import pandas as pd import numpy as np from wordcloud import WordCloud import matplotlib.pyplot as plt import re import string import requests from sklearn.feature_extraction.text import TfidfVectorizer # Create some sample text text = 'Fun, fun, awesome, awesome, tubular, astounding, superb, great, amazing, amazing, amazing, amazing' st.title("Assalamu 'alaikum ") cari = st.text_input("Cari Produk/Restaurant Halal", "soto ") # display the name when the submit button is clicked # .title() is used to get the input text string if (st.button('Submit')): result = cari.title() if (result): st.success(result) else: st.error("Masukkan input ya") df = pd.DataFrame(np.random.randn(5, 4), columns=('col %d' % i for i in range(4))) st.image("produk.png", width=None) st.write("Top 5 Halal")
def spell(spell_inputs): mana = spell_inputs highlight_choices = [ "Max", "Min", "Null", "Equals", "Less Than", "Greater Than", "Heatmap", "Contains", ] highlight_choice = st.selectbox("Select highlight option", highlight_choices) mana_clone = pd.DataFrame(index=mana.index, columns=mana.columns) if highlight_choice == "Max": st.dataframe(mana.style.highlight_max(axis=0)) if highlight_choice == "Min": st.dataframe(mana.style.highlight_min(axis=0)) if highlight_choice == "Null": try: st.dataframe(mana.style.highlight_null(axis=0)) except: st.success("No NULL values") st.write(mana) if highlight_choice == "Heatmap": st.dataframe(mana.style.background_gradient(axis=0)) if highlight_choice == "Greater Than": what_cols = st.multiselect("Columns", mana.columns) if len(what_cols) > 0: threshold = st.number_input("Value: ", 1.0) st.dataframe( mana.style.apply( highlight_greaterthan, threshold=threshold, column=what_cols, axis=1, mana_clone=mana_clone, )) if highlight_choice == "Less Than": what_cols = st.multiselect("Columns", mana.columns) if len(what_cols) > 0: threshold = st.number_input("Value: ", 1.0) st.dataframe( mana.style.apply( highlight_lessthan, threshold=threshold, column=what_cols, axis=1, mana_clone=mana_clone, )) if highlight_choice == "Equals": equal_choices = ["Number", "Text"] equal_choice = st.selectbox("Select equals option", equal_choices) what_cols = st.multiselect("Columns", mana.columns) if len(what_cols) > 0: if equal_choice == "Number": threshold = st.number_input("Value: ", 1.0) if equal_choice == "Text": threshold = st.text_input("Value: ", "") st.dataframe( mana.style.apply( highlight_equals, threshold=threshold, column=what_cols, axis=1, mana_clone=mana_clone, )) if highlight_choice == "Contains": what_cols = st.multiselect("Columns", mana.columns) if len(what_cols) > 0: threshold = st.text_input("Value: ", "") st.dataframe( mana.style.apply( highlight_contains, threshold=threshold, column=what_cols, axis=1, mana_clone=mana_clone, )) return None, mana
def run_lda(): st.header("Latent Dirichlet Allocation (LDA)") example = "https://impact.jpmorganchase.com/content/dam/jpmc/jpmorgan-chase-and-co/documents/jpmc-cr-esg-report-2019.pdf" url_report = st.text_input("Enter url of esg report", example) # make html st.subheader("Topic distribution from trained LDA model") HtmlFile = open("output/output_filename.html", 'r', encoding='utf-8') source_code = HtmlFile.read() components.html(source_code, height=800) # load word vectorizer filename_count = 'trained_models/CountVectorizer.sav' word_tf_vectorizer = joblib.load(filename_count) # load lda model filename_lda = 'trained_models/lda_fitted.sav' lda_model = joblib.load(filename_lda) nlp = preprocess.load_spacy_model() # preprocess data = preprocess_wrapper(url=url_report, open_pdf_file=None, make_sentence=False) df1 = pd.DataFrame(data, columns=["text"]) df1["lem"] = df1['text'].apply(preprocess.lemmatize, nlp=nlp) # predict transformed = predict(data=df1["lem"], word_vec=word_tf_vectorizer, lda=lda_model) # topic with highest probability # add 1 (start otherwise at 0) a = [np.argmax(distribution) + 1 for distribution in transformed] # with associated probability b = [np.max(distribution) for distribution in transformed] # consolidate LDA output into a handy dataframe df1["nr_words"] = df1["lem"].apply(lambda x: len(x.split())) df2 = pd.DataFrame(zip(a, b, transformed), columns=['topic', 'probability', 'probabilities']) esg_group = pd.concat([df1, df2], axis=1) # topic distribution plot_topic_dist(predictions=transformed, df=df1) # display dataframe with st.beta_expander("Expand results"): st.table(esg_group[["lem", "topic", "probability"]]) # topic probabilities with st.beta_expander("Expand topic distribution"): fig = px.histogram(esg_group, x="probability") st.plotly_chart(fig, use_container_width=True) # Detail results for a topic with st.beta_expander("Expand statement per topic"): topic_nr = st.number_input("Give topic number", min_value=1, max_value=10, value=6) esg_subset = esg_group[esg_group.topic == topic_nr].sort_values( by='probability', ascending=False) st.table(esg_subset[["lem", "topic", "probability"]]) # Highest probability statement for each topic with st.beta_expander( "Expand highest probability statement for each topic"): a = esg_group.loc[esg_group.groupby('topic')['probability'].idxmax()] st.table(a[["lem", "topic", "probability"]])
import streamlit as st st.header(':computer: 测试报告工具') nl = [5, 8, 3] for j in range(3): with st.beta_expander('测试指标 ' + str(j + 1), expanded=True): cl1 = [] for i in range(nl[j]): if not st.checkbox( '测试指标 ' + str(j + 1) + '.' + str(i + 1) + ' xxx 符合测试要求', value=True, key=i): k = st.number_input('输入issue个数', min_value=0, value=1, step=1, key=(i + 1) * 1000) for ki in range(k): st.text_input('填写issue ' + str(j + 1) + '.' + str(i + 1) + '.' + str(ki + 1) + ' 名称', key=(i + 1) * 1000 + ki * 100 + 1) st.text_area('填写issue ' + str(j + 1) + '.' + str(i + 1) + '.' + str(ki + 1) + ' 描述', key=(i + 1) * 1000 + ki * 100 + 1) st.file_uploader('上传issue ' + str(j + 1) + '.' + str(i + 1) + '.' + str(ki + 1) + ' 对应图片', type=None, accept_multiple_files=True, key=(i + 1) * 1000 + ki * 100 + 1)
