your Tweet Considered Hate Speech?') user_text = st.text_input('Write the Tweet', max_chars=280) # setting input as user_text with model_results: if st.button("Predict"): # removing punctuation #user_text = re.sub('[%s]' % re.escape(string.punctuation), '', user_text) user_text = clean_tweet(user_text) # Customizing stop words list stop_words = stopwords.words('english') newStopWords = ['ur','u','nd'] # new stop word remove_stopword = ['not','no','nor',"don","aren","couldn","didn","hadn","hasn","haven","isn","mustn","mightn","needn","shouldn", "wasn","wouldn","won"] # stop words that we don't want stop_words.extend(newStopWords) # add new stop word stop_words = [OldStopWords for OldStopWords in stop_words if OldStopWords not in remove_stopword] # remove some stop words # tokenizing tokens = nltk.word_tokenize(user_text) # removing stop words stopwords_removed = [token.lower() for token in tokens if not token.lower() in set(stop_words)] # taking root word lemmatizer = WordNetLemmatizer() lemmatized_output = [] for word in stopwords_removed: lemmatized_output.append(lemmatizer.lemmatize(word)) # instantiating count vectorizor tfidf = TfidfVectorizer() X_train = pickle.load(open('pickle/X_train.pkl', 'rb')) X_test = lemmatized_output tfidf_data_train = tfidf.fit_transform(X_train) tfidf_data_test = tfidf.transform(X_test) # loading in model final_model = pickle.load(open('pickle/svm_model.pkl', 'rb')) # apply model to make predictions prediction = final_model.predict(tfidf_data_test[0]) if prediction == 0: st.error('**Hate Speech :anger:**') elif prediction == 1: st.warning('**Offensive Language :collision:**') else: st.success('**Neither :smiley:**') st.text('')
def format_output(predictions_all, weighted_by_words, n_texts, articles): """ format output """ with st.beta_container(): r1_c1, r1_c2, r1_c3 = st.beta_columns((1, 1.5, 1)) out_all = pipeline.format_topic_sent(dic=predictions_all, doc=articles[0:n_texts]) out_all["nr_words"] = out_all["Original text"].apply( lambda x: len(x.split())) # topic predictions r1_c1.subheader("Topic predictions") out_top = (pd.DataFrame(predictions_all["Topic"].mean(), columns=['Confidence (%)']) * 100).sort_values( by='Confidence (%)', ascending=False) r1_c1.dataframe(out_top.style.set_precision(2)) # Show weighted normalized sentiment predictions r1_c2.subheader("Weighted normalized sentiment predictions") out_sent = pipeline.compute_weighted_sentiment( dic=predictions_all, weighted_by_words=weighted_by_words, nr_words=out_all["nr_words"]) r1_c2.dataframe(out_sent) # average sentiment r1_c3.subheader("Average sentiment") # weighted if weighted_by_words: sent_words = predictions_all['Sentiment'].multiply( out_all["nr_words"], axis="index").sum() sum_words = out_all["nr_words"].sum() out_avg_sent = pd.DataFrame(sent_words / sum_words, columns=["Confidence %"]) out_avg_sent = (out_avg_sent * 100).sort_values( by="Confidence %", ascending=False).round(3) # simple average else: out_avg_sent = pd.DataFrame(predictions_all['Sentiment'].mean(), columns=["Confidence (%)"]) * 100 r1_c3.dataframe(out_avg_sent.style.set_precision(2)) with st.beta_container(): # all individual predictions r2_c1 = st.beta_columns((1))[0] r2_c1.subheader("All predictions") r2_c1.dataframe(out_all.style.set_precision(2))
def main(): data = pd.read_csv("research-and-development-survey-2019-csv.csv") data_1 = pd.read_csv( "greenhouse-gas-emissions-by-region-industry-and-household-year-ended-2018-csv.csv" ) st.dataframe(data) st.table(data.iloc[0:10]) st.dataframe(data_1) st.table(data_1.iloc[0:10]) st.line_chart(data_1) st.json({'foo': 'bar', 'fu': 'ba'}) st.beta_container() st.beta_columns(4) col1, col2 = st.beta_columns(2) col1.subheader('Columnisation') st.beta_expander('Expander') with st.beta_expander('Expand'): st.write('Juicy deets') st.sidebar.write("# Noniot") a = st.sidebar.radio('R:', [1, 2]) st.button('Hit me') st.checkbox('Check me out') st.radio('Radio', [1, 