def edit_profile(username): """ View function to allow user to edit their profile Form will be pre-filled with data from the current user profile, this is not a necessary form to fill thus the user can exit from this page easily :param username: their currently logged in username :return: edit profile template """ form = EditProfileForm(new_username=username) # if the form is valid on submission if form.validate_on_submit(): if form.validate_form(): current_user.first_name = form.first_name.data current_user.last_name = form.last_name.data current_user.username = form.username.data current_user.about_me = form.about_me.data db.session.add(current_user) db.session.commit() flash(message="Your changes have been saved successfully", category="success") # if update is successful, redirect to user dashboard return redirect(url_for("dashboard.user_account", username=username)) else: flash(message="Profile not updated", category="error") return render_template("auth.edit_profile.html", form=form, user=current_user) return render_template("auth.edit_profile.html", form=form, user=current_user)
def edit_profile(): form = EditProfileForm(current_user.username) if form.validate_on_submit(): current_user.username = form.username.data db.session.commit() flash('Your changes have been saved.') return redirect(url_for('edit_profile')) elif request.method == 'GET': form.username.data = current_user.username return render_template('edit_profile.html', title='Edit Profile', form=form)
def edit_profile(): form = EditProfileForm(current_user.username) if form.validate_on_submit(): current_user.username = form.username.data current_user.about_me = form.about_me.data db.session.commit() flash('Ваши изменения сохранены') return redirect(url_for('edit_profile')) elif request.method == 'GET': form.username.data = current_user.username form.about_me.data = current_user.about_me return render_template('edit_profile.html', title='Edit Profile', form=form)
def edit_profile(): form = EditProfileForm(current_user.email) if form.validate_on_submit(): current_user.email = form.email.data current_user.first = form.first_name.data current_user.last = form.last_name.data db.session.commit() flash('Your changes have been saved.') return redirect(url_for('edit_profile')) elif request.method == 'GET': form.email.data = current_user.email form.first_name.data = current_user.first form.last_name.data = current_user.last return render_template('edit_profile.html', form=form)
def edit_profile(): """编辑用户信息页""" form = EditProfileForm(current_user.username) if request.method == 'GET': # 直接访问时,设置表单默认状态 form.username.data = current_user.username form.about_me.data = current_user.about_me elif form.validate_on_submit(): # 表单验证通过后更新数据库 current_user.username = form.username.data current_user.about_me = form.about_me.data db.session.commit() flash("个人信息更新成功") return redirect(url_for('edit_profile')) return render_template('edit_profile.html', title='更新用户信息', form=form)
def edit_profile(): form = EditProfileForm(request.form) if form.validate_on_submit(): # prevent non admin users from editing other users profile if not current_user.is_admin(): form.id.data = current_user.id user = User.query.get(form.id.data) user.populate_from_form(form) db.session.commit() flash('Successfully updated user info.', 'success') return {'status': 200} else: flash(form.errors, 'form-error') return {'status': 400}
def edit(): form = EditProfileForm() if form.validate_on_submit(): current_user.eFirst = form.eFirst.data current_user.eLast = form.eLast.data current_user.jFirst = form.jFirst.data current_user.jLast = form.jLast.data db.session.commit() flash("Your changes have been saved! Omedetou!") return redirect(url_for('edit')) elif request.method == 'GET': form.eFirst.data = current_user.eFirst form.eLast.data = current_user.eLast return render_template('edit.html', form=form)