def dataViz(): # Set up tkinter # root = tk.Tk() # root.withdraw() # # Make folder picker dialog appear on top of other windows # root.wm_attributes('-topmost', 1) st.title('Exploratory Data Analysis') # for maintaining session for multiple button or inputs in same page # session_state = SessionState.get(name="", button_sent=False) # button_sent = st.button("SUBMIT") # if button_sent or session_state.button_sent: # <-- first time is button interaction, next time use state to go to multiselect # session_state.button_sent = True # listnames = n.show_names(name) # selectednames=st.multiselect('Select your names',listnames) # st.write(selectednames) st.write('Please select train folder:') # clicked1 = st.button('Train Folder Picker') # if clicked1 or session_state.clicked1 : # session_state.clicked1 = True # trainPath = st.text_input('Selected folder:', filedialog.askdirectory(master=root)) # st.write(trainPath) # st.write('Please select test folder:') # clicked2 = st.button('Test Folder Picker') # if clicked2 or session_state.clicked2: # session_state.clicked2 = True # testpath = st.text_input('Selected folder:', filedialog.askdirectory(master=root)) # st.write(testpath) # st.write('Please select train annotation file:') # clicked3 = st.button('Train annotation file Picker') # if clicked3 or session_state.clicked3: # session_state.clicked3 = True # annoTrainPath = st.text_input('Selected file:', filedialog.askopenfilename(master=root)) # st.write(annoTrainPath) # st.write('Please select test annotation file:') # clicked4 = st.button('Test annotation file Picker') # if clicked4 or session_state.clicked4: # session_state.clicked4 = True # annoTestPath = st.text_input('Selected file:', filedialog.askopenfilename(master=root)) # st.write(annoTestPath) trainPath = st.text_input( 'Train Folder:', 'E:\GreatLearning\Capstone_Project\CapstoneProject_GL\data\Car Images-20210501T094840Z-001\Car Images\Train Images' ) testPath = st.text_input( 'Test Folder:', 'E:\GreatLearning\Capstone_Project\CapstoneProject_GL\data\Car Images-20210501T094840Z-001\Car Images\Test Images' ) annoTrainPath = st.text_input( 'Train annotation file:', 'E:\GreatLearning\Capstone_Project\CapstoneProject_GL\data\Annotations-20210510T185520Z-001\Annotations\Train Annotations.csv' ) annoTestPath = st.text_input( 'Test annotation file:', 'E:\GreatLearning\Capstone_Project\CapstoneProject_GL\data\Annotations-20210510T185520Z-001\Annotations\Test Annotation.csv' ) clicked1 = st.sidebar.button('Start EDA') if clicked1: #st.write('Train Selected folder:',trainPath) #st.write('Test Selected folder:',testPath) #st.write('Train Selected annotation file:',annoTrainPath) #st.write('Test Selected annotation file:',annoTestPath) eda.process(trainPath, testPath, annoTrainPath, annoTestPath)
def write(): import streamlit as st #datetime is imported so that the user's [entry, date] pair can be saved from datetime import datetime import nltk as nltk import joblib import math as math nltk.download('vader_lexicon') from nltk.sentiment.vader import SentimentIntensityAnalyzer import pandas as pd sid = SentimentIntensityAnalyzer() import scipy import torch import re #import sklearn from scipy import spatial from sentence_transformers import SentenceTransformer @st.cache(allow_output_mutation=True) def load_my_model(): model = SentenceTransformer('distilbert-base-nli-mean-tokens') return model from transformers import pipeline #@st.cache(allow_output_mutation=True) #def load_classifier(): # classifier = pipeline('sentiment-analysis') # return classifier @st.cache(allow_output_mutation=True) def load_isear(): isear = pd.read_csv("isear_embed.csv") isear = isear.drop("index", axis=1) return isear @st.cache def analysis(sentence): model = load_my_model() lis = list() m = sid.polarity_scores(sentence) score = m['compound'] a = re.split("[.!?;\n]", sentence) if len(a) > 2: b = a[len(a) - 2] + ". " + a[len(a) - 1] c = sid.polarity_scores(b) score = c['compound'] if len(a) > 3: b = a[len(a) - 3] + ". " + a[len(a) - 2] + ". " + a[len(a) - 1] c = sid.polarity_scores(b) score2 = c['compound'] else: score2 = 0 EHS = pd.read_csv("EHS.csv") sentence_embeddings = EHS.values.tolist() OPTO = pd.read_csv("OPTO.csv") optimistic_embeddings = OPTO.values.tolist() #should check what happens when i do .values.tolist() a nd why i do it isear = load_isear() isear_list = isear.values.tolist() booleon = 0 a_embeddings = model.encode(a) for j in range(len(a_embeddings)): for i in range(len(sentence_embeddings)): result = 1 - spatial.distance.cosine(sentence_embeddings[i], a_embeddings[j]) if result > .8: booleon = booleon - 1 #print(a[j]) #st.write('You sound helpless, this sentence concerned me:', a[j]) break for j in range(len(a_embeddings)): for i in range(len(optimistic_embeddings)): result = 1 - spatial.distance.cosine(optimistic_embeddings[i], a_embeddings[j]) if result > .8: booleon = booleon + 1 break rent = (booleon / len(a_embeddings)) isear_feature = 0 for j in range(len(a_embeddings)): for i in range(len(isear_list)): result = 1 - spatial.distance.cosine(isear_list[i], a_embeddings[j]) if result >= .8: isear_feature = isear_feature - 1 break hugscore = 0 classifier = pipeline('sentiment-analysis') if len(a) > 3: for i in range(0, len(a)): result = classifier(a[i]) result = pd.DataFrame(result) if str(result["label"]).count("POS") > 0: hugscore = hugscore + result['score'] if str(result["label"]).count("NEG") > 0: hugscore = hugscore - result['score'] hugscore = hugscore / len(a) hugscore = float(hugscore) lis.append([rent, isear_feature, score, score2, hugscore]) return lis sentence = st.text_area("what's on your mind?") #button = st.button() #the reason score is compute here and not inside st.button("analysis") is because now it'll be saved rather than refreshed if another button gets pressed #basically, variables inside a button aren't available outside of them. #need to append more than that to the list to get meaningful data out of this. if len(sentence) > 1: if sentence.count(".") == 0: st.write("Write more!") else: df = analysis(sentence) df = pd.DataFrame(df) df.columns = [ "rent", "isear_feature", "score", "score3", "hugscore" ] loaded_model = joblib.load("GradientBoostedClassifier90CV.sav") result = loaded_model.predict(df) if result[0] == 0: score = "pessimistic" booleon = -3 if result[0] == 1: score = "neutral" booleon = 0 if result[0] == 2: score = "optimistic" booleon = 3 #try: # lis.append([df[0]]) #except: # lis = list() # lis.append([df[0]]) #need to revise output. Output should be a page of resources with a gif on top. if st.button('Analysis'): #gonna change this to if sentence.count(x) + count(y) .... < 5, then ask them to write more. #the model does poorly on samples less than 5 sentences if len(sentence) > 1: if sentence.count(".") + sentence.count("!") + sentence.count( "?") < 5: st.write( "I'm not smart enough to analyze this without more sentences :(" ) st.markdown( "" ) elif sentence.count("..") + sentence.count("!!") > 2: st.write( "I can't analyze entries right now that have abnormal punctuation. Feel free to change your punctuation and try again." ) st.markdown( "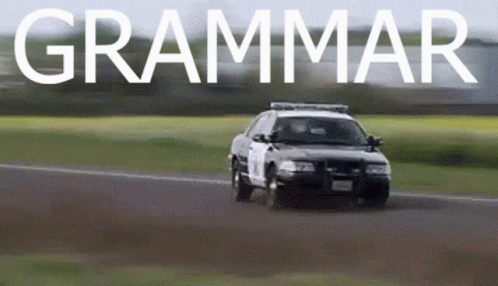" ) else: st.write("you're feeling : " + score) if score == "pessimistic": st.write("That's fine. Let it all out.") st.markdown( "" ) st.write( "Check out the resources tab to see how you can 'learn' optimism" ) st.markdown( "[Click here if you need extra help](https://suicidepreventionlifeline.org/chat/)" ) if score == "neutral": st.write( "You're just chilling. Waiting on some stuff to play out. It be like that sometimes." ) st.markdown( "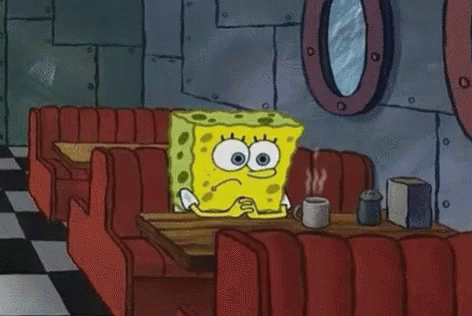" ) if score == "optimistic": st.write("You are a ray of sunshine today! Keep it up!") st.markdown( "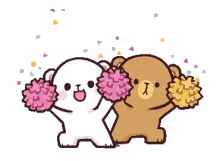" ) #st.header("Insert your username below to save your score") username = st.text_input( "Username (required for you to save your score & see your day-to-day changes): " ) today = datetime.now() #st.text_input doesn't work inside the st.button()....gotta figure out why #^above is an old note, i know why now, I just keep it there to remind me that inside button actions are way diff than outside button actions if st.button('Save my score'): import csv fields = [result[0], sentence, today] try: test = open(username + ".csv", 'r') with open(username + ".csv", 'a') as f: writer = csv.writer(f) writer.writerow(fields) except FileNotFoundError: with open(username + ".csv", 'a') as f: writer = csv.writer(f) writer.writerow(["score", "sentence", "date"]) writer.writerow(fields)
#creating the UI of model using strealit import tensorflow as tf from tensorflow.keras.preprocessing.sequence import pad_sequences from tokenizer import tokenizer , max_lenght , trunc_type import streamlit as st import numpy as np st.title("Movie Review Sentiment Analysis") st.write("This ML model will guess if a given review is positive or negative by using NLP. " "This model was trained using Tensorflow and was trained on the imdb-dataset of movie reviews.") with st.spinner("Loading Model....."): new_model = tf.keras.models.load_model('model/sentiment-analysis-model.h5') pred_review_text = st.text_input("Enter your review") if pred_review_text != '': pred = [] pred.append(pred_review_text) with st.spinner("Tokenizing Text....."): pred_seq = tokenizer.texts_to_sequences(pred) pred_padded = pad_sequences(pred_seq, maxlen=max_lenght, truncating=trunc_type) val = new_model.predict(pred_padded) new_val = str(val) st.subheader("The given review was : ") if val > [[0.5]]: st.write("Positive") else: st.write("Negative")
def main(): st.title('Social and Information Networks') st.subheader('Data analysis for Indian Politics') st.sidebar.title('Menu') menuItems = [ 'DashBoard', 'Induvidual Analyser', 'Politicians Data', 'Trends' ] item = st.sidebar.selectbox('', menuItems) hide_streamlit_style = """ <style> #MainMenu {visibility: hidden;} footer {visibility: hidden;} </style> """ st.markdown(hide_streamlit_style, unsafe_allow_html=True) github = '''[ Fork/Star on Github](https://github.com/abhayrpatel10/spartan)''' st.sidebar.info(github) if item == 'DashBoard': s = ''' The data is extracted from the various social media platform is assigned a sentiment score.The technique used to assign the score is VADER (Valence Aware Dictionary and sentiment Reasoner).The sentiment score is calculated from a lexicon rule based dictionary and the data is plotted for visual representation ### Workflow Extracting data from social media - data mostly from twitter.(The data extracted is from end of 2017 to 2018 just before 2019 Indian elections) The data was filterer using keywords like India,Indian politics,bj,congress,ncp,nda,inc,election2019 The data was cleaned - removal of links,stopwords Each tweet was asigned a sentiment score The graphs shown is the result of grouping keywords and the sentiment score ''' st.markdown(s) keyword = st.text_input('Enter a political keyword', 'bjp') x, y = tweets_class(filter_tweets(keyword)) ax = plt.bar(y, x) autolabel(ax) st.write(mpl_fig=ax) st.pyplot() startingRadius = 0.7 + (0.3 * (len(x) - 1)) for i in range(0, len(x)): scenario = y[i] percentage = x[i] textLabel = scenario + ' ' + str(percentage) print(startingRadius) remainingPie = 100 - percentage donut_sizes = [remainingPie, percentage] plt.text(0.04, startingRadius + 0.07, textLabel, horizontalalignment='center', verticalalignment='center') plt.pie(donut_sizes, radius=startingRadius, startangle=90, colors=['#d5f6da', '#5cdb6f'], wedgeprops={ "edgecolor": "white", 'linewidth': 6 }) startingRadius -= 0.3 # equal ensures pie chart is drawn as a circle (equal aspect ratio) plt.axis('equal') # create circle and place onto pie chart circle = plt.Circle(xy=(0, 0), radius=0.35, facecolor='white') plt.gca().add_artist(circle) st.pyplot() elif item == 'Induvidual Analyser': name = st.text_input('Name', 'narendramodi') getProfile(name) elif item == 'Location based analyser': f = open('data.geojson') st.map(f) elif item == 'Politicians Data': df = pd.read_csv('term-16.csv') df = df.drop([ 'sort_name', 'twitter', 'id', 'facebook', 'term', 'start_date', 'end_date', 'image', 'gender', 'wikidata', 'wikidata_group', 'wikidata_area' ], axis=1) st.table(df) elif item == 'Trends': trends()
def main(): st.title("Credit Card Defaulter Prediction App") html_temp = """ <div style="background-color:#025246 ;padding:10px"> <h2 style="color:white;text-align:center;">Are you a defaulter? </h2> </div> """ page_bg_img = ''' <style> body { background-image: url("https://images.unsplash.com/photo-1589758438368-0ad531db3366?ixlib=rb-1.2.1&ixid=eyJhcHBfaWQiOjEyMDd9&auto=format&fit=crop&w=889&q=80"); background-size: cover; } </style> ''' st.markdown(html_temp, unsafe_allow_html=True) st.markdown(page_bg_img, unsafe_allow_html=True) a = st.text_input("BALANCE LIMIT", "Type Here") b = st.text_input("SEX", "Type Here") c = st.text_input("AGE", "Type Here") d = st.text_input("BILL AMT1", "Type Here") e = st.text_input("BILL AMT 2", "Type Here") f = st.text_input("BILL AMT 3", "Type Here") g = st.text_input("BILL AMT 4", "Type Here") h = st.text_input("BILL AMT 5", "Type Here") i = st.text_input("BILL AMT 6", "Type Here") j = st.text_input("PAY AMT 1 ", "Type Here") k = st.text_input("PAY AMT 2", "Type Here") l = st.text_input("PAY AMT 3", "Type Here") m = st.text_input("PAY AMT 4", "Type Here") n = st.text_input("PAY AMT 5", "Type Here") o = st.text_input("PAY AMT 6", "Type Here") safe_html = """ <div style="background-color:#F4D03F;padding:10px > <h2 style="color:white;text-align:center;">Congratulations! You have successfully managed to maintain minimum balance </h2> </div> """ danger_html = """ <div style="background-color:#F08080;padding:10px > <h2 style="color:black ;text-align:center;">Alert! You are below minimum balance.</h2> </div> """ if st.button("Predict"): output = predict_disease(a, b, c, d, e, f, g, h, i, j, k, l, m, n, o) st.success('The probability is {}'.format(output * 100)) if output == 1: st.markdown(danger_html, unsafe_allow_html=True) else: st.markdown(safe_html, unsafe_allow_html=True)
