def giphy(config, terms): """ Return giphy for given terms. """ terms = ' '.join(terms) if terms: img = translate(terms, api_key=config['giphy']['api_key']) else: img = translate('random', api_key=config['giphy']['api_key']) # hack url = img.fixed_height.downsampled.url return [url]
def download_gif(self, term, slide_num): # If we have at least 3 local gifs, use one of those if (term in self.gifs) and (len(self.gifs[term]) > 3): return os.path.join("GIFs", "%s.gif" % random.choice(self.gifs[term])) try: # Download the gif img = translate(term) image_path = os.path.join(self.resources_dir, "%d.gif" % slide_num) wget.download(img.fixed_height.url, image_path) file_hasher = hashlib.md5() with open(image_path, "rb") as f: file_hasher.update(f.read()) file_md5 = file_hasher.hexdigest() if not (term in self.gifs): self.gifs[term] = [] if not (file_md5 in self.gifs[term]): self.gifs[term].append(file_md5) shutil.copy(image_path, os.path.join("GIFs", "%s.gif" % file_md5)) with open(os.path.join("GIFs", "hashes.json"), "w") as f: json.dump(self.gifs, f, indent=2) return image_path except: return None
def download_gif(self, term, slide_num): # If we have at least 3 local gifs, use one of those if (term in self.gifs) and (len(self.gifs[term]) > 3): return os.path.join("GIFs", "%s.gif" % random.choice(self.gifs[term])) try: # Download the gif img = translate(term) image_path = os.path.join(self.resources_dir, "%d.gif" % slide_num) wget.download(img.fixed_height.url, image_path) file_hasher = hashlib.md5() with open(image_path, "rb") as f: file_hasher.update(f.read()) file_md5 = file_hasher.hexdigest() if not (term in self.gifs): self.gifs[term] = [] if not (file_md5 in self.gifs[term]): self.gifs[term].append(file_md5) shutil.copy(image_path, os.path.join("GIFs", "%s.gif" % file_md5)) with open(os.path.join("GIFs", "hashes.json"), "w") as f: json.dump(self.gifs, f, indent=2) return image_path except: return None
async def tmute(ctx, time: int, member: discord.Member = None): if not member and not time: await ctx.send( "```🥀 Выберите пользователя и введите время мута.```") return elif not member: await ctx.send("```🥀 Выберите пользователя.```") return elif not time: await ctx.send( "```🌻 Введите время мута, или используйте команду \"!mute @user\".```" ) return elif member and time: role = discord.utils.get(ctx.guild.roles, name="замучен") embed1 = discord.Embed( title=f"{member.display_name}", description= f"{member.mention} был замучен.\n\nАдминистратор - {ctx.message.author.mention}.", color=0xff00f6) embed1.set_footer(text=f"{time_now}") img_muted = translate('muted', api_key=giphy_api_key) embed1.set_image(url=img_muted.media_url) await ctx.send(embed=embed1) await member.add_roles(role) await asyncio.create_task(unmute_task(ctx, member, role, time)) await ctx.send( f"{member.mention} был размучен.\n\nСрок блокировки - {time}.\n\nАдминистратор - {ctx.message.author.mention}.", color=0xff00f6) logging.warning( Fore.LIGHTGREEN_EX + f"{time_now} | NEW EVENT: ADMIN {ctx.message.author.display_name} TMUTE USER {member.display_name} ON {time} SECONDS!" )
def gif(bot, message, author=None, debug=False): img = giphypop.translate(phrase=message, strict=True) if debug: print(img.media_url) else: bot.post(img.media_url)
def download_gif(self, term, slide_num): # If we have at least 3 local gifs, use one of those if (term in self.gifs) and (len(self.gifs[term]) > 3): return os.path.join("GIFs", "%s.gif" % random.choice(self.gifs[term])) try: # Download the gif #img = translate(term, app_key=self.GIPHY_API_KEY) img = translate(term) image_path = os.path.join(self.resources_dir, "%d.gif" % slide_num) wget.download(img.media_url, image_path) if not (term in self.gifs): self.gifs[term] = [] if not (img.id in self.gifs[term]): self.gifs[term].append(img.id) shutil.copy(image_path, os.path.join("GIFs", "%s.gif" % img.id)) with open(os.path.join("GIFs", "hashes.json"), "w") as f: json.dump(self.gifs, f, indent=2) return image_path except: return None
async def hug(ctx, member: discord.Member = None): if not member: await ctx.send("```🥀 Данного пользователя не существует!```") elif member: embed1 = discord.Embed( title="owo 💕🔫", description= f"{ctx.message.author.mention} обнял {member.mention} ❤️", color=0xff00f6) img_hug = translate('anime hug', api_key=giphy_api_key) embed1.set_image(url=img_hug.media_url) await ctx.send(embed=embed1)
def do(msg, optionsList): #Gets the first giphy result, downloads and uploads to chat gif = giphypop.translate(msg[0]) if gif == None: return "No Results." while gif.filesize > 5000000: gif = giphypop.translate(msg[0]) url = gif.media_url dlFile = gif.id + ".gif" #Save image to disk if not os.path.isfile(etcDir+dlFile): imgData = urllib2.urlopen(url).read() output = open(etcDir+dlFile, 'wb+') output.write(imgData) output.close() sdk.media.send_doc(optionsList[1], etcDir+dlFile, optionsList[0]) return "Uploading " + msg[0] + "\n" + gif.media_url + "\n" + str(gif.filesize) + " Bytes"
def do(msg, optionsList): #Gets the first giphy result, downloads and uploads to chat gif = giphypop.translate(msg[0]) if gif == None: return "No Results." while gif.filesize > 5000000: gif = giphypop.translate(msg[0]) url = gif.media_url dlFile = gif.id + ".gif" #Save image to disk if not os.path.isfile(etcDir + dlFile): imgData = urllib2.urlopen(url).read() output = open(etcDir + dlFile, 'wb+') output.write(imgData) output.close() sdk.media.send_doc(optionsList[1], etcDir + dlFile, optionsList[0]) return "Uploading " + msg[0] + "\n" + gif.media_url + "\n" + str( gif.filesize) + " Bytes"
