def assistant(command): "if statements for executing commands" if 'computer' in command: Listening_Response = [ 'Yes Sir', 'How may I help', 'At your service', 'You called sir', 'Hello' ] engine.say(random.choice(Listening_Response)) engine.runAndWait() if 'close browser' in command: close_browser() if 'google' in command: google() if 'thank you' in command: thank_you() if 'weather' in command: weather() if 'play music' in command: engine.say('where would you like me to play') engine.runAndWait() choice = myCommand() if 'youtube' in choice: youtube() elif 'spotify' in choice: spotify()
def mode(option): if option.lower() == "mathematics": print( "Welcome to Mathematics module of infinity\n" "this module can assist you in various mathematical functions like addition, subtraction, multiplication and many more on any number that you provide\n" "Kindly follow the below mentioned instructions for the required result\n" ) m.mathematics() elif option.lower() == "weather": print( "Welcome to weather module of infinity\n" "This module can assist you in informing about the current as well as the predict the future weather conditions\n" "Kindly follow the below mentioned instructions for the accurate weather conditions\n" ) w.weather() elif option.lower() == "statistics": print( "Welcome to Statistics module of Inifinity\n" "This module can provide you various information like Population, location, Capital City, Currency, Area, etc of all the countries in the globe\n" "Kindly follow the below instructions for getting the precise information.\n" ) c.countries() elif option.lower() == "exit": print("Thank you for using Inifinity Assistance System") else: print("Sorry, This is not a valid input")
def index(): return render_template("index.html", today=dtime(), data=weather()[0]['臺北市'], data1=weather()[1]['臺北市'], data2=weather()[2]['臺北市'], usd=tb()[0], jpy=tb()[7], aud=tb()[3], eur=tb()[14], news=news())
def main(): if period == 'AM': greeting(now); weather(weather_result); #current_events(now); coffee(now); #bbc_podcast(now); else: greeting(now); weather(weather_result); #current_events(now); dinner(now);
def water(): resetGPIO(relay1) print(colors.BLUE + "Watering Plants...") GPIO.output(relay1,GPIO.HIGH) sleep(30) # Disable Relay resetGPIO(relay1) print (colors.GREEN + "Done Watering Plants") #Get Weather information weather() # get weather information notify() # send telegram notification via IFTTT # clean up GPIO GPIO.cleanup() # Exit script exit()
def travel_plan(): time_start = time.time() # docker pred_place_dict = load_transfer_model( model_path=r"/code/mode_iv3LeafFinetune_15.h5", pic_dir_path=upload_dir + "/static", pic_list=place_filenames) pred_food_dict = predict(pic_dir_path=upload_dir + "/static", pic_list=food_filenames) # print('pred_place_dict ', pred_place_dict) # print('pred_food_dict ', pred_food_dict) ''' 照片分析完成 進 類別推薦 模型 程式撰寫區 ''' place_recommend_list = place_recommend(pred_place_dict) food_recommend_list = food_recommend(pred_food_dict) print('place_recommend_list ', place_recommend_list) print('food_recommend_list ', food_recommend_list) ''' 得到 地點 進 路線規劃 模型 程式撰寫區 ''' start_place_list = ['台北車站'] final_list, travel_suggest = route_plan( start_place_list + place_recommend_list + food_recommend_list, True) print('行程規劃', final_list) print('旅遊建議', travel_suggest) weather_dict = weather() print('天氣', weather_dict) cost_time = time.time() - time_start print('計算花了', cost_time, '秒') return render_template('index.html')
def on_enter_weather_city_state(self, event): print("I'm entering weather city state") sender_id = event['sender']['id'] city_url = weather(event['message']['text']) send_text_message(sender_id, "以下是中央氣象局:" + event['message']['text']) send_text_message(sender_id, city_url) self.go_back(event)
def process(msg): if msg.startswith('#') or msg.startswith('#'): pass elif msg == u'新生指南': return freshman.get_guidance(), "news" elif msg == u'新闻' or msg == u'体育' or msg == u'体育新闻': return news.getNews(msg), "news" elif isinstance(msg, type('string')): msg = msg.lower() msg = msg.strip() if msg == 'bbc world' or msg == 'bbc china' or msg == 'bbc' or msg == 'nba': return news.getNews(msg), "news" else: return translate.translate(msg), "text" #elif msg == u'新闻': # return news_info, "text" elif msg == u'校车' or msg == u'明天校车': return xiaoche.get_timetable(msg), "text" elif msg == u'摆渡车': return ferrybus.get_timetable(msg), "text" elif msg == u'环一' or msg == u'环1': return huanyi.get_timetable(), "text" elif msg == u'天气': return weather.weather(), "text" elif msg == u'空气': return weather.get_airquality(), "text" elif re.match(u"发状态", msg): if msg[3:]: return renren.renren_status(msg[3:]), "text" else: return u"请输入状态内容", "text" else: return u"无法处理请求,请查看使用说明\n" + help_info + report_info, "text"
def early_response(self): # 构建请求头部 webhook = self.signed_webhook() # 构建请求头部 header = {"Content-Type": "application/json", "Charset": "UTF-8"} weathers = weather() # 构建请求数据 message = { "msgtype": "markdown", "markdown": { "title": "今日最早开门", "text": "### 今日最早开门" + "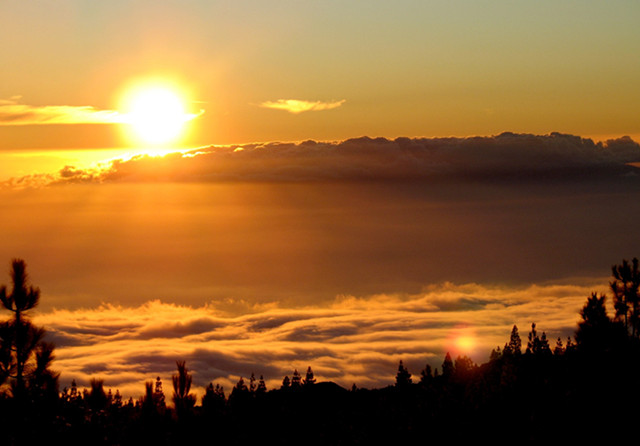\n\n" + weathers }, "at": { "atMobiles": ["15542443091"], "isAtAll": False } } # 对请求的数据进行json封装 message_json = json.dumps(message) # 发送请求 info = requests.post(url=webhook, data=message_json, headers=header)
def early_response(): webhook = "https://oapi.dingtalk.com/robot/send?access_token=94016c3f7bfc510156ea45fb88510d7721e9ce850e1f49d8fe1d59e702e54044" webhook = signed_webhook(webhook) # 构建请求头部 header = {"Content-Type": "application/json", "Charset": "UTF-8"} weathers = weather() # 构建请求数据 message = { "msgtype": "markdown", "markdown": { "title": "今日最早开门", "text": "### 今日最早开门@15542443091\n" + "> 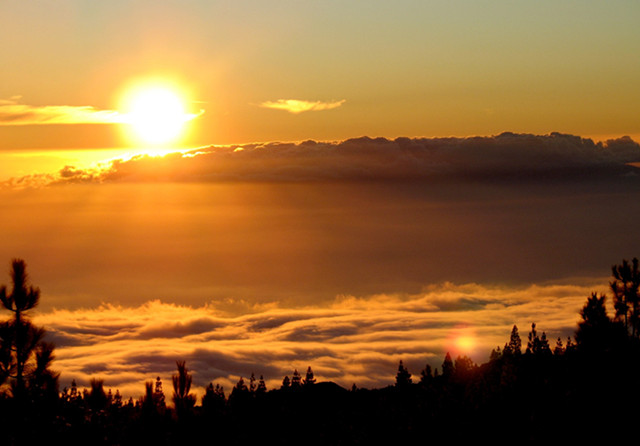\n\n" + weathers }, "at": { "atMobiles": ["15542443091"], "isAtAll": False } } # 对请求的数据进行json封装 message_json = json.dumps(message) # 发送请求 info = requests.post(url=webhook, data=message_json, headers=header) # 打印返回的结果 print(info.text)
def Weather(): req = request.get_json() location = req["action"]["detailParams"]["sys_location"]["value"] date = req["action"]["detailParams"]["sys_date"]["origin"] return json_ify(wt.weather(location, date))
def __init__(self): self.basePossibleCustomers = None self.adjustedPossibleCustomers = None self.weather = weather.weather() self.listCustomers = [] self.getBasePossibleCustomers() self.getAdjustedPossibleCustomers() self.createListCustomers()
def reply(msg): #msg = 'beijing' wt = weather() if wt.city_code.has_key(msg): result = wt.get_weather(msg) else: result = "不支持的地区\n请输入要查询的地区拼音,例如'北京'请输入'beijing'" return result