import pandas as pd # Data wrangling, Data Frame. import streamlit as st import pyodbc # import os.path # from os import path # import sys # from cmd import Cmd import subprocess import os from subprocess import Popen #### ---------------------------------#### st.title('Samples') #-------------Input Section ------------------------- Model_input = st.text_input('Model Input', 'Input model here !!!') #-------------Connection Section------------------- # def conn_server(): conn = pyodbc.connect( 'DRIVER={ODBC Driver 17 for SQL Server};SERVER=vnmsrv601.dl.net\Siplace_2008R2EX;DATABASE=MobileDB;UID=Sa;PWD=Siplace.1' ) sql = f"SELECT top 5 * FROM LabelcodeFruPP where Model ='{Model_input}'" #st.write(sql) df = pd.read_sql(sql, conn) # model ='890003434' #st.write(conn,"Connection OK") if Model_input != '': if len(df) > 0:
"<h1 style='text-align: center; '>Covid-19 search engine</h1>", unsafe_allow_html=True, ) st.markdown("<h2 style='text-align: center; '>Stay safe</h2>", unsafe_allow_html=True) # Logo logo_path = os.path.join(os.path.dirname(os.path.abspath(__file__)), "facemask.jpg") robeco_logo = Image.open(logo_path) st.image(robeco_logo, use_column_width=True) # Search bar search_query = st.text_input("Search for Covid-19 here", value="", max_chars=None, key=None, type="default") # Search API index_name = "covid-19-index" endpoint = os.environ["ACS_ENDPOINT"] credential = os.environ["ACS_API_KEY"] headers = { "Content-Type": "application/json", "api-key": credential, } search_url = f"{endpoint}/indexes/{index_name}/docs/search?api-version=2020-06-30" search_body = { "count": True,
st.markdown( '* The **Client-specific** dashboard lets you get a credit risk prediction for a specific client and provides insights into the prediction.' ) dashboard_page = st.selectbox( 'Please select a dashboard below:', options=['', 'Global Dashboard', 'Client-Specific Dashboard']) if dashboard_page == '': pass elif dashboard_page == 'Global Dashboard': top_20_credit_requests() target_amounts() else: # Print out sample IDs example_ids() # Get client ID client_id = st.text_input('Client ID:') if client_id == '': st.write('Please enter a Client ID.') else: if int(client_id) not in valid_ids: st.markdown( ':exclamation: This ID does not exist. Please enter a valid one.' ) else: # Generate filtered dataset df_small = filter_dataset() # Get prediction get_prediction() with st.spinner( 'Loading prediction details. This may take a few minutes.' ):
f"Test #{sample[2]}: {sample[3]}" + f" - Output \'{sample[1]} ({triangle.type_of_triangle[sample[1]]})\'" + f" is expected to be \'{int(sample[0])} ({triangle.type_of_triangle[sample[0]]})\'" ) st.header("Analysis") labels = 'pass', 'fail' sizes = [n_right, n_wrong] plt.pie(sizes, labels=labels, autopct='%1.1f%%', startangle=90) plt.axis('equal') st.set_option('deprecation.showPyplotGlobalUse', False) st.pyplot() if option3 == 'Input via textfield': st.write(triangle.type_of_triangle) sample_input = st.text_input( 'Input your own test samples. For Example: 1,2,4:0', ' ') real_cols = [ "side 1", "side 2", "side 3", "Expected", "Ground truth" ] real_sample_input = re.split('[,:]', sample_input) real_sample_input = np.array([float(x) for x in real_sample_input]) do_right, real_value, test_value = triangle.is_right( real_sample_input, triangle.decide_triangle_type) real_sample_input = np.append(real_sample_input, float(test_value)) print(real_sample_input) new_sample = pd.DataFrame(real_sample_input.reshape((1, -1)), columns=real_cols) st.table(new_sample) #万年历
plt.axis("off") plt.show() st.title("COP 4813 - Web Application Programming") st.header("Project 1") st.subheader("Part A - The Stories API") st.write("This app uses the Top Stories API to display the most common words used in the top current articles " "based on a specified topic selected by the user. The data is displayed as a line chart and as a line " "and as a wordcloud image.") st.write("I - Topic Selection") user_name = st.text_input("Please enter your name") options = st.selectbox("Select a topic of your interest", ["", "Arts", "Automobiles", "Books", "Business", "Fashion", "Food", "Health", "Home", "Insider", "Magazine", "Movies", "NY Region", "Obituaries", "Opinion", "Politics", "Real Estate", "Science", "Sports", "Sunday Review", "Technology", "Theater", "T-Magazine", "Travel", "Upshot", "US", "World"]) if user_name and options: st.write("Hi " + user_name + ", you selected {} as your topic of interest.".format(options)) def partA(): response2 = requests.get(new_url).json() main_functions.save_to_file(response2, "JSON_Files/response.json") if user_name and options:
'YlGn_r', 'YlOrBr', 'YlOrBr_r', 'YlOrRd', 'YlOrRd_r', 'afmhot', 'afmhot_r', 'autumn', \ 'autumn_r', 'binary', 'binary_r', 'bone', 'bone_r', 'brg', 'brg_r', 'bwr', 'bwr_r', \ 'cividis', 'cividis_r', 'cool', 'cool_r', 'coolwarm', 'coolwarm_r', 'copper', 'copper_r', \ 'cubehelix', 'cubehelix_r', 'flag', 'flag_r', 'gist_earth', 'gist_earth_r', 'gist_gray', \ 'gist_gray_r', 'gist_heat', 'gist_heat_r', 'gist_ncar', 'gist_ncar_r', 'gist_rainbow', \ 'gist_rainbow_r', 'gist_stern', 'gist_stern_r', 'gist_yarg', 'gist_yarg_r', 'gnuplot', \ 'gnuplot2', 'gnuplot2_r', 'gnuplot_r', 'gray', 'gray_r', 'hot', 'hot_r', 'hsv', 'hsv_r', \ 'inferno', 'inferno_r', 'jet', 'jet_r', 'magma', 'magma_r', 'nipy_spectral', 'nipy_spectral_r',\ 'ocean', 'ocean_r', 'pink', 'pink_r', 'plasma', 'plasma_r', 'prism', 'prism_r', 'rainbow',\ 'rainbow_r', 'seismic', 'seismic_r', 'spring', 'spring_r', 'summer', 'summer_r', 'tab10', \ 'tab10_r', 'tab20', 'tab20_r', 'tab20b', 'tab20b_r', 'tab20c', 'tab20c_r', 'terrain', 'terrain_r', \ 'twilight', 'twilight_r', 'twilight_shifted', 'twilight_shifted_r', 'viridis', 'viridis_r', 'winter', 'winter_r']) if fil == 'None': img = img[crop_h[0]:crop_h[1]:blur, crop_w[0]:crop_w[1]:blur] plt.imshow(img) else: img = img[crop_h[0]:crop_h[1]:blur, crop_w[0]:crop_w[1]:blur, 0] plt.imshow(img, cmap=fil) st.pyplot() img_name = st.text_input('Choose a name for your picture:', 'MyCoolPic.jpg') save = st.button('Save My Image!') if save and fil == 'None': plt.imsave(img_name, img) st.success('Image saved!') elif save: plt.imsave(img_name, img, cmap=fil) st.success('Image saved!')