def edit_profile(): form = EditProfileForm(current_user.username) if form.validate_on_submit(): current_user.username = form.username.data current_user.about_me = form.about_me.data db.session.commit() flash('Your changes have been saved') return redirect(url_for('edit_profile')) elif request.method == 'GET': #if its the first time loading the page, populate fields with existing Data form.username.data = current_user.username form.about_me.data = current_user.about_me return render_template('edit_profile.html', title='Edit Profile', form=form)
def edit_profile(): form = EditProfileForm(current_user.username) if form.validate_on_submit(): current_user.username = form.username.data current_user.about_me = form.about_me.data db.session.commit() flash("Your chages were applied") return redirect(url_for('edit_profile')) elif request.method == 'GET': form.username.data = current_user.username form.about_me.data = current_user.about_me return render_template("edit_profile.html", title="Edit Profile", form=form)
def edit_profile(): # check request.method, which will be GET for the initial request # and POST for a submission that failed validation form = EditProfileForm(current_user.username) if form.validate_on_submit(): current_user.username = form.username.data current_user.about_me = form.about_me.data db.session.commit() flash('Your changes have been saved.') return redirect(url_for('edit_profile')) elif request.method == 'GET': form.username.data = current_user.username form.about_me.data = current_user.about_me return render_template('edit_profile.html', title='Edit Profile', form=form)
def edit_profile(): form = EditProfileForm(current_user.username) if form.validate_on_submit(): current_user.username = form.username.data current_user.about_me = form.about_me.data db.session.commit() flash("Your changes have been saved.") return redirect(url_for("edit_profile")) elif request.method == "GET": form.username.data = current_user.username form.about_me.data = current_user.about_me return render_template("edit_profile.html", title="Edit Profile", form=form)
def edit_profile(): form = EditProfileForm(current_user.username) if form.validate_on_submit(): current_user.username = form.username.data current_user.about_me = form.about_me.data db.session.commit() flash('Your changes have been saved.') return redirect(url_for('edit_profile')) elif request.method == 'GET': form.username.data = current_user.username form.about_me.data = current_user.about_me return render_template('edit_profile.html', title='Edit Profile', form=form)
def edit_profile(): form = EditProfileForm(current_user.username) if form.validate_on_submit(): if form.avatar.data: picture_file = save_picture(form.avatar.data) current_user.avatar = picture_file current_user.about_me = form.about_me.data db.session.commit() print("Changes saved.") return redirect(url_for('user', username=current_user.username))#)) username=form.username.data)) elif request.method == 'GET': form.about_me.data = current_user.about_me print("fail") return render_template('edit_profile.html', title='Edit Profile', form=form)
def edit_profile(): form = EditProfileForm() if form.validate_on_submit(): current_user.username = form.username.data current_user.about_me = form.about_me.data db.session.commit() flash('commited') return redirect(url_for('edit_profile')) elif request.method == 'GET': form.username.data = current_user.username form.about_me.data = current_user.about_me return render_template('edit_profile.html', title='personal data edit', form=form)
def edit_profile(): form = EditProfileForm(current_user.username) if form.validate_on_submit(): current_user.username = form.username.data current_user.about_me = form.about_me.data db.session.commit() flash(_('Sucessfully updated your profile')) return redirect(url_for('edit_profile')) elif request.method == 'GET': form.username.data = current_user.username form.about_me.data = current_user.about_me return render_template('edit_profile.html', title='User Profile', form=form)
def edit_profile(): form = EditProfileForm(current_user.name) if form.validate_on_submit(): current_user.name = form.userEdit.data current_user.about = form.aboutEdit.data db.session.commit() flash('Все изменения были сохранены') return redirect(url_for('edit_profile')) elif request.method == 'GET': form.userEdit.data = current_user.name form.aboutEdit.data = current_user.about return render_template('edit_profile.html', title='Редактирование профиля пользователя', form=form)