def get_weather_info(in_weather): try: in_weather_info = re.search(r'^Weather\sis\s(\w+)', in_weather.strip()).group(1) out_weather_info = weather.weather(in_weather_info) weather_crater_percent, weather_vehicle_list = out_weather_info.get_weather_details() except: pass return weather_crater_percent, weather_vehicle_list
def __init__ (self): self.basePossibleCustomers=None self.adjustedPossibleCustomers=None self.weather=weather.weather() self.listCustomers=[] self.getBasePossibleCustomers() self.getAdjustedPossibleCustomers() self.createListCustomers()
def get(self): try: today = time.strftime('%Y%m%d') citycode = igaelib.GetGaeCfg('weather', 'city') mail.send_mail(sender='*****@*****.**', to='*****@*****.**', subject='%s' % '天气', body=weather(citycode)) except Exception as e: logging.error(format_exc()) raise e
def get_image(): city = request.args.get('city') state = request.args.get('state') reportHours = int(request.args.get('reportHours')) filePath = weather(state=state, city=city, reportHours=reportHours).createGraph() return send_file(filePath, mimetype='image/png')
def weatheroop(): print( "---------------------------------------天气情况---------------------------------------" ) wea = weather.weather() print(wea.future(0, 1, 2, 3)) print( "-------------------------------------------------------------------------------------" )
def travel_plan(): time_start = time.time() # docker pred_place_dict = load_transfer_model( model_path=r"/code/mode_iv3LeafFinetune_15.h5", pic_dir_path=upload_dir + "/static", pic_list=place_filenames) pred_food_dict = predict(pic_dir_path=upload_dir + "/static", pic_list=food_filenames) print('pred_place_dict ', pred_place_dict) print('pred_food_dict ', pred_food_dict) ''' 照片分析完成 進 類別推薦 模型 程式撰寫區 ''' place_recommend_list = place_recommend(pred_place_dict) food_recommend_list = food_recommend(pred_food_dict) print('place_recommend_list ', place_recommend_list) print('food_recommend_list ', food_recommend_list) ''' 得到 地點 進 路線規劃 模型 程式撰寫區 ''' start_place_list = [usergo] final_list, travel_suggest = route_plan( place_recommend_list=start_place_list + place_recommend_list, food_recommend_list=food_recommend_list) basic_data = {'出發日期': userdate, '旅遊天數': '1天'} # final_list = [{'出發': " '台北車站'", '到達': " 'Papa Vito'", '距離': " '11.1'", '交通時間': Decimal('0.4'), # '停留時間': Decimal('0.6'), '交通+停留時間': Decimal('1.0'), '累積時間': Decimal('1.0'), # '離開時間': datetime.datetime(2020, 5, 15, 9, 0)}, # {'出發': " 'Papa Vito'", '到達': " '淡水港燈塔'", '距離': " '16.6", '交通時間': Decimal('0.7'), # '停留時間': Decimal('0.0'), '交通+停留時間': Decimal('0.7'), '累積時間': Decimal('1.1'), # '離開時間': datetime.datetime(2020, 5, 15, 9, 42)}] # travel_suggest = '行程有點少, 請考慮增加景點' print(final_list) print(travel_suggest) weather_dict = weather() print(weather_dict) cost_time = time.time() - time_start print(cost_time, '秒') # brief = {'date':'2020/5/22', # 'days':1 # } #return render_template('recommand.html', final_list=final_list, travel_suggest=travel_suggest, # weather_dict=weather_dict, start_place_list=start_place_list, basic_data=basic_data) return render_template('recommend.html', final_list=final_list, travel_suggest=travel_suggest, weather_dict=weather_dict, start_place_list=start_place_list, basic_data=basic_data)
def textAction(xml): #文本信息处理 content=xml.find("Content").text#获得用户所输入的内容 if(u'查公交' in content): return buslineRes(content) if(u'讲笑话' in content): return joke() if(u'查天气' in content): return weather(content) youdaoRes = youdao(content) return youdaoRes
def textAction(xml): #文本信息处理 content = xml.find("Content").text #获得用户所输入的内容 if (u'查公交' in content): return buslineRes(content) if (u'讲笑话' in content): return joke() if (u'查天气' in content): return weather(content) youdaoRes = youdao(content) return youdaoRes
def processRequest(req): # Parsing the POST request body into a dictionary for easy access. req_dict = json.loads(request.data) entity_type = "" entity_value = "" speech = "" # Accessing the fields on the POST request boduy of API.ai invocation of the webhook intent = req_dict["result"]["metadata"]["intentName"] entity_key_val = req_dict["result"]["parameters"] for key in entity_key_val: entity_value = entity_key_val[key] entity_type = key # constructing the resposne string based on intent and the entity. if intent == "shopping - custom": my_input = (req.get("result").get("resolvedQuery")).lower() search_results = webscrap(my_input) res = makeWebhookResult(search_results) elif intent=="showwallet": bal=10 res= makeWebhookResult(bal) elif intent == "Default Fallback Intent": my_input = (req.get("result").get("resolvedQuery")).lower() if ("weather" in my_input) or ('tell me about weather condition' in my_input) or ( 'tell me about weather' in my_input) or ('whats the climate' in my_input): x = weather() speech = "" + x + "" res = makeWebhookResult(speech) elif ("news" in my_input) or ("top headlines" in my_input) or ("headlines" in my_input): x = news() speech = "" + x + "" res = makeWebhookResult(speech) else: try: app_id = "R2LUUJ-QTHXHRHLHK" client = wolframalpha.Client(app_id) r = client.query(my_input) answer = next(r.results).text speech = "" + answer + "" res = makeWebhookResult(speech) except: my_input = my_input.split(' ') my_input = " ".join(my_input[2:]) answer = wikipedia.summary(my_input, sentences=2) speech = "" + answer + "" res = makeWebhookResult(speech) else: speech = "no input" res = makeWebhookResult(speech) return res
def formResponse(self, receivedStr, clientAddress, client): ### Charlie Barry and Dominic Egginton keysFound, extraData = self.searchJSON(receivedStr) # IF ONLY PYTHON HAD SWITCH STATEMENTS <- :) :) if 'curse' in keysFound: ### Tom has done the curse code return "Please watch your language." elif 'currency' in keysFound: if extraData.get('currency'): currencyInfo = extraData.get('currency') currencyData = currency() answer = currencyData.convert(currencyInfo['cFrom'],currencyInfo['cTo'],currencyInfo['amount']) if answer != "": return "{} {} in {} is {}".format(currencyInfo['amount'],currencyInfo['cFrom'].upper(),currencyInfo['cTo'].upper(), answer) return "Sorry, I can't convert that." elif 'weather' in keysFound: clientIpData = self.getIpData(clientAddress) weatherData = weather() return weatherData.weatherResponse(keysFound, clientIpData, extraData) elif 'cinema' in keysFound: ### Charlie Barry and Mitko Donchev ### I apologise for the laziness of this code, need to patch it up sometime :( - Charlie from cinema import searchCinema, showTime, fetchCinema #imports all the functions from cinema.py clientIpData = self.getIpData(clientAddress) location = {'latitude': clientIpData['lat'], 'longitude': clientIpData['lon']}#gets the location of the client from