if max_faces > 0: # select interested face in picture face_idx = st.selectbox("Select face#", range(max_faces)) roi = rois[face_idx] st.image(BGR_to_RGB(roi), width=min(roi.shape[0], 300)) # initial database for known faces DB = init_data() face_encodings = DB[COLS_ENCODE].values dataframe = DB[COLS_INFO] # compare roi to known faces, show distances and similarities face_to_compare = face_recognition.face_encodings(roi)[0] dataframe['distance'] = face_recognition.face_distance( face_encodings, face_to_compare) dataframe['similarity'] = dataframe.distance.apply( lambda distance: f"{face_distance_to_conf(distance):0.2%}") st.dataframe( dataframe.sort_values("distance").iloc[:5].set_index('name')) # add roi to known database if st.checkbox('add it to knonwn faces'): face_name = st.text_input('Name:', '') face_des = st.text_input('Desciption:', '') if st.button('add'): encoding = face_to_compare.tolist() DB.loc[len(DB)] = [face_name, face_des] + encoding DB.to_csv(PATH_DATA, index=False) else: st.write('No human face detected.')
def main(): st.header('Online Stock Price Ticker') #yfinance 실행 symbol = st.text_input('심볼 입력 : ') # symbol = 'AAPL' #이거는 검색하면 나오는 거. 회사이름. 주식 보고 싶은 회사. data = yf.Ticker(symbol) today = datetime.now().date().isoformat() #계속 최선정보가 들어가게 print(today) df = data.history(start='2010-06-01', end='2021-03-22') #문자열로 넣으면 알아서 가져온다/ st.dataframe(df) st.subheader('종가') st.line_chart(df['Close']) #종가 st.subheader('거래량') st.line_chart(df['Volume']) #거래량 #yfinance 라이브러리만의 정보 # data.info #회사 정보, 얘는 딕셔너리 # data.calendar #얘는 데이터프레임 # data.major_holders #대주주 # data.institutional_holders #기간 정보 # data.recommendations # data.dividends #기간별 배당금 div_df = data.dividends st.dataframe(div_df.resample( 'Y').sum()) #타임시리즈 데이터를 resample로 묶어서 사용할 수 있다. 다 했던 거임. new_df = div_df.reset_index() new_df['Year'] = new_df[ 'Date'].dt.year #이렇게 써야 프로펫할 때 사용할 수 있음. 인덱스가 시간이면 안됨. 컬럼으로 되어있어야함. st.dataframe(new_df) fig = plt.figure() plt.bar(new_df['Year'], new_df['Dividends']) #x축 y축. #연도별 배당금을 알 수 있다. st.pyplot(fig) #여러 주식 데이터를 한번에 보여주기. favorites = ['msft', 'tsla', 'nvda', 'aapl', 'amzn'] #멀티 셀렉트로 구현해도 되겠죠 f_df = pd.DataFrame() for stock in favorites: #페이보릿 안에 있는 걸 심볼로 넣으면 된다. f_df[stock] = yf.Ticker(stock).history(start='2010-01-01', end=today)['Close'] st.dataframe(f_df) st.line_chart(f_df) #리퀘스트를 하면 리스폰스를 준다. res = requests.get( 'https://api.stocktwits.com/api/2/streams/symbol/{}.json'.format( symbol)) #JSON 형식이므로, .json() 이용. res_data = res.json() #파이썬의 딕셔너리와 리스트의 조합으로 사용가능 # st.write(res_data) for message in res_data['messages']: #아바타는 왼쪽 글은 오른쪽에. col1, col2 = st.beta_columns([1, 4 ]) #1:4의 비율로 컬럼 두개의 영역을 잡아달라. 컬럼 세개도 가능. with col1: st.image(message['user']['avatar_url']) with col2: st.write('유저 이름 : ' + message['user']['username']) st.write('트윗 내용 : ' + message['body']) st.write('올린 시간 : ' + message['created_at']) p_df = df.reset_index() #인덱스에 있는 내용을 컬럼으로 옮기고 p_df.rename(columns={'Date': 'ds', 'Close': 'y'}, inplace=True) # st.dataframe(p_df) #예측 가능 m = Prophet() m.fit(p_df) future = m.make_future_dataframe(periods=365) forecast = m.predict(future) st.dataframe(forecast) fig1 = m.plot(forecast) st.pyplot(fig1) fig2 = m.plot_components(forecast) st.pyplot(fig2)
import matplotlib.pyplot as plt import seaborn as sns import plotly.express as px import plotly.graph_objects as go from plotly.subplots import make_subplots st.set_page_config(layout='wide') st.write(""" # Dynamic Pricing Demo Page """) df = pd.read_pickle('./data/prd_training_data_220107.pkl') #st.write(df.dtypes) prd_no = st.text_input('Enter product no', '2826341919') if st.button('Enter'): tmp = df[df.prd_no == prd_no] info = tmp.iloc[0] dic = { '날짜' : info['train_ds'], '판매량' : np.square(info['train_y']), '가격' : info['train_avg_prc'] } result = pd.DataFrame(dic) result = result.astype({ '판매량' : int, '가격' : int
def main(): """Hep Mortality Prediction App""" # st.title("Hepatitis Mortality Prediction App") st.markdown(html_temp.format('royalblue'), unsafe_allow_html=True) menu = ["Home", "Login", "Signup"] sub_menu = ["Plot", "Prediction", "Metrics"] choice = st.sidebar.selectbox("Menu", menu) if choice == "Home": st.subheader("Home") # st.text("What is Hepatitis?") st.markdown(descriptive_message_temp, unsafe_allow_html=True) st.image(load_image('images/hepimage.jpeg')) elif choice == "Login": username = st.sidebar.text_input("Username") password = st.sidebar.text_input("Password", type='password') if st.sidebar.checkbox("Login"): create_usertable() hashed_pswd = generate_hashes(password) result = login_user(username, verify_hashes(password, hashed_pswd)) # if password == "12345": if result: st.success("Welcome {}".format(username)) activity = st.selectbox("Activity", submenu) if activity == "Plot": st.subheader("Data Vis Plot") df = pd.read_csv("data/clean_hepatitis_dataset.csv") st.dataframe(df) df['class'].value_counts().plot(kind='bar') st.pyplot() # Freq Dist Plot freq_df = pd.read_csv("data/freq_df_hepatitis_dataset.csv") st.bar_chart(freq_df['count']) if st.checkbox("Area Chart"): all_columns = df.columns.to_list() feat_choices = st.multiselect("Choose a Feature", all_columns) new_df = df[feat_choices] st.area_chart(new_df) elif activity == "Prediction": st.subheader("Predictive Analytics") age = st.number_input("Age", 7, 80) sex = st.radio("Sex", tuple(gender_dict.keys())) steroid = st.radio("Do You Take Steroids?", tuple(feature_dict.keys())) antivirals = st.radio("Do You Take Antivirals?", tuple(feature_dict.keys())) fatigue = st.radio("Do You Have Fatigue", tuple(feature_dict.keys())) spiders = st.radio("Presence of Spider Naeve", tuple(feature_dict.keys())) ascites = st.selectbox("Ascities", tuple(feature_dict.keys())) varices = st.selectbox("Presence of Varices", tuple(feature_dict.keys())) bilirubin = st.number_input("bilirubin Content", 0.0, 8.0) alk_phosphate = st.number_input( "Alkaline Phosphate Content", 0.0, 296.0) sgot = st.number_input("Sgot", 0.0, 648.0) albumin = st.number_input("Albumin", 0.0, 6.4) protime = st.number_input("Prothrombin Time", 0.0, 100.0) histology = st.selectbox("Histology", tuple(feature_dict.keys())) feature_list = [ age, get_value(sex, gender_dict), get_fvalue(steroid), get_fvalue(antivirals), get_fvalue(fatigue), get_fvalue(spiders), get_fvalue(ascites), get_fvalue(varices), bilirubin, alk_phosphate, sgot, albumin, int(protime), get_fvalue(histology) ] st.write(len(feature_list)) st.write(feature_list) pretty_result = { "age": age, "sex": sex, "steroid": steroid, "antivirals": antivirals, "fatigue": fatigue, "spiders": spiders, "ascites": ascites, "varices": varices, "bilirubin": bilirubin, "alk_phosphate": alk_phosphate, "sgot": sgot, "albumin": albumin, "protime": protime, "histolog": histology } st.json(pretty_result) single_sample = np.array(feature_list).reshape(1, -1) # ML model_choice = st.selectbox("Select Model", ["LR", "KNN", "DecisionTree"]) if st.button("Predict"): if model_choice == "KNN": loaded_model = load_model( "models/knn_hepB_model.pkl") prediction = loaded_model.predict(single_sample) pred_prob = loaded_model.predict_proba( single_sample) elif model_choice == "DecisionTree": loaded_model = load_model( "models/decision_tree_clf_hepB_model.pkl") prediction = loaded_model.predict(single_sample) pred_prob = loaded_model.predict_proba( single_sample) else: loaded_model = load_model( "models/logistic_regression_hepB_model.pkl") prediction = loaded_model.predict(single_sample) pred_prob = loaded_model.predict_proba( single_sample) # st.write(prediction) # prediction_label = {"Die":1,"Live":2} # final_result = get_key(prediction,prediction_label) if prediction == 1: st.warning("Patient Dies") pred_probability_score = { "Die": pred_prob[0][0] * 100, "Live": pred_prob[0][1] * 100 } st.subheader( "Prediction Probability Score using {}".format( model_choice)) st.json(pred_probability_score) st.subheader("Prescriptive Analytics") st.markdown(prescriptive_message_temp, unsafe_allow_html=True) else: st.success("Patient Lives") pred_probability_score = { "Die": pred_prob[0][0] * 100, "Live": pred_prob[0][1] * 100 } st.subheader( "Prediction Probability Score using {}".format( model_choice)) st.json(pred_probability_score) if st.checkbox("Interpret"): if model_choice == "KNN": loaded_model = load_model( "models/knn_hepB_model.pkl") elif model_choice == "DecisionTree": loaded_model = load_model( "models/decision_tree_clf_hepB_model.pkl") else: loaded_model = load_model( "models/logistic_regression_hepB_model.pkl") # loaded_model = load_model("models/logistic_regression_model.pkl") # 1 Die and 2 Live df = pd.read_csv( "data/clean_hepatitis_dataset.csv") x = df[[ 'age', 'sex', 'steroid', 'antivirals', 'fatigue', 'spiders', 'ascites', 'varices', 'bilirubin', 'alk_phosphate', 'sgot', 'albumin', 'protime', 'histology' ]] feature_names = [ 'age', 'sex', 'steroid', 'antivirals', 'fatigue', 'spiders', 'ascites', 'varices', 'bilirubin', 'alk_phosphate', 'sgot', 'albumin', 'protime', 'histology' ] class_names = ['Die(1)', 'Live(2)'] explainer = lime.lime_tabular.LimeTabularExplainer( x.values, feature_names=feature_names, class_names=class_names, discretize_continuous=True) # The Explainer Instance exp = explainer.explain_instance( np.array(feature_list), loaded_model.predict_proba, num_features=13, top_labels=1) exp.show_in_notebook(show_table=True, show_all=False) # exp.save_to_file('lime_oi.html') st.write(exp.as_list()) new_exp = exp.as_list() label_limits = [i[0] for i in new_exp] # st.write(label_limits) label_scores = [i[1] for i in new_exp] plt.barh(label_limits, label_scores) st.pyplot() plt.figure(figsize=(20, 10)) fig = exp.as_pyplot_figure() st.pyplot() else: st.warning("Incorrect Username/Password") elif choice == "SignUp": new_username = st.text_input("User name") new_password = st.text_input("Password", type='password') confirm_password = st.text_input("Confirm Password", type='password') if new_password == confirm_password: st.success("Password Confirmed") else: st.warning("Passwords not the same") if st.button("Submit"): create_usertable() hashed_new_password = generate_hashes(new_password) add_userdata(new_username, hashed_new_password) st.success("You have successfully created a new account") st.info("Login to Get Started")
import streamlit as st sentence = st.text_input('Input your sentence here:') if sentence: st.write(sentence)
import streamlit as st import numpy as np st.write("Hello World!") first_name = st.text_input('First name') if st.checkbox('Add last name'): last_name = st.text_input('Last name') else: last_name = '' repetitions = st.slider('Repetitions') st.write(' '.join([first_name, last_name] * repetitions))
def __init__(self): super(Net, self).__init__() self.memory = nn.Parameter(torch.rand((128, 128), dtype=torch.double)) def forward(self, x): x = self.memory * x # x = torch.reshape(x, (30, 30)) x = x.clamp(0, 1) return x net = Net() optimizer = optim.AdamW(net.parameters(), lr=0.005) criterion = nn.MSELoss() DATASET_PATH = st.text_input( 'DATASET PATH', value='C:\\Users\\Admin\\Downloads\\i\\n01514859\\') dataset = ImageDataset(path=DATASET_PATH, size=10) # for image_x, image_y in dataset: # st.image(image_x) # st.stop() st_orig_image = st.empty() st_memorized_image = st.empty() st_loss = st.empty() image_tensor = torch.rand((30, 30)) image = image_tensor.detach().numpy() st_orig_image.image(image, caption='Ground Truth image', width=200) image_tensors = [torch.rand((30, 30)) for i in range(10)] # x_inp = torch.ones(1)
import yaml import streamlit as st import pandas as pd import altair as alt from src.sst import extract_text from src.qna import load_qna_model, answer_ques st.title("YOUtube Question Answering: Awesome project") if st.checkbox('Initialze Configuration File'): with open('config.yaml') as f: config_dict = yaml.safe_load(f) st.info("Success!!! CONFIG YAML INITIALIZED") url_link = st.text_input('Please Provide URL Link Here', "") if url_link: st.info("Success!!! Link Detected") sst_button, qna_button = st.beta_columns(2) # if data_button.checkbox("Load Data"): # filepath = download_wav_file(video_url=url_link, video_dir=config_dict['video_dir']) # st.info("Success!!! Data Loaded") if sst_button.checkbox("Load & Run SST Model over data"): sst_text = extract_text(model_dir=config_dict['sst_model_dir'], model_name=config_dict['sst_model_name'], video_url=url_link, video_dir=config_dict['video_dir'], link_id=config_dict['link_id']) st.info("SST Prediction complete")