def main(): logging.basicConfig( format='%(asctime)s - %(name)s - %(levelname)s - %(message)s') bot = telegram.Bot(token='YOUR TOKEN HERE') try: LAST_UPDATE_ID = bot.getUpdates()[-1].update_id except IndexError: LAST_UPDATE_ID = None while True: for update in bot.getUpdates(offset=LAST_UPDATE_ID, timeout=5): text = update.message.text chat_id = update.message.chat.id update_id = update.update_id if '/start' in text: custom_keyboard = [["/quote","/gif"]] reply_markup = telegram.ReplyKeyboardMarkup(custom_keyboard, resize_keyboard=True) bot.sendMessage(chat_id=chat_id, text="Choose.", reply_markup=reply_markup) LAST_UPDATE_ID = update_id + 1 elif '/quote' in text: answer = quote() bot.sendMessage(chat_id=chat_id, text=answer) LAST_UPDATE_ID = update_id + 1 rn = random.randint(0,10) if rn == 1: new_msg = "You've been visited by the TARDIS. " bot.sendMessage(chat_id=chat_id,text=new_msg) LAST_UPDATE_ID = update_id + 1 elif rn == 0 : new_msg = "Raxacoricofallapatorious!" bot.sendMessage(chat_id=chat_id,text=new_msg) LAST_UPDATE_ID = update_id + 1 elif '/gif' in text: bot.sendMessage(chat_id=chat_id, text="All time and space, there must be a gif around.") img = translate('doctor who') bot.sendDocument(chat_id=chat_id, document=img.fixed_height.url) print "Enviar Gif " + img.fixed_height.url LAST_UPDATE_ID = update_id + 1 else: bot.sendMessage(chat_id=chat_id,text="Command not recognized") LAST_UPDATE_ID = update_id + 1
async def gif(self, ctx): # Display's a gif from a selected phrase from giphy """Returns gif syntax: s.gif [phrase]""" if(await Helper.isBanned(self,ctx) == True): # Checks bans return if(await Helper.isPerms(self,ctx,3)): # Checks permissions phrase = ctx.message.content.replace(self.bot.config['prefix']+"gif","") # Gets the term if(ctx.message.content == "s.gif"): # If the search term was blank then return friendly message await self.bot.say("```Type search ya dingus```") img = giphypop.translate(phrase) # If validation is ok search the phrase if(img is None): # If no image is found alert the user await self.bot.say("I couldn't find it try looking something else up.") await self.bot.say(img.url) #Display image await Helper.log(self,str(ctx.message.author),"giphy "+phrase,str(ctx.message.timestamp)) # Logs command
async def suicide(ctx): embed1 = discord.Embed( title=f"Прощай {ctx.message.author}", description=f"{ctx.message.author.mention} выбрал легкий путь...😢️", color=0xff00f6) img_cry = translate( 'anime cry', api_key=giphy_api_key, ) embed1.set_image(url=img_cry.media_url) await ctx.send(embed=embed1) member = ctx.message.author await member.kick() logging.critical( Fore.LIGHTGREEN_EX + f"{time_now} | NEW EVENT: USER {ctx.message.author.display_name} USE COMMAND SUICIDE!" )
def farm_gif_term(self, term, amount=25, threshold=10): self.log("Farming GIFs for '%s'..." % term) if not (term in self.gifs): self.gifs[term] = [] attempt_count = 0 while (attempt_count < threshold) and (len(self.gifs[term]) < amount): image_path = "test.gif" try: os.remove(image_path) except: pass try: #img = translate(term, app_key=self.GIPHY_API_KEY) img = translate(term) if not (img.id in self.gifs[term]): self.log_inline(" [%d/%d] " % (len(self.gifs[term]), amount)) wget.download(img.media_url, image_path) self.gifs[term].append(img.id) shutil.copy(image_path, os.path.join("GIFs", "%s.gif" % img.id)) self.log("%s.gif" % (img.id)) attempt_count = 0 else: attempt_count += 1 except: self.log("download failed") attempt_count += 1 with open(os.path.join("GIFs", "hashes.json"), "w") as f: json.dump(self.gifs, f, indent=2) with open(os.path.join("GIFs", "hashes.js"), "w") as f: f.write("gifs = {\n") for gifs_term, gifs_hashes in self.gifs.iteritems(): f.write("'%s': [\n" % gifs_term) for gif in gifs_hashes: f.write("'%s',\n" % gif) f.write("],\n") f.write("};\n") self.log("Farming GIFs for '%s' complete with %d files" % (term, len(self.gifs[term])))
def giphy(): username = "******" + request.args['user_name'] channel = "#" + request.args['channel_name'] text = request.args['text'] if text == "": return "", 200 giph = translate(text) if giph == None: resetlimit(request.args['user_name'], "gif", request.args['channel_name']) return "no giphy found, " + random.choice(insults) , 200 payload = { "channel": channel, "username": "******", "icon_url": "https://api.giphy.com/img/api_giphy_logo.png", "text": username + ' "' + text + '"\n' + giph.url, } r = requests.post(config.webhook_url, data=json.dumps(payload)) return "", 200
def hedwig(): keyword = request.values.get('text') gif = translate(keyword) channel = '#hedwig' filename = 'https://media1.giphy.com/media/fAT2Db0j0Mblu/200_s.gif' try: filename = g.random_gif(keyword).media_url except GiphyApiException: try: filename = g.translate(keyword).media_url except GiphyApiException: try: filename = g.search_list(keyword, limit=1[0]).media_url except (GiphyApiException, IndexError): pass slack.chat.post_message(channel, filename, username='******') return ''
def giphy(): username = "******" + request.args['user_name'] channel = "#" + request.args['channel_name'] text = request.args['text'] if text == "": return "", 200 giph = translate(text) if giph == None: resetlimit(request.args['user_name'], "gif", request.args['channel_name']) return "no giphy found, " + random.choice(insults), 200 payload = { "channel": channel, "username": "******", "icon_url": "https://api.giphy.com/img/api_giphy_logo.png", "text": username + ' "' + text + '"\n' + giph.url, } r = requests.post(config.webhook_url, data=json.dumps(payload)) return "", 200
async def ban(ctx, member: discord.Member = None): if not member: await ctx.send("```🥀 Выберите пользователя.```") return elif member: embed1 = discord.Embed( title=f"{member.display_name}", description= f"{member.mention} был забанен.\n\nАдминистратор - {ctx.message.author.mention}.", color=0xff00f6) embed1.set_footer(text=f"{time_now}") img_banned = translate('banned', api_key=giphy_api_key) embed1.set_image(url=img_banned.media_url) await ctx.send(embed=embed1) await member.ban() logging.warning( Fore.LIGHTGREEN_EX + f"{time_now} | NEW EVENT: ADMIN {ctx.message.author.display_name} BAN USER {member.display_name}!" )