their ip address aesObject = AESEncryption(self.key) client.sendall(aesObject.encrypt(fetchCinema(location) + "Select a cinema for more information (type 'back' to go back)"))#sends message to client while True: cinemaData = client.recv(4096)#interepts message sent from client cinemaStr = aesObject.decrypt(cinemaData).replace('!',"").replace('?',"")#decrypts message into plaintext if cinemaStr.lower() != 'back': IDC = searchCinema(location) extracinemainfo = showTime(IDC,cinemaStr) client.sendall(aesObject.encrypt(extracinemainfo))#gets extra info for cinema and sends it to client if not(extracinemainfo == 'Wrong cinema! Please try again by chosing the right number!'):#if user inputted a legitimate answer cinemaData = client.recv(4096)#recieve any string break#returns to main server code elif cinemaStr.lower() == 'back':#if user says 'back' then returns to main server code break return "Exitted Cinema Mode Successfully"#returns to main server code elif 'ipinfo' in keysFound: ipData = self.getIpData(clientAddress) return "Your IP is {}, provided by {}.".format(ipData['query'], ipData['isp']) elif 'celery' in keysFound: return "/w/https://youtu.be/MHWBEK8w_YY" else: return "Sorry, I don't understand what you are talking about."
async def on_message(message): if message.author == client.user: return msg = message.content if message.content.startswith("!hello"): await message.channel.send("Hello!") #send message to channel if message.content.startswith('!inspire'): await message.channel.send(getQuote()) if db["responding"]: options = starterEncouragements if "encouragements" in db.keys(): options = options + db["encouragements"] if any(word in msg for word in sadWords): await message.channel.send(random.choice(options)) if msg.startswith("!new"): encouragingMessage = msg.split("!new ", 1)[1] updateEncouragements(encouragingMessage) await message.channel.send("New encouraging message added.") if msg.startswith("!del"): encouragements = [] if "encouragements" in db.keys(): index = int(msg.split("!del", 1)[1]) deleteEncouragment(index) encouragements = db["encouragements"] await message.channel.send(encouragements) if msg.startswith("!list"): encouragements = [] if "encouragements" in db.keys(): encouragements = db["encouragements"] await message.channel.send(encouragements) if msg.startswith("!responding"): value = msg.split("!responding ", 1)[1] if value.lower() == "true": db["responding"] = True await message.channel.send("Responding is on.") else: db["responding"] = False await message.channel.send("Responding is off.") if msg.startswith("!weather"): cityName = msg.split("!weather ", 1)[1] await message.channel.send(weather(cityName))
def callfunction(): while 1: print("welcome to road traffic injury survey: ") print("===============================") print("-------select the survey-------") print(" 1. URBAN or RURAL area ") print(" 2. Days_of_week by multiline graph ") print(" 3. Ratio of Gender_of_Driver and Accident Severity Using pie Charts") print(" 4. Age Band and Gender_of_Driver using Multibar plot ") print(" 5. Severity and Gender_of_Driver using Multibar Plot ") print(" 6. Light Conditions using Stacked graph ") print(" 7. Weather Conditions using Stacked graph ") print(" 8. Number of Vehicles involved per accident using Boxplot ") print(" 9. Speed Limit of Vehicles During Accident with Probability ") print(" 10. Exit") print("................................") choice=input("please enter the survey choice") if choice is 1: pl.urban_rural() elif choice is 2: ml.multiline() elif choice is 3: pi.pie() elif choice is 4: ml2.multibar() elif choice is 5: ml3.multibar3() elif choice is 6: st.light() elif choice is 7: we.weather() elif choice is 8: bx.boxplt() elif choice is 9: sl.hist() elif choice is 10: sys.exit()
def brain(name, speech_text,city_name,city_code): def check_message(check): words_of_message = speech_text.split() if set(check).issubset(set(words_of_message)): return True else: return False if check_message(['who','are', 'you']): general_conversations.who_are_you() elif check_message(['how', 'i', 'look']) or check_message(['how', 'am', 'i']): general_conversations.how_am_i() elif check_message(['tell', 'joke']): general_conversations.tell_joke() elif check_message(['who', 'am', 'i']): general_conversations.who_am_i(name) elif check_message(['where', 'born']): general_conversations.where_born() elif check_message(['how', 'are', 'you']): general_conversations.how_are_you() elif check_message(['time']): tell_time.what_is_time() elif check_message(['how', 'weather']) or check_message(['hows', 'weather']): weather.weather(city_name, city_code) elif check_message(['define']): define_subject.define_subject(speech_text) elif check_message([‘business’, ‘news’]): business_news_reader.news_reader()
def init(data): #Lobby init data.me = User("Me") data.nameChange = "" data.otherStrangers = dict() data.isLobby = True data.isPlaying = False #scrolling data.scrollMargin = 30 data.scrollX = 0 data.scrollY = 0 #platforms (will be randomly generated) data.testPlatform = Platform(data.width - (2 * data.scrollMargin), data.height - 30, 40) data.bottomPlatform = Platform(0, data.height - 5, data.width * 3) data.p1 = Platform(data.width - (3 * data.scrollMargin), data.height - 60, 40) data.p2 = Platform(data.width - (4 * data.scrollMargin), data.height - 80, 40) data.p3 = Platform(data.width + (3 * data.scrollMargin), data.height - 60, 40) data.p4 = Platform(data.width + (4 * data.scrollMargin), data.height - 80, 40) data.platforms = [ data.testPlatform, data.bottomPlatform, data.p1, data.p2, data.p3, data.p4 ] #Runner init data.player = Player(data.scrollMargin, data.height - 10) data.isJumping = False data.isAcceleratingRight = False data.isAcceleratingLeft = False data.isFalling = False data.canMove = True data.currentPlatform = data.bottomPlatform data.stunCount = 0 #God init data.gameWeather = weather(0) data.canRain = True data.canLightning = True data.willLightning = False data.isLightning = False data.lightning = None data.lightningEnd = data.height data.lightningCounter = 0
def main(): print("1. Time") print("2. Ip") print("3. Weather \n") choice = input("Choice : ") print() if choice == "1": print("1. Time") print("2. Calendar of the month") print("3. Calendar of the year") print("4. Epoch \n") scdChoice = input("Choice : ") print() if scdChoice == "1": temps.temps() elif scdChoice == "2": temps.calendarOfTheMonth() elif scdChoice == "3": temps.calendarOfTheYear() elif scdChoice == "4": temps.epoch() else: print("Error !") elif choice == "2": ip.ipInfo() elif choice == "3": weather.weather() else: print("Error !")