2, 3]) st.selectbox('Select', [1, 2, 3]) st.multiselect('Multiselect', [1, 2, 3]) st.slider('Slide me', min_value=0, max_value=10) st.select_slider('Slide to select', options=[1, '2']) st.text_input('Enter some text') st.number_input('Enter a number') st.text_area('Area for textual entry') st.date_input('Date input') st.time_input('Time entry') st.file_uploader('File uploader') st.color_picker('Pick a color') st.progress(90) st.spinner() with st.spinner(text='In progress'): time.sleep(5) st.success('Done') st.balloons() st.error('Error message') st.warning('Warning message') st.info('Info message') st.success('Success message') st.exception("e")
def main(): st.set_page_config(page_title="Titanic Survival App") overview = st.beta_container() left, right = st.beta_columns(2) prediction = st.beta_container() with overview: st.title("Titanic App") st.markdown( "Model: Random Forest Classifier, trained on titanic training dataset from [Kaggle](https://www.kaggle.com/c/titanic/data). See associated notebook for details on how the model was trained." ) with left: sex_radio = st.radio("Sex", list(sex_d.keys()), format_func=lambda x: sex_d[x]) pclass_radio = st.radio("Ticket Class", list(pclass_d.keys()), format_func=lambda x: pclass_d[x]) embarked_radio = st.radio("Port of Embarkment", list(embarked_d.keys()), index=2, format_func=lambda x: embarked_d[x]) with right: age_slider = st.slider("Age", value=50, min_value=1, max_value=100) sibsp_slider = st.slider("# Siblings / Spouses on board", min_value=0, max_value=8) parch_slider = st.slider("# Parents / Children on board", min_value=0, max_value=6) fare_slider = st.slider("Passenger Fare", min_value=0, max_value=500, step=10) data = [[ pclass_radio, sex_radio, age_slider, sibsp_slider, parch_slider, fare_slider, embarked_radio ]] survival = model.predict(data) s_confidence = model.predict_proba(data) with prediction: st.header("Survived? {0}".format("Yes" if survival[0] == 1 else "No")) st.subheader("Confidence {0:.2f} %".format( s_confidence[0][survival][0] * 100))
def visualizer(df): visualize = st.beta_container() with visualize: visualize.subheader('1. Dataset') visualize.markdown('1.1. Glimpse of dataset') visualize.write(df.head(10)) # Using all column except for the last column as X X = df.iloc[:, :-1] Y = df.iloc[:, -1] # Selecting the last column as Y visualize.markdown('1.2. Dataset dimension') cols = visualize.beta_columns(2) cols[0].write('X') cols[0].info('{} rows, {} attributes'.format( X.shape[0], X.shape[1])) cols[1].write('Y') cols[1].info('{} responses'.format(Y.shape[0])) visualize.markdown('1.3. Variable details:') visualize.write('X variables (first 20 are shown)') attributes = list(X.columns[:20]) visualize.markdown('{}'.format(attributes)) visualize.write('Y variable') visualize.markdown('{}'.format(Y.name))
def show_dataframe(self, minimal=True): with st.beta_container(): # Options options = [table[0] for table in self.connection.cursor().execute( "SELECT name FROM sqlite_master WHERE type='table';").fetchall()] table = st.selectbox(self.text, options, index=3) st.info(f"Note: due to limited output size, the displayed DataFrame is limited to the first " f"{self.limit_rows} rows only.\n\nHowever, the Pandas Profiling Report " f"calculates on the full DataFrame.") col1, col2 = st.beta_columns(2) with col1: df = _load_df(table, self.connection) self.show_df = df.head(self.limit_rows) # Only shows limited rows self.profile_df = df # Show DataFrame's info buffer = io.StringIO() df.info(buf=buffer) st.text(buffer.getvalue()) # Show HiPlot xp = hip.Experiment.from_dataframe(self.show_df) xp.display_st(key="hip") with col2: # Show Pandas Profile Report self.profile_report = pp.ProfileReport(self.profile_df, minimal=minimal, progress_bar=False) with st.spinner("Generating profile report..."): components.html(self.profile_report.to_html(), height=1500, scrolling=True)