async def unmute(ctx, member: discord.Member = None): if not member: await ctx.send("```🥀 Выберите пользователя.```") return elif member: role = discord.utils.get(ctx.guild.roles, name="замучен") embed1 = discord.Embed( title=f"{member.display_name}", description= f"{member.mention} был размучен.\n\nАдминистратор - {ctx.message.author.mention}.", color=0xff00f6) embed1.set_footer(text=f"{time_now}") img_unmute = translate('unmuted', api_key=giphy_api_key) embed1.set_image(url=img_unmute.media_url) await ctx.send(embed=embed1) await member.remove_roles(role) logging.warning( Fore.LIGHTGREEN_EX + f"{time_now} | NEW EVENT: ADMIN {ctx.message.author.display_name} UNMUTE USER {member.display_name}!" )
def farm_gif_term(self, term, amount=25, threshold=10): self.log("Farming GIFs for %s..." % term) if not (term in self.gifs): self.gifs[term] = [] attempt_count = 0 while (attempt_count < threshold) and (len(self.gifs[term]) < amount): image_path = "test.gif" try: os.remove(image_path) except: pass try: img = translate(term) wget.download(img.fixed_height.url, image_path) image_md5 = self.get_file_md5("test.gif") if not (image_md5 in self.gifs[term]): self.gifs[term].append(image_md5) shutil.copy(image_path, os.path.join("GIFs", "%s.gif" % image_md5)) self.log(" GIF saved to archive. %d/%d GIFs." % (len(self.gifs[term]), amount)) attempt_count = 0 else: self.log(" Already had GIF!") attempt_count += 1 except: self.log(" Downloading failed") attempt_count += 1 self.log("Farming of %s GIFs complete, now holding %d GIFs" % (term, len(self.gifs[term]))) with open(os.path.join("GIFs", "hashes.json"), "w") as f: json.dump(self.gifs, f, indent=2)
def main(): logging.basicConfig( format='%(asctime)s - %(name)s - %(levelname)s - %(message)s') bot = telegram.Bot(token='YOUR BOT AUTHORIZATION TOKEN') try: LAST_UPDATE_ID = bot.getUpdates()[-1].update_id except IndexError: LAST_UPDATE_ID = None while True: for update in bot.getUpdates(offset=LAST_UPDATE_ID, timeout=5): text = update.message.text chat_id = update.message.chat.id update_id = update.update_id if '/start' in text: custom_keyboard = [["/quote", "/gif"]] reply_markup = telegram.ReplyKeyboardMarkup(custom_keyboard, resize_keyboard=True) bot.sendMessage(chat_id=chat_id, text="Chose.", reply_markup=reply_markup) LAST_UPDATE_ID = update_id + 1 elif '/quote' in text: answer = quote() bot.sendMessage(chat_id=chat_id, text=answer) LAST_UPDATE_ID = update_id + 1 elif '/gif' in text: bot.sendMessage(chat_id=chat_id, text="Nick is searching for an awesome gif.") img = translate('nicolas cage') bot.sendDocument(chat_id=chat_id, document=img.fixed_height.url) print "Enviar Gif " + img.fixed_height.url LAST_UPDATE_ID = update_id + 1
def main(): logging.basicConfig( format='%(asctime)s - %(name)s - %(levelname)s - %(message)s') bot = telegram.Bot(token='HERE GOES THE TOKEN!') try: LAST_UPDATE_ID = bot.getUpdates()[-1].update_id except IndexError: LAST_UPDATE_ID = None while True: for update in bot.getUpdates(offset=LAST_UPDATE_ID, timeout=5): text = update.message.text chat_id = update.message.chat.id update_id = update.update_id if '/start' in text: custom_keyboard = [["/quote", "/gif"]] reply_markup = telegram.ReplyKeyboardMarkup(custom_keyboard, resize_keyboard=True) bot.sendMessage(chat_id=chat_id, text="I'm Mr. Meeseeks! Look at me!!", reply_markup=reply_markup) LAST_UPDATE_ID = update_id + 1 elif '/quote' in text: answer = quote() bot.sendMessage(chat_id=chat_id, text=answer) LAST_UPDATE_ID = update_id + 1 elif '/gif' in text: bot.sendMessage(chat_id=chat_id, text="Ooohhh can do. ") img = translate('rick and morty') bot.sendDocument(chat_id=chat_id, document=img.fixed_height.url) print "Enviar Gif " + img.fixed_height.url LAST_UPDATE_ID = update_id + 1
def farm_gif_term(self, term, amount=25, threshold=10): self.log("Farming GIFs for %s..." % term) if not (term in self.gifs): self.gifs[term] = [] attempt_count = 0 while (attempt_count < threshold) and (len(self.gifs[term]) < amount): image_path = "test.gif" try: os.remove(image_path) except: pass try: img = translate(term) wget.download(img.fixed_height.url, image_path) image_md5 = self.get_file_md5("test.gif") if not (image_md5 in self.gifs[term]): self.gifs[term].append(image_md5) shutil.copy(image_path, os.path.join("GIFs", "%s.gif" % image_md5)) self.log(" GIF saved to archive. %d/%d GIFs." % (len(self.gifs[term]), amount)) attempt_count = 0 else: self.log(" Already had GIF!") attempt_count += 1 except: self.log(" Downloading failed") attempt_count += 1 self.log("Farming of %s GIFs complete, now holding %d GIFs" % (term, len(self.gifs[term]))) with open(os.path.join("GIFs", "hashes.json"), "w") as f: json.dump(self.gifs, f, indent=2)
# print len(tweet) print(" ") print("Ready for new Tweet!") print(" ") try: while True: # nature_words() data = ser.readline().rstrip() data_dec = (data.decode('utf-8')) data_dec_s = data_dec.split(":") x,y = data_dec_s[0], data_dec_s[1] y = int(y) if 20 <= y <= 399: adj = random.choice(ADJECTIVE) img = translate(adj, api_key='api_key') print(x, y, adj) print(img.url) print(img.bitly) tweet = "Twitterplanten på NNV: Hjelp! @nordnorskviten " + " Jeg trenger vann med en gang! " + "Tilfeldig gif: " + adj + " " + img.bitly main() elif y > 400: adj2 = random.choice(ADJECTIVE2) img2 = translate(adj2, api_key='api_key') print(x, y, adj2) print(img2.url) print(img2.bitly) tweet = "Twitterplanten på NNV: Hurra, jeg trenger ikke vann nå! " + "Tilfeldig gif: " + adj2 + " " + img2.bitly main() finally:
status = api.update_status(status=tweet) print(tweet) time.sleep(600) try: while True: data = ser.readline().rstrip() data_s = data.split(":") x, y = data_s[0], data_s[1] y = int(y) if 3 <= y <= 25: print("Alert! ") count = count + 1 print(count) img = translate('chinchilla', api_key='dc6zaTOxFJmzC') print(img.url) print(img.bitly) # g = safygiphy.Giphy() # r = g.random(tag="success") # print(r) # tweet = random.choice(WORDS1) + " " + random.choice(WORDS2) + " " + random.choice(WORDS3) + " " + random.choice(HASTAG) + " - Random tweet from Aragorn Chinchilla #chinchilla #microbit #python" tweet = "I like to " + random.choice(VERB) + " " + random.choice( ADJECTIVE ) + " " + random.choice(COLOUR) + " " + random.choice( NOUN ) + " " + random.choice( HASTAG ) + " - Random tweet from Aragorn Chinchilla #chinchilla #microbit #python" + " " + img.bitly main()