# ============================================================================= # INIT scraper = hts.HTS() _max_width_() # ============================================================================= # App definition st.title("🌐 HTML Table Scraper 🕸️") st.markdown(" A simple HTML table scraper made in Python 🐍 & the amazing [Streamlit!](https://www.streamlit.io/) ") st.markdown('### **1️⃣ Enter a URL to scrape **') try: url = st.text_input("", value='https://stackexchange.com/leagues/1/alltime/stackoverflow', max_chars=None, key=None, type='default') if url: arr = ['https://', 'http://'] if any(c in url for c in arr): scraper.url = url #@st.cache(persist=True, show_spinner=False) scraper.load() if scraper.table_count == 1: st.write("This webpage contains 1 table" ) else: st.write("This webpage contains %s tables" % scraper.table_count) if st.button("Show scraped tables"):
def show(): """Shows the sidebar components for the template and returns user inputs as dict.""" inputs = {} with st.sidebar: st.write("## Model") model = st.selectbox("Which model?", list(MODELS.keys())) # Show model variants if model has multiple ones. if isinstance(MODELS[model], dict): # different model variants model_variant = st.selectbox("Which variant?", list(MODELS[model].keys())) inputs["model_func"] = MODELS[model][model_variant] else: # only one variant inputs["model_func"] = MODELS[model] inputs["num_classes"] = st.number_input( "How many classes/output units?", 1, None, 1000, ) st.markdown( "<sup>Default: 1000 classes for training on ImageNet</sup>", unsafe_allow_html=True, ) inputs["pretrained"] = st.checkbox("Use pre-trained model") if inputs["pretrained"]: if inputs["num_classes"] != 1000: classes_note = "<br><b>Note: Final layer will not be trained if using more/less than 1000 classes!</b>" else: classes_note = "" st.markdown( f'<sup>Pre-training on ImageNet, <a href="https://pytorch.org/docs/stable/torchvision/models.html">details</a>{classes_note}</sup>', unsafe_allow_html=True, ) st.write("## Input data") inputs["data_format"] = st.selectbox( "Which data do you want to use?", ("Public dataset", "Numpy arrays", "Image files"), ) if inputs["data_format"] == "Numpy arrays": st.write(""" Expected format: `[images, labels]` - `images` has array shape (num samples, color channels, height, width) - `labels` has array shape (num samples, ) """) elif inputs["data_format"] == "Image files": st.write(""" Expected format: One folder per class, e.g. ``` train +-- dogs | +-- lassie.jpg | +-- komissar-rex.png +-- cats | +-- garfield.png | +-- smelly-cat.png ``` See also [this example dir](https://github.com/jrieke/traingenerator/tree/main/data/image-data) """) elif inputs["data_format"] == "Public dataset": inputs["dataset"] = st.selectbox( "Which one?", ("MNIST", "FashionMNIST", "CIFAR10")) st.write("## Preprocessing") # st.checkbox("Convert to grayscale") # st.checkbox("Convert to RGB", True) # TODO: Maybe show disabled checkbox here to make it more aligned with the # display above. # st.markdown( # '<label data-baseweb="checkbox" class="st-eb st-b4 st-ec st-d4 st-ed st-at st-as st-ee st-e5 st-av st-aw st-ay st-ax"><span role="checkbox" aria-checked="true" class="st-eg st-b2 st-bo st-eh st-ei st-ej st-ek st-el st-bb st-bj st-bk st-bl st-bm st-em st-en st-eo st-ep st-eq st-er st-es st-et st-av st-aw st-ax st-ay st-eu st-cb st-ev st-ew st-ex st-ey st-ez st-f0 st-f1 st-f2 st-c5 st-f3 st-f4 st-f5" style="background-color: rgb(150, 150, 150);"></span><input aria-checked="true" type="checkbox" class="st-b0 st-an st-cv st-bd st-di st-f6 st-cr" value=""><div class="st-ev st-f7 st-bp st-ae st-af st-ag st-f8 st-ai st-aj">sdf</div></label>', # unsafe_allow_html=True, # ) st.write("Resize images to 256 (required for this model)") st.write("Center-crop images to 224 (required for this model)") if inputs["pretrained"]: st.write("Scale mean and std for pre-trained model") st.write("## Training") inputs["gpu"] = st.checkbox("Use GPU if available", True) inputs["checkpoint"] = st.checkbox("Save model checkpoint each epoch") if inputs["checkpoint"]: st.markdown( "<sup>Checkpoints are saved to timestamped dir in `./checkpoints`. They may consume a lot of storage!</sup>", unsafe_allow_html=True, ) inputs["loss"] = st.selectbox( "Loss function", ("CrossEntropyLoss", "BCEWithLogitsLoss")) inputs["optimizer"] = st.selectbox("Optimizer", list(OPTIMIZERS.keys())) default_lr = OPTIMIZERS[inputs["optimizer"]] inputs["lr"] = st.number_input("Learning rate", 0.000, None, default_lr, format="%f") inputs["batch_size"] = st.number_input("Batch size", 1, None, 128) inputs["num_epochs"] = st.number_input("Epochs", 1, None, 3) inputs["print_every"] = st.number_input( "Print progress every ... batches", 1, None, 1) st.write("## Visualizations") inputs["visualization_tool"] = st.selectbox( "How to log metrics?", ("Not at all", "Tensorboard", "Aim", "Weights & Biases", "comet.ml"), ) if inputs["visualization_tool"] == "Aim": inputs["aim_experiment"] = st.text_input( "Experiment name (optional)") st.markdown( '<sup>View by running: `aim up`</br>See full documentation <a href="https://github.com/aimhubio/aim#contents" target="_blank">here</a></sup>', unsafe_allow_html=True, ) elif inputs["visualization_tool"] == "Weights & Biases": inputs["wb_project"] = st.text_input("W&B project name (optional)") inputs["wb_name"] = st.text_input("W&B experiment name (optional)") elif inputs["visualization_tool"] == "comet.ml": # TODO: Add a tracker how many people click on this link. "[Sign up for comet.ml](https://www.comet.ml/) :comet: " inputs["comet_api_key"] = st.text_input("Comet API key (required)") inputs["comet_project"] = st.text_input( "Comet project name (optional)") elif inputs["visualization_tool"] == "Tensorboard": st.markdown( "<sup>Logs are saved to timestamped dir in `./logs`. View by running: `tensorboard --logdir=./logs`</sup>", unsafe_allow_html=True, ) # "Which plots do you want to add?" # # TODO: Show some examples. # st.checkbox("Sample images", True) # st.checkbox("Confusion matrix", True) # "## Saving" # st.checkbox("Save config file", True) # st.checkbox("Save console output", True) # st.checkbox("Save finished model", True) # if model in TORCH_MODELS: # st.checkbox("Save checkpoint after each epoch", True) return inputs