def init(data): # load data.xyz as appropriate data.gameWeather = weather(0) data.scrollMargin = 30 data.player = Player(data.width - data.scrollMargin, data.height - 10) data.testPlatform = Platform(data.width - (2 * data.scrollMargin), data.height - 30, 40) data.bottomPlatform = Platform(0, data.height - 5, data.width) data.platforms = [data.testPlatform, data.bottomPlatform] data.canRain = True data.canLightning = True data.willLightning = False data.isLightning = False data.lightning = None data.lightningCounter = 0 data.timeCount = 0
def waterCycle(t): global gWateringStatus now = time.time() # check soil moisture if ( GPIO.input(moisture_pin) == GPIO.LOW ): print("Soil above moisture threshhold, skip watering") gWateringStatus = False # wait a minute before return then = time.time() + 60 while ( time.time() < then ): updateSched() sleep(1) return message = "watering, gpio on at " + str(datetime.fromtimestamp(now)) print(message) mqttc.connect(broker,1883) mqttc.publish(queue,message) mqttc.disconnect() GPIO.output(valve_pin, GPIO.LOW) gWateringStatus = True # get weather forecast jfc = weather.weather() # adjust duration down by prediction of rain duration = t * (1. - jfc["hourly"]["data"][0]["precipProbability"]) print("watering for " + str(duration) + " seconds") then = time.time() + duration while ( (gWateringStatus == True) and (time.time() < then ) ): # in case watering is canceled by the STOP button updateSched() sleep(1) now = time.time() message = "Done watering, gpio off " + str(datetime.fromtimestamp(now)) print(message) mqttc.connect(broker,1883) mqttc.publish(queue,message) mqttc.disconnect() GPIO.output(valve_pin, GPIO.HIGH) if ( gWateringStatus == "Canceled" ): # if canceled, wait a minute before resuming normal so another cycle doesn't kick off then = time.time() + 60 while ( time.time() < then ): updateSched() sleep(1) gWateringStatus = False now = time.time() # for watering less than a minute, so no double cycle while ( time.time() < now + (60 - duration)): updateSched() sleep(1)
def main(): # appid = os.environ.get("OPENWEATHERMAP_APPID") weather = Weather.weather(300) effects = animations.animations() effects.setcolor(animations.black) radar = Radar.RCWL_0516() radar.begin(15) timer = time.time() # start with the timer off while True: # if the radar is detecting a person, set the timer for 5 minutes if radar.isOn(): timer = time.time() # reset the timer print("Radar ON") else: pass # if the timer has expired, sleep and wait for the radar to come on if time.time() - timer > (60 * 5): #5 minutes if effects.np[0] != animations.black: effects.setcolor(animations.black) time.sleep_ms(50) continue # retreive the current weather forecast weather.getWeather(config.OPENWEATHERMAP_APPID, config.LOCATION) ani = None if weather.weather == "Thunderstorm": ani = effects.lightning elif weather.weather in ["Rain", "Drizzle", "Squall", "Mist"]: ani = effects.flicker cloud = animations.white if weather.current < 40: cloud = animations.blue elif weather.current < 70: cloud = animations.green elif weather.current < 80: cloud = animations.gold elif weather.current < 90: cloud = (90, 0, 0) else: cloud = animations.red effects.setcolor(cloud) if ani: ani()
def view_weather(self): location = self.input_location.send_location() self.location_logo.setText(location) weather_infos = weather.weather(location) for i in range(0, len(weather_infos)): weather_infos[i] = str(weather_infos[i]) if i == 0: weather_infos[i] = weather_infos[i] + ' ℃' if i == 1: weather_infos[i] = weather_infos[i] + ' %' if i == 2: weather_infos[i] = weather_infos[i] + ' m/s' self.view_list[i].setText(weather_infos[i])
def __init__(self): super(MyPyQt,self).__init__() self.setupUi(self) self.setWindowTitle("Weather Query")#改变窗口名 self.setWindowIcon(QIcon('title.jpg'))#改变图标 self.__weather = weather()#私有变量 天气 self.__airqu=airquality()#私有变量 空气质量 self.setStyleSheet('''QLineEdit{color:white;background:transparent;border:1px solid gray; border-radius:10px;padding:2px 4px;font-weight:1000;} QPushButton{border-radius:10px;color:white;background:transparent;border:1px solid gray; padding:2px 4px;font-weight:1000;font-weight:500;font-family: "Helvetica Neue", Helvetica, Arial, sans-serif;} QLabel{color:white;border-radius:10px;padding:2px 4px; font-size:18px; font-weight:500;font-family: "Helvetica Neue", Helvetica, Arial, sans-serif;} QTextEdit{color:white;background:transparent;border:1px solid gray; font-weight:1000; border-radius:10px;padding:2px 4px;} ''')#用QSS美化ui self.setWindowOpacity(0.95) # 设置窗口透明度 window_pale = QtGui.QPalette() window_pale.setBrush(self.backgroundRole(), QtGui.QBrush(QtGui.QPixmap("E:/study/spring.2019/python/lab/lab1/background1.png"))) self.setPalette(window_pale)#设置背景图 #以下对特殊控件美化 self.label_2.setStyleSheet( """QLabel{border:None;color:white;font-size:25px;font-weight:500; font-family: "Helvetica Neue", Helvetica, Arial, sans-serif;}""") self.label_3.setStyleSheet( """QLabel{border:None;color:white;font-size:21px;font-weight:500; font-family: "Helvetica Neue", Helvetica, Arial, sans-serif;}""") self.label.setStyleSheet( """QLabel{border:None;color:white;font-size:25px;font-weight:500; font-family: "Helvetica Neue", Helvetica, Arial, sans-serif;}""") self.label_8.setStyleSheet( """QLabel{border:None;color:white;font-size:25px;font-weight:500; font-family: "Helvetica Neue", Helvetica, Arial, sans-serif;}""") self.label_19.setStyleSheet( """QLabel{border:None;color:white;font-size:25px;font-weight:500; font-family: "Helvetica Neue", Helvetica, Arial, sans-serif;}""") self.label_13.setStyleSheet( """QLabel{border:None;color:white;font-size:25px;font-weight:500; font-family: "Helvetica Neue", Helvetica, Arial, sans-serif;}""") self.label_22.setStyleSheet( """QLabel{border:None;color:white;font-size:25px;font-weight:500; font-family: "Helvetica Neue", Helvetica, Arial, sans-serif;}""")
