def edit_profile(username): """ View function to allow user to edit their profile Form will be pre-filled with data from the current user profile, this is not a necessary form to fill thus the user can exit from this page easily :param username: their currently logged in username :return: edit profile template """ form = EditProfileForm(new_username=username) # if the form is valid on submission if form.validate_on_submit(): if form.validate_form(): current_user.first_name = form.first_name.data current_user.last_name = form.last_name.data current_user.username = form.username.data current_user.about_me = form.about_me.data db.session.add(current_user) db.session.commit() flash(message="Your changes have been saved successfully", category="success") # if update is successful, redirect to user dashboard return redirect(url_for("dashboard.user_account", username=username)) else: flash(message="Profile not updated", category="error") return render_template("auth.edit_profile.html", form=form, user=current_user) return render_template("auth.edit_profile.html", form=form, user=current_user)
def edit_profile(): form = EditProfileForm(current_user.username) if form.validate_on_submit(): current_user.username = form.username.data db.session.commit() flash('Your changes have been saved.') return redirect(url_for('edit_profile')) elif request.method == 'GET': form.username.data = current_user.username return render_template('edit_profile.html', title='Edit Profile', form=form)
def edit_profile(): form = EditProfileForm(current_user.username) if form.validate_on_submit(): current_user.username = form.username.data current_user.about_me = form.about_me.data db.session.commit() flash('Ваши изменения сохранены') return redirect(url_for('edit_profile')) elif request.method == 'GET': form.username.data = current_user.username form.about_me.data = current_user.about_me return render_template('edit_profile.html', title='Edit Profile', form=form)
def edit_profile(): form = EditProfileForm(request.form) if form.validate_on_submit(): # prevent non admin users from editing other users profile if not current_user.is_admin(): form.id.data = current_user.id user = User.query.get(form.id.data) user.populate_from_form(form) db.session.commit() flash('Successfully updated user info.', 'success') return {'status': 200} else: flash(form.errors, 'form-error') return {'status': 400}
def edit_profile(): form = EditProfileForm(current_user.username) if form.validate_on_submit(): current_user.username = form.username.data current_user.about_me = form.about_me.data db.session.commit() flash("Your chages were applied") return redirect(url_for('edit_profile')) elif request.method == 'GET': form.username.data = current_user.username form.about_me.data = current_user.about_me return render_template("edit_profile.html", title="Edit Profile", form=form)
def edit_profile(): ''' function to let a user edit their profile''' form = EditProfileForm(current_user.username) if form.validate_on_submit(): current_user.username = form.username.data current_user.about_me = form.about_me.data db.session.commit() flash(_('Your changes have been saved.')) return redirect(url_for('edit_profile')) elif request.method == 'GET': form.username.data = current_user.username form.about_me.data = current_user.about_me return render_template('edit_profile.html', title=_('Edit Profile'), form=form)
def edit_profile(): form = EditProfileForm(current_user.display_name) if form.validate_on_submit(): current_user.display_name = form.username.data current_user.about_me = form.about_me.data current_user.set_username() db.session.commit() flash('Changes have been saved.') return redirect(url_for('edit_profile')) elif request.method == 'GET': form.username.data = current_user.display_name form.about_me.data = current_user.about_me return render_template('edit_profile.html', title='Edit Profile', form=form)
def edit_profile(): form = EditProfileForm(current_user.username) if form.validate_on_submit(): current_user.username = form.username.data current_user.about_me = form.about_me.data db.session.commit() flash(_('Your changes have been saved.')) return redirect(url_for('edit_profile')) elif request.method == 'GET': form.username.data = current_user.username form.about_me.data #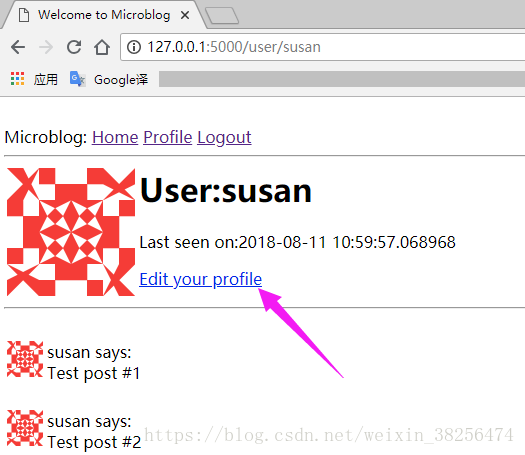 = current_user.about_me return render_template('edit_profile.html', title='Edit Profile', form=form)
def edit_profile(): form = EditProfileForm() if form.validate_on_submit(): current_user.username = form.username.data current_user.about_me = form.about_me.data db.session.commit() flash('Your changes have been saved') return redirect(url_for('edit_profile')) # populate the forms when page is visited elif request.method == 'GET': form.username.data = current_user.username form.about_me.data = current_user.about_me return render_template('edit_profile.html', title='Edit Profile', form=form)
def edit_profile(): form = EditProfileForm(current_user.username) if form.validate_on_submit(): current_user.username = form.username.data current_user.about_me = form.about_me.data db.session.commit() flash('Your changes were updated') return redirect(url_for('index')) if request.method == 'GET': form.username.data = current_user.username form.about_me.data = current_user.about_me return render_template('edit_profile.html', form=form, title='Edit Profile')