def edit_profile(): # form = EditProfileForm() # Rmv'd in Ch7 7 for the following form = EditProfileForm(current_user.username) # Added in chapter 7 if form.validate_on_submit(): current_user.username = form.username.data current_user.about_me = form.about_me.data db.session.commit() flash(_('Your changes have been saved.')) elif request.method == 'GET': form.username.data = current_user.username form.about_me.data = current_user.about_me return render_template('edit_profile.html', title=_('Edit Profile'), form=form)
def edit_profile(): form = EditProfileForm() if form.validate_on_submit(): current_user.nome = unidecode.unidecode(form.username.data) current_user.email = form.email.data db.session.commit() flash('Suas alterações foram salvas(e automaticamente removido as ' + 'acentuações ;) )') return redirect(url_for('index')) elif request.method == 'GET': # busca do banco os dados para exibir ao usuário o que está salvo form.username.data = current_user.nome form.email.data = current_user.email return render_template('editar.html', title='Editar Perfil', form=form)
def edit_profile(): form = EditProfileForm(current_user.username) if form.validate_on_submit(): current_user.username = form.username.data db.session.commit() flash('Ditt användarnamn har sparats som {}'.format( current_user.username)) return redirect(url_for('edit_profile')) elif request.method == 'GET': form.username.data = current_user.username return render_template('edit_profile.html', title='Redigera profil', form=form, headline='Redigera profil')
def edit_profile(): form = EditProfileForm() if form.validate_on_submit(): current_user.username = form.username.data current_user.about_me = form.about_me.data db.session.commit() flash('Ваши изменения сохранены') return redirect(url_for('edit_profile')) elif request.method == 'GET': form.username.data = current_user.username form.about_me.data = current_user.about_me return render_template('edit_profile.html', form=form, title='Редактирование профиля')
def account(username): if current_user.username == username: # wtp = want to play, cp = currently playing games = {'wtp': [], 'cp': [], 'finished': []} user_games = list(current_user.saved_games.keys()) for game in user_games: games[current_user.saved_games[game]].append( requests.get(f'https://api.rawg.io/api/games/{game}').json()) collection_form = CreateCollectionForm(obj=current_user) profile_form = EditProfileForm(obj=current_user) if request.method == 'POST': if profile_form.validate_on_submit(): avatar_url = upload_avatar(request) current_user.update_profile(profile_form.data['name'], profile_form.data['city'], avatar_url) return render_template('account_own.html', user=current_user, games=games, collection_form=collection_form, profile_form=profile_form) return render_template('account_own.html', user=current_user, games=games, collection_form=collection_form, profile_form=profile_form) else: user = User.objects(username=username).first() # wtp = want to play, cp = currently playing games = {'wtp': [], 'cp': [], 'finished': []} user_games = list(user.saved_games.keys()) for game in user_games: games[user.saved_games[game]].append( requests.get(f'https://api.rawg.io/api/games/{game}').json()) public_collections = Collection.objects(username=username, is_private=False).all() return render_template('account.html', user=user, games=games, collections=public_collections)
def edit_profile(): error = None image_file = url_for('static', filename='profile_pics/' + current_user.image_file) user = UserModel.query.filter_by( username=current_user.username).first_or_404() form = EditProfileForm(request.form) if form.validate_on_submit(): current_user.first_name = form.first_name.data current_user.last_name = form.last_name.data current_user.email = form.email.data current_user.phone_number = form.phone_number.data current_user.address_line_1 = form.address_line_1.data current_user.address_line_2 = form.address_line_2.data current_user.city = form.city.data current_user.state = form.state.data if form.state.data == 'Select State': error = "Select a state." return render_template('edit_profile.html', title='Edit Profile', form=form, user=user, error=error, image_file=image_file) current_user.zip_code = form.zip_code.data db.session.commit() print("just posted") flash('Your changes have been saved.') return redirect(url_for('profile')) elif request.method == 'GET': form.first_name.data = current_user.first_name form.last_name.data = current_user.last_name form.email.data = current_user.email form.phone_number.data = current_user.phone_number form.address_line_1.data = current_user.address_line_1 form.address_line_2.data = current_user.address_line_2 form.city.data = current_user.city form.state.data = current_user.state form.zip_code.data = current_user.zip_code return render_template('edit_profile.html', title='Edit Profile', form=form, user=user, error=error, image_file=image_file) return render_template('profile.html', user=user, error=error, image_file=image_file)
def edit_profile(): form = EditProfileForm() giveaiq_username = current_user.username giveaiq_displayname = giveaiq_username[3:] giveaiq_accounttype = giveaiq_username[0:3] if form.validate_on_submit(): notify_me = form.notify_me.data promote_me = form.promote_me.data if notify_me: notify_me = 1 else: notify_me = 0 if promote_me: promote_me = 1 else: promote_me = 0 if giveaiq_accounttype == "TW-": cmd = "UPDATE usertwitter SET notify_me='{0}', promote_me='{1}' WHERE screen_name='{2}'".format( notify_me, promote_me, current_user.displayname) elif giveaiq_accounttype == "TG-": cmd = "UPDATE usertelegram SET notify_me='{0}', promote_me='{1}' WHERE name='{2}'".format( notify_me, promote_me, current_user.displayname) db.session.execute(cmd, ) db.session.commit() flash('Your changes have been saved.') return redirect(url_for('edit_profile')) elif request.method == 'GET': if giveaiq_accounttype == "TW-": cmd = "SELECT notify_me, promote_me FROM usertwitter WHERE screen_name='{0}'".format( current_user.displayname) elif giveaiq_accounttype == "TG-": cmd = "SELECT notify_me, promote_me FROM usertelegram WHERE name='{0}'".format( current_user.displayname) edit_me = db.session.execute(cmd, ).fetchall() if edit_me: for worder in edit_me: notify_me = worder[0] promote_me = worder[1] if notify_me == 1: form.notify_me.data = notify_me if promote_me == 1: form.promote_me.data = promote_me return render_template('edit_profile.html', title='Edit Profile', form=form)
def edit_profile(): form = EditProfileForm() if form.validate_on_submit(): user = session.get("id") password = request.form["password"] print(password) if not user.check_password(password): flash("비밀번호가 맞지 않습니다. ") return redirect(url_for("edit_profile")) user.set_password(form.new_password.data) db.session.add(user) db.session.commit() flash("수정이 완료되었습니다!") return redirect(url_for("mypage")) return render_template("/user/edit_profile.html", form=form)
def edit_profile(): """Render the page for profile editing.""" form = EditProfileForm(current_user.Username) if form.validate_on_submit(): current_user.Username = form.username.data current_user.Bio = form.bio.data db.session.commit() flash('Profile edit successful.') return redirect(url_for('profile', Username=current_user.Username)) elif request.method == 'GET': form.username.data = current_user.Username form.bio.data = current_user.Bio return render_template('edit_profile.html', title='Edit Profile', form=form)
def test_edit_profile_email_missing(self): edit_profile_form = { 'first_name': 'testsdgsdg', 'last_name': 'sgfsdgdgdg', 'engage_anonymously': True, 'gender': 'female', 'alias': 'sdgsdgsdgdg', 'year_of_birth': 1989, 'avatar': None, 'mobile_number': '8767564444', 'email': '*****@*****.**', 'identity': '' } form = EditProfileForm(data=edit_profile_form) self.assertEqual(form.is_valid(), False)
def edit_profile(): form = EditProfileForm(current_user.username) if form.validate_on_submit(): # 如果验证成功,则是正确提交的内容,直接存入数据库 current_user.username = form.username.data current_user.about_me = form.about_me.data db.session.commit() flash('Your changes have been saved.') return redirect(url_for('edit_profile')) elif request.method == 'GET': # 如果是GET请求,则是第一次请求,获取默认的内容并返回页面输入 form.username.data = current_user.username form.about_me.data = current_user.about_me # 否则,验证失败且是POST请求,则直接返回原页面重新输入 return render_template('edit_profile.html', title='Edit Profile', form=form)