def index(request): w = weather.weather() status = w.get_status() # 구름 상태를 가져온다. ex) Clear icons = w.get_weather_icon_url() temp = round(w.get_temperature(unit='celsius')['temp'], 1) # 온도를 가져온다. round로 소수점 1자리 까지만 now = time.localtime() s = "%04d-%02d-%02d %02d:%02d:%02d" % (now.tm_year, now.tm_mon, now.tm_mday, now.tm_hour, now.tm_min, now.tm_sec) # 현재 시각을 s 에 저장 ex) 2019-10-24 14:03:59 return render(request, 'index.html', { 'status': status, 'icons': icons, 'temp': temp, 'time': s, })
def processRequest(req): print("Request:") print(json.dumps(req, indent=4)) if req.get("result").get("action") == "yahooWeatherForecast": res = weather.weather(req) elif req.get("result").get("action") == "commodityprice": data = req res = price(data) # elif req.get("result").get("action") == "sayfeatures": # res=feature() # elif req.get("result").get("action") == "getChemicalSymbol": # data = req # res = makeWebhookResultForGetChemicalSymbol(data) elif req.get("result").get("action") == "news": res = news.news() else: return {} return res
def reply(msg): #msg = 'beijing' wt = weather() pymsg = pinyin.get(msg) if wt.city_code.has_key(pymsg): result = wt.get_weather(pymsg) else: result = "你在说什么?我只支持天气查询哦.\n\n试着输入要查询的地区吧,比如'北京'" joke_num = False for key in joke: reg = re.search(key, pymsg) if reg: result = joke[reg.group(0)] joke_num = True if not joke_num: robot = turing() result = robot.reply(msg) return result
def implement(self, content, user='******'): try: content = content.strip() if content == '天气' or content.lower() == 'weather': reply = ['markdown', 'Weather:', weather(True)] elif content == '开门' or content.lower() == 'open' or content.lower( ) == 'open the door': # 小米开关开门 maid1_commander = Maid1_Commander() report = maid1_commander.command1() reply = report self.earliest() elif content == '门的状况' or content.lower() == 'door': # 小米开关的状况 maid1_commander = Maid1_Commander() report = maid1_commander.command2() reply = f"Power:{report.power} | Temperature:{report.temperature}" elif content[:2] == '翻译': # 翻译 lang, content = Tolang(content[2:]) reply = BaiduTranslate(content, lang) elif content == '语言列表' or content == 'language dict': reply = print_language() # elif content == '你好' or content == '嗨' or content.lower() == 'hello' or content.lower() == 'hi': # # 夸奖 # r = random.randint(0,2) # reply_list=['Hello.', "Hi.", "Glad to serve you."] # reply = reply_list[r] # elif content == '干得漂亮' or content == '干得不错' or content == '做得不错' \ # or content == '做得漂亮' or content == '谢谢' or content == '谢谢你' or content == '你真帅' \ # or content.lower() == 'good job' or content.lower() == 'well done' or content.lower() == 'nice' \ # or content.lower() == 'thank you' or content.lower() == 'thanks': # # 夸奖 # r = random.randint(0,2) # reply_list=['You are welcome.', "It's my pleasure.", "You're welcome."] # reply = reply_list[r] else: # 聊天 code, reply = chatting(content, user, self.chatterID) print(f"Chat Code:{code}") return reply except: # 出错 return 'Something wrong with me.'
async def on_message(message): # for debugging, we can see what the user said here print(f"{message.author}, {message.content}") # Enable rudimentary commands to display the API calls. if str(message.content).startswith('-'): # Check the news via an RSS parser if str(message.content).startswith('-news'): news = royaa_news() await message.channel.send(embed=news) # Check the weather via JSON, requires a city with the message, # e.g., -weather frankfurt if str(message.content).startswith('-weather'): weather_response = weather(message.content) await message.channel.send(embed=weather_response) # Check the fuel prices in Jordan by webscraping if str(message.content).startswith('-fuel'): fuel_response = fuel(message.content) await message.channel.send(embed=fuel_response) # Check the corona cases, requires a country, e.g., -corona sweden if str(message.content).startswith('-corona'): corona_response = corona(message.content) await message.channel.send(embed=corona_response) # Make Rasa calls by writing a message that starts with '!' # To run rasa, do `python .\rasa_folder\run_rasa.py` # with venv enabled, in terminal of course. if str(message.content).startswith('!'): checkMessage = query_rasa(message.content, True) # This if statement prevents rasa from talking with itsself. # It is not necessary since we have startswith('!') but # we might need it later on. if message.author == client.user: return # Send the message from rasa to discord await message.channel.send(checkMessage)
def waterCycle(t): global gWateringStatus now = time.time() # check soil moisture if ( gpio.input(moisture_pin) == gpio.LOW ): print("Soil above moisture threshhold, skip watering") gWateringStatus = False # wait a minute before return then = time.time() + 60 while ( time.time() < then ): updateSched() sleep(1) return print("watering, gpio on at " + str(now)) gpio.output(valve_pin, gpio.LOW) # post ON time to Azure Queue postdata = {'ON':''} postdata['ON'] = now print(postazq.postazq(postdata)) gWateringStatus = True # get weather forecast jfc = weather.weather() # adjust duration down by prediction of rain duration = t * (1. - jfc["hourly"]["data"][0]["precipProbability"]) print("watering for " + str(duration) + " seconds") then = time.time() + duration while ( (gWateringStatus == True) and (time.time() < then ) ): # in case watering is canceled by the STOP button updateSched() sleep(1) now = time.time() print("Done watering, gpio off " + str(now)) gpio.output(valve_pin, gpio.HIGH) # post OFF time to Azure Queue postdata = {'OFF':''} postdata['OFF'] = now print(postazq.postazq(postdata)) if ( gWateringStatus == "Canceled" ): # if canceled, wait a minute before resuming normal so another cycle doesn't kick off then = time.time() + 60 while ( time.time() < then ): updateSched() sleep(1) gWateringStatus = False