def edit_profile(): form = EditProfileForm(current_user.username) if form.validate_on_submit(): current_user.username = form.username.data current_user.about_me = form.about_me.data db.session.commit() flash("Your changes have been saved") return redirect(url_for("edit_profile")) elif request.method == "GET": form.username.data = current_user.username form.about_me.data = current_user.about_me # we skip the else clause that is POST request with validation errors return render_template("edit_profile.html", title="Edit Profile", form=form)
def edit_profile(): form = EditProfileForm() if form.validate_on_submit(): current_user.username = form.username.data current_user.email = form.email.data db.session.commit() flash('Your changes have been saved.') return redirect(url_for('edit_profile')) elif request.method == 'GET': form.username.data = current_user.username form.email.data = current_user.email return render_template('edit_profile.html', title='Edit Profile', form=form)
def edit_profile(): form = EditProfileForm(current_user.username) if form.validate_on_submit(): current_user.username = form.username.data current_user.about_me = form.about_me.data db.session.commit() flash(_('Ваши изменения сохранены.')) return redirect(url_for('edit_profile')) elif request.method == 'GET': form.username.data = current_user.username form.about_me.data = current_user.about_me return render_template('edit_profile.html', title=_('Редактирование Профиля'), form=form, edit_profile_flg=False)
def edit_profile(): form = EditProfileForm(current_user.username) if form.validate_on_submit(): current_user.username = form.username.data current_user.about_me = form.about_me.data db.session.commit() flash('Your change have been saved.') return redirect(url_for('index')) elif request.method == 'GET': print('insid ethe leif of eidit _profile') form.username.data = current_user.username form.about_me.data = current_user.about_me return render_template('edit_profile.html', title='Edit Profile', form=form)
def edit(): form = EditProfileForm(g.user.nickname) if form.validate_on_submit(): g.user.nickname = form.nickname.data g.user.about_me = form.about_me.data g.user.email = form.email.data db.session.add(g.user) db.session.commit() flash('Your changes have been saved') return redirect(url_for('user', nickname=g.user.nickname)) else: form.nickname.data = g.user.nickname form.about_me.data = g.user.about_me form.email.data = g.user.email return render_template('edit.html', form=form)
def edit_profile(): '''个人资料修改接口''' form = EditProfileForm(current_user.username) if form.validate_on_submit(): current_user.username = form.username.data current_user.about_me = form.about_me.data db.session.commit() flash('修改成功') return redirect(url_for('edit_profile')) elif request.method == 'GET': form.username.data = current_user.username form.about_me.data = current_user.about_me return render_template('edit_profile.html', title='Edit Profile', form=form)
def edit_user(username): user = User.query.filter_by(username=username).first_or_404() form = EditProfileForm(current_user) if form.validate_on_submit(): current_user.username = form.username.data current_user.about_me = form.about_me.data db.session.commit() flash('Your changes have been saved.') return redirect(url_for('edit_user', username=current_user.username)) elif request.method == 'GET' and current_user.username is user.username: form.username.data = current_user.username form.about_me.data = current_user.about_me return render_template('edit_profile.html', title='Edit Profile', form=form, cancel=request.referrer)
def edit_profile(): form = EditProfileForm() if form.validate_on_submit(): f = form.photo.data filename = secure_filename(f.filename) f.save(os.path.join(app.config['PHOTO_PATH'], filename)) current_user.username = form.username.data current_user.photo = f.filename db.session.commit() return redirect(url_for('user', username=current_user.username)) elif request.method == 'GET': form.username.data = current_user.username return render_template('edit_profile.html', title='Edit Profile', form=form)
def edit_profile(): form = EditProfileForm(current_user.email, current_user.phone) if form.validate_on_submit(): current_user.first_name = form.first_name.data current_user.last_name = form.last_name.data current_user.email = form.email.data current_user.phone = form.phone.data db.session.commit() flash('تغییرات ذخیره شد') return redirect(url_for('user', first_name=current_user.first_name)) elif request.method == 'GET': form.first_name.data = current_user.first_name form.last_name.data = current_user.last_name form.email.data = current_user.email form.phone.data = current_user.phone return render_template('edit_profile.html', form=form)
def edit_profile(): form = EditProfileForm(current_user.username) if form.validate_on_submit(): current_user.username = form.username.data current_user.position_id = form.position.data.id current_user.about_me = form.about_me.data db.session.commit() flash('Your changes have been saved.') return redirect(url_for('user', username=current_user.username)) elif request.method == 'GET': form.username.data = current_user.username form.position.data = current_user.position_id form.about_me.data = current_user.about_me return render_template('edit_profile.html', title='Edit Profile', form=form)