def cabin_processing(self, alldata_sum): st.write("(3)-5. Cabin") alldata_sum['Cabin_init'] = alldata_sum['Cabin'].apply( lambda x: str(x)[0]) with st.beta_container(): col1, col2, col3 = st.beta_columns([1, 1, 1]) with col1: st.write("Mean vs count") # Survival rate and number of records by acronym of Cabin st.write(alldata_sum[alldata_sum['train_or_test'] == 0] ['Survived'].groupby(alldata_sum['Cabin_init']).agg( ['mean', 'count'])) with col2: st.write("Before") # Survival rate and number of records by acronym of Cabin st.write( pd.crosstab(alldata_sum['Cabin_init'], alldata_sum['train_or_test'])) #少数派のCabin_initを統合 alldata_sum['Cabin_init'].replace(['G', 'T'], 'Rare', inplace=True) with col3: st.write("After") # Survival rate and number of records by acronym of Cabin st.write( pd.crosstab(alldata_sum['Cabin_init'], alldata_sum['train_or_test'])) return alldata_sum
def main(): """Main function.""" inverter = StyleGANInverter(model_name, mode=mode, learning_rate=ini_lr, iteration=step, reconstruction_loss_weight=lambda_l2, perceptual_loss_weight=lambda_feat, regularization_loss_weight=lambda_enc, clip_loss_weight=lambda_clip, description=description) image_size = inverter.G.resolution text_inputs = torch.cat([clip.tokenize(description)]).cuda() # Invert images. # uploaded_file = uploaded_file.read() if uploaded_file is not None: image = Image.open(uploaded_file) # st.image(image, caption='Uploaded Image.', use_column_width=True) # st.write("") st.write("Just a second...") image = resize_image(np.array(image), (image_size, image_size)) _, viz_results = inverter.easy_invert(image, 1) if mode == 'man': final_result = np.hstack([image, viz_results[-1]]) else: final_result = np.hstack([viz_results[1], viz_results[-1]]) # return final_result with st.beta_container(): st.image(final_result, use_column_width=True)
def __init__(self): x = st.slider('Select a value') st.write(x, 'squared is', x * x) st.write("Here's our first attempt at using data to create a table:") st.write( pd.DataFrame({ 'first column': [1, 2, 3, 4], 'second column': [10, 20, 30, 40] })) st.beta_container() self.col1, self.col3 = st.beta_columns(2) add_selectbox = st.sidebar.selectbox( "Symbol", ("Email", "Home phone", "Mobile phone")) genre = st.sidebar.radio())
def app(): image = st.beta_container() with image: image = Image.open('data_science.jpeg') st.image(image, caption='')
def app(): st.markdown("<h1 style='text-align: center; color: teal; font-size:5vw'>Defect extraction model</h1>", unsafe_allow_html=True) st.markdown('<style> .stButton>button {font-size: 20px;color: teal;}</style>', unsafe_allow_html=True) with st.spinner("Loading relevant data from database & models...Please wait..."): topic_vectorizer, topic_model, topic_df, defect_model, defect_vectorizer = load() st.write('') with st.beta_container(): text_input = st.text_area(label="Input your review") num_topics = st.number_input("Number of candidate topics",1, 10) if st.button('Extract defects'): if not text_input.strip(): st.error("No text was entered") else: df = pd.DataFrame(data=[text_input], columns=['comment']) df['cleaned_text'] = clean_text(df['comment'], "data/uc3/labels_generator/contractions.txt", "data/uc3/labels_generator/slangs.txt") # First check if defects or not # features = defect_vectorizer.transform(text_input) features = pd.DataFrame(defect_vectorizer.transform(df['cleaned_text']).toarray()) predicted = defect_model.predict(features)[0] if predicted == 1: # If defect is predicted st.header(f"Top {num_topics} topics (in order)") data = app_preprocess(df) data = topic_vectorizer.transform(data['cleaned_text']) mask = np.array(topic_model.predict_proba(data))[0][0] ind = (-mask).argsort()[:num_topics] st.write(topic_df.loc[topic_df.index[list(ind)]]) else: st.header(f"There are no defects identified in the review")