def process(msg): if msg == 'Hello2BizUser': return welcome_msg+help_info elif isinstance(msg, type('string')): return translate.translate(msg) elif msg == u'校车' or msg == u'明天校车': return xiaoche.get_timetable(msg) elif msg == u'环一' or msg == u'环1': return huanyi.get_timetable() elif msg == u'天气': return weather.weather() elif msg == u'空气': return weather.get_airquality() elif re.match(u"发状态", msg): if msg[3:]: return renren.renren_status(msg[3:]) else: return u"请输入状态内容" else: return u"无法处理请求,请查看使用说明" + help_info + report_info
def _proc_text(msg, resp): if msg in ('help' , 'Help'): raise MsgException(HELP) if msg in ('me' , 'Me'): raise MsgException(ABOUTME) if msg.startswith('bug'): log.info('BUG|%s' , msg) raise MsgException('感谢你的留意 ^_^ ') if msg.startswith('天气'): items = msg.split(None , 1) if len(items) == 2: resp['Content'] = weather.weather(items[1].strip()) else: raise MsgException('查询天气格式: 天气 + 空格 + 城市名称\n如:天气 饶平') elif msg.startswith('音乐'): items = msg.split(None , 1) if len(items) == 2: proc_music(items[1].strip(), resp) else: raise MsgException(MUSIC_MSG) else: resp['Content'] = xiaodou.chat(msg)
def get_daily_rainfall(id, data_start_date, data_end_date, local): day_rainfalls = [] filename = "tmp/rain_%s_%s_%s" % (id, data_start_date.strftime("%Y-%m-%d"), data_end_date.strftime("%Y-%m-%d")) if os.path.exists(filename): print("loaded rain") return pickle.load(open(filename, 'rb')) if local: iday = data_start_date while iday < data_end_date: inextday = iday + datetime.timedelta(days=1) day_rainfalls.append([iday, min(50, getRainInmm(id, iday.year, iday.month, iday.day))]) iday = inextday else: fmidata = [] places = ["kumpula,Helsinki", "kaisaniemi,Helsinki", "harmaja,Helsinki", "tapiola,Espoo", "nuuksio,Espoo"] for p in places: data = weather.weather(apikey, data_start_date, data_end_date, place=p) fmidata.append([[datetime.datetime.strptime(d, '%Y-%m-%d'), max(0, data[d]['rrday'])] for d in data]) fmidata = [[z[0][0], sum(p[1] for p in z) / len(places)] for z in zip(*fmidata)] day_rainfalls = fmidata day_rainfalls.sort() pickle.dump(day_rainfalls, open(filename, 'wb')) return day_rainfalls
def timemachine(self, pid): maxid = self.db.query("SELECT MAX(id) AS maxid FROM sweets")[0].maxid max_page = int(maxid+self.pageNum-1)//int(self.pageNum) heart_type = self.get_argument('heart_type', None) if heart_type: sql = "SELECT * FROM sweets WHERE `hearted` = %s ORDER BY time DESC LIMIT %s offset %s" % (int(heart_type), int(self.pageNum), int(self.pageNum)*int(pid)) else: sql = "SELECT * FROM sweets ORDER BY time DESC LIMIT %s offset %s" % (int(self.pageNum), int(self.pageNum)*int(pid)) sweets = self.db.query( sql ) for s in sweets: s.lover = self.get_user_by_id(s.lover_id) s.time = get_relativ_time(s.time) self.setCode(s) page = { 'max' : max_page, 'pid' : int(pid), 'next' : int(pid)+1, 'prev' : int(pid)-1, } h = self.getAnalytic() t = self.getTag() w = weather.weather(self) n = self.getNote() k = self.getFlickr() self.render("home.html", isMobile = self.isMobile(), heart=h, sweets=sweets, page=page, weather=w, note=n, kiss=k, #kiss=None, tag=t, currentTag=None, )
temp_data = [] prec_data = [] print date_list[:10] for i in date_list: if i[:10] in weather_dict: temp_data.append(float(weather_dict[i[:10]]['tmax'])) prec_data.append(float(weather_dict[i[:10]]['rrday'])) else: temp_data.append(np.nan) prec_data.append(np.nan) pump_codes = joblib.load('./pump_codes_list') weather_data = weather.weather(None, '2013-06-01', '2016-04-16') temp_data = np.array(temp_data, dtype=np.float32) prec_data = np.array(prec_data, dtype=np.float32) print temp_data print np.nanmin(temp_data), np.nanmax(temp_data), 'temp min/max' print np.nanmin(prec_data), np.nanmax(prec_data), 'prec min/max' print np.nanpercentile(prec_data, [2.5, 97.5]), 'prec percentile' print np.nanmedian(prec_data), 'precc median' print np.nanpercentile(temp_data, [2.5, 97.5]), 'temp percentile' print np.nanmedian(temp_data), 'temp median' def gen_plot(activity, stds, pump,comp_start, comp_end): comp_act = get_activity.get_data(pump, comp_start, comp_end) print comp_act, '******'
def wthr(content): return weather.weather(content)
def search(shelve_file): print("SEARCHER") again="yes" s=shelve.open("fortune_shelve") while (again=="yes"): found=0 #REMOVES AND/OR AndCount,OrCount = 0,0 found_set=set([]) Query_Set=set() query=input("query:") split_query=query.split() if (len(split_query) > 1): while ("and" in split_query): split_query.remove("and") AndCount=AndCount+1 while ("or" in split_query): split_query.remove("or") OrCount=OrCount+1 Query_Set=set(split_query) Query_list=list(Query_Set) total=len(Query_list) found=0 slot_set=set({}) dt1=datetime.now() if AndCount>=1 or total==1 or AndCount==0 and OrCount==0: print("\nAND SEARCH FOR\n\n ",Query_list) for query in Query_list: if query in s: #if query is in shelve found=found+1 if len(slot_set)>0: slot_set=set(s[query])&slot_set else: slot_set=set(s[query]) if found == len(Query_list): for spots in list(slot_set): print("FOUND AT : ", spots) x=[] if (OrCount>=1 and AndCount==0): print("\nOR SEARCH FOR ",Query_list) print("\n") for query in Query_list: if query in s: for paths in set(s[query]): x.append(paths) for spots in set(x): print("\nFOUND AT ", spots) dt2=datetime.now() weather.weather(Query_list) print("\n\nTotal Search time = ",dt2.microsecond-dt1.microsecond," microsecnds") again = input("do you want to play again? ")