def edit_profile(): edit_profile_form = EditProfileForm(orig_username=current_user.username) if edit_profile_form.validate_on_submit(): current_user.username = edit_profile_form.username.data current_user.about_me = edit_profile_form.about_me.data db_instance.session.commit() flash("Your changes have been saved") return redirect(url_for("user_profile", username=current_user.username)) elif request.method == "GET": edit_profile_form.username.data = current_user.username if current_user.about_me: edit_profile_form.about_me.data = current_user.about_me return render_template("edit_profile.html", title="Edit Profile", form=edit_profile_form)
def edit_profile(): form = EditProfileForm(current_user.username) if form.validate_on_submit(): # copy form values to user object # if validate_on_submit is false, it is because it was get request or # post with some data invalid current_user.username=form.username.data current_user.about_me = form.about_me.data db.session.commit() flash('Your changes have been saved.') return redirect(url_for('user', username=current_user.username)) elif request.method == 'GET': # pre-populate the input fields with values from the database form.username.data = current_user.username form.about_me.data = current_user.about_me return render_template('edit_profile.html', title='Edit Profile', form=form)
def edit_profile(): form = EditProfileForm(current_user.username) # submit으로 post 요청했을 경우 if form.validate_on_submit(): current_user.username = form.username.data current_user.about_me = form.about_me.data db.session.commit() flash('Your changes have been saved.') return redirect(url_for('edit_profile')) # 웹에 접속하여 GET 요청했을 경우 elif request.method == 'GET': form.username.data = current_user.username form.about_me.data = current_user.about_me return render_template('edit_profile.html', title='Edit Profile', form=form)
def edit_profile(): form = EditProfileForm() person = Person.query.filter_by(user_id=current_user.id).first_or_404() if form.validate_on_submit(): current_user.username = form.username.data person.name = form.name.data person.sex_id = form.sex.data db.session.commit() flash('Tus cambios han sido guardados.') return redirect(url_for('user', username=current_user.username)) elif request.method == 'GET': form.username.data = current_user.username form.name.data = person.name return render_template('edit_profile.html', title='Editar Perfil', form=form)
def edit_profile(): form = EditProfileForm(current_user.username) # form.profile_images.choices = [] if form.validate_on_submit(): current_user.username = form.username.data.lower() current_user.about_me = form.about_me.data current_user.first_name = form.first_name.data current_user.last_name = form.last_name.data db.session.commit() flash('Profile updated successfully.', category='success') return redirect('user/'+current_user.username) elif request.method == 'GET': form.username.data = current_user.username form.about_me.data = current_user.about_me return render_template('edit_profile.html', title='Edit Profile', form=form, user=user)
def edit_profile(): form = EditProfileForm(current_user.username) if form.validate_on_submit(): current_user.username = form.username.data current_user.about_me = form.about_me.data db.session.commit() flash('Dina ändringar har sparats.') return redirect(url_for('edit_profile')) elif request.method == 'GET': form.username.data = current_user.username form.about_me.data = current_user.about_me return render_template('edit_profile.html', drop_down_cats=drop_down_cats, title='Edit Profile', form=form, loggedin=True)
def edit_profile(): form = EditProfileForm() if form.validate_on_submit(): current_user.username = form.username.data current_user.about_me = form.about_me.data db.session.commit() flash('Your profile is updated !') return redirect(url_for('edit_profile')) elif request.method == 'GET': form.username.data = current_user.username form.about_me.data = current_user.about_me else: flash('Something went wrong!!!') return render_template('edit_profile.html', title="Edit Profile", form=form)
def edit_profile(): form = EditProfileForm(current_user.username) if form.validate_on_submit(): current_user.full_name = form.full_name.data current_user.username = form.username.data current_user.email = form.email.data current_user.about_me = form.about_me.data db.session.commit() flash('Your profile description has been updated!') return(redirect(url_for('edit_profile'))) elif request.method == 'GET': form.full_name.data = current_user.full_name form.username.data = current_user.username form.email.data = current_user.email form.about_me.data = current_user.about_me return(render_template('edit_profile.html', title='Edit Profile', form=form))