def lb(self): st.title('Reasons for pursuing a Masters') with st.beta_container(): st.markdown(""" There might be many reasonss why one might want to pursue such a degree.The common one's include: - **Deep dive into a particular field** A common reason for many aspirant's is striving to know more about a particular field of interst in much more depth. This usually stems out of curiosity. - **Switching your career** Often many aspirants chose to switch their career after exposure to a given field of choice. They might or might not have complete idea of their field of interest , coming from related field . This is usually good since such candidates can bring their perspective and expertise(if related) into the current role. - **Looking for new Challenge** Many aspirants might be looking to challenge and enhance their skills . It might serve as a personal challenge that they are willing to overcome. - **Carrer growth** There are many statistics regarding career growth after a Master's degree. Although usuallly such statistics learn towards higher incomes after completion, one should not purely base the decision for money's sake.This can end up being dangerous as you might regret doing your job a lot. """) with st.beta_expander('~Expand more '): st.markdown( 'Well that took a **different turn** altogether😶') st.image(RLTY, caption="Funny ")
def create_train_test(self, alldata_sum): # Define target variables and columns unnecessary for learning target_col = 'Survived' drop_col = ['PassengerId','Survived', 'Name', 'Fare', 'Ticket', 'Cabin', 'train_or_test', 'Parch', 'FamilySize', 'SibSp'] # Separate first integrated train and test train = alldata_sum[alldata_sum['train_or_test'] == 0] test = alldata_sum[alldata_sum['train_or_test'] == 1] # Holds only the features required for learning train_feature = train.drop(columns=drop_col) test_feature = test.drop(columns=drop_col) train_tagert = train[target_col] st.write('The size of the train data:' + str(train_feature.shape)) st.write('The size of the test data:' + str(test_feature.shape)) if train_feature.shape[1] != test_feature.shape[1]: st.warning("Note: Bad status of feature. Please check your preprocessing") with st.beta_container(): col1, col2 = st.beta_columns([1, 1]) with col1: st.write("Before ") st.write(alldata_sum.dtypes) with col2: st.write("After") st.write(train_feature.dtypes) return train_feature, train_tagert, test_feature
def page(): title_container = st.beta_container() title_container.title("パスワードの再設定") title_container = None api_key = st.secrets["firebase_config"]["api_key"] uri = f"https://identitytoolkit.googleapis.com/v1/accounts:sendOobCode?key={api_key}" email = st.text_input("再設定用メールアドレス") send_button = st.button("上記メールアドレスへ再設定用リンクを送信") if send_button: res = reset(uri, email) if "error" in res: error = res["error"]["message"] if error == "EMAIL_NOT_FOUND": error_text = "このメールアドレスは登録されていません" elif error == "MISSING_EMAIL": error_text = "メールアドレスを入力してください" elif error == "INVALID_EMAIL": error_text = "このメールアドレスは無効です" else: error_text = error st.error(error_text) else: st.success("送信が完了しました")
def generatetweetlayout(name, tweet, created_at, url): tweet = st.beta_container() with tweet: st.markdown(f'**Author:** _{name}_ | **Tweet Date:** _{created_at}_') st.write(tweet) st.markdown(f'''<a href={url}>Read Original Tweet...</a> <br>''', unsafe_allow_html=True)
def run_app(): st.set_page_config('Pygiator', layout='centered') appStyle() # sidebar file_1 = st.sidebar.file_uploader('First File', key='file1in', type=['py']) file_2 = st.sidebar.file_uploader('Second File', key='file2in', type=['py']) # main page st.title('Pygiator - Plagiarism Finder for Python Source Code') c1, c2 = computeCode(file_1, file_2) if 0 not in [c1, c2]: winnowing(c1, c2) blankLine() printResult(c1, c2) else: # home page is printed if no files selected a = st.beta_container() logo_file = open('./misc/logo.svg') logo_data = logo_file.read() st.write(renderSvg(logo_data), unsafe_allow_html=True) logo_file.close() a.write('Enter the source file paths in the sidebar.')