def run(self): try: s = serial.Serial('/dev/ttyUSB0', 9600, timeout=10) except: self.log("Error connecting to Serial port") pass msg = "~128" msg += "~S0111111100002359" msg += "testing" #don't know why this works msg += "\r\r\r" s.write(msg) s.close() s = serial.Serial('/dev/ttyUSB0', 9600, timeout=10) msg = "~128" # set the time on startup msg += "~E" + time.strftime("%u1%y%m%d%H%M%S") self.log('-- time update --') self.log(msg) msg += "\r\r\r" s.write(msg) s.close() while True: now_playing = '' try: current_time = int(time.time()) if (GPIO.input(23) == False): print("Shutting down") os.system("poweroff") time.sleep(.5) if (GPIO.input(22) == False): print("22 Working") time.sleep(.5) if (GPIO.input(27) == False): son = sonos() son.playpause() time.sleep(.5) if (GPIO.input(18) == False): self.lastsonostime = 0 son = sonos() son.skip() time.sleep(.5) time.sleep(.1) # check sonos newsonos = False if(self.lastsonostime < (current_time - self.sonos_expires)): son = sonos() now_playing = son.now_playing() if(self.lastsong == '' or now_playing != self.lastsong): newsonos = True now_playing = self.translate(now_playing) self.lastsonostime = current_time # check news newnews = False #if(self.lastnewstime < (current_time - self.news_expires)): # self.log('--checking news--') # feed = feedparser.parse('http://api.breakingnews.com/api/v1/item/?format=rss') # news_age = (current_time + (60*60*5)) - time.mktime(feed['entries'][0]['updated_parsed']) # if(news_age < (10*60)): # if(self.lastnews != feed['entries'][0]['title']): # self.lastnews = feed['entries'][0]['title'] # newnews = True # else: # if(self.lastnews != ''): # self.lastnews = '' # newnews = True # self.lastnewstime = current_time # check weather newweather = False if(self.lastweather < (current_time - self.weather_expires)): self.log('-- weather update --') newweather = True self.lastweather = current_time w = weather() self.conditions = w.get() # check mlb scores newmlb = False score = '' if(self.lastmlbtime < (current_time - self.mlb_expires)): self.lastmlbtime = current_time m = mlb() score = m.today_by_team('PHI') if(score != self.lastmlb): self.log('-- mlb update --') newmlb = True self.lastmlb = score # update sign if we have new shit if(newmlb or newweather or newnews or newsonos): self.log('-- sign update --') self.lastsong = now_playing #self.lastscore = lastscore s = serial.Serial('/dev/ttyUSB0', 9600, timeout=10) #wake up msg = "~128~f0" # set the time on startup #msg += "~E" + time.strftime("%u1%y%m%d%H%M%S") #self.log('-- time update --') #self.log(msg) #msg += "\r\r\r" msg += "\rC\\c" #effect msg += "\\b" msg += "\a" #color msg += now_playing self.log(now_playing) #time & temp msg += "\rS\\c" #pacman msg += "\w" #small msg += '^D - ' + self.conditions['current_temp'] + ' F' self.log(self.conditions['current_temp'] + ' F') #news if(self.lastnews): msg += "\rS\\c" msg += "\w" msg += "BREAKING: " + self.translate(self.lastnews) self.log("BREAKING: " + self.translate(self.lastnews)) #forecast msg += "\rS\\c" msg += "\w" #small msg += self.translate(self.conditions['summary']) self.log(self.translate(self.conditions['summary'])) #cinco de julio msg += "\rC\\c" #explosions msg += self.lastmlb self.log(self.lastmlb) #end msg += "\r\r\r" s.write(msg) s.close() except KeyboardInterrupt: print("Ok ok, quitting") sys.exit(1) except: self.log("Unexpected error.") pprint.pprint(sys.exc_info()) #self.log("Unexpected error: " + sys.exc_info()[0]) pass GPIO.cleanup()
def __init__(self, ip='0.0.0.0', port=9000, log_level=logging.DEBUG): self.ip = ip self.port = port self.author = __author__ self.version = __version__ self.file_path = os.path.realpath(__file__) self.dir_path = os.path.dirname(self.file_path) # mark system start time self.system_initialized = datetime.now() # weixin about self.appid = 'wxb1efccbbb5bafcbb' self.appsecret = '9d64356f48062e46159b4d179dea5c44' self.token = 'shenhailuanma' self.access_token = '' self.signature = None self.echostr = None self.timestamp = None self.nonce = None self.wechat = WechatBasic(token=self.token, appid=self.appid, appsecret=self.appsecret) self.weather = weather() # database self.database = Database() # set the logger self.log_level = logging.DEBUG self.log_path = 'myWeixinServer.log' self.logger = logging.getLogger('myWeixinServer') self.logger.setLevel(self.log_level) # create a handler for write the log to file. fh = logging.FileHandler(self.log_path) fh.setLevel(self.log_level) # create a handler for print the log info on console. ch = logging.StreamHandler() ch.setLevel(self.log_level) # set the log format formatter = logging.Formatter('%(asctime)s - %(name)s - %(levelname)s - %(message)s') fh.setFormatter(formatter) ch.setFormatter(formatter) # add the handlers to logger self.logger.addHandler(fh) self.logger.addHandler(ch) self.logger.info('init over.') ####### web test ###### @bottle.route('/') def index_get(): try: # get the post data self.logger.debug('handle a GET request: /, ') # e.g : /?signature=04d39d841082682dc7623945528d8086cc9ece97&echostr=8242236714827861439×tamp=1440564411&nonce=2061393952 # get the data self.logger.debug('handle the request data: %s' %(bottle.request.query_string)) #self.logger.debug('handle the request signature:%s' %(bottle.request.query.signature)) #self.logger.debug('handle the request echostr:%s' %(bottle.request.query.echostr)) #self.logger.debug('handle the request timestamp:%s' %(bottle.request.query.timestamp)) #self.logger.debug('handle the request nonce:%s' %(bottle.request.query.nonce)) return bottle.request.query.echostr except Exception,ex: return "%s" %(ex) return "Hello, this is myWeixinServer."