def get_giphy(text): img = giphypop.translate(text) return img.media_url
async def gif(self,ctx,*,query): async with ctx.typing(): img=gp.translate(query) await ctx.send(img.media_url)
def feed(): # if the picture is rated, add it to the history if rate != None: add_to_history(request.form.get("photo_id")) # get a picture that's unique and not from yourself picture_info = get_right_picture(request.form.get("photo_id"), request.form.get("rate"), request.form.get("check_comment")) if picture_info == "comment": picture_info = get_picture_info(request.form.get("photo_id")) # give apology when no pictures left to rate if picture_info == "apology": return apology("all out of photo's") # store frequently used variables user_id = picture_info[0]["id"] photo_id = int(picture_info[0]["photo_id"]) # store frequently used render template for feed redirect_to_feed = render_template( "feed.html", photo_path=picture_info[0]["photo_path"], rating=round(picture_info[0]["rating"], 1), gifs=show_gifs(photo_id), username=get_username(user_id), user_id=user_id, comments=show_comments(photo_id), photo_id=photo_id, caption=picture_info[0]["caption"]) if request.method == "POST": # report a persons post if request.form.get("report") != None: report(photo_id, user_id) return redirect_to_feed # add to history after rating if request.form.get("rate") != None: rating = int(request.form.get("rate")) rate(rating, request.form.get("photo_id")) return redirect_to_feed # add comments if request.form.get("comment") != None: # add gif as a comment if request.form.get("comment").startswith("/gif"): query = request.form.get("comment")[len("/gif"):] giphy = translate(query, api_key="OqJEhuVDXwcAVJbRre1ubPPRj2nkjMWh") gif = giphy.fixed_height.downsampled.url add_gif(gif, request.form.get("photo_id"), session["user_id"]) return redirect_to_feed # add a normal comment else: add_comment(request.form.get("comment"), request.form.get("photo_id"), session["user_id"]) return redirect_to_feed else: # redirect to feed when GET return redirect_to_feed
async def on_message(message): # we do not want the bot to reply to itself if message.author == client.user: return #help command. if message.content.startswith('{}help'.format(cmd)): await client.send_message(message.channel, ':mailbox_with_mail: {0.author.mention}'.format(message)) msg = '```Heres a list of my commands:'\ '\n\n-ping - Bot simply replies ping (unless your special) useful for knowing whether or not It\'s online'\ '\n\n-cat - Bot posts a random cat picture'\ '\n\n-say [message] - Bot echos your message'\ '\n\n-trump - Bot posts a random Trump gif'\ '\n\n-hillary - Bot posts a random Hillary gif'\ '\n\n-bernie - Bot posts a random Bernie gif'\ '\n\n-horse - Bot posts a random horse gif'\ '\n\n-dog - Bot posts a random dog gif'\ '\n\n-restart - restarts the bot (only works for Blake and Zach aka the bot devs)'\ '\n\n-shutdown - turns the bot off (again only works for Blake and Zach)'\ '\n\n-leave [server name] - gets the bot to leave a server (Only works for Blake)'\ '\n\n-guess - starts the guessing game'\ '\n\n-mark - a special song written by Bahar for Mark'\ '\n\n-invite - get an invite link for the bot so it can join your server!'\ '\n\nBot coded by Blake with help from Zach.```'\ await client.send_message(message.author, msg) #Ping command. elif message.content.startswith('{}ping'.format(cmd)): if message.author.id == '129437909131591680': msg = 'Bang, Bang! {0.author.mention}'.format(message) await client.send_message(message.channel, msg) elif message.author.id == '183790956754108416': msg = 'Hello Master! :wave: {0.author.mention}'.format(message) await client.send_message(message.channel, msg) elif message.author.id == '129439119737749505': msg = 'You\'re a nerd. you ain\'t got no balls! {0.author.mention}'.format(message) await client.send_message(message.channel, msg) elif message.author.id == '238469392155803649': msg = 'Hello Queen Alexis! :wave: {0.author.mention}'.format(message) await client.send_message(message.channel, msg) elif message.author.id == '230196465807392769': msg = 'My Queen! :heart: {0.author.mention}'.format(message) await client.send_message(message.channel, msg) elif message.author.id == '192664155348533248': msg = 'Mr. Pattems! :grin: {0.author.mention}'.format(message) await client.send_message(message.channel, msg) else: msg = 'PONG! {0.author.mention}'.format(message) await client.send_message(message.channel, msg) #cat command elif message.content.startswith('{}cat'.format(cmd)): async with aiohttp.get('http://random.cat/meow') as r: if r.status == 200: js = await r.json() await client.send_message(message.channel,js['file']) #Trump command elif message.content.startswith('{}trump'.format(cmd)): img = translate('Trump', api_key='dc6zaTOxFJmzC') await client.send_message(message.channel,img) #Hillary command elif message.content.startswith('{}hillary'.format(cmd)): img = translate('Hillary', api_key='dc6zaTOxFJmzC') await client.send_message(message.channel,img) #Bernie command elif message.content.startswith('{}bernie'.format(cmd)): img = translate('Bernie', api_key='dc6zaTOxFJmzC') await client.send_message(message.channel,img) #Horse command elif message.content.startswith('{}horse'.format(cmd)): img = translate('Horse', api_key='dc6zaTOxFJmzC') await client.send_message(message.channel,img) #Dog command elif message.content.startswith('{}dog'.format(cmd)): img = translate('Dog', api_key='dc6zaTOxFJmzC') await client.send_message(message.channel,img) #Say command elif message.content.startswith('{}say'.format(cmd)): messageToTTS = message.content[5:] await client.send_message(message.channel, content=messageToTTS) #Restart command elif message.content.startswith('{}restart'.format(cmd)): if message.author.id == '183790956754108416': msg = '```Restarting!!```' await client.send_message(message.channel, msg) os.execl(sys.executable, sys.executable, *sys.argv) elif message.author.id == '129437909131591680': msg = '```Restarting!!```' await client.send_message(message.channel, msg) os.execl(sys.executable, sys.executable, *sys.argv) else: msg = '```ERROR: What the F**K do you think you\'re doing?!