def test_nested_with(self): with st.beta_container(): with st.beta_container(): st.markdown("Level 2 with") msg = self.get_message_from_queue() self.assertEqual( make_delta_path(RootContainer.MAIN, (0, 0), 0), msg.metadata.delta_path, ) st.markdown("Level 1 with") msg = self.get_message_from_queue() self.assertEqual( make_delta_path(RootContainer.MAIN, (0, ), 1), msg.metadata.delta_path, )
def page(): target_to_function = { "イベント(東北大学ホームページ)": "liTagsOfEventsOfHomePageFromToday", "ニュース": "htmlOfNewsOfHomePage", "在学生向け情報": "htmlOfScienceFacultyForCurrentStudents", "セミナー": "liTagsOfSeminarsOfMathFacultyFromToday", "集中講義": "liTagsOfIntensiveLectureOfMathFaclutyFromToday", "談話会": "liTagsOfColloquiumOfMathFaclutyFromToday", "研究集会": "liTagsOfMeetingOfMathFaclutyFromToday", "お知らせ(物理学科・物理学専攻)": "liTagsOfNoticeOfPhysicsFaclutyFromToday", "イベント(物理学科・物理学専攻)": "liTagsOfEventsOfPhysicsFaclutyFromToday", "イベント紹介(学部1&2年生)": "liTagsOfEventsOfPhysicsFaclutyForFirstSecond", "イベント紹介(学部3&4年生)": "liTagsOfEventsOfPhysicsFaclutyForThirdFourth", "イベント紹介(大学院生)": "liTagsOfEventsOfPhysicsFaclutyForGraduates", "イベント紹介(大学院受験生)": "liTagsOfEventsOfPhysicsFaclutyForGraduateCandidates", "重要なお知らせ": "liTagsOfImportantsOfChemistryFacluty", "入試関連情報": "liTagsOfAdmissionsOfChemistryFacluty", "インフォメーション": "ddTagsOfExternalInformationOfGeoscienceFacluty", "お知らせ(ニュース)": "liTagsOfNewsOfEarthPhysicsMajor", "お知らせ(専攻内情報)": "liTagsOfInternalInfoOfEarthPhysicsMajor", "大学院入試説明会": "articleTagsOfEntranceBriefingOfEarthPhysicsMajor", "大学院入試案内": "articleTagsOfExamInfoOfEarthPhysicsMajor", "お知らせ(天文学教室)": "divTagsOfInfoOfAstronomicalMajor", "入試情報": "entryTagsOfExamInfoOfLifeScienceMajor", "最新情報": "entryTagsOfInternalInfoOfLifeScienceMajor", "教務課からのお知らせ": "zengaku_kyomuka_li_tags", "授業案内": "zengaku_guide_html", } title_container = st.beta_container() title_container.title("対象サイトの選択/サイト内容の取得") is_button_pushed = st.button("サイト内容の取得を実行") flash_info_container = st.beta_container() select() user_table = UserTable(table_index=0) if is_button_pushed: for (site_name, is_enabled) in st.session_state["sites"].items(): if is_enabled: st.session_state["scraping_result_dict"][site_name] = user_table.query(target_to_function[site_name]) with flash_info_container: if st.session_state["scraping_result_dict"] == {}: st.warning("対象サイトを選択してください") else: st.info("左側のメニューから取得結果一覧を押してください")
def app(): # Read Data checkins = pd.read_csv('ese-l1-exam-checkins-dump.csv') checkins['date'] = pd.to_datetime( checkins['date'], format='%Y-%m-%d') # Convert str to DateType st.title('Welcome to the Employee Check-In Dashboard!') st.markdown(''' This application aims to assist Human Resource personnel with easy to understand information! Please use the Navigation bar on the left to get started with the application. ''') # Initial insights from the dataset stats = st.beta_container() with stats: st.title("Currently