#!/usr/bin/env python from weather import weather import datetime import sys if(len(sys.argv)<2): print 'Error: use format--> ./sample.py "enter you city name here"' else: city = weather(sys.argv[1]) print 'Weather |',city.name,city.country,'at', print (datetime.datetime.fromtimestamp(int(city.dt)).strftime('%H:%M:%S %m-%d-%Y ')) print '------------------------------------------' print '\t','Temp:',city.temp,'Celsius' print '\t','Temp(min,max):','(',city.temp_min,',',city.temp_max,')','Celsius' print '\t','Pressure:',city.pressure,'hPa' print '\t','Humidity:',city.humidity,'%' print '\t',city.sky print '\t','Clouds %:',city.cloud print '\t','Wind speed:',city.wind ,'mps'
from sqlalchemy import create_engine from sqlalchemy.orm import sessionmaker from logger_base import Item, Average, Base import time, weather, datetime from dateutil import parser engine = create_engine('sqlite:///logbase.db') Base.metadata.bind = engine weer = weather.weather() DBSession = sessionmaker(bind=engine) session = DBSession() while 1: print datetime.time() a = weer.measure() dt = parser.parse(a['time']) light = Item(name='light', value=a['light'], timestamp=dt) pressure = Item(name='pressure', value=a['pressure'], timestamp=dt) temp = Item(name='temp', value=a['temp'], timestamp=dt) session.add(light) session.add(pressure) session.add(temp) session.commit() time.sleep(60)
from_addr = '*****@*****.**' password = '******' to_addr =['*****@*****.**','*****@*****.**'] to_addr2 =['*****@*****.**','*****@*****.**'] smtp_server = 'smtp.163.com' msg = MIMEMultipart() msg['From'] = _format_addr('Jameslong <%s>' % from_addr) msg['To'] = _format_addr('小鱼 <%s>' % to_addr2) msg['Subject'] = Header('I am a robor …', 'utf-8').encode() #NanChang url = 'http://www.weather.com.cn/weather/101240101.shtml' wea = weather.weather(url) html = wea.getContent() html = str(html[0]) msg.attach(MIMEText('<div style="background:url(http://img5q.duitang.com/uploads/blog/201410/05/20141005191614_iccht.jpeg);background-size:100% auto; background-repeat: y-repeat;"><h1 style="text-align:center;">Jameslong,南昌天气</h1>' + html +'<h1 style="text-align:center; font-size:32px;padding-top:120px;">Good morning!</h1></div>','html','utf-8')) #ChongQing url_cq = 'http://www.weather.com.cn/weather/101040100.shtml' wea_cq = weather.weather(url_cq) html_cq = wea_cq.getContent() html_cq = str(html_cq[0]) msg_cq = MIMEMultipart() msg_cq['From'] = _format_addr('南山一颗树 <%s>' % from_addr) msg_cq['To'] = _format_addr('梦园 <%s>' % to_addr) msg_cq['Subject'] = Header('I am a robor …', 'utf-8').encode() server = smtplib.SMTP(smtp_server, 25)
# Clear matrix on exit. Otherwise it's annoying if you need to break and # fiddle with some code while LEDs are blinding you. def clearOnExit(): """ Clear matrix on exit. Otherwise it's annoying if you need to break and fiddle with some code while LEDs are blinding you. """ matrix.Clear() atexit.register(clearOnExit) myWeather = weather("KBOS") for i in xrange(10, 0, -1): draw.rectangle((0, 0, width, height), fill=(0, 0, 0)) draw.text((0, 0), "Restarting In", font=font, fill=routeColor) draw.text((0, 12), str(i), font=font, fill=routeColor) # Offscreen buffer is copied to screen matrix.SetImage(image.im.id, 0, 0) time.sleep(1) matrix.Clear() # Populate a list of predict objects (from predict.py) from stops[]. # While at it, also determine the widest tile width -- the labels # accompanying each prediction. The way this is written, they're all the # same width, whatever the maximum is we figure here. tileWidth = font.getsize(
def say_status(msg=None): tts(version_text()) time.sleep(len(version_text().split()) * (1 / 2.1)) weather()
import searcher import data_load import indexer import WEBcrawler import weather weather.weather() visit_url.visit_url() indexer.indexer() searcher.searcher() data_load.traverser() d = indexer.indexer("raw_data.pickle","shelve") searcher.search(d)
def get(self): Access_CronJob = True headers = self.request.headers.items() for key, value in headers: if (key == 'X-Appengine-Cron') and (value == 'true'): Access_CronJob = True break # 如果不是CronJob来源的请求,记录日志并放弃操作 if (not Access_CronJob): logging.debug('CronJobCheck() access denied!') logging.critical('如果这个请求不是由你手动触发的话,这意味者你的CronJobKey已经泄漏!请立即修改CronJobKey以防被他人利用') return mydate = datetime.utcnow() + timedelta(hours=+8) ts_hour = mydate.time().hour ts_min = mydate.time().minute dbug = self.request.get('debug') logging.debug(dbug) # 7:00早安世界 if (((ts_hour == 7) and ( 30 <= ts_min <= 32)) or (dbug=='morning')): # 7:00 error = False try: wther=weather.weather() except weather.FetchError: logging.error("Weather Fetch Error!") error = True msg_idx=random.randint(0,len(config.MSG_GET_UP)-1) if error: msg = '%s%s' % (config.MSG_GET_UP[msg_idx],config.BOT_HASHTAG) else: msg = '%s 今天%s的天气是:%s %s' % \ (config.MSG_GET_UP[msg_idx], config.CITY, wther, config.BOT_HASHTAG) OAuth_UpdateTweet(msg) # 早安世界 logging.info("%s:%d" % (msg,wther)) # 23:30 晚安世界 elif ((ts_hour == 23) and (30 <= ts_min <=32)): # 23:30 msg_idx=random.randint(0,len(config.MSG_SLEEP)-1) msg = '%s%s' % (config.MSG_SLEEP[msg_idx], config.BOT_HASHTAG) OAuth_UpdateTweet(msg) # 晚安世界 logging.info(msg) # 每小时一条命令 elif (((7<=ts_hour<=23) and (15<=ts_min<=17)) or (dbug=='cli')): msg = command.random() if msg != None: msg = msg.replace("# commandlinefu.com by David Winterbottom\n\n#","//") msg = '%s %s' % ( "叮咚!小bot教CLI时间到了!", msg[:-1]) msg +="#commandlinefu #xdlinux" logging.info(msg) OAuth_UpdateTweet(msg) # 扫TL,转推 auth = tweepy.OAuthHandler(config.CONSUMER_KEY, config.CONSUMER_SECRET) auth.set_access_token(config.ACCESS_TOKEN, config.ACCESS_SECRET) api = tweepy.API(auth) #since id tweetid=SinceID.all().get() logging.info(tweetid) if ( tweetid == None ): logging.warning("Initial!") tweetid=SinceID() timeline = api.home_timeline() else: logging.info("Since ID is: %d" % tweetid.since_id) timeline = api.home_timeline(since_id=tweetid.since_id) #self.response.out.write('GETTING TIMELINE<br />') regx=re.compile(config.RT_REGEX,re.I|re.M) mgc = re.compile(config.MGC,re.I|re.M) talk_to_me = re.compile(config.TALK,re.I|re.M) tweets=timeline[::-1] # 时间是倒序的 if tweets == []: logging.info("no new tweets!") return msg=None seg = SEG() for tweet in tweets: user = tweet.user.screen_name if user == 'xdtuxbot': continue text = tweet.text n = mgc.search(text) if n != None: continue t = talk_to_me.search(text) if (not t) and text[0]=='@': continue wlist = seg.cut(text.encode('utf-8')) logging.info( ' '.join(wlist) ) for w in wlist: if w in config.RT_LIST: break else: continue if t: bot = TalkBot() reply = bot.respond( talk_to_me.sub("",text) ).decode('UTF-8') if reply != '': msg = u"@%s %s" % (user, reply) try: if msg: OAuth_UpdateTweet(msg,reply=tweet.id) # 发送到Twitter logging.info('Send Tweet: %s' % (msg)) else: api.retweet(tweet.id) except tweepy.TweepError, e: logging.error('Tweepy Error:%s' % e) except Exception, e: logging.error('Uknow Error:%s' % e)
distave = 0 count = 0 channel = 0 delay = 0 flag1 = 0 flag2 = 0 flag3 = 0 counts = 0 voltave = 0 sign = 0 threshold_u = 0.17 threshold_b = 65 volts = [1, 1, 1, 1, 1] dists = [99, 99, 99] color = "orange" condition = weather.weather() addr = bulb.address() condition = "sunny" bulb.on(addr) bulb.on(addr) bulb.on(addr) bulb.off(addr) bulb.off(addr) bulb.off(addr) if re.search("rain", condition): sign = 1 while True: cursor.execute("select weather, color from controller") table = cursor.fetchall() tp = [0, 0]