```' await client.send_message(message.channel, msg) #shutdown command elif message.content.startswith('{}shutdown'.format(cmd)): if message.author.id == '183790956754108416': await client.send_message(message.channel, 'Peace out guys! :v:') await client.logout() elif message.author.id == '129437909131591680': await client.send_message(message.channel, 'Peace out guys! :v:') await client.logout() else: msg = '```ERROR: What the F**K do you think you\'re doing?!```' await client.send_message(message.channel, msg) #leave command elif message.content.startswith('{}leave'.format(cmd)): if message.author.id == '183790956754108416': await client.send_message(message.channel, 'Ok, I\'ll leave that server! :ok_hand:') await client.leave_server(discord.utils.get(client.servers)) else: msg = '```ERROR: What the F**K do you think you\'re doing?!```' await client.send_message(message.channel, msg) #invite command elif message.content.startswith('{}invite'.format(cmd)): msg = 'use this link to invite me to your server! :smiley:'\ '\n\nhttps://discordapp.com/oauth2/authorize?&client_id=223249543578255360&scope=bot&permissions=0'\ '\n\nNote from the Developer: I reserve the right to remove my bot from your server for any reason without any prior notice, enjoy my bot!'\ await client.send_message(message.channel, msg) #Mark command elif message.content.startswith('{}mark'.format(cmd)): msg = '```Mark is a nerd. He ain\'t got no balls.'\ '\n\nHis dick isn\'t big and his asshole\'s not small.'\ '\n\nThem weird ol voices make you wanna die.'\ '\n\nSingin\' pen pinapple until the day that I die.'\ '\n\nSingin\' pen pineapple until the day I die.```'\ await client.send_message(message.channel, msg) #Guess command elif message.content.startswith('{}guess'.format(cmd)): await client.send_message(message.channel, 'Guess a number between 1 to 10') def guess_check(m): return m.content.isdigit() guess = await client.wait_for_message(timeout=5.0, author=message.author, check=guess_check) answer = random.randint(1, 10) if guess is None: fmt = 'Sorry, you took too long. It was {}.' await client.send_message(message.channel, fmt.format(answer)) return if int(guess.content) == answer: await client.send_message(message.channel, 'You are right!') else: await client.send_message(message.channel, 'Sorry. It is actually {}.'.format(answer))
async def gif(ctx, *, message: str): """ Fetches gif """ img = translate(message, api_key=giphyKey) url = img.url await ctx.send(url)
async def on_ready(): print(f'{client.user} has connected to Discord!') user = await client.fetch_user('User ID') img = translate('love') await user.send(img.url) await user.send("Love you :heart:")
def __init__(self, phrase): self.img = translate(phrase, self.api_key)
### Post header info. open(post_path, 'w').close() postfile = open(post_path, 'a') postfile.write("Run Burgundy - Decentralized Fitness Group - Activity Log: " + str(today) + ".\n") ### This line is the title of the post postfile.write("fitnation running cycling hiking fitness\n") ### This line holds the tags postfile.write("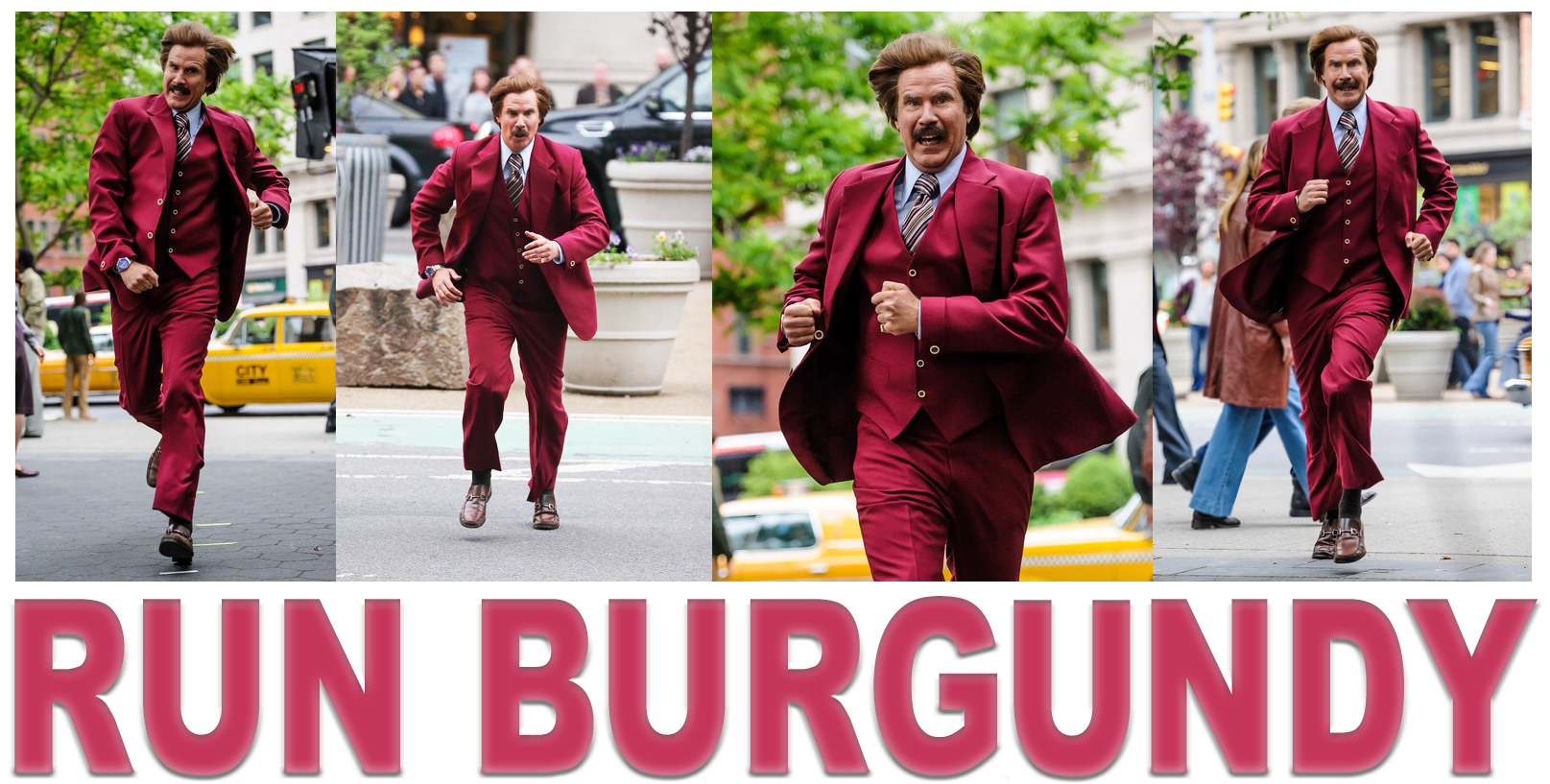\n") ### This line should be the header image ### Write something to pull custom weekly post from separate text file postfile.write("## <center>Welcome to Week No. 08, San Diego!</center>\n") postfile.write("### <center>If you like exercising, earning money, and getting the freshest #fitnation fitness news delivered to your eyeholes -- then I'm afraid you've come to the right place.</center>\n#### <center>We're now accepting members! Lets talk in the discord, or the comments below!</center>\n") postfile.write("<center></center>\n") postfile.write("### <center> ... the fitness team had oddly strong hearts.</center>\n How to join our fitness group:\n * Make yourself a (free!) account for athletics tracking at [Runalyze](https://runalyze.com) (open-source running / athletics analytics site);\n * Post some GPS tracked runs/bikes/hikes to your new Runalyze account; \n * Talk to @mstafford or @aussieninja to prove you're real;\n * Vote on these daily posts to increase their rewards;\n * At the end of each week, the SBD rewards get distributed based on kilometres travelled!\n") postfile.write("## <center>Neato, gang!</center>") # Gif and rewards summary below gif = translate('anchorman') postfile.write("<center></center>\n") postfile.write("<center></center>\n") postfile.write("#### Current rewards available for last weeks exercises = " + claimable + "!<br>This will be divided up amongst group members based on weekly kilometres ran & cycled!\n") ### Rewards from prev. week postfile.write("*Note that 1km of running is weighted the same as 3km of cycling!*\n") postfile.write("## Below is a summary of the groups runs/bikes/hikes as of " + str(today) + ".\n") postfile.write("*Weekly summary is from Monday->Sunday. Monthly summary is from the 1st to end of month.*\n") def get_dists(): run_day = 0.0 bike_day = 0.0 other_day = 0.0 run_week = 0.0 ##cumulative running km's for week bike_week = 0.0 ##cumulative biking km's for week other_week = 0.0 ##cumulative hiking (other) km's for week run_month = 0.0 ##cumulative running km's for month
print(tweet) print len(tweet) print(" ") print("Ready for new Tweet!") print(" ") try: while True: # nature_words() data = ser.readline().rstrip() data_s = data.split(":") x,y = data_s[0], data_s[1] y = int(y) if 20 <= y <= 399: adj = random.choice(ADJECTIVE) img = translate(adj, api_key='dc6zaTOxFJmzC') print(x, y, adj) print(img.url) print(img.bitly) tweet = "HELP! @larsgimse " + " I am " + adj + ". Need WATER now!! I can not dream of " + random.choice(COLOUR) + " " + random.choice(NOUN) + ". Nature: " + random.choice(nat_split) + " and " + random.choice(nat_split) + " " + img.bitly + " " + random.choice(HASTAG) if len(tweet) > 135: tweet = "HELP! @larsgimse " + " I am " + adj + ". Need WATER now!! I can not dream of " + random.choice(COLOUR) + " " + random.choice(NOUN) + ". Nature: " + random.choice(nat_split) + " and " + random.choice(nat_split) + " " + img.bitly + " " + random.choice(HASTAG) main() elif y > 400: adj2 = random.choice(ADJECTIVE2) img2 = translate(adj2, api_key='dc6zaTOxFJmzC') print(x, y, adj2) print(img2.url) print(img2.bitly) tweet = "I am " + adj2 + ". Need no water today. I dream of " + random.choice(COLOUR) + " " + random.choice(NOUN) + ". Nature: " + random.choice(nat_split) + " and " + random.choice(nat_split) + " " + img2.bitly + " " + random.choice(HASTAG) if len(tweet) > 135:
async def gifme(ctx, message): """ Just do !gifme (phrase) : Gives you a nice gif from giphy depending on the phrase. """ img = translate(message) await bot.say(img)
def test_translate_alias(self, giphy): giphy.return_value = giphy translate(term='foo', api_key='bar', strict=False, rating=None) giphy.assert_called_with(api_key='bar', strict=False) giphy.translate.assert_called_with(term='foo', phrase=None, rating=None)
def echo(): global LAST_UPDATE_ID # Request updates from last updated_id for update in bot.getUpdates(offset=LAST_UPDATE_ID): if LAST_UPDATE_ID < update.update_id: # chat_id is required to reply any message chat_id = update.message.chat_id message = update.message.text if (message): if '/start' in message or '/help' in message or '/list' in message or '/commands' in message: bot.sendChatAction(chat_id=chat_id, action=telegram.ChatAction.TYPING) bot.sendMessage(chat_id=chat_id, text=help.encode('utf8'), disable_web_page_preview=True) ##################----- PublicPlugins -----################## #Require API keys '''Youtube search''' if '/yt' in message or '/youtube' in message: bot.sendChatAction(chat_id=chat_id, action=telegram.ChatAction.TYPING) replacer = {'/youtube': '', '/yt': ''} search_term = replace_all(message, replacer) if len(search_term) < 1: bot.sendMessage( chat_id=chat_id, text= 'Youtube API calls are costly. Use it like /yt keywords; Ex, /yt Iron Maiden' ) else: bot.sendMessage( chat_id=chat_id, text=youtube(search_term).encode('utf8')) '''Twitter latest tweets of user''' if '/twitter' in message or '/tw' in message: bot.sendChatAction(chat_id=chat_id, action=telegram.ChatAction.TYPING) replacer = {'/twitter': '', '/tw': ''} username = replace_all(message, replacer) if len(username) < 1: bot.sendMessage( chat_id=chat_id, text='Use it like: /tw username; Ex, /tw pytacular' ) else: bot.sendMessage(chat_id=chat_id, text=twitter(username).encode('utf8')) '''Gets twitter trends by country /tt countryname, ex /tt India ''' if '/tt' in message: bot.sendChatAction(chat_id=chat_id, action=telegram.ChatAction.TYPING) replacer = {'/tt ': ''} place = replace_all(message, replacer) bot.sendMessage(chat_id=chat_id, text=twittertrends(place).encode('utf8')) '''Search twitter for top 4 related tweets. /ts #Privacy''' if '/ts' in message: bot.sendChatAction(chat_id=chat_id, action=telegram.ChatAction.TYPING) replacer = {'/ts ': ''} search_term = replace_all(message, replacer) bot.sendMessage( chat_id=chat_id, text=twittersearch(search_term).encode('utf8')) '''Instagram latest posts of user''' if '/insta' in message or '/instagram' in message: bot.sendChatAction(chat_id=chat_id, action=telegram.ChatAction.TYPING) bot.sendMessage( chat_id=chat_id, text= 'Instagram has restricted API access. This will not work anymore. Sorry :(' ) '''Game "Hot or Not" ''' if '/hon' in message or '/hotornot' in message: bot.sendChatAction(chat_id=chat_id, action=telegram.ChatAction.TYPING) custom_keyboard = [[ telegram.Emoji.THUMBS_UP_SIGN, telegram.Emoji.THUMBS_DOWN_SIGN ]] reply_markup = telegram.ReplyKeyboardMarkup( custom_keyboard, resize_keyboard=True, one_time_keyboard=True) image = imgur_hon() bot.sendMessage( chat_id=chat_id, text='Fetched from Imgur Subreddit r/models : ' + image, reply_markup=reply_markup) '''Bing Image Search''' if '/image' in message or '/img' in message: bot.sendChatAction(chat_id=chat_id, action=telegram.ChatAction.TYPING) replacer = {'/image': '', '/img': ''} search_term = replace_all(message, replacer) pic_link = bingsearch(search_term, 'Image') bot.sendMessage(chat_id=chat_id, text=pic_link) '''Microsoft translator''' if '/translate' in message: message = message.replace('/translate', '').encode('utf8') message_broken = shlex.split(message) error = 'Not enough parameters. Use, /translate en hi "Hello world" or /translate help to know more' if not len(message_broken) < 1: if message_broken[0] == 'help': help_string = """ Example, /translate en hi "Hello world" ar-Arabic | bs-Latn-Bosnian (Latin) | bg-Bulgarian | ca-Catalan | zh-CHS-Chinese Simplified | zh-CHT-Chinese Traditional|hr-Croatian | cs-Czech | da-Danish | nl-Dutch |en-English | cy-Welsh | et-Estonian | fi-Finnish | fr-French | de-German | el-Greek | ht-Haitian Creole | he-Hebrew | hi-Hindi | mww-Hmong Daw | hu-Hungarian | id-Indonesian | it-Italian | ja-Japanese | tlh-Klingon | tlh - Qaak-Klingon (pIqaD) | ko-Korean | lv-Latvian | lt-Lithuanian | ms-Malay | mt-Maltese | no-Norwegian | fa-Persian | pl-Polish | pt-Portuguese | otq-Querétaro Otomi | ro-Romanian | ru-Russian | sr-Cyrl-Serbian (Cyrillic) | sr-Latn-Serbian (Latin) | sk-Slovak | sl-Slovenian | es-Spanish | sv-Swedish | th-Thai | tr-Turkish | uk-Ukrainian | ur-Urdu | vi-Vietnamese | """ bot.sendMessage(chat_id=chat_id, text=help_string) else: if len(message_broken) < 3: bot.sendMessage(chat_id=chat_id, text=error) else: lang_from = message_broken[0] lang_to = message_broken[1] lang_text = message_broken[2] print(lang_from + lang_to + lang_text) bot.sendMessage(chat_id=chat_id, text=btranslate( lang_text, lang_from, lang_to)) else: bot.sendMessage(chat_id=chat_id, text=error) '''Random cat pic''' if '/cats' in message: bot.sendMessage( chat_id=chat_id, text='Hold on, digging out a random cat pic!') url = "http://thecatapi.com/api/images/get?api_key=" + cat_API_key + "&format=xml" xml_src = urllib.request.urlopen(url) data = xml_src.read() xml_src.close() data = xmltodict.parse(data) piclink = data['response']['data']['images']['image'][ 'url'] source_url = data['response']['data']['images']['image'][ 'source_url'] threadobjcats = UploadThread(bot, chat_id, piclink, caption=source_url) threadobjcats.setName('catsthread') threadobjcats.start() # Don't need an API key '''Google search''' if '/google' in message: bot.sendChatAction(chat_id=chat_id, action=telegram.ChatAction.TYPING) search_term = message.replace('/google', '') if len(search_term) < 1: bot.sendMessage( chat_id=chat_id, text='Use it like: /google what is a bot') else: bot.sendMessage(chat_id=chat_id, text=google(search_term)) '''Wikipedia search''' if '/wiki' in message: bot.sendChatAction(chat_id=chat_id, action=telegram.ChatAction.TYPING) search_term = message.replace('/wiki ', '') if len(search_term) < 1: bot.sendMessage(chat_id=chat_id, text='Use it like: /wiki Anaconda') else: reply = wiki(search_term) bot.sendMessage(chat_id=chat_id, text=reply) if ("Cannot acces link!" in reply): reply = "No wikipedia article on that but got some google results for you \n" + google( message) bot.sendMessage(chat_id=chat_id, text=reply) '''Weather by city,state''' if '/weather' in message: bot.sendChatAction(chat_id=chat_id, action=telegram.ChatAction.TYPING) reply = weather(message) bot.sendMessage(chat_id=chat_id, text=reply) '''Github public feed of any user''' if '/github' in message or '/gh' in message: bot.sendChatAction(chat_id=chat_id, action=telegram.ChatAction.TYPING) replacer = {'/github': '', '/gh': ''} username = replace_all(message, replacer) if len(username) < 1: bot.sendMessage( chat_id=chat_id, text= 'Use it like: /github username Ex, /github bhavyanshu or /gh bhavyanshu' ) else: bot.sendMessage(chat_id=chat_id, text=gitfeed(username)) '''Giphy to search for gif by keyword''' if '/giphy' in message or '/gif' in message: bot.sendChatAction(chat_id=chat_id, action=telegram.ChatAction.TYPING) replacer = {'/giphy': '', '/gif': ''} search_term = replace_all(message, replacer) if len(search_term) < 1: bot.sendMessage( chat_id=chat_id, text= 'Use it like: /giphy keyword ; Ex, /giphy cats or /gif cats' ) else: img = translate(search_term) print(img.fixed_height.downsampled.url) bot.sendMessage( chat_id=chat_id, text= 'Hang in there. Fetching gif..-Powered by GIPHY!') bot.sendChatAction( chat_id=chat_id, action=telegram.ChatAction.UPLOAD_PHOTO) threadobjgiphy = UploadThread( bot, chat_id, img.fixed_height.downsampled.url.encode('utf-8')) threadobjgiphy.setName('giphythread') threadobjgiphy.start() '''Basic calculator''' if '/calc' in message: head, sep, tail = message.partition('/') input_nums = tail.replace('calc', '') input_nums = input_nums.replace('\'', '') finalexp = shlex.split(input_nums) exp = finalexp[0] bot.sendChatAction(chat_id=chat_id, action=telegram.ChatAction.TYPING) error = 'You think I can compute apple+mongo? Don\'t add alphabet in between please. Use like, /calc 2+2-5(4+8)' if not exp: bot.sendMessage( chat_id=chat_id, text='Y u no type math expression? >.<') elif re.search('[a-zA-Z]', exp): bot.sendMessage(chat_id=chat_id, text=error) else: bot.sendMessage(chat_id=chat_id, text=calculate(exp)) # Updates global offset to get the new updates LAST_UPDATE_ID = update.update_id
res = wolf.request()["queryresult"] if res["success"] and res.get("pods"): answer = "" for pod in res["pods"]: if pod["subpods"][0]["plaintext"] and len(pod["subpods"][0]["plaintext"] + answer) < 110: answer += pod["subpods"][0]["plaintext"] + "\n" # tag questioner in response answer = "@" + status._json["user"]["screen_name"] + " " + answer print("ANSWER: " + answer) else: answer = "@" + status._json["user"]["screen_name"] + " " + giphypop.translate("confused").url api.update_status(status=answer, in_reply_to_status_id = status.id) #raise TweepError("RequestError: Wolfram|Alpha query was unsuccessful") except TweepError as err: print("TweepError: " + str(err)) print() break except KeyError as err: print("KeyError: " + str(err)) continue else: try:
def echo(): global LAST_UPDATE_ID # Request updates from last updated_id for update in bot.getUpdates(offset=LAST_UPDATE_ID): if LAST_UPDATE_ID < update.update_id: # chat_id is required to reply any message chat_id = update.message.chat_id message = update.message.text if (message): if '/start' in message or '/help' in message or '/list' in message or '/commands' in message: bot.sendChatAction(chat_id=chat_id, action=telegram.ChatAction.TYPING) bot.sendMessage(chat_id=chat_id,text=help.encode('utf8'),disable_web_page_preview=True) ##################----- PublicPlugins -----################## #Require API keys '''Youtube search''' if '/yt' in message or '/youtube' in message: bot.sendChatAction(chat_id=chat_id, action=telegram.ChatAction.TYPING) replacer = {'/youtube':'','/yt':''} search_term = replace_all(message,replacer) if len(search_term)<1: bot.sendMessage(chat_id=chat_id,text='Youtube API calls are costly. Use it like /yt keywords; Ex, /yt Iron Maiden') else: bot.sendMessage(chat_id=chat_id,text=youtube(search_term).encode('utf8')) '''Twitter latest tweets of user''' if '/twitter' in message or '/tw' in message: bot.sendChatAction(chat_id=chat_id, action=telegram.ChatAction.TYPING) replacer = {'/twitter':'','/tw':''} username = replace_all(message,replacer) if len(username)<1: bot.sendMessage(chat_id=chat_id,text='Use it like: /tw username; Ex, /tw pytacular') else: bot.sendMessage(chat_id=chat_id,text=twitter(username).encode('utf8')) '''Gets twitter trends by country /tt countryname, ex /tt India ''' if '/tt' in message: bot.sendChatAction(chat_id=chat_id, action=telegram.ChatAction.TYPING) replacer = {'/tt ':''} place = replace_all(message,replacer) bot.sendMessage(chat_id=chat_id,text=twittertrends(place).encode('utf8')) '''Search twitter for top 4 related tweets. /ts #Privacy''' if '/ts' in message: bot.sendChatAction(chat_id=chat_id, action=telegram.ChatAction.TYPING) replacer = {'/ts ':''} search_term = replace_all(message,replacer) bot.sendMessage(chat_id=chat_id,text=twittersearch(search_term).encode('utf8')) '''Instagram latest posts of user''' if '/insta' in message or '/instagram' in message: bot.sendChatAction(chat_id=chat_id, action=telegram.ChatAction.TYPING) bot.sendMessage(chat_id=chat_id,text='Instagram has restricted API access. This will not work anymore. Sorry :(') '''Game "Hot or Not" ''' if '/hon' in message or '/hotornot' in message: bot.sendChatAction(chat_id=chat_id, action=telegram.ChatAction.TYPING) custom_keyboard = [[ telegram.Emoji.THUMBS_UP_SIGN, telegram.Emoji.THUMBS_DOWN_SIGN ]] reply_markup = telegram.ReplyKeyboardMarkup(custom_keyboard,resize_keyboard=True,one_time_keyboard=True) image = imgur_hon() bot.sendMessage(chat_id=chat_id,text='Fetched from Imgur Subreddit r/models : '+image, reply_markup=reply_markup) '''Bing Image Search''' if '/image' in message or '/img' in message: bot.sendChatAction(chat_id=chat_id,action=telegram.ChatAction.TYPING) replacer = {'/image':'','/img':''} search_term = replace_all(message,replacer) pic_link = bingsearch(search_term,'Image') bot.sendMessage(chat_id=chat_id,text=pic_link) '''Microsoft translator''' if '/translate' in message: message = message.replace('/translate','').encode('utf8') message_broken = shlex.split(message) error = 'Not enough parameters. Use, /translate en hi "Hello world" or /translate help to know more' if not len(message_broken)<1: if message_broken[0] == 'help': help_string = """ Example, /translate en hi "Hello world" ar-Arabic | bs-Latn-Bosnian (Latin) | bg-Bulgarian | ca-Catalan | zh-CHS-Chinese Simplified | zh-CHT-Chinese Traditional|hr-Croatian | cs-Czech | da-Danish | nl-Dutch |en-English | cy-Welsh | et-Estonian | fi-Finnish | fr-French | de-German | el-Greek | ht-Haitian Creole | he-Hebrew | hi-Hindi | mww-Hmong Daw | hu-Hungarian | id-Indonesian | it-Italian | ja-Japanese | tlh-Klingon | tlh - Qaak-Klingon (pIqaD) | ko-Korean | lv-Latvian | lt-Lithuanian | ms-Malay | mt-Maltese | no-Norwegian | fa-Persian | pl-Polish | pt-Portuguese | otq-Querétaro Otomi | ro-Romanian | ru-Russian | sr-Cyrl-Serbian (Cyrillic) | sr-Latn-Serbian (Latin) | sk-Slovak | sl-Slovenian | es-Spanish | sv-Swedish | th-Thai | tr-Turkish | uk-Ukrainian | ur-Urdu | vi-Vietnamese | """ bot.sendMessage(chat_id=chat_id,text=help_string) else: if len(message_broken)<3: bot.sendMessage(chat_id=chat_id,text=error) else: lang_from = message_broken[0] lang_to = message_broken[1] lang_text = message_broken[2] print lang_from+lang_to+lang_text bot.sendMessage(chat_id=chat_id,text=btranslate(lang_text,lang_from,lang_to)) else: bot.sendMessage(chat_id=chat_id,text=error) '''Random cat pic''' if '/cats' in message: bot.sendMessage(chat_id=chat_id,text='Hold on, digging out a random cat pic!') url = "http://thecatapi.com/api/images/get?api_key="+cat_API_key+"&format=xml" xml_src = urllib2.urlopen(url) data = xml_src.read() xml_src.close() data = xmltodict.parse(data) piclink = data['response']['data']['images']['image']['url'] source_url = data['response']['data']['images']['image']['source_url'] threadobjcats = UploadThread(bot,chat_id,piclink,caption=source_url) threadobjcats.setName('catsthread') threadobjcats.start() # Don't need an API key '''Google search''' if '/google' in message: bot.sendChatAction(chat_id=chat_id, action=telegram.ChatAction.TYPING) search_term = message.replace('/google','') if len(search_term)<1: bot.sendMessage(chat_id=chat_id,text='Use it like: /google what is a bot') else: bot.sendMessage(chat_id=chat_id,text=google(search_term)) '''Wikipedia search''' if '/wiki' in message: bot.sendChatAction(chat_id=chat_id, action=telegram.ChatAction.TYPING) search_term = message.replace('/wiki ','') if len(search_term)<1: bot.sendMessage(chat_id=chat_id,text='Use it like: /wiki Anaconda') else: reply=wiki(search_term) bot.sendMessage(chat_id=chat_id,text=reply) if ("Cannot acces link!" in reply): reply="No wikipedia article on that but got some google results for you \n"+google(message) bot.sendMessage(chat_id=chat_id,text=reply) '''Weather by city,state''' if '/weather' in message: bot.sendChatAction(chat_id=chat_id, action=telegram.ChatAction.TYPING) reply=weather(message) bot.sendMessage(chat_id=chat_id,text=reply) '''Github public feed of any user''' if '/github' in message or '/gh' in message: bot.sendChatAction(chat_id=chat_id,action=telegram.ChatAction.TYPING) replacer = {'/github':'','/gh':''} username = replace_all(message,replacer) if len(username)<1: bot.sendMessage(chat_id=chat_id,text='Use it like: /github username Ex, /github bhavyanshu or /gh bhavyanshu') else: bot.sendMessage(chat_id=chat_id,text=gitfeed(username)) '''Giphy to search for gif by keyword''' if '/giphy' in message or '/gif' in message: bot.sendChatAction(chat_id=chat_id, action=telegram.ChatAction.TYPING) replacer = {'/giphy':'','/gif':''} search_term = replace_all(message,replacer) if len(search_term)<1: bot.sendMessage(chat_id=chat_id,text='Use it like: /giphy keyword ; Ex, /giphy cats or /gif cats') else: img = translate(search_term) print img.fixed_height.downsampled.url bot.sendMessage(chat_id=chat_id,text='Hang in there. Fetching gif..-Powered by GIPHY!') bot.sendChatAction(chat_id=chat_id, action=telegram.ChatAction.UPLOAD_PHOTO) threadobjgiphy = UploadThread(bot,chat_id,img.fixed_height.downsampled.url.encode('utf-8')) threadobjgiphy.setName('giphythread') threadobjgiphy.start() '''Basic calculator''' if '/calc' in message: head, sep, tail = message.partition('/') input_nums = tail.replace('calc','') input_nums = input_nums.replace('\'','') finalexp = shlex.split(input_nums) exp = finalexp[0] bot.sendChatAction(chat_id=chat_id, action=telegram.ChatAction.TYPING) error = 'You think I can compute apple+mongo? Don\'t add alphabet in between please. Use like, /calc 2+2-5(4+8)' if not exp: bot.sendMessage(chat_id=chat_id,text='Y u no type math expression? >.<') elif re.search('[a-zA-Z]', exp): bot.sendMessage(chat_id=chat_id,text=error) else: bot.sendMessage(chat_id=chat_id,text=calculate(exp)) # Updates global offset to get the new updates LAST_UPDATE_ID = update.update_id
def giffy(message): img = translate(message, api_key='dc6zaTOxFJmzC') gif = img.fixed_height.url print(gif) return gif