def edit_profile(): form = EditProfileForm(current_user.username) if form.validate_on_submit(): current_user.username = form.username.data current_user.about_me = form.about_me.data db.session.commit() flash(_('Your changes have been saved.')) return redirect(url_for('edit_profile')) elif request.method == 'GET': form.username.data = current_user.username form.about_me.data #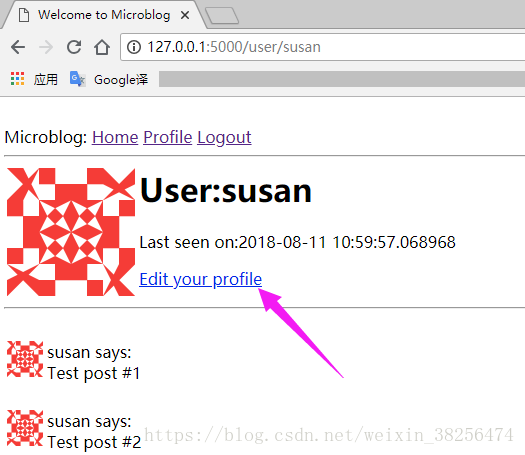 = current_user.about_me return render_template('edit_profile.html', title='Edit Profile', form=form)
def edit_profile(): form = EditProfileForm(current_user.username) if form.validate_on_submit(): current_user.username = form.username.data current_user.note = form.note.data res = mysql.Mod("user", {"id": current_user.id}, { "username": "******" % current_user.username, "note": "'%s'" % current_user.note}) if res != 0: flash('Your changes have been saved.') return redirect(url_for('edit_profile')) elif request.method == 'GET': form.username.data = current_user.username form.note.data = current_user.note return render_template('edit_profile.html', title='Edit Profile', form=form)
def edit_profile(): form = EditProfileForm(current_user.username) # if validate_on_submit() returns True I copy the data from the form into the user object and then write the object to the database if form.validate_on_submit(): current_user.username = form.username.data current_user.about_me = form.about_me.data db.session.commit() flash('Your changes have been saved.') return redirect(url_for('edit_profile')) elif request.method == 'GET': form.username.data = current_user.username form.about_me.data = current_user.about_me return render_template('edit_profile.html', title='Edit Profile', form=form)
def edit_profile(): from app.forms import EditProfileForm form = EditProfileForm() current_user = request.form['FirstName'] if form.validate_on_submit(): current_user.username = form.username.data current_user.about_me = form.about_me.data # db.session.commit() flash('Your changes have been saved.') return redirect(('edit_profile')) elif request.method == 'GET': form.username.data = current_user.username form.about_me.data = current_user.about_me return render_template('edit_profile.html')
def edit(): form = EditProfileForm(g.user.nickname) if form.validate_on_submit(): g.user.nickname = form.nickname.data g.user.about_me = form.about_me.data g.user.email = form.email.data db.session.add(g.user) db.session.commit() flash('Your changes have been saved') return redirect(url_for('user', nickname=g.user.nickname)) else: form.nickname.data = g.user.nickname form.about_me.data = g.user.about_me form.email.data = g.user.email return render_template('edit.html', form=form)
def edit_profile(): form = EditProfileForm() # Jeśli funkcja validate_on_submit () zwraca wartość True, kopiuję dane z formularza do obiektu użytkownika, # a następnie zapisuję obiekt w bazie danych. if form.validate_on_submit(): current_user.username = form.username.data current_user.about_me = form.about_me.data db.session.commit() flash('Supcio! Twoje zmiany zostały zapisane.') return redirect(url_for('user', username=current_user.username)) elif request.method == 'GET': form.username.data = current_user.username form.about_me.data = current_user.about_me return render_template('edit_profile.html', title='Edit Profile', form=form)
def edit_profile(): """ Route for edit profile page. Gets data form EditProfileForm and update current user object. """ form = EditProfileForm(current_user.username, current_user.email) if form.validate_on_submit(): current_user.username = form.username.data current_user.email = form.email.data db.session.commit() flash('Your changes have been saved.') return redirect(url_for('edit_profile')) elif request.method == 'GET': form.username.data = current_user.username form.email.data = current_user.email return render_template('edit_profile.html', title='Edit Profile', form=form)