def edit_profile(): form = EditProfileForm() if form.validate_on_submit(): current_user.username = form.username.data current_user.about_me = form.about_me.data db.session.commit() flash('Your changes have been saved.') return redirect(url_for('edit_profile')) elif request.method == 'GET': ''' This elif clause is when a user is trying to edit the form. We present the current data from the db. ''' form.username.data = current_user.username form.about_me.data = current_user.about_me return render_template('edit_profile.html', title='Edit Profile', form=form)
def edit_profile(): form = EditProfileForm(current_user.username) if form.validate_on_submit(): # set current user data current_user.username = form.username.data current_user.about_me = form.about_me.data db.session.commit() flash('Your changes have been saved.') return redirect(url_for('edit_profile')) elif request.method == 'GET': # display the current profile (allows you to change username too form.username.data = current_user.username form.about_me.data = current_user.about_me return render_template('edit_profile.html', title='Edit Profile', form=form)
def edit_profile(): form = EditProfileForm(current_user.username) if form.validate_on_submit(): if form.picture.data: picture_fn = save_picture(form.picture.data) current_user.image = picture_fn current_user.username = form.username.data current_user.about_me = form.about_me.data db.session.commit() flash('Your changes have been saved.') return redirect(url_for('edit_profile')) elif request.method == 'GET': form.username.data = current_user.username form.about_me.data = current_user.about_me return render_template('edit_profile.html', title='Edit Profile', form=form, user=current_user)
def edit_profile(): form = EditProfileForm(current_user.username) if form.validate_on_submit(): print(4) current_user.username = form.username.data current_user.about_me = form.about_me.data db.session.commit() flash("Changes have been saved ") return redirect(url_for('edit_profile')) elif request.method == 'GET': print(5) form.username.data = current_user.username form.about_me.data = current_user.about_me return render_template('edit_profile.html', title="Edit Profile", form=form)
def edit_profile(): form = EditProfileForm(current_user.username) if form.validate_on_submit(): current_user.username = form.username.data current_user.telephone = form.telephone.data current_user.email = form.email.data db.session.commit() flash('Ваши изменения были сохранены.') return redirect(url_for('index')) elif request.method == 'GET': form.username.data = current_user.username form.telephone.data = current_user.telephone form.email.data = current_user.email return render_template('edit_profile.html', title='Редактирование профиля', form=form)
def edit_profile(): form = EditProfileForm(current_user.username) if form.validate_on_submit(): current_user.username = form.username.data current_user.about_me = form.about_me.data db.session.commit() flash("Your changes have been saved.") return redirect(url_for('edit_profile')) # prepopulating the form with database info elif request.method == 'GET': form.username.data = current_user.username form.about_me.data = current_user.about_me return render_template('edit_profile.html', title='Edit Profile', form=form)
def edit_profile(): form = EditProfileForm() if form.validate_on_submit(): #if the above test returns true, i copy the data from the form into the user object and then write #the object to the database. LOOK BELOW RETURN FOR MORE COMMENT current_user.username = form.username.data current_user.about_me = form.about_me.data db.session.commit() flash('Your changes have been saves') return redirect(url_for('edit_profile')) elif request.method == 'GET': form.username.data = current_user.username form.about_me.data = current_user.about_me return render_template('edit_profile.html', title='Edit Profile', form=form)
def edit_profile(): form = EditProfileForm(current_user.username) if form.validate_on_submit(): current_user.username = form.username.data current_user.about_me = form.about_me.data db.session.commit() flash('Your changes have been saved') elif request.method == "GET": form.username.data = current_user.username form.about_me.data = current_user.about_me return render_template('edit_profile.html', title="Edit Profile", form=form)
def edit_profile(): form = EditProfileForm() if form.validate_on_submit(): # Commit amended user information to db current_user.username = form.username.data current_user.about_me = form.about_me.data db.session.commit() flash('Your changes have been saved') return redirect(url_for('edit_profile')) elif request.method == 'GET': # Initial form display, pre-populate existing information form.username.data = current_user.username form.about_me.data = current_user.about_me return render_template( 'edit_profile.html', title='Edit Profile', form=form )
def test_validate_user_can_not_edit_existing_username(self): """>>>> Test the user can not register with an already taken username""" form = EditProfileForm(new_username=self.test_author2_username, about_me=ascii_letters * 4) self.assertFalse(form.validate_on_submit())
def test_validates_length_of_form(self): """>>>> Test that the edit profile form about me section is no more than 250 characters""" form = EditProfileForm(new_username=self.test_author1_username, about_me=ascii_letters * 5) self.assertFalse(form.validate_on_submit())