you have tracked...") dates, projects, managers, users = st.beta_columns(4) with dates: st.title(str(checkins['date'].nunique())) st.markdown('number of days logged.') with projects: st.title(str(checkins['project_id'].nunique())) st.markdown('unique projects') with managers: st.title(str(checkins['manager_id'].nunique())) st.markdown('number of managers') with users: st.title(str(checkins['user_id'].nunique())) st.markdown('unique employees') today = st.beta_container() today_date = checkins['date'].iloc[-1] with today: st.title("Our last log was on " + str(today_date)) st.markdown('Today your company has tracked...') today_data = checkins.loc[checkins['date'] == today_date] emp_t, proj_t, hours_t = st.beta_columns(3) with emp_t: st.title(str(today_data['user_id'].nunique())) st.markdown('Employees Checked-in') with proj_t: st.title(str(today_data['project_id'].nunique())) st.markdown('Projects Logged') with hours_t: st.title(str(("%.1f" % today_data['hours'].sum()))) st.markdown('Total Hours Worked Today')
def bernoulli_ttest_ui(tech_note_path): '''The Two-sample Student's t-test - Bernoulli variables section. ''' # Render the header. with st.beta_container(): st.title('Two-sample Student\'s t-test') st.header('Bernoulli variables') st.info('If the outcome variable is binary, for example, if you are testing for Click-through Rates (CTR) or customer retention rates, we suggest using a Chi-squared test. ') # Render input widgets with st.beta_container(): col1, col2 = st.beta_columns([1, 1]) with col1: st.subheader('Group A') visitors_1 = st.number_input( 'Visitors A: ', min_value=10, value=80000) conversions_1 = st.number_input( 'Conversions A: ', min_value=0, value=1600) conf_level = st.select_slider('Confidence level: ', ('0.90', '0.95', '0.99')) with col2: st.subheader('Group B') visitors_2 = st.number_input( 'Visitors B: ', min_value=10, value=80000) conversions_2 = st.number_input( 'Conversions B: ', min_value=0, value=1696) hypo_type = st.radio('Hypothesis type: ', ('One-sided', 'Two-sided')) # Assert visitors>=conversions try: assert visitors_1 >= conversions_1 except: raise ValueError('Visitors must be more the the converted. ') # Calculate statistics mu_1, mu_2, sigma_1, sigma_2 = bernoulli_stats( visitors_1, visitors_2, conversions_1, conversions_2) tstat, p_value, tstat_denom, pooled_sd, effect_size = scipy_ttest_ind_from_stats( mu_1, mu_2, sigma_1, sigma_2, visitors_1, visitors_2) observed_power = sm_tt_ind_solve_power(effect_size=effect_size, n1=visitors_1, n2=visitors_2, alpha=1-float(conf_level), power=None, hypo_type=hypo_type, if_plot=False) # Render the results ttest_plot(mu_1, mu_2, sigma_1, sigma_2, conf_level, tstat, p_value, tstat_denom, hypo_type, observed_power) # Render technical notes with st.beta_expander(label='Technical notes'): with open(tech_note_path, 'r') as tech_note: st.markdown(tech_note.read())