def edit_profile(): form = EditProfileForm(current_user.username) if form.validate_on_submit(): current_user.username = form.username.data current_user.about_me = form.about_me.data db.session.commit() flash("Profile updated!") return redirect(url_for("profile", username=current_user.username)) elif request.method == "GET": form.username.data = current_user.username form.about_me.data = current_user.about_me return render_template("edit_profile.html", title='Edit Profile', form=form)
def edit_profile(): form = EditProfileForm(current_user.username) if form.validate_on_submit(): current_user.username = form.username.data current_user.about_me = form.about_me.data db.session.commit() flash('Your changes have been saved.') return redirect(url_for('edit_profile')) # when we first click edit profile -- getting the page elif request.method == 'GET': # pre-populate the fields with the data stored in database form.username.data = current_user.username form.about_me.data = current_user.about_me # for validation error, will be populated by WTForms return render_template('edit_profile.html', title='Edit Profile', form=form)
def edit_profile(): if not current_user.id: return render_template('admin/error.html') userdata = user.get_user_details(current_user.id) editprofile_form = EditProfileForm(request.form, obj=userdata) if request.method == 'POST' and editprofile_form.validate(): userdata = user.update_user(request.form) return render_template('admin/editprofile.html', form=editprofile_form) else: editprofile_form.populate_obj(userdata) return render_template('admin/editprofile.html', form=editprofile_form, id=userdata.id)
def edit_profile(): form = EditProfileForm() if form.validate_on_submit(): current_user.username = form.username.data current_user.about_me = form.about_me.data db.session.commit() flash('Your changes have been saved.') return redirect(url_for('edit_profile')) elif request.method == 'GET': ''' This elif clause is when a user is trying to edit the form. We present the current data from the db. ''' form.username.data = current_user.username form.about_me.data = current_user.about_me return render_template('edit_profile.html', title='Edit Profile', form=form)
def test_edit_profile_email_already_exist(self): # This beloe email address has already been set to user in setup() call. edit_profile_form = { 'username': '******', # New edited email address 'first_name': 'testsdgsdg', 'last_name': 'sgfsdgdgdg', 'engage_anonymously': True, 'gender': 'female', 'alias': 'sdgsdgsdgdg', 'year_of_birth': 1989, 'avatar': None, 'mobile_number': '8767564444', 'email': '*****@*****.**', # Current user email address 'identity': '' } form = EditProfileForm(data=edit_profile_form) self.assertEqual(form.is_valid(), False)
def test_validate_user_should_be_able_to_edit_profile_with_unique_username(self): """>>>> Test that user can edit their account with unique username and password""" form = EditProfileForm(new_username="******", about_me=ascii_letters * 3) self.assertTrue(form.validate_form())
def test_validate_user_can_not_edit_existing_username(self): """>>>> Test the user can not register with an already taken username""" form = EditProfileForm(new_username=self.test_author2_username, about_me=ascii_letters * 4) self.assertFalse(form.validate_on_submit())
def test_validates_length_of_form(self): """>>>> Test that the edit profile form about me section is no more than 250 characters""" form = EditProfileForm(new_username=self.test_author1_username, about_me=ascii_letters * 5) self.assertFalse(form.validate_on_submit())
context['user'] = CustomUser.objects.get(pk=request.user.pk) except Exception, e: raise Http404('404') return render(request, 'profile_page.html', context) def edit_profile(request): context = {} try: user = CustomUser.objects.get(pk=request.user.pk) except Exception, e: raise Http404('404') form = EditProfileForm(request.POST or None, instance=user) context['form'] = form if form.is_valid(): form.save() return redirect('/profile/') else: print form.errors return render(request, 'edit_profile.html', context) @staff_member_required def delete_album(request, pk): album = Album.objects.get(pk=pk)