def run_app(): # dataset_textbox = st.sidebar.text_input('dataset path', value='C:\\Users\\Admin\\Downloads\\i\\n01514859\\') DATASET_PATH = st.text_input( 'DATASET PATH', value='C:\\Users\\Admin\\Downloads\\i\\n01514859\\') epoch_loc = st.empty() prog_bar = st.empty() loss_loc = st.empty() global_loss_loc = st.empty() loss_chart = st.empty() glob_loss_chart = st.empty() row0 = st.empty() row1 = st.empty() row2 = st.empty() row3 = st.empty() row4 = st.empty() row5 = st.empty() # st.stop() PATH = "upscaler.pt" net = Net() # too lazy to detect if the file exits. try: net.load_state_dict(torch.load(PATH)) st.write('MODEL LOADED!') except Exception: pass cuda = torch.device('cuda') net.to(cuda) # criterion = nn.CrossEntropyLoss() # criterion = nn.MSELoss() criterion = kornia.losses.PSNRLoss(1.0) LEARNING_RATE = 0.01 optimizer = optim.AdamW(net.parameters(), lr=LEARNING_RATE) # st.title('image upscaler') img = load_img('image.png') losses = deque(maxlen=100) global_losses = deque(maxlen=100) EPOCHS = 500 BATCH_SIZE = 10 dataset = ImageDataset(path=DATASET_PATH) def collate_wrapper(samples): return samples train_loader = DataLoader(dataset, batch_size=BATCH_SIZE, shuffle=True, collate_fn=collate_wrapper) for epoch in range(EPOCHS): i = 1 epoch_loc.write(f"EPOCH:\t{epoch}/{EPOCHS - 1}") global_loss = torch.tensor([0.0], device=cuda) optimizer.zero_grad() # TODO: confirm that shuffle works # -------------------- for batch in train_loader: optimizer.zero_grad() loss = torch.tensor([0.0], device=cuda) for sample in batch: x, y = sample x = torch.tensor(x) x = torch.unsqueeze(x, 0) try: image = x.permute(0, 3, 1, 2) image = F.interpolate(image, size=(128, 128)) image = image.permute(0, 2, 3, 1) row1.image(image.numpy(), width=250, caption='original image') except Exception: break x = x.permute(0, 3, 1, 2) y = F.interpolate(x, size=(128, 128)) x = F.interpolate(x, size=(32, 32)) x = F.interpolate(x, size=(128, 128)) row2.image(x.permute(0, 2, 3, 1).detach().numpy(), width=250, caption='Downsampled') prog_bar.progress(i / len(dataset)) i += 1 out = net(x.detach().cuda().float()) diff = torch.abs(out.detach().cpu() - y.detach().cpu()) diff_image = diff.permute(0, 2, 3, 1).numpy() row5.image(diff_image, width=250, caption='absolute difference') row3.image(out.permute(0, 2, 3, 1).detach().cpu().numpy(), width=250, caption='Reconstructed') loss = 1 / criterion(out, y.detach().cuda().float()) # loss = criterion(out, y.detach().cuda().float()) row4.write(f'LOSS: {loss.detach().cpu()}') # loss.backward() # optimizer.step() # st.stop() losses.append(loss.detach().cpu().numpy()) loss_chart.line_chart(pd.DataFrame(losses, columns=[ 'loss', ])) global_loss += loss loss_loc.write(f"LOSS:\t{loss.detach().cpu()}") loss.backward() optimizer.step() global_loss_loc.write( f"GLOBAL LOSS:\t{global_loss.detach().cpu()} \nGLOB AVERAGE LOSS:\t{global_loss.detach().cpu()/len(dataset)}" ) global_losses.append(global_loss.detach().cpu().numpy()) glob_loss_chart.line_chart( pd.DataFrame(global_losses, columns=[ 'global_loss', ])) try: torch.save(net.state_dict(), PATH) st.write('MODEL SAVED!') except Exception: pass
import streamlit as st import sklearn import joblib model = joblib.load('Movie Review Sentiment') st.title('Movie Sentiment') ip = st.text_input('Enter your review') op = model.predict([ip]) if st.button('Predict'): st.title(op[0])
def main(): st.title("Credit card default prediction model") lim_bal = st.text_input("Limit Balance", "Type Here") education = st.text_input("Education level", "Type Here") marriage = st.text_input("Marital status", "Type Here") age = st.text_input("Customer age", "Type Here") pay1 = st.text_input("September payment status", "Type Here") bill1 = st.text_input("Bill statement september", "Type Here") bill2 = st.text_input("Bill statement august", "Type Here") bill3 = st.text_input("Bill statement july", "Type Here") bill4 = st.text_input("Bill statement june", "Type Here") bill5 = st.text_input("Bill statement may", "Type Here") bill6 = st.text_input("Bill statement april", "Type Here") payamt1 = st.text_input("amount statement september", "Type Here") payamt2 = st.text_input("amount statement august", "Type Here") payamt3 = st.text_input("amount statement july", "Type Here") payamt4 = st.text_input("amount statement june", "Type Here") payamt5 = st.text_input("amount statement may", "Type Here") payamt6 = st.text_input("amount statement april", "Type Here") if st.button("Predict"): result = classifier.predict([[ lim_bal, education, marriage, age, pay1, bill1, bill2, bill3, bill4, bill5, bill6, payamt1, payamt2, payamt3, payamt4, payamt5, payamt6 ]]) print(result) st.success("The output is {}".format(result))
if 'correct' in result[0:8].lower(): st.balloons() return result ## Request Challenge Description url = 'https://kata.geosci.ai/challenge/true-vertical-depth' r = get_question(url) if st.checkbox('**Check to show instructions from Agile for this challenge.**', value=False): st.markdown(r.text, unsafe_allow_html=True) ## Set up request framework for QA st.title('My solution') st.markdown('The data for this Kata challenge will be randomized based upon the key below. Feel free to change it to check the consistency of answers!') my_key = st.text_input(label='Enter a key to initiate request (any string of characters)',value='armstrys') ## Input r = get_data(url, my_key) st.subheader('Let\'s throw the text in a dataframe') st.write(''' The input text is relatively tame in this case. We can just pass the text through `io.StringIO` and into `pandas.read_csv`. We'll then eliminate some unknown and `NaN` values. ''') with st.echo(): cols = [ 'X', 'Y',
from preprocessing_utils import * html_temp = """ <div style="background-color:blue;padding:10px"> <h1 style="color:white;text-align:center;">News Article Clustering</h1> <h3 style="color:white;text-align:center;">Data Mining Assignment4 - Web-Content Mining</h3> <h3 style="color:white;text-align:center;">Enosh Nyarige</h3> </div> """ st.markdown(html_temp, unsafe_allow_html=True) df = pd.read_csv('Articlesclusters.csv') # Get user input news_article = st.text_input( "What is the content in the article you want to cluster?") news_category = st.multiselect( 'Multiselect', ['Sports', 'Politics', 'Business', 'Arts/Culture/Celebrities ']) news_url = st.text_input("Link to the article: ") if news_article and news_category and news_url: st.write("**Content in the News Article **: ", news_article) st.write("**Selected news category **:", news_category) st.write("**Attached link to news rticle **:", news_url) k_means = pickle.load(open('kmeans_model.pkl', 'rb')) tfidf_vectorizer = pickle.load(open('vectorizer.pkl', 'rb')) tfidf_pca = pickle.load(open('tfidf_pca.pkl', 'rb')) preprocessed_news_article = preprocess_article(news_article)